Assembling, Linking, and Running Programs
어셈블리 빌드하는 순서
- Assemble-Link Execute Cycle
- The following diagram describes the steps from creating a source program through executing the compiled program.
- If the source code is modified, Steps 2 through 4 must be repeated.
- Assembler는 어셈블리 instruction을 machine instruction으로 바꾼다(.obj).
- .obj file에서는 사용하는 주소를 모두 0을 기준으로 한다(상대 주소(relative address)).
- Linker는 .obj 파일을 라이브러리 함수와 결합하여 .exe file을 만든다.
- Loader는 .exe 파일을 메모리에 load 하여 실행될 수 있도록 한다.
- 상대 주소를 절대 주소로 바꾸는 등 여러 과정을 거치는데, 이는 시스템 프로그래밍을 공부하여 알 수 있다.
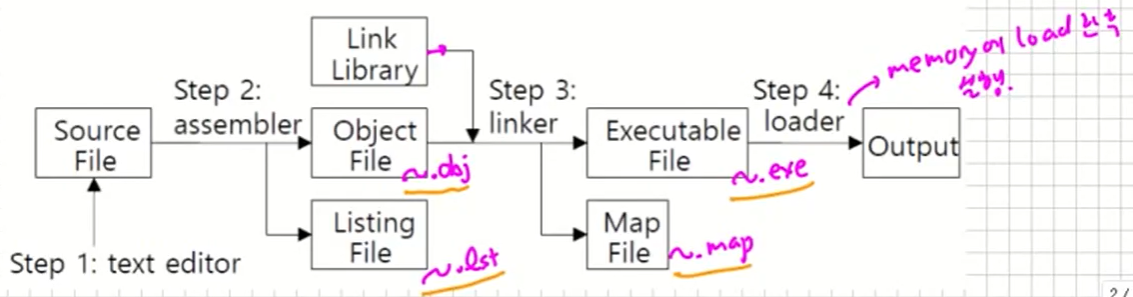
Assembler는 ~.asm -> .obj로 만들 것인데 단순히 assembly instruction을 machine instruction으로 바꾸는 역활을 한다. 항상 0번지에서 시작하도록 만든다.
그리고 Linker가 함수들을 다 더해서 ~.exe 파일을 만든다.
loader가 ~.exe파일을 메모리에 올릴 것이다.
이때의 시작 주소는 dynamic하게 바뀐다.
Listing File
아래쪽에 보면 프로그램이 어떻게 커파일되는지 확인할 수 있다.
opcode는 보통 8byte인것도 확인할 수 있다.
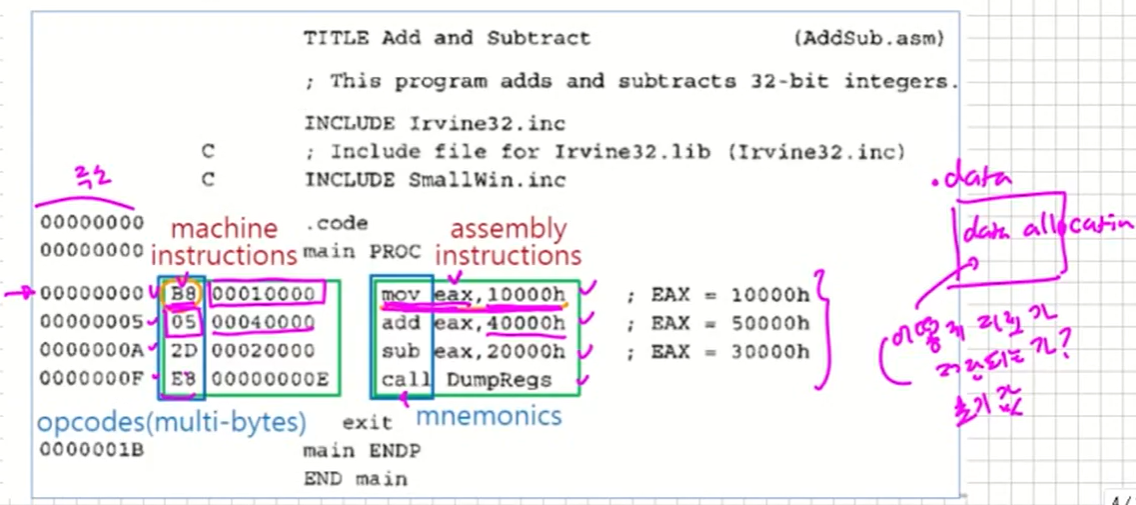
- Also you can see segment names, symbols (variables, procedures, and constants)
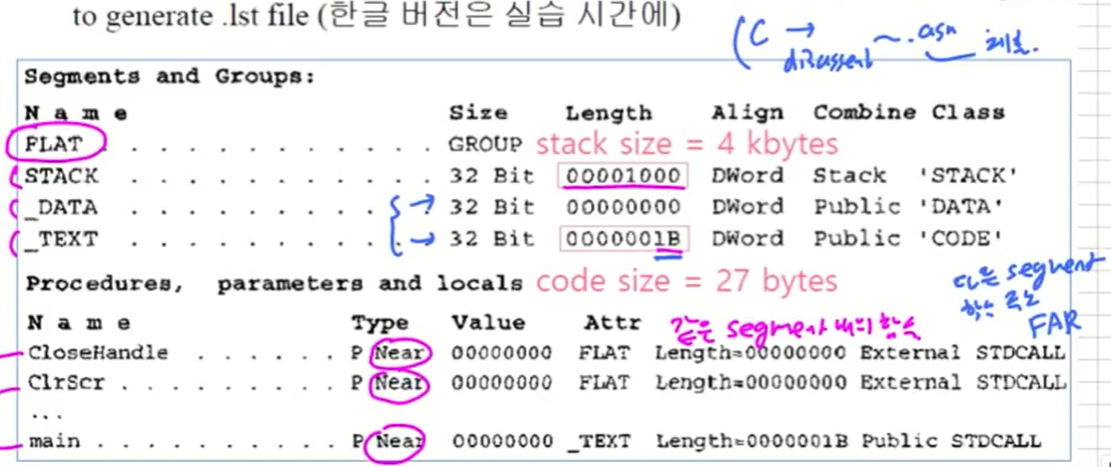
Near은 같은 Segment내의 함수 다른 segment 함수는 Far이라고 함
나중에 Size도 체크할 수 있으니 정확하게 문제를 풀자
Map File
대규모 프로젝트용이라는데 교수님도 모르신다는데 제가 어떻게 알아요;;
Defining Data
Intrinsic Data Types
- BYTE, SBYTE : 8-bit unsigned integer; 8-bit signed integer
- WORD, SWORD : 16-bit unsigned & signed integer : unsigned short; short
- DWORD, SDWORD : 32-bit unsigned & signed integer : unsigned int; int
- Signed와 Unsigned와의 차이
- C 언어에서는 확연히 차이가 난다(int, unsigned int)
- 어셈블리에서는 단지 구분을 쉽게 하기 위함이다. 즉 프로그래머용
- SBYTE로 할당한 변수를 unsigned로 사용해도 문제 없다.
