5. PLOTLY(반응형 그래프)
PLOTLY
- 인터랙티브 그래프 생성한다.
- dict 형식으로 명령어 작성한다.
- JSON 데이터 형식으로 저장한다.
- 다양한 방식으로 Export 가능하다.
- 한글 지원이 되어 따러 환경설정할 게 없다.
- 로그스케일을 사용하는 이유
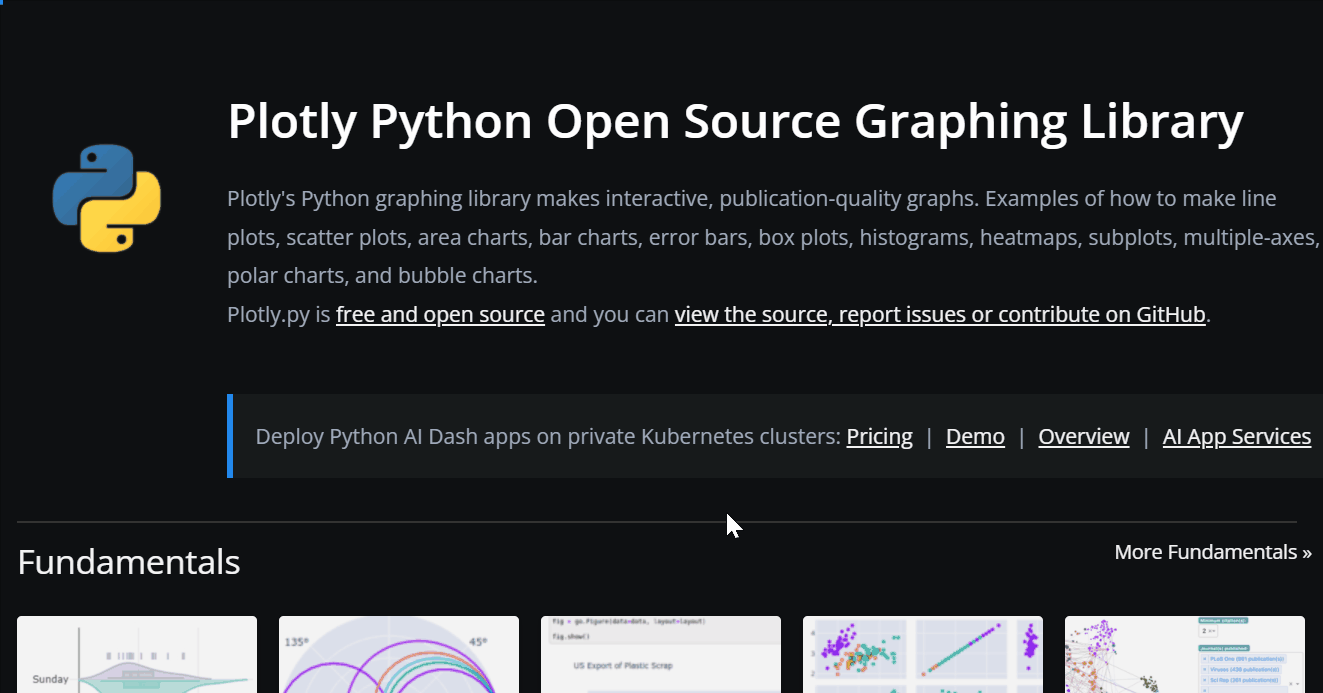
0) 환경설정
!pip install plotly
!pip install --upgrade plotly
import plotly.io as pio
import plotly.express as px
import plotly.graph_objects as go
import plotly.figure_factory as ff
from plotly.subplots import make_subplots
from plotly.validators.scatter.marker import SymbolValidator
import numpy as np
import pandas as pd
from urllib.request import urlopen
import json
0_1) 내보내기(저장)
with open('파일명.json', 'a') as f:
f.write(fig.to_json())
1) dict 형식으로 그리는 방법
fig = dict({
"data": [{"type": "bar",
"x": [1, 2, 3],
"y": [1, 3, 2]}],
"layout": {"title": {"text": "딕셔너리로 그린 그래프"}}
})
pio.show(fig)
2) Express를 통해서 그리는 방법
#tips
total_bill tip sex smoker day time size
0 16.99 1.01 Female No Sun Dinner 2
1 10.34 1.66 Male No Sun Dinner 3
2 21.01 3.50 Male No Sun Dinner 3
3 23.68 3.31 Male No Sun Dinner 2
4 24.59 3.61 Female No Sun Dinner 4
... ... ... ... ... ... ... ...
