ImageView
- 이미지 사이즈가 자동 스케일링 됨 (이미지가 구겨지지 않음)
- 짧은면을 기준으로 맞춘다
- scaleType="centerCrop" : 썸네일 이미지 보여줄 때 많이 씀
- 이미지는 리소스의 번호를 가져오는 방법이 없다 => Tag 기능을 활용해 번호 달아주기 방법 활용!
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical"
android:padding="16dp">
<ImageView
android:id="@+id/imgV"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@drawable/flag_korea"
android:scaleType="centerInside"
android:background="@drawable/ic_launcher_background"/>
<Button
android:id="@+id/btnAus"
android:layout_marginTop="8dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="australia"/>
<Button
android:id="@+id/btnBgi"
android:layout_marginTop="3dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="belgium"/>
<Button
android:id="@+id/btnKor"
android:layout_marginTop="3dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="korea"/>
<Button
android:id="@+id/btnIty"
android:layout_marginTop="3dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="italy"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<ImageView
android:layout_width="50dp"
android:layout_height="wrap_content"
android:src="@drawable/flag_france"
android:layout_marginRight="50dp"/>
<ImageView
android:layout_width="50dp"
android:layout_height="wrap_content"
android:src="@drawable/flag_france"
android:adjustViewBounds="true"/>
</LinearLayout>
</LinearLayout>
package com.bsj0420.ex04imageview;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
ImageView imgV;
Button btnAus;
Button btnBgi;
Button btnKor;
Button btnIty;
int num =0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imgV = findViewById(R.id.imgV);
btnAus = findViewById(R.id.btnAus);
btnBgi = findViewById(R.id.btnBgi);
btnKor = findViewById(R.id.btnKor);
btnIty = findViewById(R.id.btnIty);
btnAus.setOnClickListener(listener);
btnBgi.setOnClickListener(listener);
btnKor.setOnClickListener(listener);
btnIty.setOnClickListener(listener);
imgV.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
imgV.setImageResource(R.drawable.flag_australia + num);
num++;
if(num > 12) num=0;
}
});
}
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
int id = view.getId();
if(id == R.id.btnAus) imgV.setImageResource(R.drawable.flag_australia);
else if (id == R.id.btnBgi) imgV.setImageResource(R.drawable.flag_belgium);
else if (id == R.id.btnKor) imgV.setImageResource(R.drawable.flag_korea);
else imgV.setImageResource(R.drawable.flag_italy);
}
};
}
토이예제
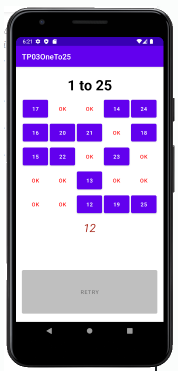
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity"
android:weightSum="10"
android:padding="16dp">
<TextView
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:text="1 to 25"
android:textSize="38sp"
android:textColor="@color/black"
android:gravity="center"
android:textStyle="bold"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:weightSum="5"
android:orientation="horizontal">
<Button
android:id="@+id/b01"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b02"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b03"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b04"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b05"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:weightSum="5"
android:orientation="horizontal">
<Button
android:id="@+id/b06"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b07"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b08"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b09"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b10"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:weightSum="5"
android:orientation="horizontal">
<Button
android:id="@+id/b11"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b12"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b13"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b14"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b15"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:weightSum="5"
android:orientation="horizontal">
<Button
android:id="@+id/b16"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b17"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b18"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b19"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b20"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:weightSum="5"
android:orientation="horizontal">
<Button
android:id="@+id/b21"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b22"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b23"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b24"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
<Button
android:id="@+id/b25"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_margin="2dp"
/>
</LinearLayout>
<TextView
android:id="@+id/crrNum"
android:layout_marginTop="10dp"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="2"
android:layout_gravity="center_horizontal"
android:textStyle="italic"
android:textColor="#AC291F"
android:textSize="30sp"
/>
<Button
android:id="@+id/btn_retry"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2"
android:text="RETRY"
android:backgroundTint="#BFBFBF"
android:enabled="false"/>
</LinearLayout>
package com.bsj0420.tp03oneto25;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
public class MainActivity extends AppCompatActivity {
Button[] btnArr = new Button[25];
TextView crrNum;
Button btnRetry;
int num = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
crrNum = findViewById(R.id.crrNum);
btnRetry = findViewById(R.id.btn_retry);
ArrayList<Integer> listNum = new ArrayList<>();
for(int i=1; i<=btnArr.length; i++) listNum.add(i);
Collections.shuffle(listNum);
for (int i=0; i<btnArr.length; i++) {
btnArr[i] = findViewById(R.id.b01 + (i));
btnArr[i].setText(listNum.get(i) + "");
btnArr[i].setOnClickListener(listener);
btnArr[i].setTag(listNum.get(i));
}
btnRetry.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
}
});
}
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String s =view.getTag().toString();
int n = Integer.parseInt(s);
if(n == num) {
view.setVisibility(View.INVISIBLE);
num++;
crrNum.setText(num + "");
if(num > 25 ) {
crrNum.setText("Clear~~");
btnRetry.setEnabled(true);
}
}
}
};
}