- QWORD : 64-bit integer
- TBYTE : 80-bit integer
- REAL4 : 4-byte IEEE short real
- REAL8 : 8-byte IEEE long real
- REAL10 : 10-byte IEEE extended real
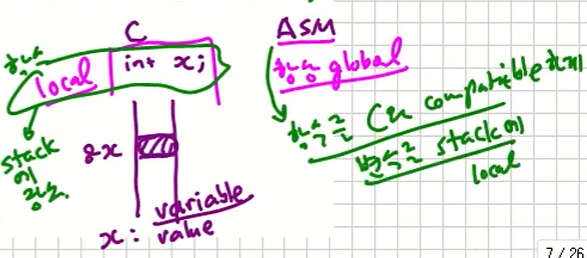
Data Definition Statement
Defining Bytes
- Defining a single byte of storage
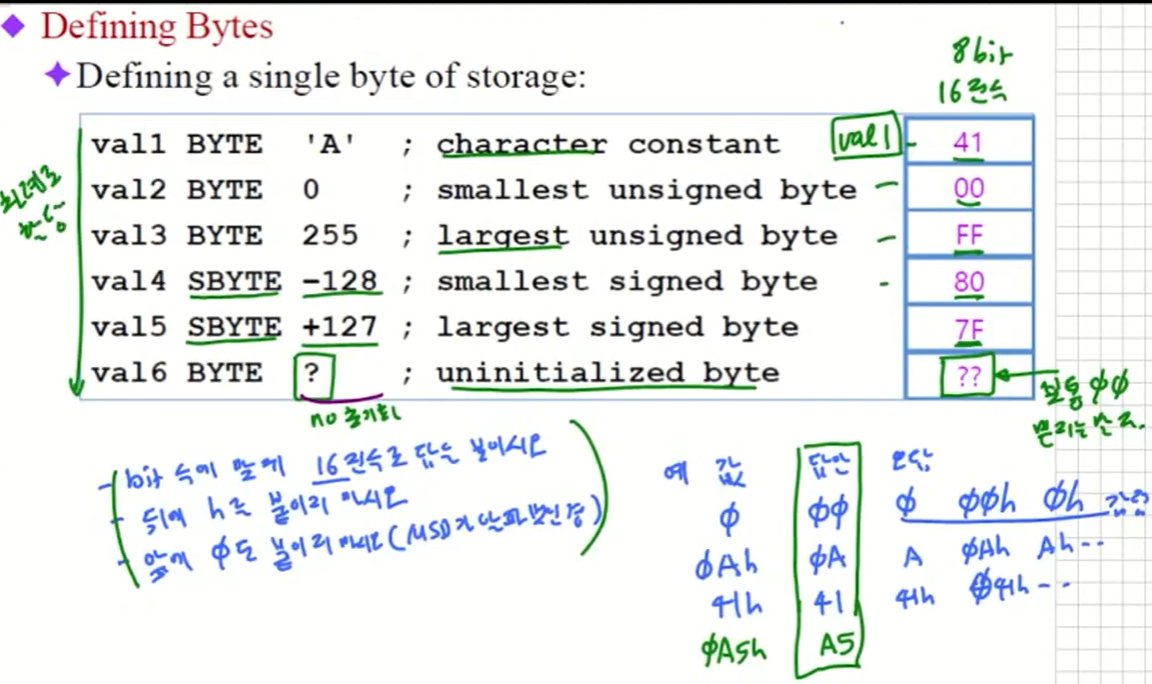
나중에 시험에 bit 수에 맞게 16진수로 답을 보이시오라는 말이 나온다.
뒤에 h를 붙이지 마시오
앞에 0도 붙이지 마시오
혹은 어셈블리 문법에 맞도록 보이시오라고 요구할 수 있다.
그럼 정말로 16진수가 되도록 남은 비트는 0으로 채우되 그 앞에 0과 뒤에 h를 붙이지 말자
-
MASM does not prevent you from initializing a BYTE with a negative value, but it's considered poor style.
-
If you declare a SBYTE variable, the Microsoft debugger will automatically display its value in decimal with a leading sign. SBYTE인 경우 signed 8bit으로 표시(조사식항)
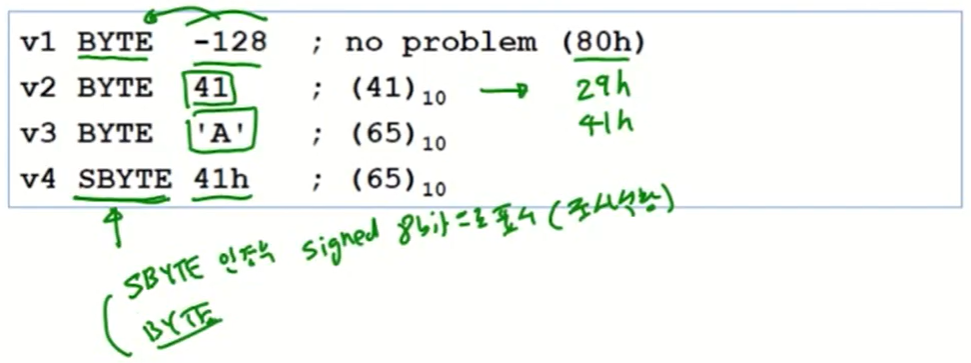
범위만 벗어나지 않으면 어떻게 표시하든 알아서 16진수로 변환해준다. 대신에 Microsoft에서는 SBYTE는 signed로 표시가 된다.
-
Defining multiple bytes of storage with initialization:
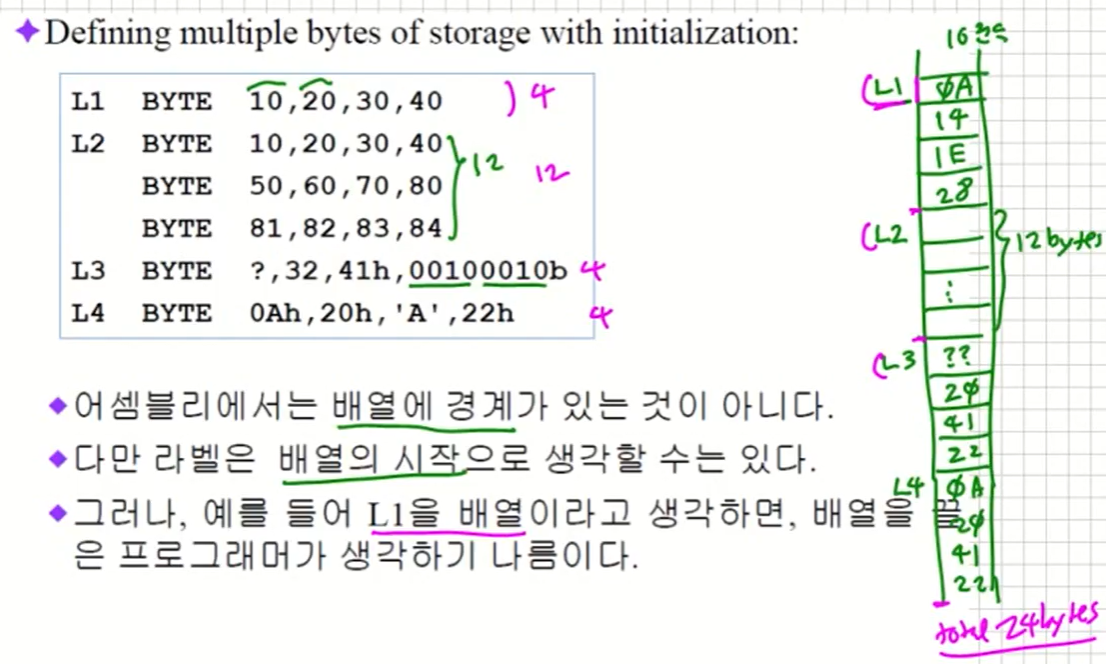
- 어셈블리에서는 배열에 경계가 있는 것이 아니다.
- 다만 라벨은 배열의 시작으로 생각할 수는 있다.
- 그러나, 예를 들어 L1을 배열이라고 생각하면, 배열을 끝은 프로그래머가 생각하기 나름이다.
Defining Strings
- A string is implemented as an array of characters.
- For convenience, it is usually enclosed in quotation marks.
- It usually has a null byte at the end.
