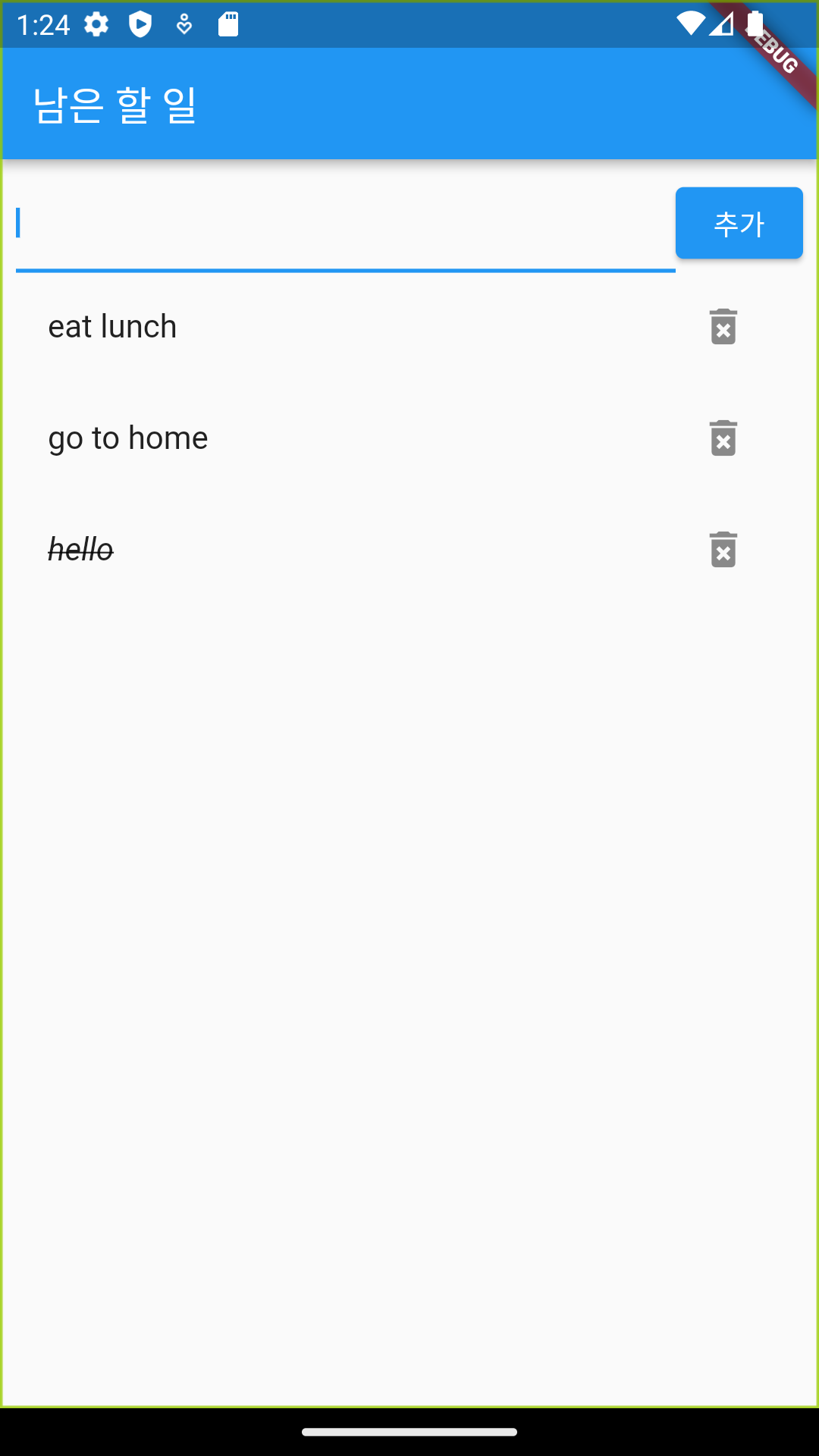
//Todo class
class Todo{
bool isDone = false;
String title;
Todo(this.title);
}
//MyApp class
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'To-do list',
theme: ThemeData(primarySwatch: Colors.blue),
home: TodoListPage(),
);
}
}
class TodoListPage extends StatefulWidget {
const TodoListPage({Key? key}) : super(key: key);
@override
State<TodoListPage> createState() => _TodoListPageState();
}
class _TodoListPageState extends State<TodoListPage> {
final _item = <Todo>[];
var _todoController = TextEditingController();
//할 일 추가
void _addTodo(Todo todo) {
setState(() {
_item.add(todo);
_todoController.text = '';
});
}
//할 일 삭제
void _deleteTodo(Todo todo) {
setState(() {
_item.remove(todo);
});
}
//할 일 완료
void _toggleTodo(Todo todo) {
setState(() {
todo.isDone = !todo.isDone;
});
}
@override
void dispose(){
_todoController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('남은 할 일'),
),
body: Padding(
padding: const EdgeInsets.all(8.0), //양방향 동일한 패딩
child: Column(
children: [
Row(
children: [
Expanded(child: TextField(
controller: _todoController,
),
),
ElevatedButton(
onPressed: () => _addTodo(Todo(_todoController.text)),
child: Text('추가')
)
],
),
Expanded(
child: ListView(
children: _item.map((todo) => _buildItemWidget(todo)).toList(),
),
)
],
)
)
);
}
Widget _buildItemWidget(Todo todo) {
return ListTile(
onTap: () => _toggleTodo(todo),
title: Text(
todo.title,
style: todo.isDone? const TextStyle( //삼항연산자, 스타일을 넣거나 안 넣거나 (isDone)
decoration: TextDecoration.lineThrough,
fontStyle: FontStyle.italic,
):null,
),
trailing: IconButton(
icon: const Icon(Icons.delete_forever),
onPressed: () => _deleteTodo(todo),
),
);
}
}