What is Graphics Pipeline?
The Graphics Pipeline is a sequence of processes, called Stages, that run on graphics hardware. We push data into the pipeline which runs the data through these stages to get a final 2D image representing the 3D scene.
⇒ 3차원 환경을 2차원 이미지로 표현하기 위한 stage
- Fixed Function : the pipeline stages can be configured
- Programmable : the pipeline stages can be programmed
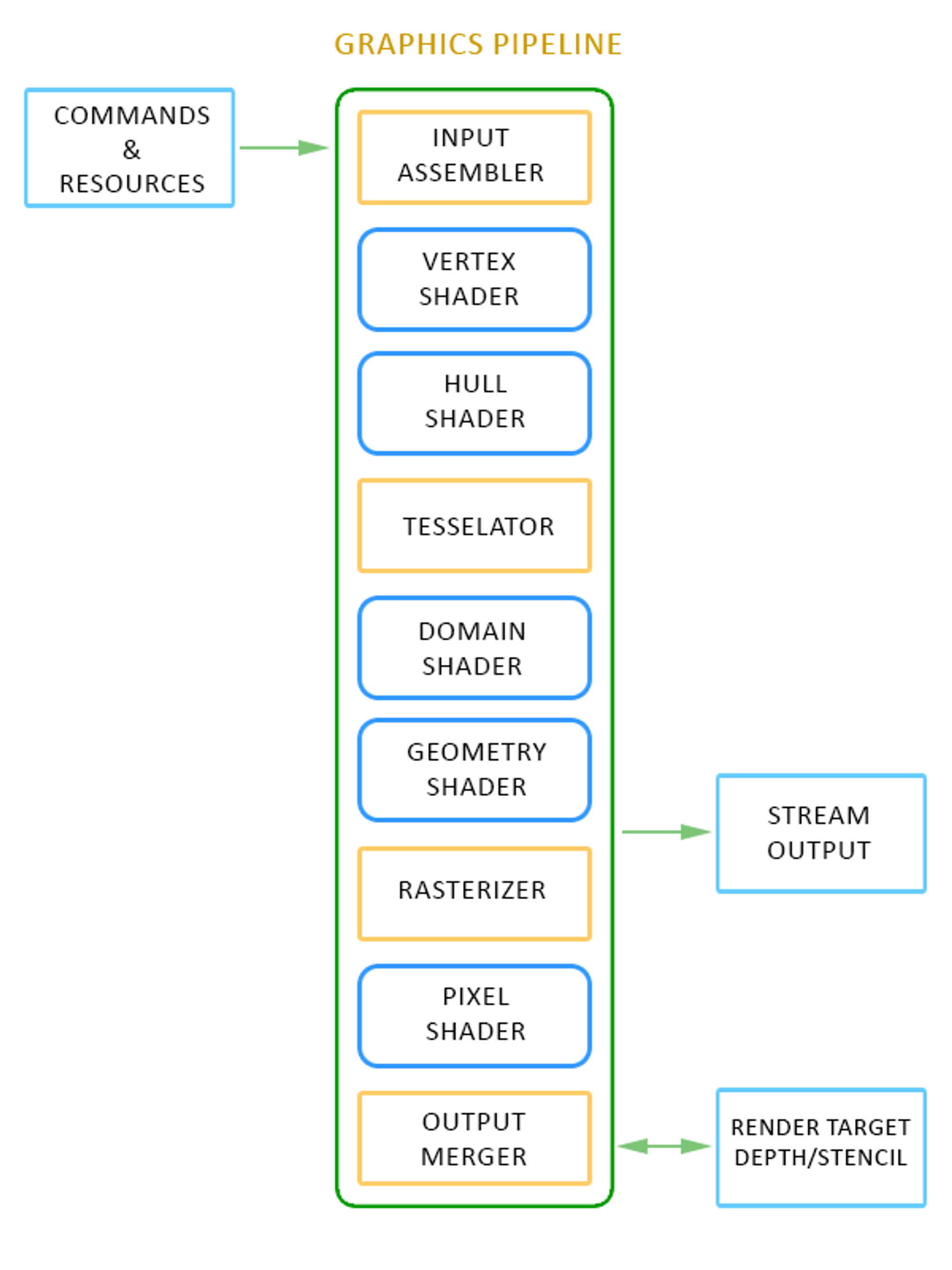
- fixed function stage → we do not do the programming to implement it
- 정점 데이터를 읽는 방법을 알 수 있도록 IA에 Input Layout을 제공
- primitives로 data를 assemble한 후 pipeline에 이 primitives를 공급
- string의 형태의 system generated values를 primitives에 부착 → 이러한 value를 Semantics라 함
D3D12_INPUT_ELEMENT_DESC layout[] =
{
{ "POSITION", 0, DXGI_FORMAT_R32G32B32_FLOAT, 0, 0, D3D12_INPUT_PER_VERTEX_DATA, 0 },
};
→ IA that each vertex in the vertex buffer has one element