- Examples:
빈칸 : 20h
A : 41h
a : 61h
0 : 30h
\n : 0dh, 0ah
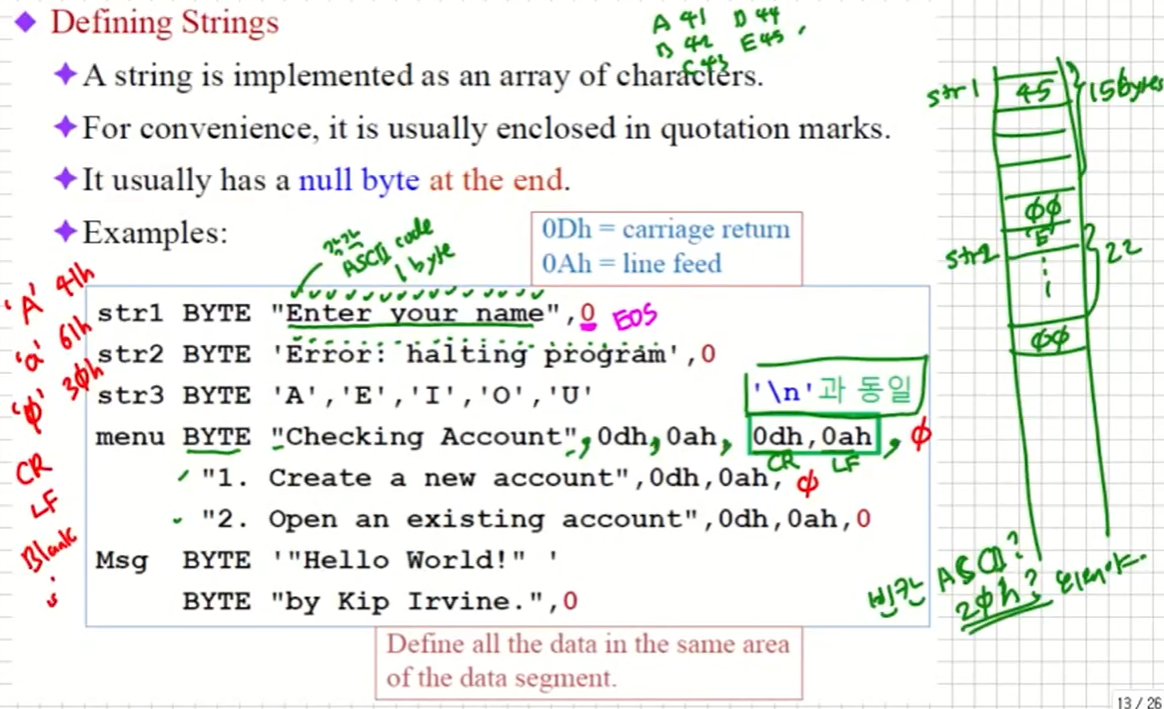
DUP Operator
- Use DUP to allocate (create space for) an array or string. : 반복 초기화
- Syntax: counter갯수 DUP ( arguments초기값)
- Counter and argument must be constants or constant expressions.
- Arguments can be constant list separated by comma.
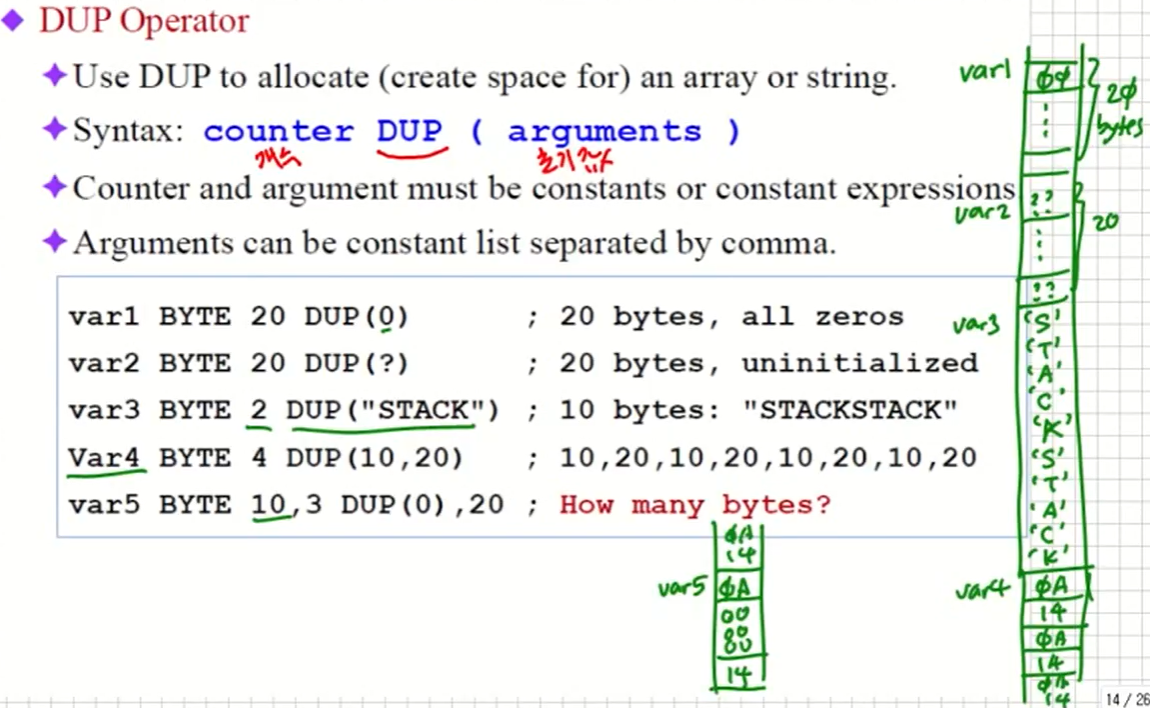
총 20 + 20 + 10 + 8 + 5 = 63byte
Declaring Uninitialized Data
초기화 하지 않은 경우
- Use the .data? directive to declare an uninitialized data segment. : .data 초기화 가능 storage, .data?는 초기화 불가능
- Within the segment, declare variables with "?" initializers:

- Advantage: the program's EXE file size is reduced. : EXE file의 크기를 줄일 수 있다. 그래서 assembly와 linker가 EXE 파일 만들때 그 자리에 symbol만 남기어 놓고 나중에 loader가 해당 위치를 allocation한다.
Assembler Source File 구조
- Source 코드에서는 .data, .data?, .code를 섞어 작성해도 무방하다.
- 어셈블러는 같은 segment끼리 묶어 나열한다.
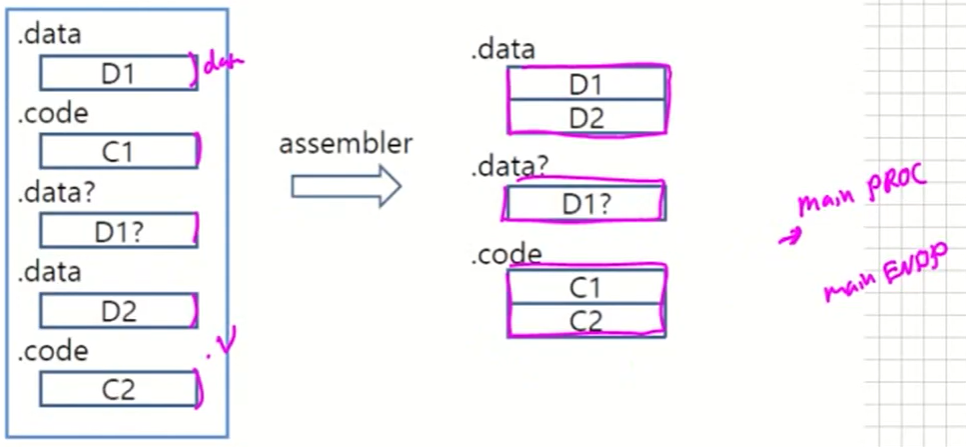
Little Endian Order
모든 메모리 주소는 byte 단위
- All data types larger than a byte store their individual bytes in reverse order. The least significant byte occurs at the first (lowest) memory address. : LSB 부터 저장하는 방식을 Little Endian Order이라고 한다 (그 반대는 Big endian order)
- Example: val1 DWORD 123456798h (4byte인데 저장순서는 어떻게 하는가?)
