실습1
package org.comstudy.day08;
class tv {
private int size;
public tv(int size) { this.size = size; }
protected int getSize() { return size; }
}
class colorTV extends tv{
private int Color;
public colorTV(int size, int Color) {
super(size);
this.Color = Color;
}
public void printProperty() {
System.out.println(super.getSize()+"인치 " + Color+"컬러");
}
}
public class ch5_pr1 {
public static void main(String [] args) {
colorTV myTV = new colorTV(32, 1024);
myTV.printProperty();
}
}
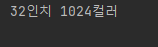
실습2
package org.comstudy.day08;
class TV {
private int size;
public TV() {}
public TV(int size) { this.size = size; }
protected int getSize() { return size; }
}
class ColorTV extends TV {
private int color;
public ColorTV(int size, int color) {
super(size);
this.color = color;
}
void printProperty() {
System.out.print(getSize()+"인치 "+ color+"컬러");
}
}
class IPTV extends ColorTV {
String ip;
public IPTV(String ip, int size, int color) {
super(size,color);
this.ip = ip;
}
void printProperty() {
System.out.print("나의 IPTV는 "+ip+" 주소의 ");
super.printProperty();
}
}
public class ch5_pr2 {
public static void main(String[] args) {
IPTV iptv = new IPTV("192.1.1.2", 32, 2048);
iptv.printProperty();
}
}

실습3
package org.comstudy.day08;
import java.util.Scanner;
abstract class Converter{
protected abstract double convert(double src);
protected abstract String getSrcString();
protected abstract String getDestString();
protected double ratio;
public void run() {
Scanner sc = new Scanner(System.in);
System.out.println(getSrcString() + "을 " + getDestString() + "로 바꿉니다.");
System.out.print(getSrcString() + "을 입력하세요>> ");
double val = sc.nextDouble();
double res = convert(val);
System.out.println("변환 결과: " + res + getDestString() + "입니다");
sc.close();
}
}
class Won2Dollar extends Converter{
public Won2Dollar(double ratio) {
this.ratio = ratio;
}
@Override
protected double convert(double src) {
return src/ratio;
}
@Override
protected String getSrcString() {
return "원";
}
@Override
protected String getDestString() {
return "달러";
}
}
public class ch5_pr3 {
public static void main(String[] args) {
Won2Dollar toDollar = new Won2Dollar(1200);
toDollar.run();
}
}
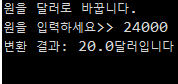
실습4
package org.comstudy.day08;
import java.util.Scanner;
abstract class ConVerter{
protected abstract double convert(double src);
protected abstract String getSrcString();
protected abstract String getDestString();
protected double ratio;
public void run() {
Scanner sc = new Scanner(System.in);
System.out.println(getSrcString() + "을 " + getDestString() + "로 바꿉니다.");
System.out.print(getSrcString() + "을 입력하세요>> ");
double val = sc.nextDouble();
double res = convert(val);
System.out.println("변환 결과: " + res + getDestString() + "입니다");
sc.close();
}
}
class Km2Mile extends ConVerter{
public Km2Mile(double ratio) {
this.ratio = ratio;
}
@Override
protected double convert(double src) {
return src/ratio;
}
@Override
protected String getSrcString() {
return "km";
}
@Override
protected String getDestString() {
return "mile";
}
}
public class ch5_pr4 {
public static void main(String[] args) {
Km2Mile toMile = new Km2Mile(1.6);
toMile.run();
}
}
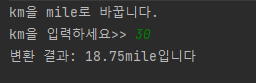
실습5
package org.comstudy.day08;
class Point{
private int x, y;
public Point(int x, int y) { this.x = x; this.y = y; }
public int getX() { return x; }
public int getY() { return y; }
protected void move(int x, int y) { this.x = x; this.y = y; }
}
class ColorPoint extends Point {
private String color;
