addProduct.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="/css/bootstrap.min.css">
<script type="text/javascript" src="/ckeditor/ckeditor.js"></script>
<title>상품등록</title>
</head>
<body>
<jsp:include page="/ch03/top.jsp" />
<div class="jumbotron">
<div class="container">
<h1 class="display-3">상품등록</h1>
</div>
</div>
<div class="container">
<form name="newProduct" action="addProduct_process.jsp" method="post" class="form-horizontal">
<div class="form-group row">
<label class="col-sm-2">상품코드</label>
<div class="col-sm-3">
<input type="text" name="productId" class="form-control" />
</div>
</div>
<div class="form-group row">
<label class="col-sm-2">상품명</label>
<div class="col-sm-3">
<input type="text" name="pname" class="form-control" />
</div>
</div>
<div class="form-group row">
<label class="col-sm-2">가격</label>
<div class="col-sm-3">
<input type="text" name="uniPrice" class="form-control" />
</div>
</div>
<div class="form-group row">
<label class="col-sm-2">상세정보</label>
<div class="col-sm-5">
<textarea name="description" class="form-control"></textarea>
</div>
</div>
<div class="form-group row">
<label class="col-sm-2">제조사</label>
<div class="col-sm-3">
<input type="text" name="menfacturer" class="form-control" />
</div>
</div>
<div class="form-group row">
<label class="col-sm-2">분류</label>
<div class="col-sm-3">
<input type="text" name="category" class="form-control" />
</div>
</div>
<div class="form-group row">
<label class="col-sm-2">재고수</label>
<div class="col-sm-3">
<input type="text" name="uniInStock" class="form-control"/>
</div>
</div>
<div class="form-group row">
