로또 번호 생성기
package org.comstudy.day04;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Random;
public class Lotto {
static Random rand = new Random();
public static void lotto1() {
final int SIZE = 6;
final int LENGTH = 45;
int[] lotto = new int[SIZE];
for(int i=0; i<SIZE; i++) {
lotto[i]= rand.nextInt(LENGTH)+1;
for(int j=0; j<i; j++) {
if(lotto[i] == lotto[j]) {
i--;
}
if(lotto[i]<lotto[j]) {
int tmp = lotto[i];
lotto[i] = lotto[j];
lotto[j] = tmp;
}
}
}
System.out.println(Arrays.toString(lotto));
}
public static void lotto2() {
final int SIZE = 6;
final int LENGTH = 45;
int index=0;
int[] lotto = new int[SIZE];
while(index!=6) {
lotto[index] = 1+(int)(Math.random()*45);
for(int i=0; i<index; i++) {
if(lotto[i] == lotto[index]) {
index--;
break;
}
}
index++;
}
System.out.println(Arrays.toString(lotto));
}
public static void lotto3() {
final int SIZE = 6;
final int LENGTH = 45;
int lotto[] = new int[SIZE];
for(int i =0; i<SIZE; i++) {
while(true) {
int tmp= rand.nextInt(LENGTH)+1;
boolean check = false;
for(int j=0; j<i; j++) {
if(lotto[j]==tmp) {
check = true;
break;
}else {
check = false;
}
}
if(check == false) {
lotto[i] = tmp;
break;
}
}
}
System.out.println(Arrays.toString(lotto));
}
public static void lotto4() {
List<Integer> numList = new ArrayList<>();
for (int i = 1; i <= 45; i++)
numList.add(i);
Collections.shuffle(numList);
numList = numList.subList(0, 6);
System.out.println(numList.toString());
}
public static void main(String[] args) {
lotto1();
lotto2();
lotto3();
lotto4();
}
}
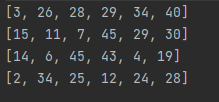
Chapter4 Open Challenge
package org.comstudy.day04;
import java.util.Scanner;
public class Chap4_OpenChallenge {
static Scanner sc = new Scanner(System.in);
static Scanner sc2 = new Scanner(System.in);
public static void main(String[] args) {
System.out.print("게임에 참가하는 인원은 몇명입니까? >> ");
int player_num = sc.nextInt();
Player[] player = new Player[player_num];
for(int i=0; i<player.length; i++){
System.out.print("참가자의 이름을 입력하세요 >> ");
String name = sc.next();
player[i] = new Player();
player[i].setName(name);
}
System.out.println("시작하는 단어는 아버지입니다.");
String word = "아버지";
boolean flag = true;
while (flag){
for(int i=0; i<player.length; i++){
System.out.print(player[i].getName()+">>");
String word2 = sc2.next();
int lastIndex = word.length() - 1;
char lastChar = word.charAt(lastIndex);
char firstChar = word2.charAt(0);
if(firstChar==lastChar){
word = word2;
}else{
System.out.println(player[i].getName()+"이(가) 졌습니다");
flag = false;
break;
}
}
}
}
}
class Player{
String name;
public void setName(String name) {
this.name = name;
}
public String getName(){
return name;
}
}
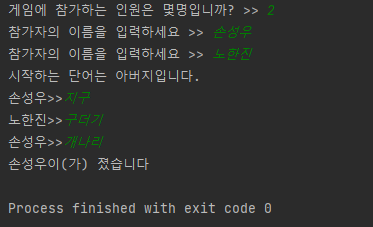
실습1
package org.comstudy.homework;
class TV {
String comm;
int year;
int inch;
public TV(String comm, int year, int inch) {
this.comm = comm;
this.inch = inch;
this.year = year;
}
public void show() {
System.out.printf("%s에서 만든 %d년형 %d인치 TV",comm,year,inch);
}
public static void main(String[] args) {
TV myTv = new TV("LG",2017,32);
myTv.show();
}
}

실습2
package org.comstudy.homework;
import java.util.Scanner;
class Grade {
int m_score;
int s_score;
int e_score;
public Grade(int m_score, int s_score, int e_score) {
this.m_score = m_score;
this.s_score = s_score;
this.e_score = e_score;
}
public int average() {
int a = (m_score+s_score+e_score)/3;
return a;
}
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
System.out.print("수학, 과학, 영어 순으로 3개의 점수 입력 >> ");
int math = sc.nextInt();
int science = sc.nextInt();
int english = sc.nextInt();
Grade me = new Grade(math,science,english);
System.out.println("평균은 " + me.average());
}
}

실습3
package org.comstudy.homework;
class Song {
String title;
String artist;
int year;
String country;
public Song() {}
public Song( String title, String artist, int year, String country){
