[1] BottomTabNavigator
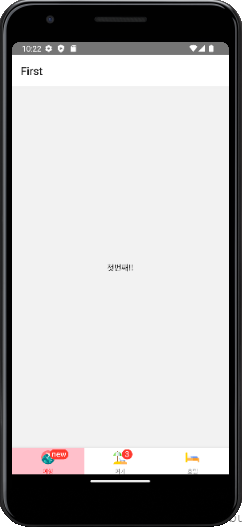
메인 화면
import React from "react";
import {Image, StyleSheet} from "react-native";
import { NavigationContainer } from "@react-navigation/native";
import { createBottomTabNavigator } from "@react-navigation/bottom-tabs";
export type BottomTabScreenList = {
First : undefined,
Second : undefined,
Third : undefined,
}
const BottomTab = createBottomTabNavigator<BottomTabScreenList>()
import FirstTab from "./screen_bottomtab/FirstTab";
import SecondTab from "./screen_bottomtab/SecondTab";
import ThirdTab from "./screen_bottomtab/ThirdTab";
export default function Main():JSX.Element {
return (
<NavigationContainer>
<BottomTab.Navigator
initialRouteName="Second"
screenOptions={{
tabBarActiveTintColor : '#fff',
tabBarInactiveTintColor : 'grey',
tabBarActiveBackgroundColor : 'pink',
tabBarShowLabel : true,
tabBarLabelPosition : "below-icon"
}}
>
{}
<BottomTab.Screen
name="First"
component={FirstTab}
options={{
tabBarLabel : "여행",
tabBarIcon : ()=>{return <Image style={style.icon} source={require('./image/travel.png')}></Image>},
tabBarBadge: 'new',
tabBarActiveTintColor:'red',
}}
></BottomTab.Screen>
<BottomTab.Screen
name="Second"
component={SecondTab}
></BottomTab.Screen>
<BottomTab.Screen
name="Third"
component={ThirdTab}
options={{
tabBarLabel : "호텔",
tabBarIcon : ()=> <Image style={style.icon} source={require('./image/bed.png')}></Image>,
}}
></BottomTab.Screen>
</BottomTab.Navigator>
</NavigationContainer>
)
}
const style = StyleSheet.create({
root: {
flex : 1,
padding : 16,
justifyContent : "center",
alignItems : "center",
},
text : {
padding:8,
color : 'black'
},
icon : {
width : 25,
height : 25
}
})
탭 화면
탭1
import React from "react";
import {View, Text, Button, StyleSheet} from 'react-native'
import {BottomTabScreenProps } from '@react-navigation/bottom-tabs'
import { BottomTabScreenList } from "../Main";
type FirstProps = BottomTabScreenProps<BottomTabScreenList, "First">
export default function FirstTab(props:FirstProps) {
return (
<View style={style.root}>
<Text style={style.text}>첫번째!!</Text>
</View>
)
}
const style = StyleSheet.create({
root: {
flex : 1,
padding : 16,
justifyContent : "center",
alignItems : "center",
},
text : {
padding:8,
color : 'black'
}
})
탭2
import React from "react";
import {View, Text, Image, Button, StyleSheet} from 'react-native'
import {BottomTabScreenProps } from '@react-navigation/bottom-tabs'
import { BottomTabScreenList } from "../Main";
type SecondProps = BottomTabScreenProps<BottomTabScreenList, "Second">
export default function SecondTab(props:SecondProps) {
props.navigation.setOptions({
tabBarLabel : "여가",
tabBarIcon : () => <Image style={style.icon} source={require('../image/beach.png')}></Image>,
tabBarBadge : '3',
})
return (
<View style={style.root}>
<Text style={style.text}>Second</Text>
</View>
)
}
const style = StyleSheet.create({
root: {
flex : 1,
padding : 16,
justifyContent : "center",
alignItems : "center",
},
text : {
padding:8,
color : 'black'
},
icon : {
width : 25,
height : 25,
}
})
탭3
import React from "react";
import {View, Text, Button, StyleSheet} from 'react-native'
import {BottomTabScreenProps } from '@react-navigation/bottom-tabs'
import { BottomTabScreenList } from "../Main";
type ThirdProps = BottomTabScreenProps<BottomTabScreenList, "Third">
export default function ThirdTab(props:ThirdProps) {
return (