<label class="col-sm-2">상태</label>
<div class="col-sm-5">
<input type="radio" name="condition" id="condition1" value="new" />
<label for = "condition1">신규제품</label>
<input type="radio" name="condition" id="condition2" value="Old" />
<label for = "condition2">중고제품</label>
<input type="radio" name="condition" id="condition3" value="Refurbished" />
<label for = "condition3">재생제품</label>
</div>
</div>
<div class="form-group row">
<div class="col-sm-offset-2 col-sm-10">
<input type="submit" class="btn btn-primary" value="등록" />
</div>
</div>
</form>
</div>
<script type="text/javascript">
CKEDITOR.replace("description")
</script>
<jsp:include page="/ch03/bottom.jsp" />
</body>
</html>
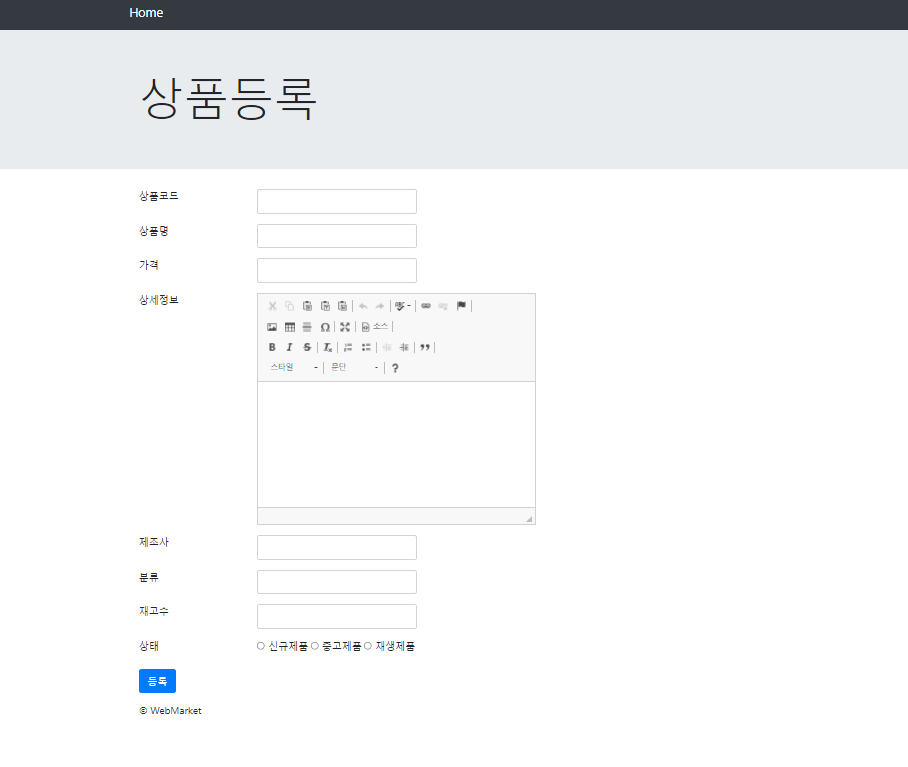
addProduct_process.jsp
<%@page import="dto.Product"%>
<%@page import="ch04.com.dao.ProductRepository"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
//1. request객체의 인코딩을 UTF-8로 설정
request.setCharacterEncoding("UTF-8");
//2. 파라미터 목록을 받자
String productId = request.getParameter ("productId");
String pname = request.getParameter ("pname");
String uniPrice = request.getParameter ("uniPrice");
String description = request.getParameter ("description");
String menfacturer = request.getParameter ("menfacturer");
String category = request.getParameter ("category");
String uniInStock = request.getParameter ("uniInStock");
String condition = request.getParameter ("condition");
//getParameter로 받으면 String으로 받게 되는데
price랑 stock은 int형이기 때문에 값이 있을 경우 int형으로 형변환 시키는것
Integer price;
if(uniPrice.isEmpty()){
price = 0;
}else{
price = Integer.valueOf(uniPrice);
}