239 29.03 5.92 Male No Sat Dinner 3
240 27.18 2.00 Female Yes Sat Dinner 2
241 22.67 2.00 Male Yes Sat Dinner 2
242 17.82 1.75 Male No Sat Dinner 2
243 18.78 3.00 Female No Thur Dinner 2
fig1 = px.그래프명(tips,
x='tip',
y='total_bill',
color='sex',
size='tip',
marginal_x = 'box',
marginal_y = 'histogram',
trendline="ols",
trendline_color_override="grey",
hover_name='day',
hover_data=['day', 'size'],
title='Tips by Total Bill - Scatter Plot',
facet_col='day',
width=800,
height=600)
fig1.show()
3) Graph_objects를 통해서 그리는 방법
- Graph_objects의 약자인 go를 통해서 Figure객체를 선언하고 Figure내에 필요한 Data와 Layout등을 설정함
- 섬세한 커스터마이징이 가능
- 그래프를 겹쳐그릴 수 있음
- graph-objects
- Reference
A) fig
- go.Figure
- data : 데이터에 관한 정보
- layout : 제목, 폰트, 축, 범례 등 레이아웃 설정 정보
- go.update_layout : fig에 레이아웃 추가 업데이트
- go.add_trace : fig에 시각요소 추가 삽입 (subplot, map, 추가 그래프 등)
B) tips 데이터셋으로 실습
fig2 = go.Figure(
data=[go.Histogram(name ='Tips per Size',
x=tips['size'],
y=tips['tip'],
hoverlabel = dict(bgcolor = 'white'),
),
],
layout=go.Layout(
title='Tips 데이터 분석',
xaxis=dict(
title = '팁금액과 방문인원수',
titlefont_size=20,
tickfont_size=10),
yaxis=dict(
title= '전체 금액',
titlefont_size=15,
tickfont_size=10),
bargroupgap=0.3,
autosize=True))
fig2.show()
C) iris 데이터셋으로 실습
iris = px.data.iris()
iris
import plotly.graph_objects as go
fig = go.Figure(data=go.Scatter(
y = iris['sepal_length'],
mode='markers',
marker=dict(
size=20,
color=iris['petal_length'],
colorscale='Viridis',
showscale=True,
line_width=1,
)
))
fig.update_layout(title='Iris Data')
fig.show()
4) 그래프 종류
gapminder = px.data.gapminder()
gap2007 = gapminder[gapminder['year'] == 2007]
fig5 = px.scatter(gap2007,
x='gdpPercap',
y='lifeExp',
color='continent',
size='pop',
log_x=True,
size_max=40,
