구조 분해 할당은 ES6의 등장과 함께 구현된 방식이다. 리액트에서 구조 분해를 사용하면 객체 안에 있는 필드 값을 원하는 변수에 대입할 수 있다. 코드의 가독성을 높이고 간략화를 할 때 유용하게 사용된다.
참고로 ES6에서 구조 분해는 다음과 같은 방식으로 사용됐다.
//배열 구조 분해
const points = [20, 30, 40];
const [x, y, z] = points;
console.log(x, y, z); // 20 30 40
//객체 구조 분해
// ES5
const car = {
type: 'Toyota',
color: 'Silver',
model: 2007
};
const type = car.type;
const color = car.color;
const model = car.model;
console.log(type, color, model); // Toyota Silver 2007
// ES6
const {type, color, model} = car;
console.log(type, color, model); // // Toyota Silver 2007
App.js와 그의 자식 컴포넌트인 Greet.js가 있다고 가정해보자.
// App.js
import React from 'react';
import Greet from './Greet'
class App extends React.Component {
render() {
return (
<div>
<Greet name="Diana" heroName="Wonder Woman" />
</div>
)
}
}
export default App
// Greet.js
import React from 'react'
const Greet = props => {
return (
<div>
<h1>
Hello {props.name} a.k.a {props.heroName}
</h1>
</div>
)
}
export default Greet
위의 경우처럼 자식 컴포넌트인 Greet.js에서 App 객체에서 정의한 값을 사용하려면 props.key를 이용해야 한다.
const Greet = ({ name, heroName }) => {
return (
<div>
<h1>
Hello {name} a.k.a {heroName}
</h1>
</div>
)
}
const Greet = props => {
const { name, heroName } = props;
return (
<div>
<h1>
Hello {name} a.k.a {heroName}
</h1>
</div>
)
}
클래스 컴포넌트에서는 props 또는 state를 보통 render()안에서 분해한다.
// App.js
import React from 'react';
import Welcome from './Welcome'
class App extends React.Component {
render() {
return (
<div>
<Welcome name="Bruce" heroName="Batman" />
</div>
)
}
}
export default App
// Welcome.js
class Welcome extends Component {
render() {
const { name, heroName } = this.props
return (
<h1>
Welcome {name} a.k.a {heroName}
</h1>
)
}
}
// Welcome.js
class Welcome extends Component {
render() {
const { name, heroName } = this.props
const { state1, state2 } = this.state
return (
<h1>
Welcome {name} a.k.a {heroName}
</h1>
)
}
}
```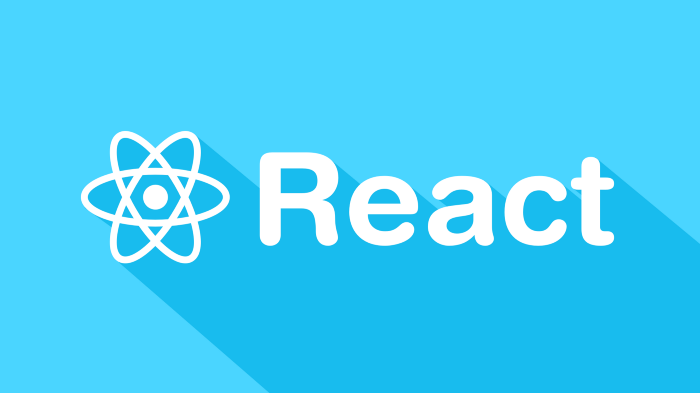
감사합니다 큰도움됐어요