Vertex Shader(VS) Stage
이전의 입력 어셈블러로부터 받은 정점을 처리하여 변환하거나 정점별로 조명을 적용하는 등, 정점별로 작업을 수행하는 단계
- programmable stage
- transformation, scaling, lighting, displacement mapping for textures and stuff like that
- VS는 프로그램의 vertex를 수정할 필요가 없더라도 파이프라인이 작동하기 위해 항상 구현되어야 함 → 가장 간단한 형태의 VS는 그냥 다음 담계로 vertex position을 넘겨준다
float4 main(float4 pos : POSITION) : SV_POSITION
{
return pos;
}
Tesselataoion Stages
- the Hull Shader, Tessellator, and the Domain Shader stages는 tesselation을 만들기 위해 함께 일한다.
- Tesselation이 하는 일은 primitive object(삼각형, 선)를 여러 개의 작은 부분으로 나누어 모델의 디테일을 증가시키며 매우 빠르다
- screen에 보여지기 전에 GPU에 생성하고 메모리에 저장되지 않는다.
→ 메모리에 저장이 되어야만 하는 CPU에서 생성하는 것보다 많은 시간이 절약된다.
- tesselation을 사용하여 low poly model → highly detailed polly
테셀레이션은 표면을 일정한 형태의 기하도형으로 나눠야 하는데, 입력으로 들어온 삼각형이나 선에 해당하는 기하 도형을 생성하는 단계
Hull Shader(HS) Stage
- the first of Tesselation Stages & programmable stage