hover_name='country',
title='2007년의 대륙별 기대수명',
)
fig5.show()
.png)
gapAsia = gapminder[gapminder['continent']=='Asia']
fig = px.line(gapAsia,
x="year",
y="lifeExp",
color='country',
hover_name='country',
title='Life expectancy in Asia')
fig.show()
.png)
B_1) scatter + Line Chart
.png)
야후파이낸스 모듈 활용하기
a) 환경설정
!pip install yfinance
import pandas as pd
import numpy as np
import yfinance as yf
samsung = yf.download('005930.KS',
start='2001-11-12',
end='2022-03-31',
progress=False)
samsung = samsung.reset_index()
fig = px.line(samsung,
x="Date",
y="Close",
hover_name='Date',
range_x=['2020-01-01', '2022-03-29'],
title='samsung')
fig.show()
.png)
canada = gapminder[gapminder['country']=='Canada']
fig = px.bar(canada,
x='year',
y='pop',
hover_data=['gdpPercap', 'lifeExp'],
color='lifeExp',
title='Life expectancy in Canada',
width=600, height=600,
labels={'pop':'population of Canada for y', 'year':'year for x'}
)
fig.show()
.png)
D_1) Bar + animation
fig = px.bar(Asia, x='country', y='lifeExp', color='country',
animation_frame='year',
animation_group='country',
title='Life expectancy in Asia',
range_y=[28 ,100.603000])
fig.show()
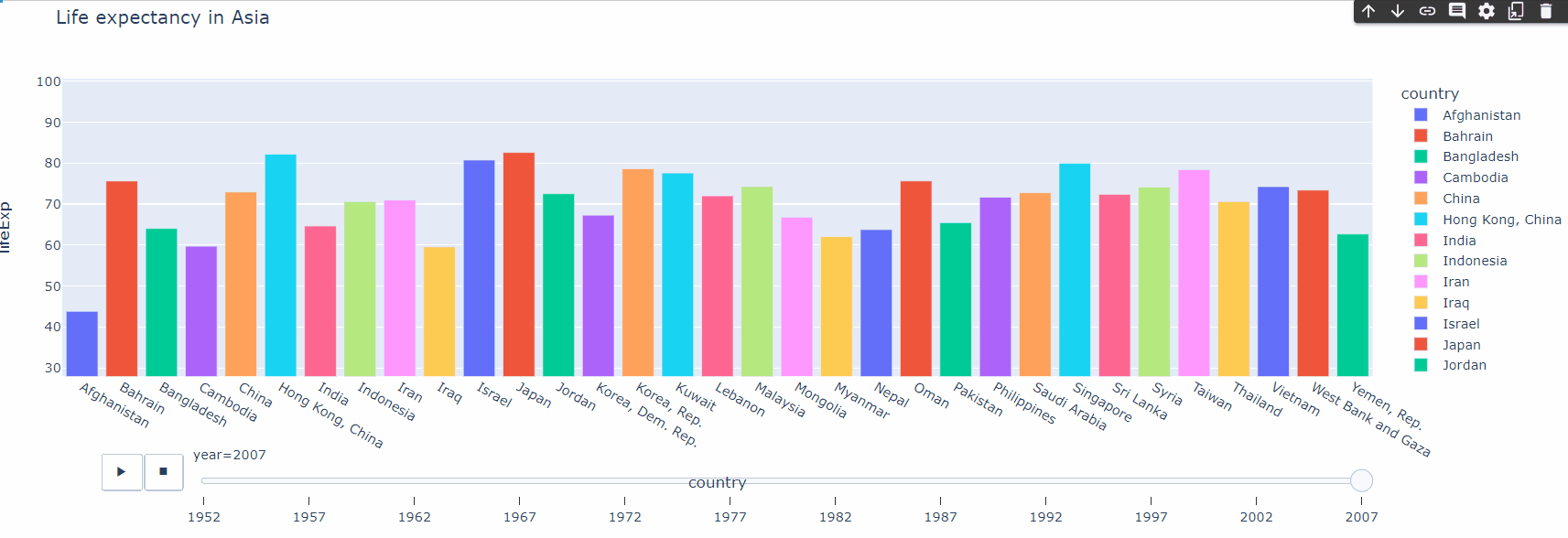
df = px.data.tips()
fig = px.box(df, x="time", y="total_bill", points='all')
fig.show()
.png)
fig = px.histogram(tips,
x='total_bill',
color='sex',
nbins=20, # 20개 묶음
opacity=0.6, #투명도 설정
color_discrete_sequence=['red', 'blue']) #컬러 각각 지정
fig.show()
.png)
※ query 이용하여 불린 인덱싱 대신하기
자료명.query('조건')
import plotly.express as px
import plotly
df = px.data.gapminder().query("year == 2007")
fig = px.treemap(df,
path=[px.Constant("all "),'continent','country'],
values='lifeExp',
color='lifeExp',
labels={'lifeExp':'기대수명'})
fig.update_layout(margin = dict(t=50, l=25, r=25, b=25))