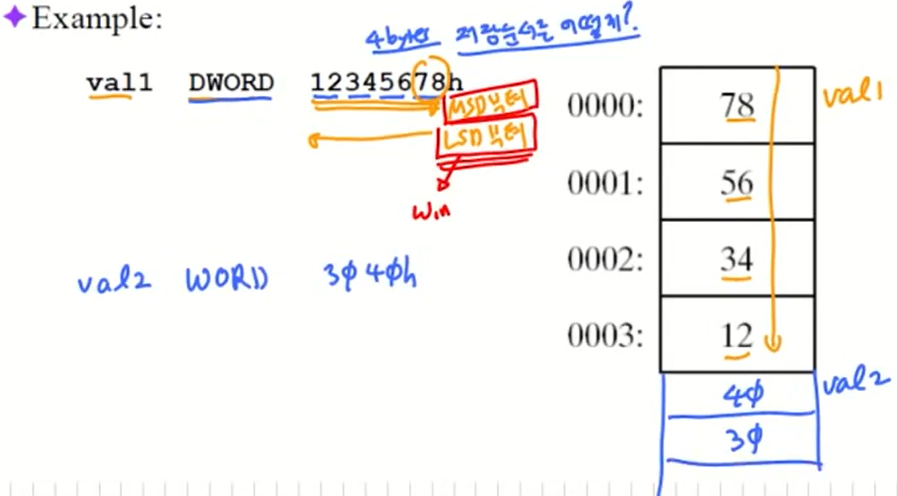
이렇게 저장되어 있기 때문에 읽을때는 거꾸로 읽게 된다.
추가로 .lst 파일에서 data저장이 LSB 부터이다.
Defining WORD and SWORD Data (16 bit)
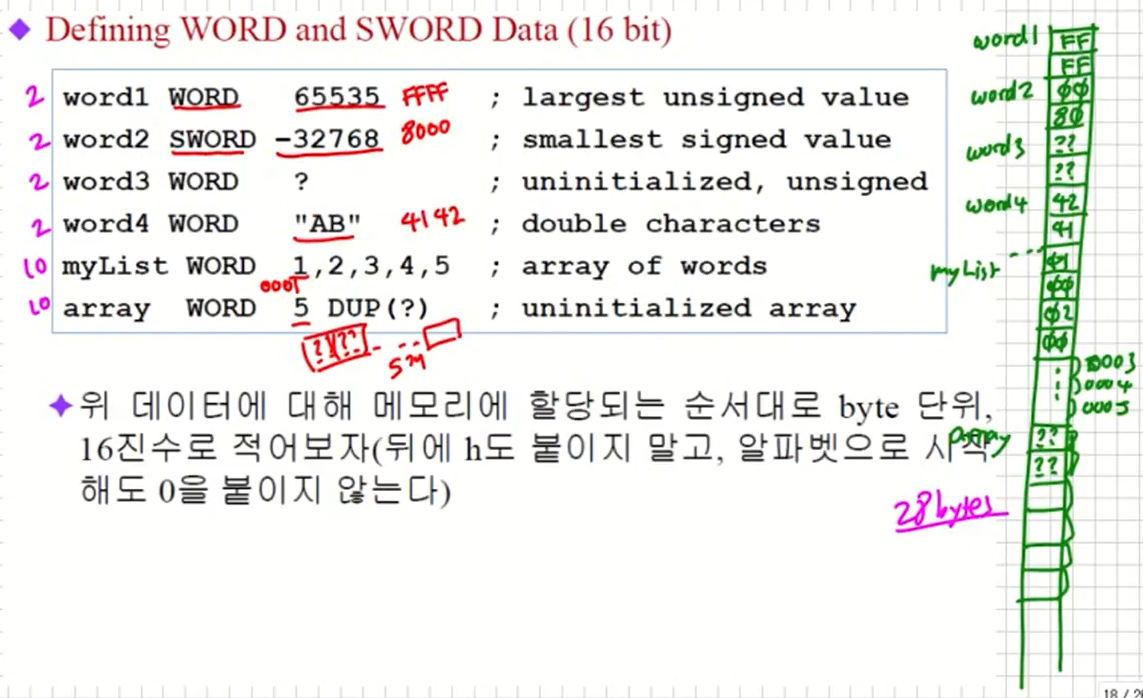
총합 28bytes이다.
Defining DWORD and SDWORD Data (32 bit)
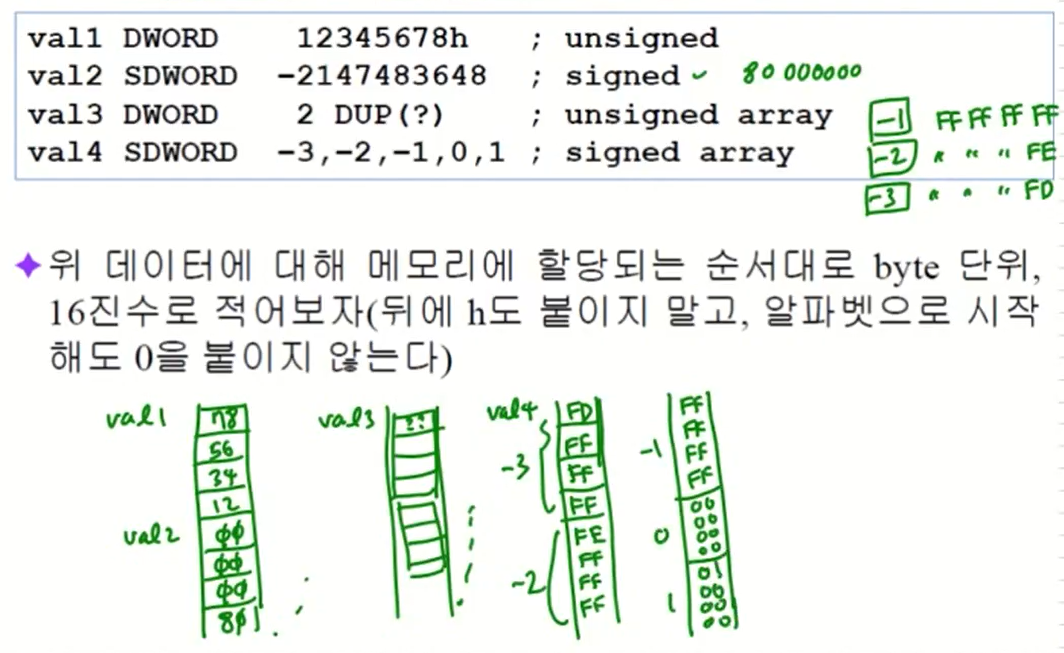
총합 36bytes이다.