- primitive 를 더 디테일하게 만들기 위해 새로운 vertices를 추가하는 방법과 위치를 계산하는 단계
- 그 후 data를 the Tessellator Stage and the Domain Shader Stage로 전송한다.
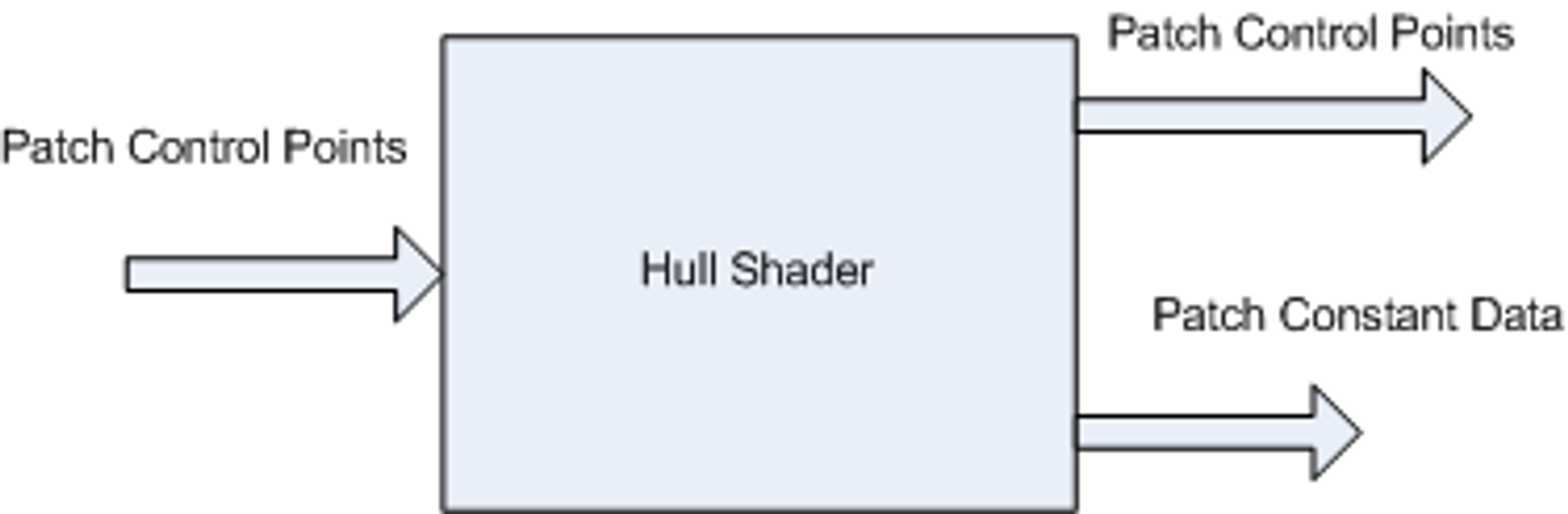
Tessellator (TS) Stage
기하 도형을 나타내는 도메인의 샘플링 패턴을 만들고, 더욱 작은 삼각형이나 점 혹은 선과같은 개체 집합을 생성하여 샘플을 연결하는 단계이다.
- the second stage of Tesselation process & Fixed Function stage
- primitive들을 더 작게 나누는 것
- 도메인(사각형, 삼각형 또는 선)을 여러 개의 더 작은 개체(삼각형, 점 또는 선)로 세분화하는 것
Domain Shader (DS) Stage
각 도메인 샘플에 해당하는 정점의 위치를 계산하는 단계이다.
- the third stage of Tesselation process & Programmable Function stage
- Hull Shader Stage로부터 새 vertices의 위치를 취하고, Tesselator Stage로 vertices를 변환하여 더 상세하게 만든다.
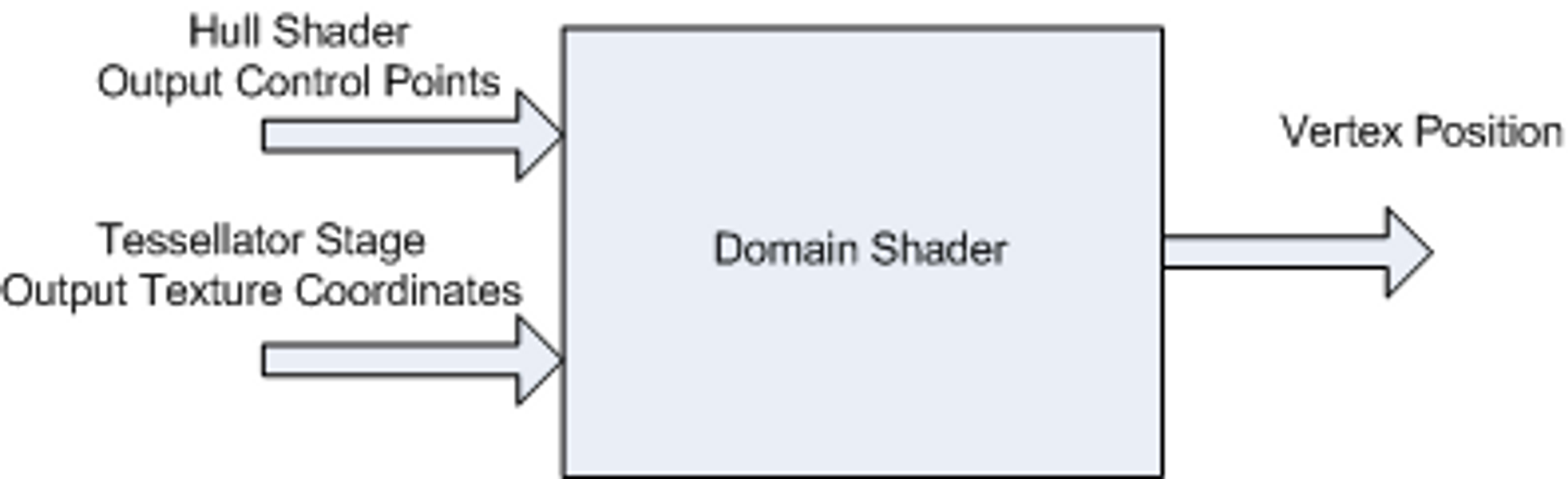
Geometry Shader (GS) Stage
- Programmable Function Stage
- accept primitives as input → 3 vertices for triangles, 2 for lines, and one for a point.
