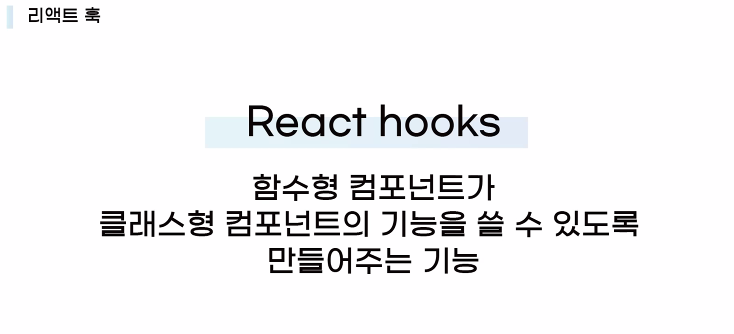
Increase, decrease
import React, { Component } from 'react'
/**
* - class component 단축키: rcc +enter
* -
*/
export default class Ex13 extends Component {
constructor(props){
/**생성자 함수: 가장 먼저 실행되는 공간
* state값, 변수값, 초기화하는 공간
* super(props)와 함께 사용하는 것을 권고라고 있음
* 밑에서 this문법 사용 시 생성자 내에서 정의되지 않아 버그 발생
*/
super(props);
this.state = {counter : 0 };
this.handleIncrement = this.handleIncrement.bind(this);
this.handleDecrement = this.handleDecrement.bind(this);
}
handleIncrement(){
console.log('handleIncrement function');
this.setState({
counter : this.state.counter += 1
});
}
handleDecrement(){
console.log('handleDecrement function');
this.setState({
counter : this.state.counter -= 1
});
}
render() {
return (
<div>
<h1>Class counter : {this.state.counter}</h1>
<button onClick={this.handleIncrement}>+</button>
<button onClick={this.handleDecrement}>-</button>
</div>
)
}
}
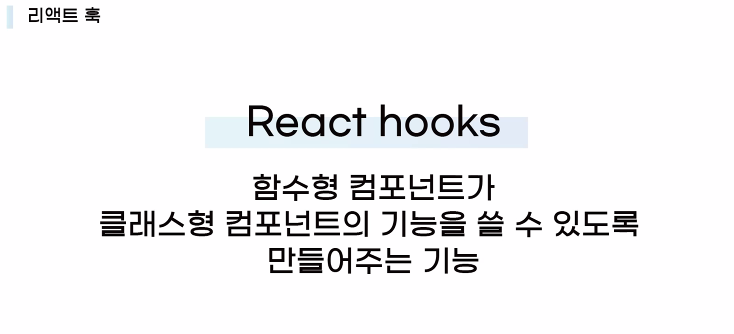
Increase
import React, {useEffect, useState} from 'react'
const Ex14 = () => {
/**
* classComponent에서는 시점을 지정할 수 있는 life cycle이라는 게 존재 (ex13.jsx)
* => function component에서는 사용이 불가능
* 대체 사용이 가능한 홋 useEffect를 사용
* 1. useEffect(함수, [])
* componetnDidAmoutn를 대체
* UI 세팅이 완료되어 dom 에 접근 가능
* api call
* 주의사항
* -> 비어있더라도 array는 꼭 적어줘야함
* 2. useEffect (함수, [state])
* componentDidUpdate를 대체
* array 안에 있는 값이 갱신된 직후에 호춯
* 최초 랜더링 때 한번 호출
* 변화를 감지할 대상은 복수가 될 수도 있다.
*/
console.log('1. constructor를 대체하는 함수 초기화');
useEffect(()=>{
console.log('3. 화면 render 끝');
},[]);
const [num,setNum] = useState(0);
const btnCk = ()=>{
setNum(num+1)
}
useEffect(()=>{
console.log('Update');
},[num]);
return (
<div>
{console.log('2. render를 대체하는 return')}
<h1>Function Counter: {num}</h1>
<button onClick={btnCk}>+1</button>
</div>
)
}
export default Ex14