long stock;
if(uniInStock.isEmpty()){
stock = 0;
}else{
stock = Long.valueOf(uniInStock);
}
// ProductRepository dao = new ProductRepository();
// 이렇게 하지 않고 싱글톤을 이용해 객체를 한번만 만들자!
//3. ProductRespository 클래스의 인스턴스 생성
ProductRepository dao = ProductRepository.getInstance();
//상품을 하나하나 넣고 있는것
Product newProduct = new Product();
newProduct.setProductId(productId);
newProduct.setPname(pname);
newProduct.setUniPrice(price);
newProduct.setDescription(description);
newProduct.setManufacturer(menfacturer);
newProduct.setCategory(category);
newProduct.setUnitsInStock(stock);
newProduct.setCondition(condition);
out.print("newProduct : " + newProduct.toString());
//4. 상품을 추가하자
//public void addProduct(Product product) {
dao.addProduct(newProduct);
//5. 상품 목록(products.jsp)으로 돌아가자.(추가된 상품이 보이게됨)
response.sendRedirect("products.jsp");
%>
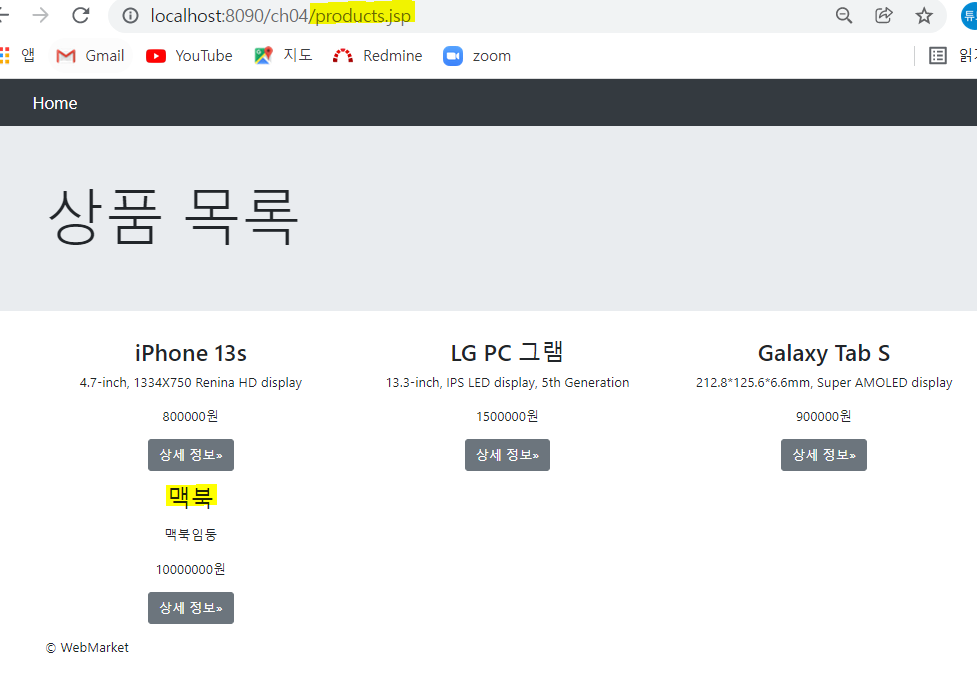
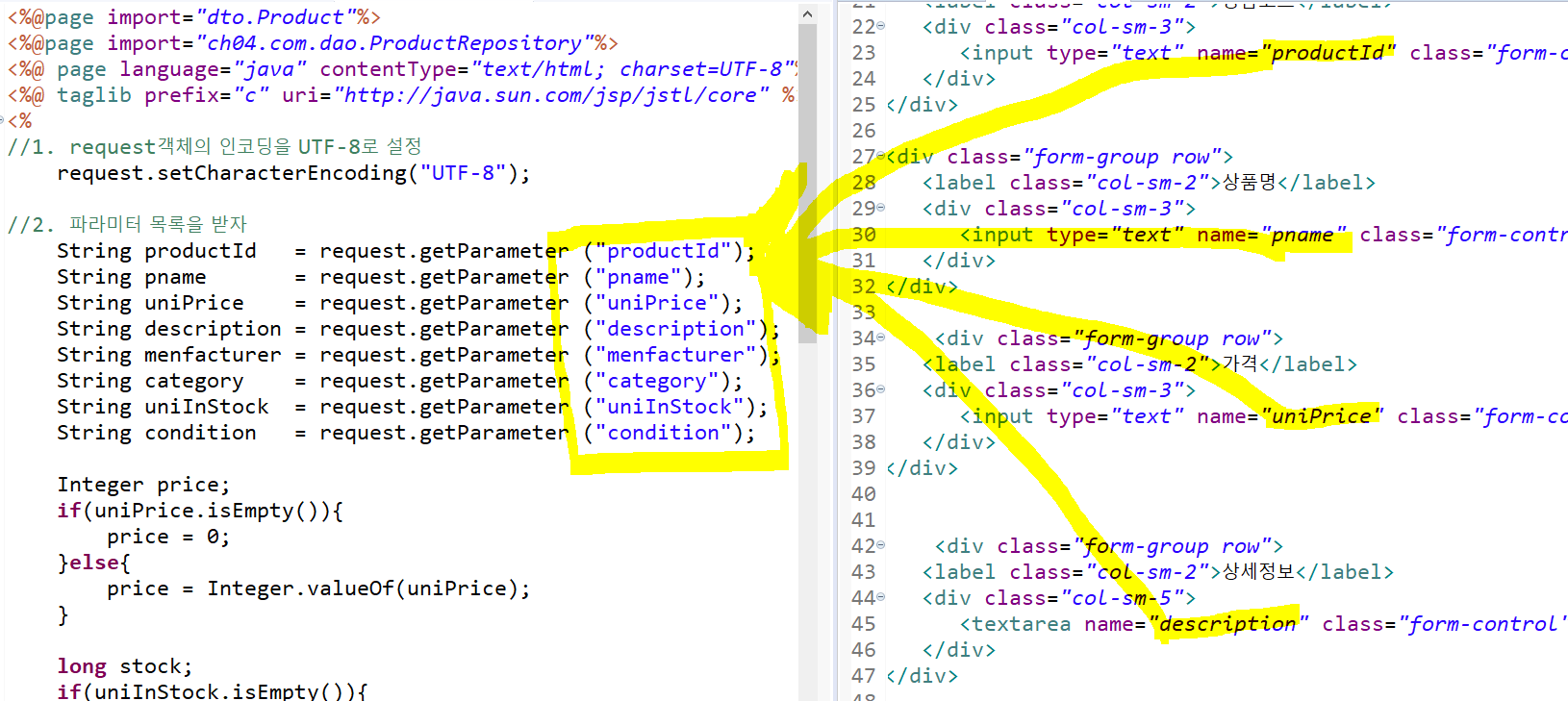
ProductRepository.java
package ch04.com.dao;
import java.util.ArrayList;
import java.util.List;
import dto.Product;
//자바빈즈로 사용할 상품 데이터 접근 클래스
public class ProductRepository {
private List<Product> listOfProducts = new ArrayList<Product>();
//싱글톤 패턴 만들기: 객체를 단 한번 만들어 공유해서 사용함(메모리 부하 최소화)
private static ProductRepository instance = new ProductRepository();
public static ProductRepository getInstance() {
return instance;
}
//생성자
public ProductRepository() {
Product phone = new Product("P1234", "iPhone 13s", 800000);
phone.setDescription("4.7-inch, 1334X750 Renina HD display");
phone.setCategory("Smart Phone");
phone.setManufacturer("Apple");
phone.setUnitsInStock(1000);