public ColorPoint(int x, int y, String color) {
super(x,y);
this.color = color;
}
public void setXY(int x, int y) {
move(x,y);
}
public void setColor(String color) {
this.color = color;
}
public String toString() {
String str = color+"색의 ("+getX()+","+getY()+")의 점" ;
return str;
}
}
public class ch5_pr5 {
public static void main(String[] args) {
ColorPoint cp = new ColorPoint(5, 5, "YELLOW");
cp.setXY(10,20);
cp.setColor("RED");
String str = cp.toString();
System.out.println(str+ "입니다.");
}
}
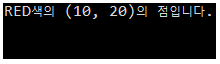
실습6
package org.comstudy.day08;
class point{
private int x, y;
public point(int x, int y) { this.x = x; this.y = y; }
public int getX() { return x; }
public int getY() { return y; }
protected void move(int x, int y) { this.x = x; this.y = y; }
}
class Colorpoint extends point {
private String color;
public Colorpoint() {
super(0,0);
this.color = "BLACK";
}
public Colorpoint(int x, int y) {
super(x,y);
this.color = "BLACK";
}
public void setXY(int x, int y) {
move(x,y);
}
public void setColor(String color) {
this.color = color;
}
public String toStr(){
String str = color+ "색의 ("+getX()+","+getY()+")의 점" ;
return str;
}
}
public class ch5_pr6 {
public static void main(String[] args) {
Colorpoint zeroPoint = new Colorpoint();
System.out.println(zeroPoint.toStr()+ "입니다.");
Colorpoint cp = new Colorpoint(10,10);
cp.setXY(5,5);
cp.setColor("RED");
System.out.println(cp.toStr()+ "입니다.");
}
}
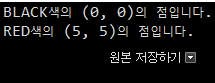
실습7
package org.comstudy.day08;
class Point3D extends Point {
int z;
public Point3D(int x, int y, int z) {
super(x, y);
this.z = z;
}
public void moveUp(){
z++;
}
public void moveDown() {
z--;
}
public void move(int x, int y, int z){
move(x,y);
this.z = z;
}
public String toString(){
return "(" + getX() + ", " + getY() + ", " + z + ")의 점";
}
}
public class ch5_pr7 {
public static void main(String[] args) {
Point3D p = new Point3D(1,2,3);
System.out.println(p.toString() + "입니다.");
p.moveUp();
System.out.println(p.toString() + "입니다.");
p.moveDown();
p.move(10,10);
System.out.println(p.toString() + "입니다.");
p.move(100,200,300);
System.out.println(p.toString() + "입니다.");
}
}
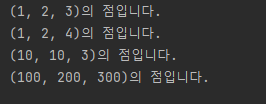
실습8
package org.comstudy.day08;
class PositivePoint extends Point{
public PositivePoint() {
super(0, 0);
}
public PositivePoint(int x, int y){
super(x,y);
if (getX()<0 || getY()<0){
move(0,0);
}
}
public void move(int x, int y){
if (x<0 || y<0){
return;
}else {super.move(x,y);}
}
public String toString(){
return "(" + getX() + ", " + getY() + ")의 점";
}
}
public class ch5_pr8 {
public static void main(String[] args) {
PositivePoint p = new PositivePoint();
p.move(10, 10);
System.out.println(p.toString() + "입니다.");
p.move(-5, 5);
System.out.println(p.toString() + "입니다.");
PositivePoint p2 = new PositivePoint(-10, -10);
System.out.println(p2.toString() + "입니다.");
}
}
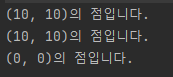
실습9
package org.comstudy21.day08;
import java.util.Scanner;
interface Stack {
int length();
int capacity();
String pop();
boolean push(String val);
}
class StringStack implements Stack{
private int num;
private int set;
private String[] stack;
public StringStack(int num) {
this.num = num;
this.set = 0;
stack = new String[num];
}
public int length() {
return set;
}
public int capacity() {
return stack.length;
}
public String pop() {
if(set-1<0)
return null;
set--;
String s = stack[set];
return s;
}
public boolean push(String val) {
if(set<num) {
stack[set] = val;
set++;
return true;
}else