Defining QWORD, TBYTE, Real Data
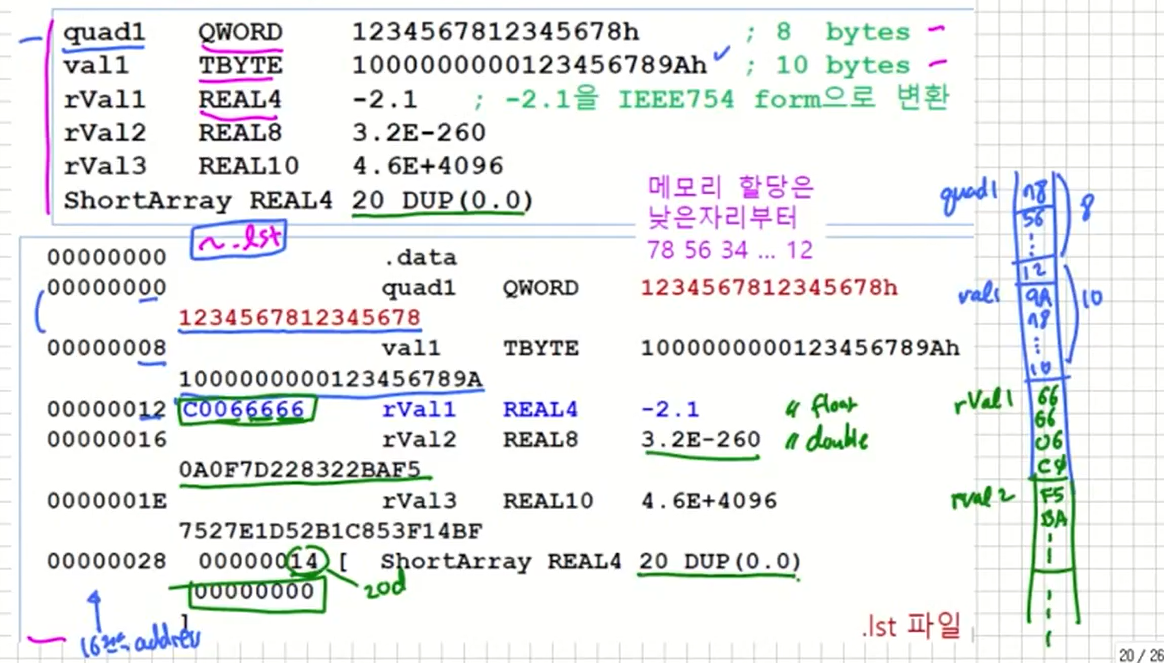
lst 파일을 보면 내부에 어떻게 저장되었는지 확인 가능하다.
Adding Variables to AddSub
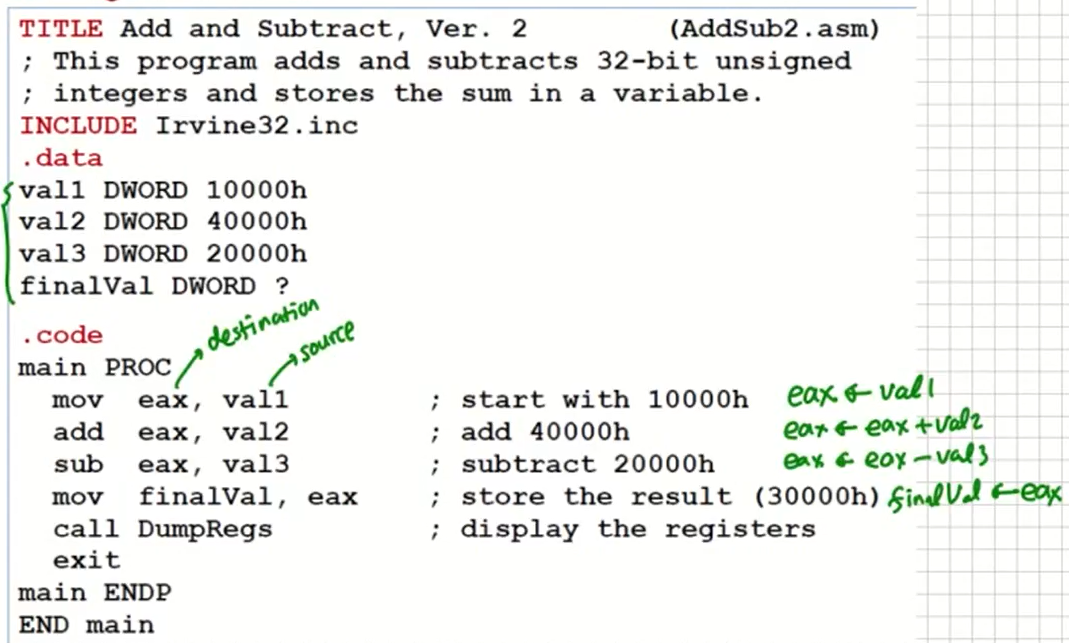
5. Symbolic Constants
Equal-Sign Directive
상수 값은 symbolize하면 편리(#define 과 동일, 가독성이 좋아짐)
- name = expression (32bit int exp or const)
- May be redefined and name is called a symbolic constant.
- good programming style to use symbols.
- Example
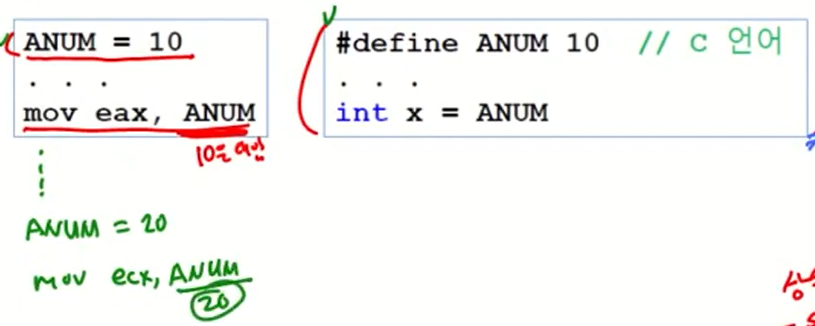
EQU Directive
- Define a symbol as either an integer or text expression.
- Cannot be redefined. : 재정의가 불가능하다는 차이점이 존재
- Example

TEXTEQU Directive
글자 정의 매크로
- Define a symbol as either an integer or text expression.
- Called a text macro.
- Can be redefined
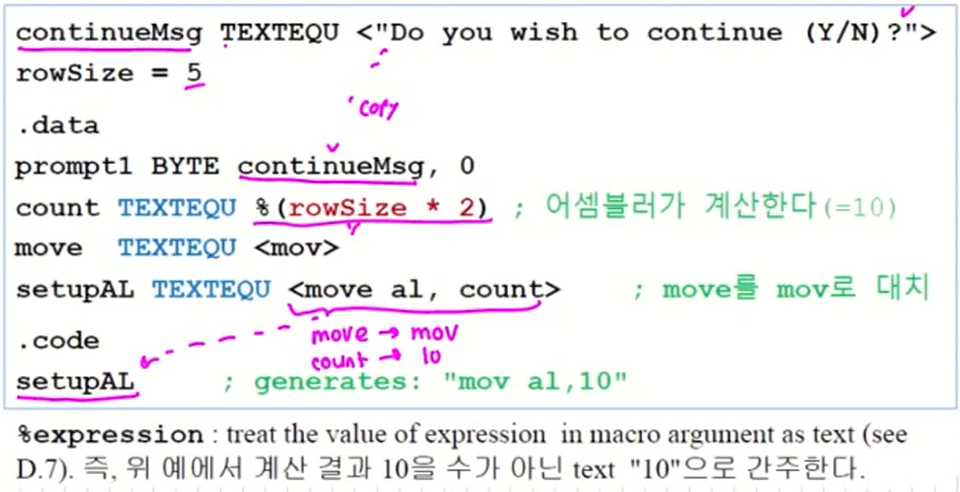
% 사용시 어셈블러가 직접 계산
Current location counter: $
현재 위치를 의미, $는 상수값
- Subtract address of list.
- Difference is the number of bytes.