- create or destroy primitives, where the VS cannot → We could turn one point into a quad or a triangle with this stage, which makes it perfect for use in a particle engine for example
Stream Output (SO) Stage
- Geometry Shader Stage or Vertex Shader Stage가 없는 경우 pipeline에서 vertex data를 얻기 위해 사용
- SO에서 메모리로 보내진 vertex data는 하나 이상의 buffer에 저장됨
- SO로부터의 Vertex data output : lists (line lists or triangle lists)
- Incomplete primitives are NEVER sent out
→ Incomplete primitives are primitives such as triangles with only 2 vertices or a line with only one vertex.
Rasterizer Stage (RS)
- 3D 그래픽을 실시간으로 구성하기 위해 벡터 정보를 픽셀로 구성되는 래스터 이미지로 변환하는 단계이다.
- During rasterization
- 입력으로 들어온 primitive 들은 pixel로 변환이 되며 각각의 정점별 값을 보간하게된다.
- clipping vertices to the view frustum, performing a divide by z to provide perspective, mapping primitives to a 2D viewport, and determining how to invoke the pixel shader
- While using a pixel shader is optional, always performs
→ clipping
→ a perspective divide to transform the points into homogeneous space
→ maps the vertices to the viewport.
✔clipping
→ 화면에 보이지 않는 부분은 그리지 않는 것
Pixel Shader (PS) Stage
- Programmable stage & optional stage
- 픽셀별 조명 및 후처리와 같은 화면에 표시되는 각 픽셀을 계산하고 수정하여 풍부한 음영 기술을 사용할 수 있게 하는 단계
- Pixel shader
constant variables, texture data, interpolated per-vertex values와 다른 데이터를 조합하여 per-pixel 출력을 산출하는 프로그램
- 하는 일 : 각 픽셀 조각의 최종 색상을 계산하는 것
- pixel fragment : 스크린에 그려질 잠재적 pixel
ex) 원 뒤에 사각형 픽셀이 있을 때, 최종적으로 화면에 표시되는 것은 depth가 더 작은 원의 픽셀이다.
Output Merger (OM) Stage
OM은 파이프라인 상태, 픽셀 셰이더에서 생성된 픽셀 데이터, 렌더링 대상의 내용 및 깊이/스텐실 버퍼의 내용을 조합하여 최종 렌더링된 픽셀 색을 생성합니다.
- pixel fragments and depth/stencil buffer 를 사용하여 최종적으로 렌더링 대상에 기록되는 픽셀 색을 생성
- OM Stage는 표시되는 픽셀(depth-stencil testing 포함)을 결정하고 최종 픽셀 색을 혼합하기 위한 마지막 단계
- render target은 OM에 바인딩하는 Texture 2D 리소스이다.
Depth-Stencil Testing
texture resource로 생성되는 depth-stencil buffer는 depth data와 stencil data를 모두 포함
- depth data : 카메라에 가장 가까운 픽셀을 결정하는 데 사용
- stencil data : 업데이트할 수 있는 픽셀을 마스크하는 데 사용
⇒ output-merger stage는 depth & stencil values data을 모두 사용하여 픽셀을 그려야 하는지 여부를 결정. 다음 다이어그램에서는 Depth-Stencil Testing를 수행하는 방법을 개념적으로 보여 준다.