fig.show()
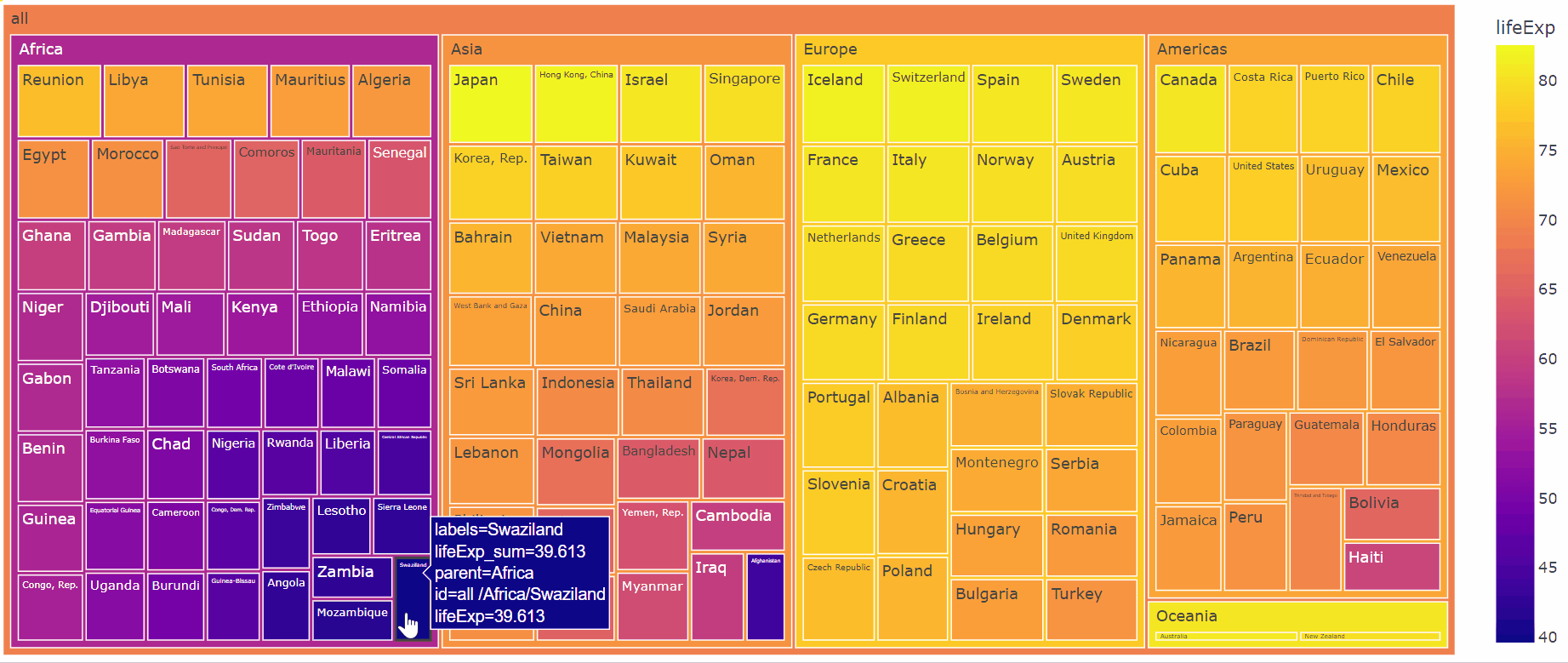
### E) [Sunburst Chart](https://plotly.com/python/sunburst-charts/)
```py
import plotly.express as px
import numpy as np
df = px.data.gapminder().query("year == 2007")
fig = px.sunburst(df, path=['continent', 'country'], values='pop',
color='lifeExp', hover_data=['iso_alpha'],
color_continuous_scale='RdBu',
color_continuous_midpoint=np.average(df['lifeExp'], weights=df['pop']))
fig.show()
```
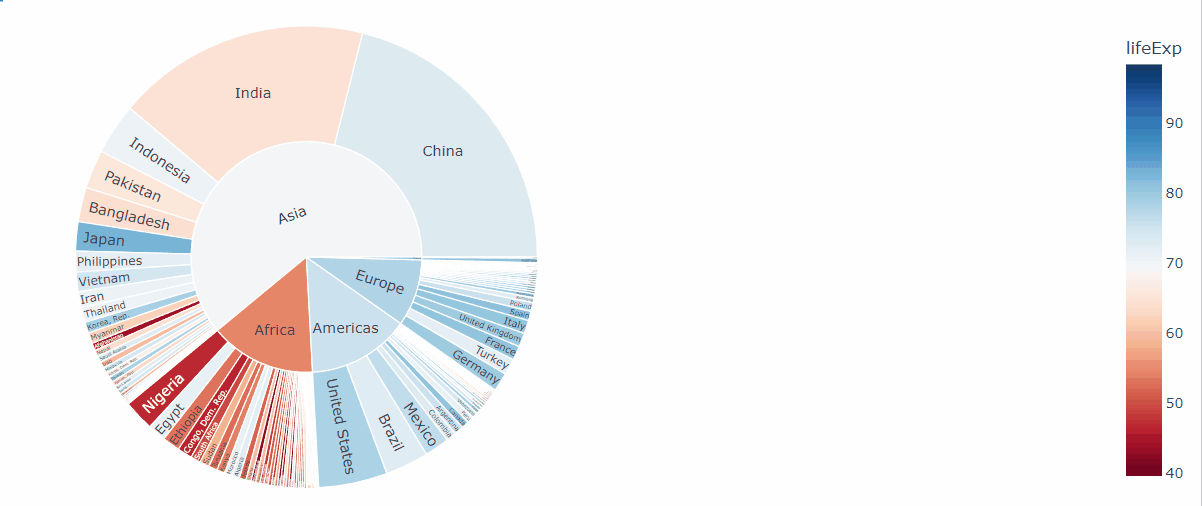
- 사용 가능한 템플릿
- 'plotly'(기본)
- 'ggplot2'
- 'seaborn'
- 'simple_white'
- 'plotly'
- 'plotly_white'
- 'plotly_dark'
- 'presentation'
- 'xgridoff'
- 'ygridoff'
- 'gridon'
- 'none'
import plotly.io as pio
import plotly.express as px
pio.templates.default = "plotly"
df = px.data.gapminder()
df_2007 = df.query("year==2007")
fig = px.scatter(df_2007,
x="gdpPercap", y="lifeExp", size="pop", color="continent",
log_x=True, size_max=60,
title="Gapminder 2007: current default theme")
fig.show()
a) 'plotly'(기본)
.png)
b) 'ggplot2'
.png)
c) 'plotly_dark'
.png)
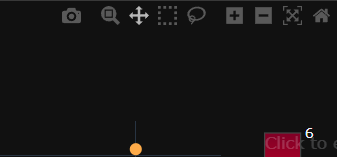
config = dict({'scrollZoom':True, 'displaylogo':False,'displayModeBar': True, 'editable':True, 'responsive': False, 'staticPlot': True})
B_1) 그리기 기능 넣기
fig.show(config={'modeBarButtonsToAdd':['drawline',
'drawopenpath',
'drawclosedpath',
'drawcircle',
'drawrect',
'eraseshape'
]})
C) 높이, 너비 및 여백 조정
fig.update_layout(title='Tips by Total Bill',
margin=dict(l=10, r=20, t=30, b=40, pad=2),
paper_bgcolor='yellow',
width=500, height=500)
fig.show(config=config)
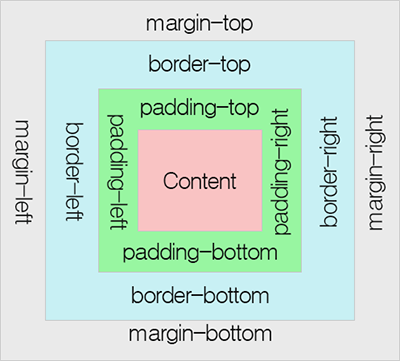
D) 글자 표현법
a) 날짜표현, 소숫점 자릿수 표현, 축 그리기
fig.update_layout(
xaxis_tickformat = '%d %B (%a)<br>%Y'
yaxis_tickformat = '.2%'