return false;
}
}
public class ch5_pr9 {
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
System.out.print("총 스택 저장 공간의 크기 입력 >> ");
int a = sc.nextInt();
StringStack ss = new StringStack(a);
while(true) {
System.out.print("문자열 입력 >> ");
String val = sc.next();
if(val.equals("그만"))
break;
if(!ss.push(val)) {
System.out.println("스택이 꽉차서 푸시 불가!");
}
}
System.out.print("스택에 저장된 모든 문자열 팝 : ");
for(int i = 0; i<ss.capacity(); i++) {
String s = ss.pop();
System.out.print(s+" ");
}
}
}
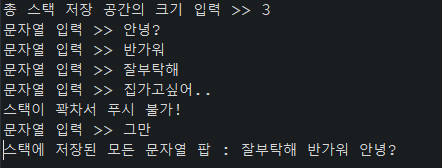
실습10
package org.comstudy21.day08;
abstract class PairMap{
protected String keyArray [];
protected String valueArray [];
abstract String get(String key);
abstract void put(String key, String value);
abstract String delete(String key);
abstract int length();
}
class Dictionary extends PairMap{
public Dictionary(int i) {
keyArray = new String[i];
valueArray = new String[i];
}
@Override
String get(String key) {
for(int i=0; i<keyArray.length; i++) {
if(keyArray[i] == key) {
return valueArray[i];
}
}
return null;
}
@Override
void put(String key, String value) {
for(int i=0; i<keyArray.length; i++) {
if(keyArray[i] == key) {
valueArray[i] = value;
break;
}
else if(keyArray[i] == null) {
keyArray[i] = key;
valueArray[i] = value;
break;
}
}
}
@Override
String delete(String key) {
String result = "";
for(int i=0; i<keyArray.length; i++) {
if(keyArray[i] == key) {
keyArray[i] = null;
result = valueArray[i];
valueArray[i] = null;
return result;
}
}
return null;
}
@Override
int length() {
int count = 0;
for(int i=0; i<keyArray.length; i++) {
if(keyArray[i] != null){
System.out.println(keyArray[i]);
count++;
}
}
return count;
}
}
public class ch5_pr10 {
public static void main(String[] args) {
Dictionary dic = new Dictionary(10);
dic.put("황기태", "자바");
dic.put("이재문", "파이썬");
dic.put("이재문", "C++");
System.out.println("이재문의 값은 " + dic.get("이재문"));
System.out.println("황기태의 값은 " + dic.get("황기태"));
dic.delete("황기태");
System.out.println("황기태의 값은 " + dic.get("황기태"));
}
}
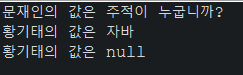
실습11
package org.comstudy.day09;
import java.util.Scanner;
abstract class Calc{
protected int a;
protected int b;
public void setValue(int a, int b) {
this.a = a;
this.b = b;
}
public abstract int calculate();
}
class Add extends Calc{
@Override
public int calculate() {
return a + b;
}
}
class SUB extends Calc{
@Override
public int calculate() {
return a - b;
}
}
class Mul extends Calc{
@Override
public int calculate() {
return a * b;
}
}
class Div extends Calc{
@Override
public int calculate() {
try {
return a / b;
}catch(Exception e){
if(a==0 || b==0) {
System.out.println("0으로 나눌수 없다.");
}
return 0;
}
}
}
public class ch5_pr11 {
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
System.out.print("두 정수와 연산자를 입력하시오>> ");
int x = sc.nextInt();
int y = sc.nextInt();
char operator = sc.next().charAt(0);
Calc calc;
switch(operator) {
case '+':
calc = new Add();
calc.setValue(x, y);
System.out.println(calc.calculate());
break;
case '-':
calc = new SUB();
calc.setValue(x, y);
System.out.println(calc.calculate());
break;
case '*':
calc = new Mul();
calc.setValue(x, y);
System.out.println(calc.calculate());
break;
case '/':
calc = new Div();
calc.setValue(x, y);
System.out.println(calc.calculate());
break;
}
}
}

실습12
package org.comstudy.day09;
import java.util.Scanner;
abstract class Shape {
private Shape next;
public Shape() {next = null; }
public void setNext(Shape obj) { next = obj; }
public Shape getNext() { return next; }
public abstract void draw();