- Examples
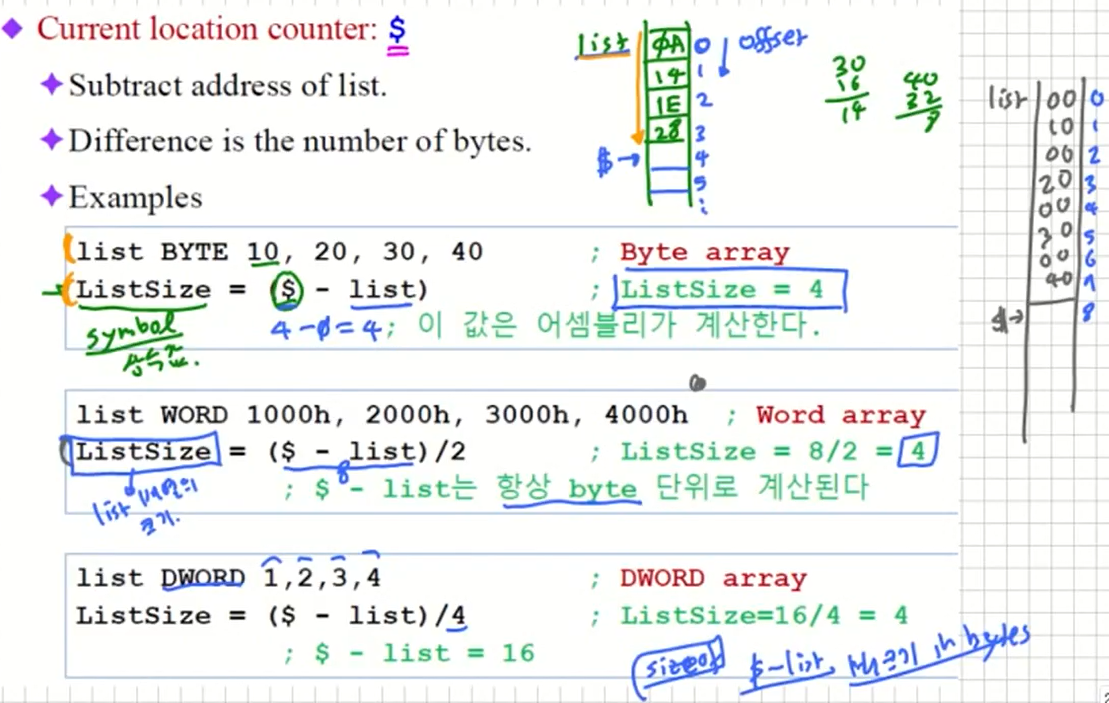
More Examples
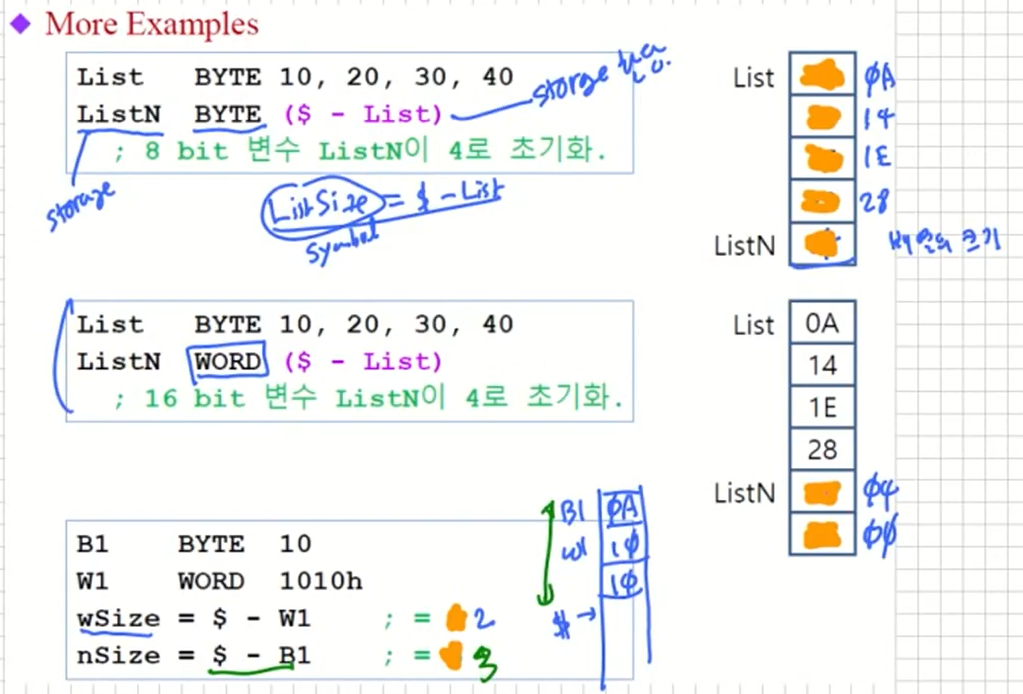
6. Real-Address Mode Programming
- Generate 16-bit MS-DOS Programs
- Code segment의 주소를 CS에 저장하고(하위 4 bit = 0), IP에
offset을 저장. (CS:IP, Code의 현재 fetch한 instruction의 주소를 가지고 있다.)
- Windows 10에서는 DOSBox에서 실행 가능(MS-DOS
Emulator)
- Embbeded system에서 8086을 사용한다면 필요할수도...
- Advantages
- Enables calling of MS-DOS and BIOS functions : MS-DOS와 BIOS에 있는 함수들을 자유롭게 사용가능
- No memory access restrictions : 메모리의 전범위를 이용가능(1M Byte)
- Disadvantages
- Must be aware of both segments and offsets : 세그먼트당 64kb를 넘어가면 안된다. 다수 세그먼트 가능.
- Cannot call Win32 functions (Windows 95 onward) : Win32 함수를 호출 할 수 없다.
- Limited to 640K program memory : 640K의 프로그램 메모리 제한
- Requirements
- 16bit 프로그래밍 할때는 Irvine16.inc를 포함하고, 아래 코드를 추가하여야 한다.

data segment의 위치를 setting 해주어야 한다.
@data 는 data segment의 segment address로 ds(data segment register)에 넣어야 한다. 이때 직접 immediate operand를 할당 할 수 없다. 그래서 ax에 @data를 넣고 ds에 ax의 값을 옮기어서 ds에 값을 assign하게 된다.
그래서 @data라는 것은 .data의 시작 주소를 이야기 한다.
Add and Subtract, 16-Bit Version
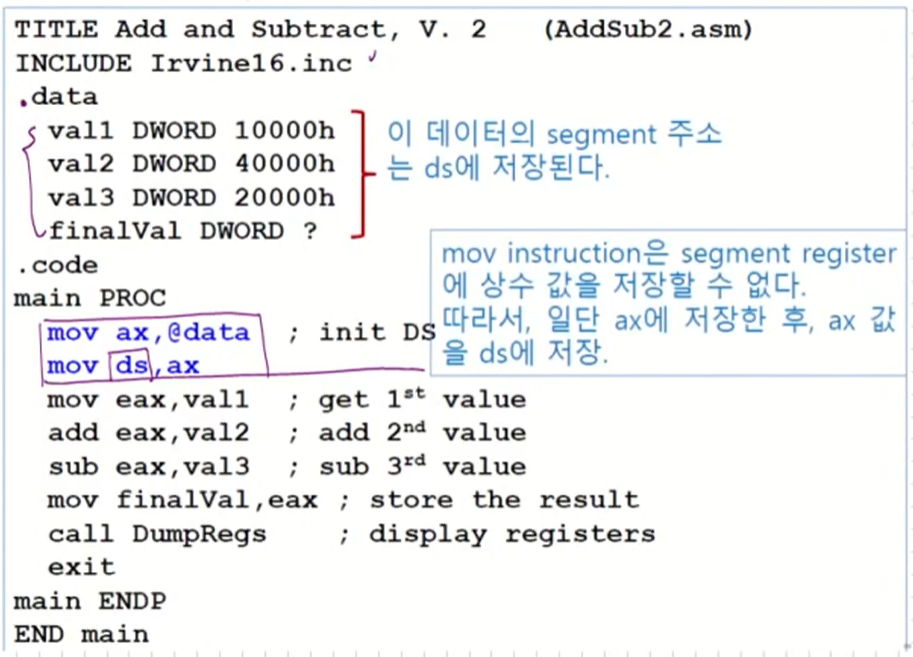
64-Bit Programming
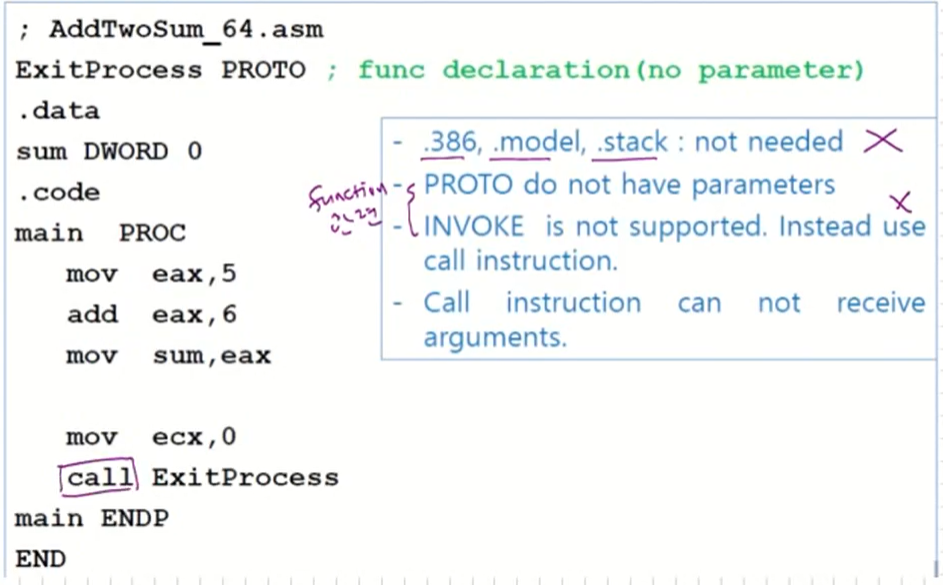
Call를 이용해서 parameter passing을 호출
그래서 call 함수 앞에 사전작업(parameter을 전달하기 위해 => register, memory, stack을 통한다)을 해야 한다.