)
fig.update_xaxes(
showgrid=True,
ticks="outside",
tickson="boundaries",
ticklen=20
)
b) 라벨링
fig = px.scatter(iris, x='petal_length', y='petal_width', color='species',
labels={'petal_length':'Petal Length (cm)',
'petal_width':'Petal Width (cm)',
'species' : 'Species'})
c) 폰트 설정
fig.update_layout(
font_family="Courier New",
font_color="blue",
title_font_family="Times New Roman",
title_font_color="red",
legend_title_font_color="green"
)
- rows: 행의 갯수 (ex) 2)
- cols: 열의 갯수 (ex) 3)
- shared_xaxes: x축을 공유할지 여부 (True, False)
- shared_yaxes: y축을 공유할지 여부 (True, False)
- start_cell: 시작위치 정하기 (ex) "top-left")
- print_grid: 그리드 출력여부 (True, False)
- horizontal_spacing: 그래프간 가로 간격
- vertical_spacing: 그래프간 가로 간격
- subplot_titles: 플롯별 타이틀
- column_widths: 가로 넓이
- row_heights: 세로 넓이
- specs: 플롯별 옵션
- insets: 플롯별 그리드 레이아웃 구성
- column_titles: 열 제목
- row_titles: 행 제목
- x_title: x축 이름
- y_title: y축 이름
from plotly.subplots import make_subplots
import plotly.graph_objects as go
fig = make_subplots(rows=1, cols=2)
fig.add_trace(
go.Scatter(x=[1, 2, 3], y=[4, 5, 6]),
row=1, col=1
)
fig.add_trace(
go.Scatter(x=[20, 30, 40], y=[50, 60, 70]),
row=1, col=2
)
fig.update_layout(height=600, width=800, title_text="Side By Side Subplots")
fig.show()
fig = make_subplots(rows=3, cols=1)
fig.append_trace(go.Scatter(
x=[3, 4, 5],
y=[1000, 1100, 1200],
), row=1, col=1)
fig.append_trace(go.Scatter(
x=[2, 3, 4],
y=[100, 110, 120],
), row=2, col=1)
fig.append_trace(go.Scatter(
x=[0, 1, 2],
y=[10, 11, 12]
), row=3, col=1)
fig = make_subplots(rows=1, cols=2, column_widths=[0.7, 0.3])
fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6]),
row=1, col=1)
fig.add_trace(go.Scatter(x=[20, 30, 40], y=[50, 60, 70]),
row=1, col=2)
fig.show()