const ActivityCU = () => {
const [formInfo, setFormInfo] = useState({
values: { title: '', content: '' },
errors: { title: '제목은 필수 입력 값입니다.', content: '내용은 필수 입력 값입니다. ' },
touched: { title: false, content: false },
});
const handleBlur = (e) => {
let cpy = JSON.parse(JSON.stringify(formInfo));
cpy.touched[e.target.name] = true;
setFormInfo(cpy);
}
const handleChange = (e) => {
console.log(e);
let cpy = JSON.parse(JSON.stringify(formInfo));
let inputValue = e.target.value;
if (e.target.name === 'title') {
if (inputValue.length === 0) {
cpy.errors[e.target.name] = '제목은 필수 입력사항입니다.';
} else if (inputValue.length > 30) {
cpy.errors[e.target.name] = '제목은 30글자 이하 입력해주세요.';
} else {
cpy.errors[e.target.name] = '';
}
} else if (e.target.name === 'content') {
if (inputValue.length === 0) {
cpy.errors[e.target.name] = '내용은 필수 입력사항입니다.';
} else if (inputValue.length > 500) {
cpy.errors[e.target.name] = '내용은 500글자 이하 입력해주세요.';
} else {
cpy.errors[e.target.name] = '';
}
}
cpy.values[e.target.name] = inputValue;
setFormInfo(cpy);
}
const handleSubmit = (e) => {
e.preventDefault();
let cpy = JSON.parse(JSON.stringify(formInfo));
cpy.touched.title = true;
cpy.touched.content = true;
setFormInfo(cpy);
if (formInfo.errors.title === '' && formInfo.errors.content === '') {
alert('정상적으로 입력되었습니다. 서버로 전송합니다.');
}
}
return (
<section>
<h1>새 게시글 작성하기</h1>
<ActivityForm
onSubmit={handleSubmit}
>
<ActivityInputWrap>
<label htmlFor="title">게시글 제목</label>
<input
onBlur={handleBlur}
onChange={handleChange}
value={formInfo.values.title}
name='title'
id="title" />
{formInfo.touched.title && <p>{formInfo.errors.title}</p>}
</ActivityInputWrap>
<ActivityInputWrap>
<label htmlFor="writerEmail">작성자</label>
<input disabled value={'로그인한 사람 아이디'} id="writerEmail" />
</ActivityInputWrap>
<ActivityInputWrap>
<label htmlFor="content">게시글 내용</label>
<textarea
onBlur={handleBlur}
onChange={handleChange}
value={formInfo.values.content}
name='content'
id="content">
</textarea>
{formInfo.touched.content && <p>{formInfo.errors.content}</p>}
</ActivityInputWrap>
<button
onClick={handleSubmit}
type='button'
>글 등록하기</button>
</ActivityInputWrap>
</ActivityForm>
</section >
);
}
export default ActivityCU;
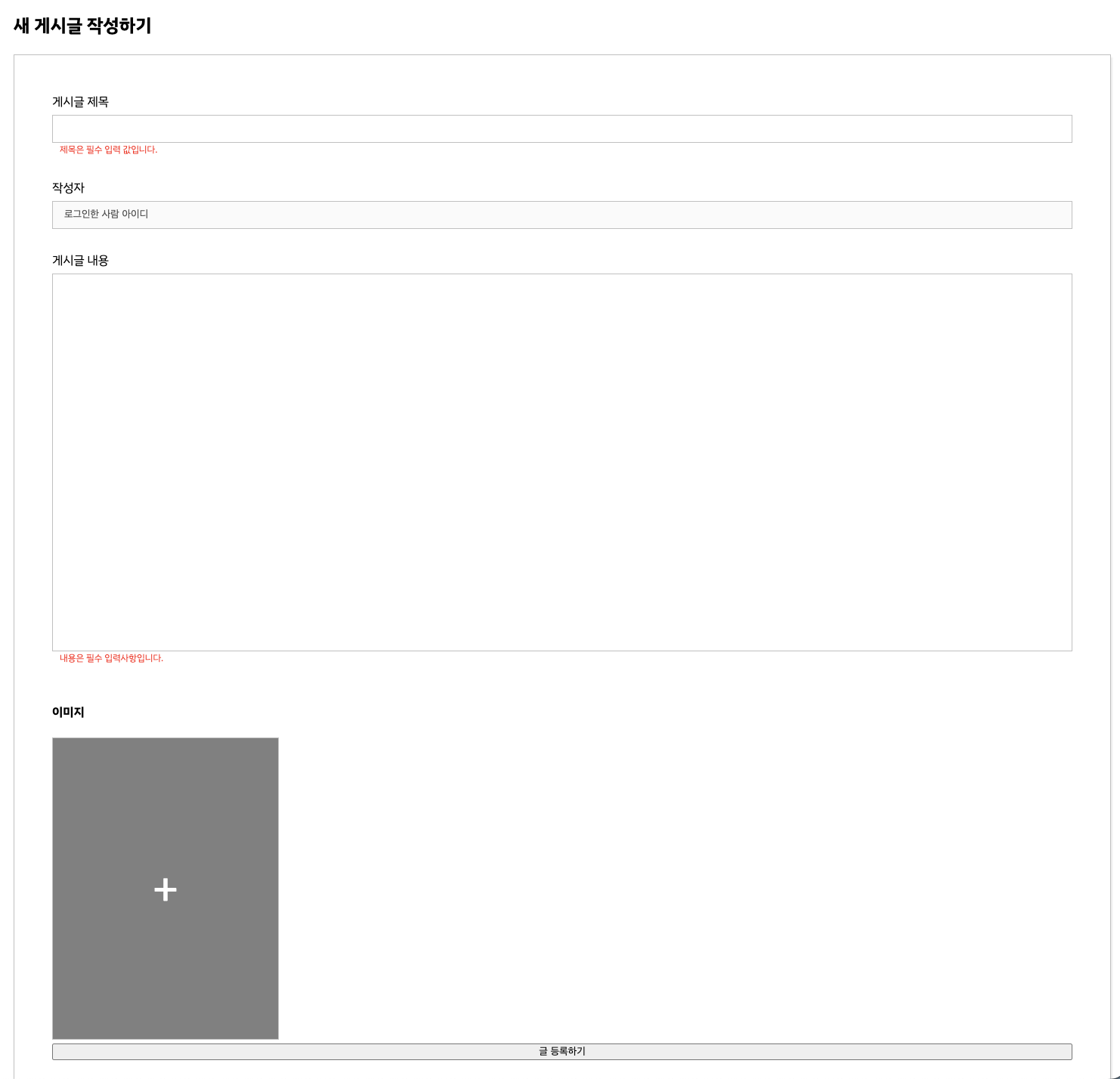