7. Data Transfer Instructions
Operand Types
- Three basic types of operands:
- Immediate – constant values (8, 16, or 32 bits) : 상수값
- value is encoded within the instruction
- Register – the name of a register : 레지스터
- register name is converted to a number and encoded within the instruction
- Memory – reference to a location in memory : 메모리에서 읽고 쓰는 operand들
- memory address is encoded within the instruction, or a register holds the address of a memory location
- Direct, indirect, direct offset, indexed operand 등이 있다.
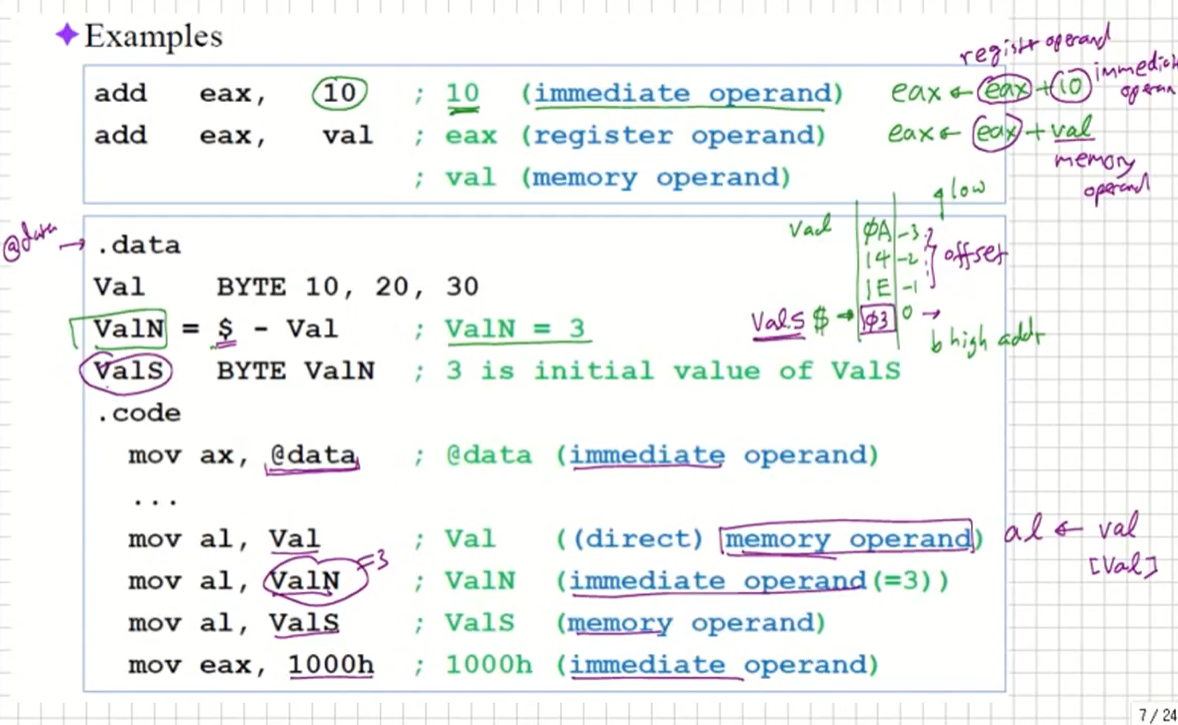
Instruction Operand Notation(See Appendix B)
r8 : 8bit register
r16 : 16bit register
r32 : 32bit register
reg : any general-purpose register
sreg :
r/m 8 : register 또는 memory 8bit
mem : 8,16,32bit memory operand를 사용할 수 있다.
Direct Memory Operands
- A direct memory operand is a named reference to storage in
memory. : memory에 할당된 곳에 label이 달려있으면 거기에서 무언가를 가지고 올때 Direct Memory라고 부른다.
- The named reference (label) is automatically dereferenced by the assembler.
- Example
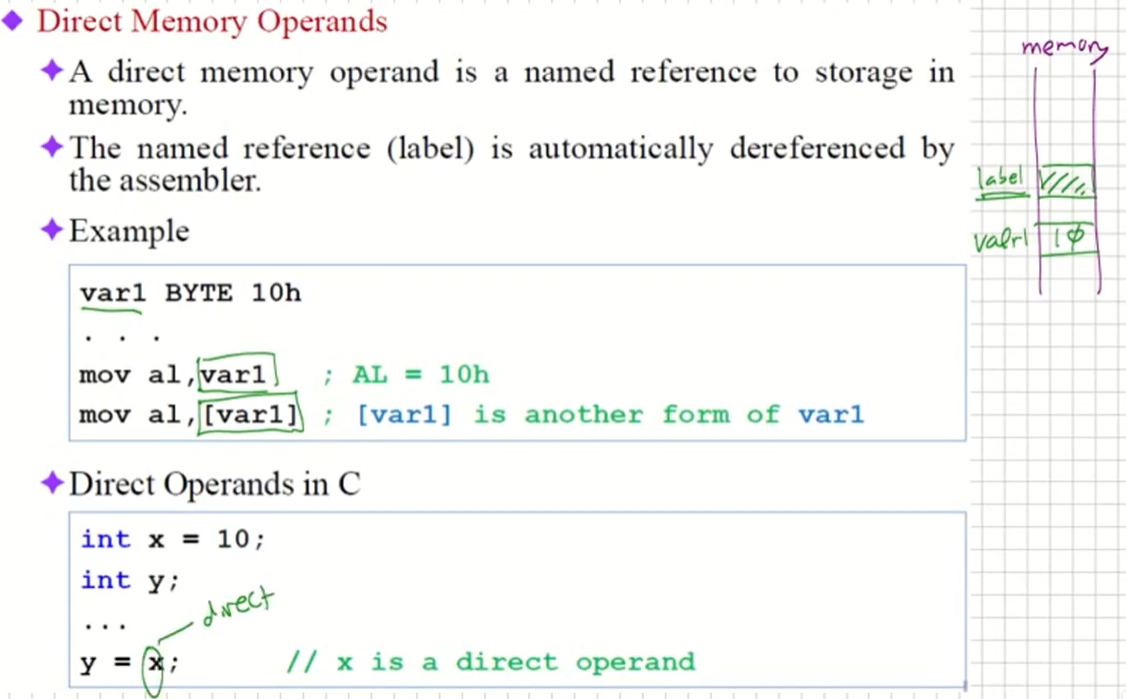
위의 var1과 [var1]은 같은거다.
