1. 특정 문자 들어오기까지의 문자열 반환
- 사용자에게 ‘q’라는 문자가 들어오기 전까지 입력받아 모든 문자를 하나의 문자열로 바꾸는 프로그램
- 입력되는 문자는
알파벳, 숫자, 특수문자
모두 가능
- 예시
‘h’, ‘e’, ‘l’, ‘l’, ‘o’, ‘1’, ‘!’, ‘q’
→ “hello1!"
package me.day09.practice;
import java.util.Scanner;
public class Practice01 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
StringBuilder stringBuilder = new StringBuilder();
while (true) {
char ch = scanner.next().charAt(0);
if (ch == 'q') break;
stringBuilder.append(ch);
}
String answer = stringBuilder.toString();
System.out.println("answer = " + answer);
scanner.close();
}
}
2. reverse() 사용하지 않고 역순 저장하기
package me.day09.practice;
import java.util.Scanner;
public class Practice02 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
StringBuilder stringBuilder = new StringBuilder();
while (true) {
char ch = scanner.next().charAt(0);
if (ch == 'q') break;
stringBuilder.insert(0, ch);
}
String answer = stringBuilder.toString();
System.out.println("answer = " + answer);
scanner.close();
}
}
3. 유효성 검사하여 i번 입력받기
- 아래의 코드는 5명의 성적을 입력받아 합계와 평균을 구하는 프로그램이다.
- 성적 유효범위는 0 ≤ 성적 ≤ 100이라고 가정
- 성적 유효범위 이외의 데이터가 들어올 경우
유효하지 않은 성적 입력값입니다
를 출력하고 다시 입력받을 수 있도록 코드를 수정
package me.day09.practice;
import java.util.Scanner;
public class Practice03 {
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
final int NUM = 5;
double sum = 0;
double avg = 0;
for(int i = 0; i < NUM; i++) {
sum += inputScore(i);
}
avg = sum / NUM;
System.out.println("\n===========================");
System.out.println("sum = " + sum);
System.out.println("avg = " + avg);
}
public static double inputScore(int i) {
while (true) {
System.out.printf("%d 학생의 성적 입력: ", i + 1);
double score = sc.nextDouble();
if (!(score >= 0 && score <= 100)) {
System.out.println("유효하지 않은 성적 입력값입니다");
continue;
}
return score;
}
}
}
4. 이중 for문
- 우리가 가진 수는
1, 2, 3, 4, 5
가 있다고 가정하자.
- 해당 수 내에서 발생할 수 있는 조합의 쌍과 수를 출력하는 프로그램을 작성하시오.
- 예상 결과
(1, 2)
(1, 3)
(1, 4)
(1, 5)
(2, 3)
(2, 4)
(2, 5)
(3, 4)
(3, 5)
(4, 5)
package me.day09.practice;
public class Practice04 {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
for (int j = i + 1; j <= 5; j++) {
System.out.printf("(%d, %d)\n", i, j);
}
}
}
}
5. 삼중 for문
- 삼각형이 성립할 수 있는 조건은
a + b > c (가장 긴변)
이다.
- 삼각형이 될 수 있는
1 ≤ a, b, c ≤ 100
정수 중 피타고라스를 만족하는 (a, b, c) 쌍의 개수를 구하시오.
- 피타고라스 조건 :
a*a + b*b == c*c
package me.day09.practice;
public class Practice05 {
public static void main(String[] args) {
int count = 0;
for (int a = 1; a <= 100 ; a++) {
for (int b = a + 1; b <= 100 ; b++) {
for (int c = b + 1; c <= 100; c++) {
if (a * a + b * b == c * c) {
System.out.printf("(%d %d %d)\n", a, b, c);
count++;
}
}
}
}
System.out.println("count = " + count);
}
}
6. 별찍기
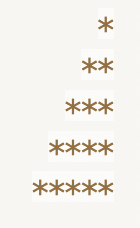
package me.day09.practice;
public class Practice06 {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 5; j++) {
if (i + j >= 6) System.out.print("*");
else System.out.print(" ");
}
System.out.println();
}
}
}
7. 무한루프 해결하기
package me.day09.practice;
public class Practice08 {
public static void main(String[] args) {
int i = 0;
while (i < 10) {
System.out.println(i);
i++;
}
int k = 1;
while (k <= 5) {
int l = 1;
while (l <= 5) {
System.out.print("*");
l++;
}
System.out.println();
k++;
}
}
}