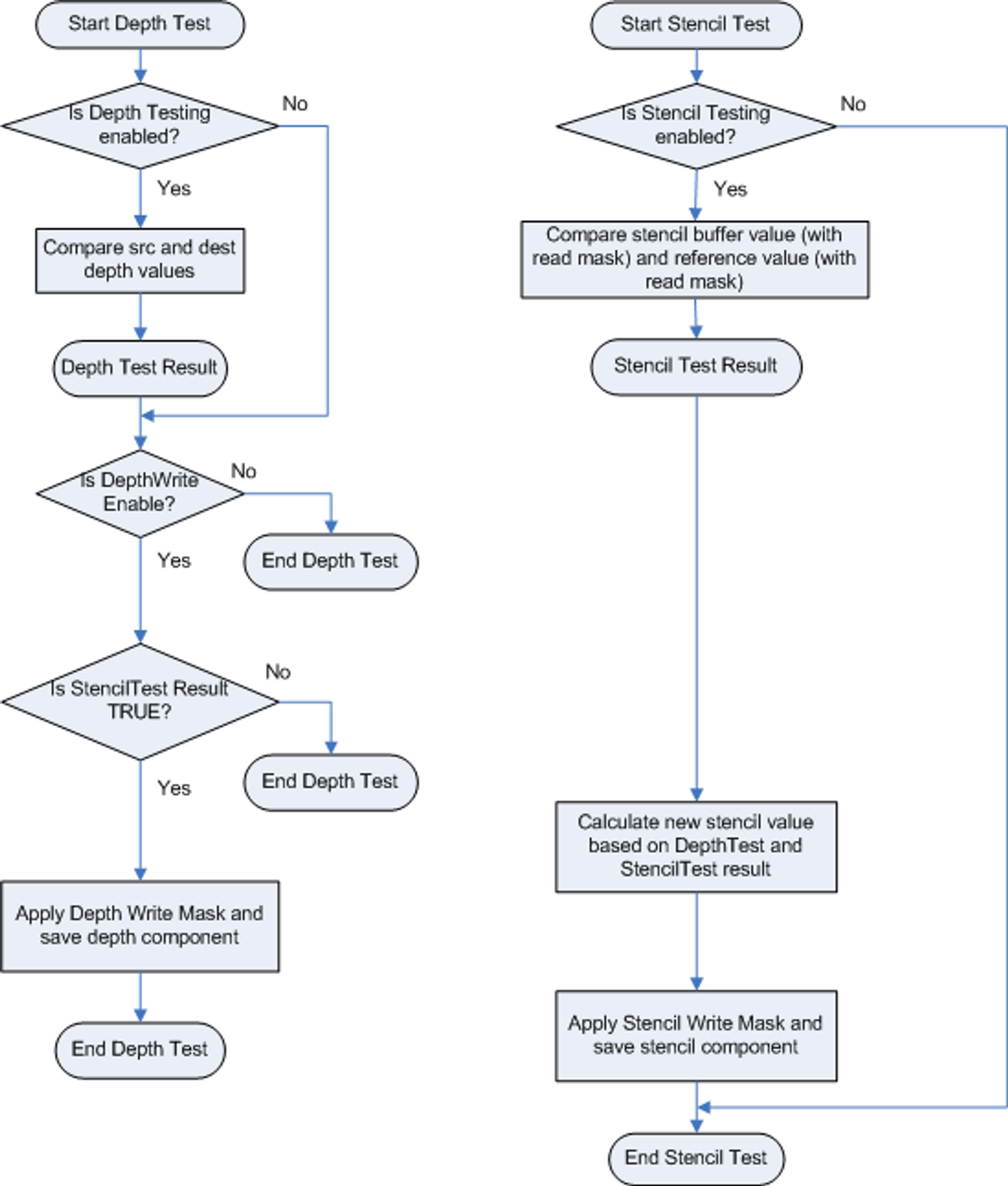
References
https://www.braynzarsoft.net/viewtutorial/q16390-03-initializing-directx-12
https://learn.microsoft.com/en-us/windows/win32/direct3d12/direct3d-12-graphics