this.title = title;
this.artist = artist;
this.year = year;
this.country = country;
}
public void show() {
System.out.printf("%d년 %s의 %s가 부른 %s",year, country, artist, title);
}
public static void main(String[] args) {
Song song = new Song("Dancing Queen","ABBA",1978,"스웨덴국적");
song.show();
}
}

실습4
package org.comstudy.homework;
class Rectangle {
int x;
int y;
int width;
int height;
public Rectangle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
public int square() {
return width*height;
}
public void show() {
System.out.printf("(%d,%d)에서 크기가 %dx%d인 사각형\n",x,y,width,height);
}
public boolean contains(Rectangle r) {
if(x+width >= r.x+r.width && y+height >= r.y+r.height) {
return true;
}else {
return false;
}
}
public static void main(String[] args) {
Rectangle r = new Rectangle(2,2,8,7);
Rectangle s = new Rectangle(5,5,6,6);
Rectangle t = new Rectangle(1,1,10,10);
r.show();
System.out.println("s의 면적은 " + s.square());
if(t.contains(r)) System.out.println("t는 r을 포함합니다.");
if(t.contains(s)) System.out.println("t는 s을 포함합니다.");
}
}
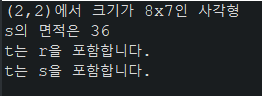
실습5
package org.comstudy.homework;
import java.util.Scanner;
class Circle {
private double x,y;
private int radius;
public Circle(double x, double y, int radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
public void show() {
System.out.printf("(%.1f,%.1f)%d\n",x,y,radius);
}
}
public class CircleEx5{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Circle[] c = new Circle[3];
for(int i=0; i<3; i++) {
System.out.print("x, y, radius >> ");
double x = sc.nextDouble();
double y = sc.nextDouble();
int radius = sc.nextInt();
c[i] = new Circle(x,y,radius);
}
for(int i=0; i<c.length; i++)
c[i].show();
sc.close();
}
}
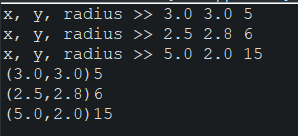
실습6
package org.comstudy.homework;
import java.util.Scanner;
class Circle {
private double x,y;
private int radius;
public Circle(double x, double y, int radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
public int getR() {
return radius;
}
public void show() {
System.out.printf("가장 면적이 큰 원은 (%.1f,%.1f)%d",x,y,radius);
}
}
public class CircleEx5{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Circle[] c = new Circle[3];
int max = 0;
for(int i=0; i<3; i++) {
System.out.print("x, y, radius >> ");
double x = sc.nextDouble();
double y = sc.nextDouble();
int radius = sc.nextInt();
c[i] = new Circle(x,y,radius);
}
for(int i=0; i<c.length; i++) {
if(c[max].getR() < c[i].getR())
max = i;
}
c[max].show();
sc.close();
}
}
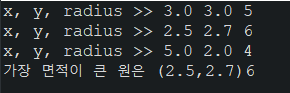
실습7
package org.comstudy.homework;
import java.util.Scanner;
class Day{
private String work;
public void set(String work) {
this.work = work;
}
public String get() {
return work;
}
public void show() {
if(work == null) System.out.println("없습니다.");
else System.out.println(work + "입니다.");
}
}
public class MonthSchedule {
static Scanner sc = new Scanner(System.in);
Day[] schedule = null;
boolean isday = true;
int num = 0;
public MonthSchedule(int day){
num = day;
schedule = new Day[num];
for(int i=0; i<schedule.length; i++) {
schedule[i] = new Day();
}
}
public void input() {
System.out.print("날짜(1~30)? ");
int day = sc.nextInt();
System.out.print("할일(빈칸없이입력)? >> ");
String work = sc.next();
schedule[day].set(work);
}
public void view() {
System.out.print("날짜(1~30)? ");
int day = sc.nextInt();
System.out.println(day + "일의 할 일은 " + schedule[day].get() + "입니다.");
}
public void finish() {
System.out.println("프로그램을 종료합니다.");
isday = false;
}
public void run() {
System.out.println("이번달 스케쥴 관리 프로그램.");
while(isday) {
System.out.print("할일(입력:1, 보기:2, 끝내기:3) >> ");
int pick = sc.nextInt();
switch(pick) {
case 1:
input();
break;
case 2:
view();
break;
case 3:
finish();
break;
}
System.out.println();
}
}