<View style={style.root}>
<Text style={style.text}>Third</Text>
</View>
)
}
const style = StyleSheet.create({
root: {
flex : 1,
padding : 16,
justifyContent : "center",
alignItems : "center",
},
text : {
padding:8,
color : 'black'
}
})
[2] MaterialTopTabs
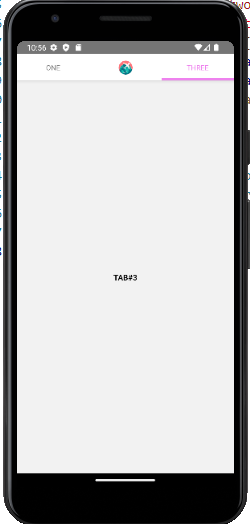
메인 화면
import React from "react";
import { StyleSheet, Image } from "react-native";
import { NavigationContainer } from "@react-navigation/native";
import { createMaterialTopTabNavigator } from "@react-navigation/material-top-tabs";
export type TopTabScreenList = {
one : undefined,
two : undefined,
three : undefined
}
const TopTab = createMaterialTopTabNavigator<TopTabScreenList>()
import FirstTab from "./sceen_metrial_top_tab/FirstTab";
import SecondTab from "./sceen_metrial_top_tab/SecondTab";
import ThirdTab from "./sceen_metrial_top_tab/ThirdTab";
export default function MainMaterialTopTab() {
return (
<NavigationContainer>
<TopTab.Navigator
tabBarPosition="top"
screenOptions={{
swipeEnabled : true,
tabBarActiveTintColor : 'violet',
tabBarInactiveTintColor : 'grey',
tabBarIndicatorStyle : {
backgroundColor:'violet',
height : 4,
},
tabBarShowIcon : true,
}}
>
<TopTab.Screen name="one" component={FirstTab}></TopTab.Screen>
<TopTab.Screen
name="two"
component={SecondTab}
options={{
tabBarLabel : '하이',
tabBarShowLabel : false,
tabBarIcon : () => <Image style={{width:25, height:25}} source={require('./image/travel.png')}></Image>
}}
></TopTab.Screen>
<TopTab.Screen name="three" component={ThirdTab}></TopTab.Screen>
</TopTab.Navigator>
</NavigationContainer>
)
}
탭 화면
탭1
import React from 'react'
import { View, Text, StyleSheet } from 'react-native'
import { MaterialTopTabScreenProps } from '@react-navigation/material-top-tabs'
import { TopTabScreenList } from '../MainMaterialTopTab'
type FirstProps= MaterialTopTabScreenProps<TopTabScreenList,"one">
export default function FirstTab(props:FirstProps):JSX.Element{
return (
<View style={style.root}>
<Text style={style.text}>TAB#1</Text>
</View>
)
}
const style= StyleSheet.create({
root:{flex:1, justifyContent:'center', alignItems:'center'},
text:{padding:8, color:'black', fontWeight:'bold'},
})
탭2
import React from 'react'
import { View, Text, StyleSheet } from 'react-native'
import { MaterialTopTabScreenProps } from '@react-navigation/material-top-tabs'
import { TopTabScreenList } from '../MainMaterialTopTab'
type SecondProps= MaterialTopTabScreenProps<TopTabScreenList,"two">
export default function SecondTab(props:SecondProps):JSX.Element{
return (
<View style={style.root}>
<Text style={style.text}>TAB#2</Text>
</View>
)
}
const style= StyleSheet.create({
root:{flex:1, justifyContent:'center', alignItems:'center'},
text:{padding:8, color:'black', fontWeight:'bold'},
})
탭3
import React from 'react'
import { View, Text, StyleSheet } from 'react-native'
import { MaterialTopTabScreenProps } from '@react-navigation/material-top-tabs'
import { TopTabScreenList } from '../MainMaterialTopTab'
type ThirdProps= MaterialTopTabScreenProps<TopTabScreenList,"three">
export default function ThirdTab(props:ThirdProps):JSX.Element{
return (
<View style={style.root}>
<Text style={style.text}>TAB#3</Text>
</View>
)
}
const style= StyleSheet.create({
root:{flex:1, justifyContent:'center', alignItems:'center'},
text:{padding:8, color:'black', fontWeight:'bold'},
})
Draw (현재 버전 맞지 않아 실습은 못함..)
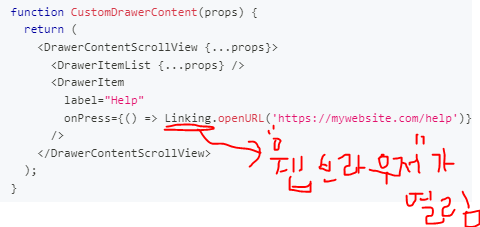