phone.setCondition("New");
Product notebook = new Product("P12345", "LG PC 그램", 1500000);
notebook.setDescription("13.3-inch, IPS LED display, 5th Generation");
notebook.setCategory("Notebook");
notebook.setManufacturer("LG");
notebook.setUnitsInStock(1000);
notebook.setCondition("Refurbished");
Product tablet = new Product("P1236", "Galaxy Tab S", 900000);
tablet.setDescription("212.8*125.6*6.6mm, Super AMOLED display");
tablet.setCategory("Tablet");
tablet.setManufacturer("Samsung");
tablet.setUnitsInStock(1000);
tablet.setCondition("Old");
//List<Product> 객체 타입의 변수에 저장
listOfProducts.add(phone);
listOfProducts.add(notebook);
listOfProducts.add(tablet);
}//end constructor
//객체 타입의 변수 listOfProducts에 저장된 모든 상품 목록을 가져옴
//SELECT * FROM PROD;
public List<Product> getAllProducts(){
return listOfProducts;
}
//상품 상세 정보를 가져오는 메소드(id = P1234)
//: 파라미터를 던져줘서 prduct타입으로 상품을 리턴
//SELECT * FROM PROD WHERE PROD_ID = 'P101000001';
public Product getProductById(String productId) {
//선언만..
Product productById = null;
//listOfProducts.size() : 3건(phone, notebook, tablet)
//List<Product> listOfProducts
for(int i=0; i<listOfProducts.size();i++) {
Product product = listOfProducts.get(i);
//등록된 상품이 있어야하고, 상품코드가 있어야하며,
//그때의 그 상품코드와 매개변수에 담긴 파라미터 상품코드가 같을때에만 실행
if(product != null && product.getProductId()!=null &&
product.getProductId().equals(productId)) {
//모두 트루일때 실행하는 조건문
productById = product;
break;
}//end if
}//end for
return productById;
}
//상품 추가 만들기
public void addProduct(Product product) {
//private List<Product> listOfProducts = new ArrayList<Product>();
listOfProducts.add(product);
}
}