public static void main(String[] args) {
MonthSchedule april = new MonthSchedule(30);
april.run();
}
}
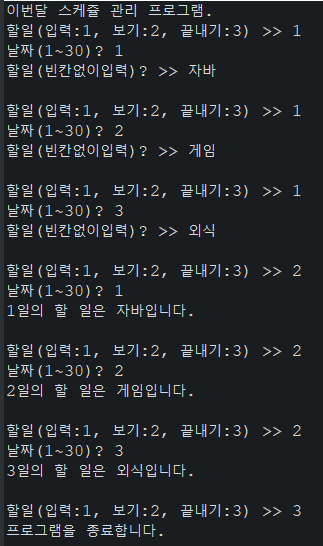
실습8
package org.comstudy.homework;
import java.util.Scanner;
class Phone{
private String name;
private String tel;
public Phone(String name, String tel) {
this.name = name;
this.tel = tel;
}
public String getName() {
return name;
}
public String getTel() {
return tel;
}
}
public class PhoneBook {
static Scanner sc = new Scanner(System.in);
Phone[] phone;
int num = 0;
public PhoneBook() {
System.out.print("인원수 >> ");
int num = sc.nextInt();
phone = new Phone[num];
}
public void input() {
for(int i=0; i<phone.length; i++) {
System.out.print("이름과 전화번호(이름과 번호는 빈 칸없이 입력) >> ");
String name = sc.next();
String tel = sc.next();
phone[i] = new Phone(name, tel);
}
System.out.println("저장되었습니다...");
}
public void find() {
while(true) {
boolean op1 = false;
System.out.print("검색할 이름 >> ");
String text = sc.next();
if(text.equals("그만"))
break;
for(int i=0; i<phone.length; i++) {
if(text.equals(phone[i].getName())) {
System.out.printf("%s의 번호는 %s 입니다.\n",text,phone[i].getTel());
op1=true;
}
}
if(op1==false) {
System.out.println(text+"이 없습니다.");
}
}
}
public static void main(String[] args) {
PhoneBook pb = new PhoneBook();
pb.input();
pb.find();
}
}
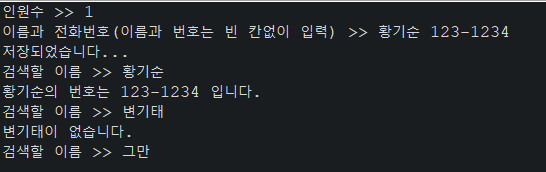
실습9
package org.comstudy.homework;
import java.util.Arrays;
class ArrayUtil{
public static int[] concat(int[] a, int[] b) {
int[] arr = new int[a.length+b.length];
for(int i=0; i<a.length; i++) {
arr[i] = a[i];
}
for(int j=0; j<b.length; j++) {
arr[a.length+j] = b[j];
}
return arr;
}
public static void print(int[] a) {
System.out.println(Arrays.toString(a));
}
}
public class staticEx {
public static void main(String[] args) {
int[] array1 = {1,5,7,9};
int[] array2 = {3,6,-1,100,77};
int[] array3 = ArrayUtil.concat(array1, array2);
ArrayUtil.print(array3);
}
}

실습10
package org.comstudy.homework;
import java.util.Scanner;
class Dictionary{
private static String[] kor = {"사랑", "아기", "돈", "미래", "희망" };
private static String[] eng = {"love", "baby", "money", "future", "hope" };
public static String kor2Eng(String word) {
for(int i = 0; i<kor.length; i++) {
if(kor[i].equals(word)) {
return eng[i];
}
}
return word+"은(는) 사전에 없습니다.";
}
}
class DictApp{
void run(){
System.out.println("한영 단어 검색 프로그램입니다.");
while(true) {
Scanner sc = new Scanner(System.in);
System.out.print("한글단어? >> ");
String text = sc.next();
if(text.equals("그만"))
break;
System.out.println(Dictionary.kor2Eng(text));
}
}
}
public class ch4_ex10 {
public static void main(String[] args) {
DictApp da = new DictApp();
da.run();
}
}
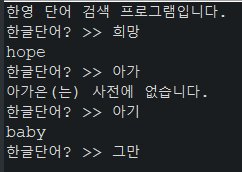
실습11
package org.comstudy.homework;
import java.util.Scanner;
class Add{
int a,b;
int res;
void setValue(int a, int b){
res = a+b;
}
int calculate() {
return res;
}
}
class Sub{
int a,b;
int res;
void setValue(int a, int b){
res = a-b;
}
int calculate() {
return res;
}
}
class Mul{
int a,b;
int res;
void setValue(int a, int b){
res = a*b;
}
int calculate() {
return res;
}
}
class Div{
int a,b;
int res;
void setValue(int a, int b){
res = a/b;
}
int calculate() {
if (b!=0)
return a/b;
else
return a/(b+1);
}
}
public class ch4_Ex11 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("두 정수와 연산자를 입력하시오 >> ");
int a = sc.nextInt();
int b = sc.nextInt();
char c = sc.next().charAt(0);
if(c=='+') {
Add add = new Add();
add.setValue(a, b);
System.out.println(add.calculate());
}else if(c =='-') {
Sub sub = new Sub();
sub.setValue(a, b);
System.out.println(sub.calculate());
}else if(c=='*') {
Mul mul = new Mul();
mul.setValue(a, b);
System.out.println(mul.calculate());
}else if(c=='/') {
Div div = new Div();
div.setValue(a, b);
System.out.println(div.calculate());
}
}
}
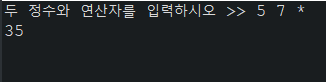