}
class Line extends Shape{
@Override
public void draw() {
System.out.println("Line");
}
}
class Rect extends Shape{
@Override
public void draw() {
System.out.println("Rect");
}
}
class Circle extends Shape{
@Override
public void draw() {
System.out.println("Circle");
}
}
class Editor{
private Shape head;
private Shape tail;
public Editor(){
this.head = null;
this.tail = null;
}
public void Insert(int p) {
Shape tmp = null;
switch(p) {
case 1: p= new Line(); break;
case 2: p= new Rect(); break;
case 3: p= new Circle(); break;
default :
System.out.println("Error!");
break;
}
if(head==null) {
head = p;
tail = p;
}else {
tail.setNext(p);
tail = p;
}
}
public void Delete(int del) {
Shape current_node = head;
Shape p= head ;
if(head == null) {
System.out.println("삭제할 수 없습니다.");
}
if(del == 1) {
if(head==tail) {
head = null;
tail = null;
return;
} else {
head = head.getNext();
return;
}
}
else {
int i;
for(i = 1; i<del; i++) {
p = current_node;
current_node = current_node.getNext();
if(current_node == null) {
System.out.println("삭제할 수 없습니다.");
return;
}
}
if(current_node == tail ) {
tail = p;
tail.setNext(null);
}else {
p.setNext(current_node.getNext());
}
}
if(del < 1) {
System.out.println("Error!!");
}
}
public void printAll() {
Shape obj = head;
while(obj != null){
obj.draw();
obj = obj.getNext();
}
}
}
public class ch5_pr12 {
Scanner sc = new Scanner(System.in);
Editor ed = new Editor();
public ch5_pr12() {
System.out.println("그래픽 에디터 beauty을 실행합니다.");
}
public void run() {
while(true) {
System.out.print("삽입(1), 삭제(2), 모두 보기(3), 종료(4)>>");
int num = sc.nextInt();
switch(num){
case 1:
System.out.print("Line(1), Rect(2), Circle(3)>>");
int insert = sc.nextInt();
ed.Insert(insert);
break;
case 2:
System.out.print("삭제할 도형의 위치>>");
int del = sc.nextInt();
ed.Delete(del);
break;
case 3:
ed.printAll();
break;
case 4:
System.out.println("beauty을 종료합니다.");
return;
default:
System.out.println("잘못 된 값을 입력하셨습니다.");
break;
}
}
}
public static void main(String[] args) {
ch5_pr12 a = new ch5_pr12();
a.run();
}
}
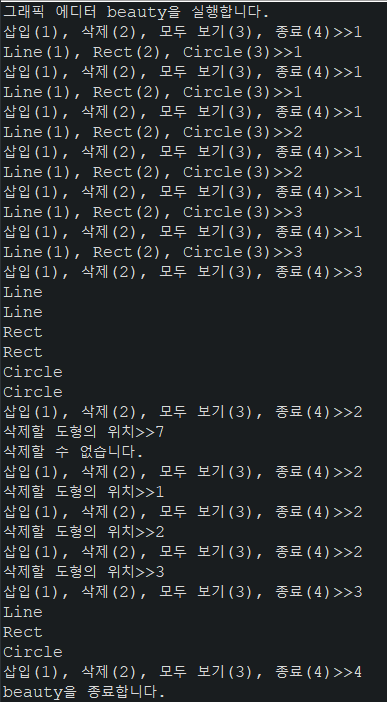
실습13
package org.comstudy.day09;
interface Shape2{
final double PI = 3.14;
void draw();
double getArea();
default public void redraw() {
System.out.print("--- 다시 그립니다. ");
draw();
}
}
class Circle2 implements Shape2{
private int i;
public Circle2(int i) { this.i = i; }
@Override
public void draw() {
System.out.println( "반지름이 " + i + "인 원입니다.");
}
@Override
public double getArea() {
return PI * Math.pow(i, 2);
}
}
public class ch5_pr13 {
public static void main(String[] args) {
Shape2 donut = new Circle2(10);
donut.redraw();
System.out.println("면적은 " + donut.getArea());
}
}

실습14
package org.comstudy.day09;
class Oval implements Shape2{
int x,y;
public Oval(int x, int y) {
this.x = x; this.y = y;
}
@Override
public void draw() {
System.out.printf("%d x %d에 내접하는 타원입니다. \n",x,y);
}
@Override
public double getArea() {
return PI * x * y;
}
}
class Rect2 implements Shape2{
int x,y;
public Rect2(int x, int y) {
this.x = x; this.y = y;
}
@Override
public void draw() {
System.out.printf("%d x %d크기의 사각형 입니다. \n",x,y);
}
@Override
public double getArea() {
return x * y;
}
}
public class ch5_pr14 {
public static void main(String[] args) {
Shape2 [] list = new Shape2[3];
list[0] = new Circle2(10);
list[1] = new Oval(20,30);
list[2] = new Rect2(10,40);
for(int i=0; i<list.length; i++) list[i].redraw();
for(int i=0; i<list.length; i++) System.out.println("면적은 " + list[i].getArea());
}
}
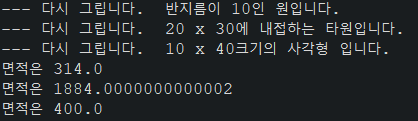