MOV Instruction
-
Move from source to destination.
-
Syntax:

-
No more than one memory operand permitted : memory operand가 한개만 사용가능하다.( ex. mov var1, var2 ; 오류)
-
CS, EIP, and IP cannot be the destination(current fetch instruction address) destination으로 사용하면 안된다.
-
No immediate to segment moves : segmet address에 immediate를 작성 불가 (mov ds, 1oh : 오류)
두 operand의 size가 같아야 한다. 아니면 오류
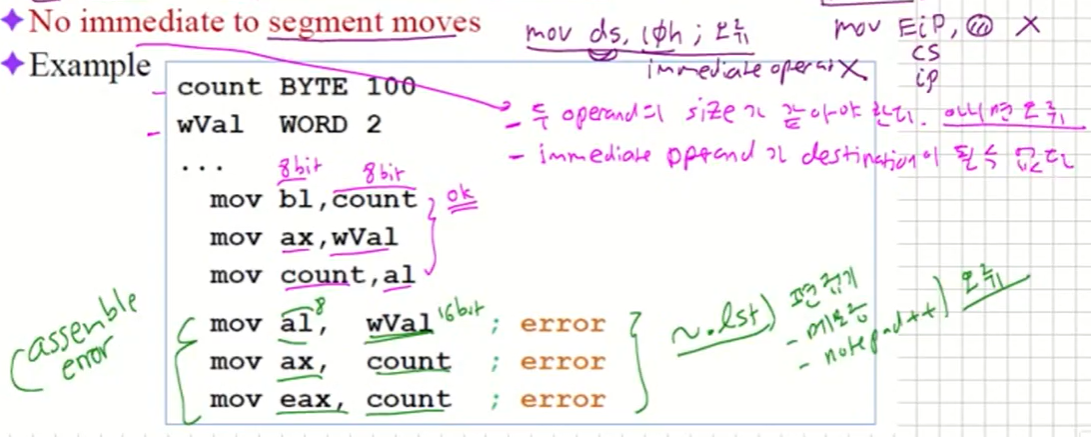
-
Example
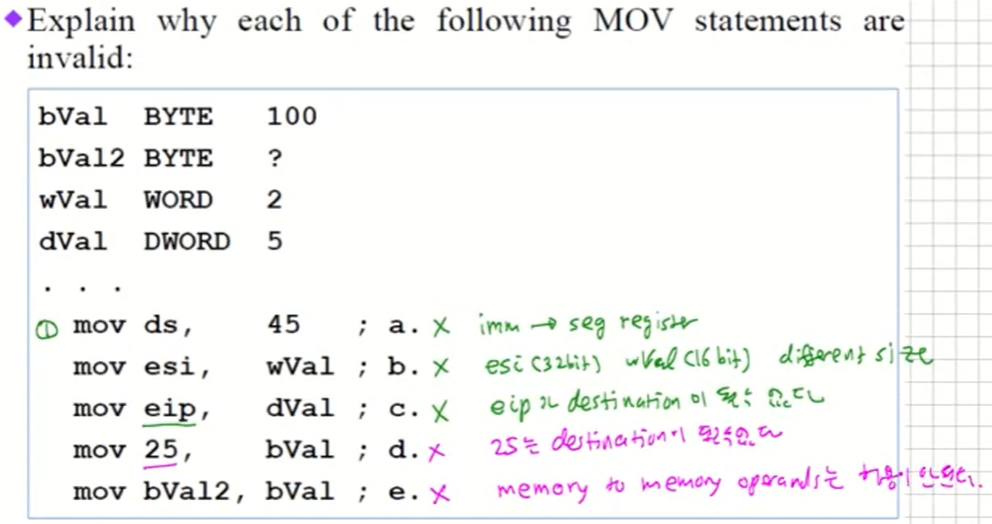
첫번째꺼는 immediate를 seg register에 넣을려고 했다.
esi(32bit)에 wVal(16bit) 사이즈가 다르다.
eip가 destination 이 될 수 없다.
25는 destination이 될 수 없다.
memory to memory operand는 허용이 안된다.
Reminder
- 프로그램 작성에 필요한 주된 Operations
- Arithmetic
+, -, x, /, % : add, sub, mul, div, mod
- Logical
and, or, nor, xor
- Data Transfer
MOV Instruction : =
- Program Control
if, for, etc.
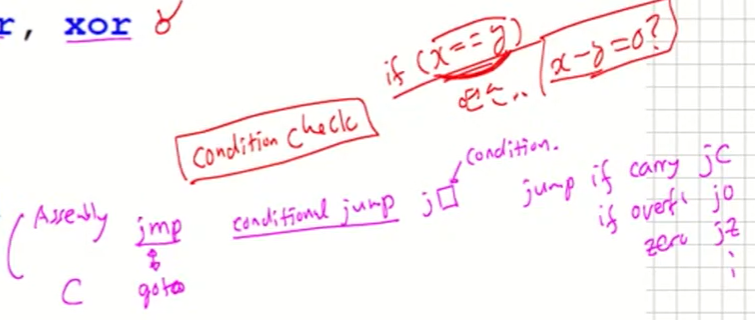
- Input / Output
어셈블리도 외부에 입출력하는게 어렵기 떄문에 library를 사용한다.
Instructions for Bit Extension
- Zero Extension (MOVZX instruction)
mov ax, bl 할 경우 오류가 난다. 하지만 이걸 꼭 옮기고 싶을때 destination이 물론 더 커야 한다. 이때 MOVZX를 쓰면된다.
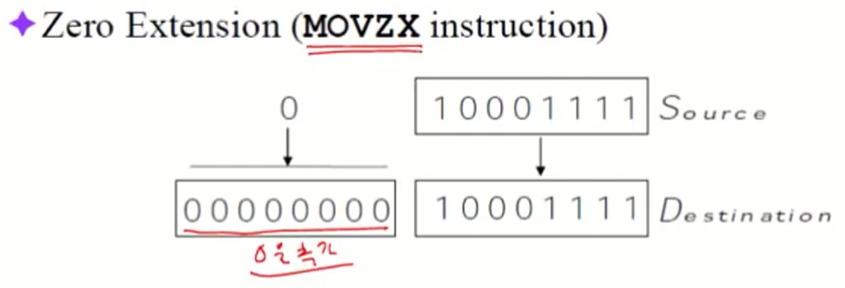
이때 destination은 반드시 레지스터여야한다.
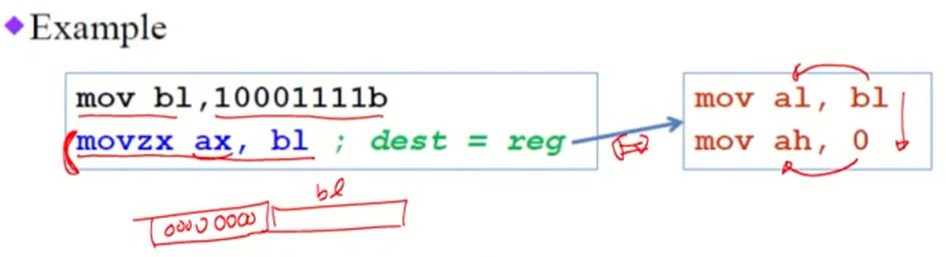
- Sign Extension (MOVSX instruction)
Signed integer : 2's complement를 사용 이떄 MSB는 sign bit이다. 만약 이걸 확장하고자 한다면 sign bit를 모두 복사한다.
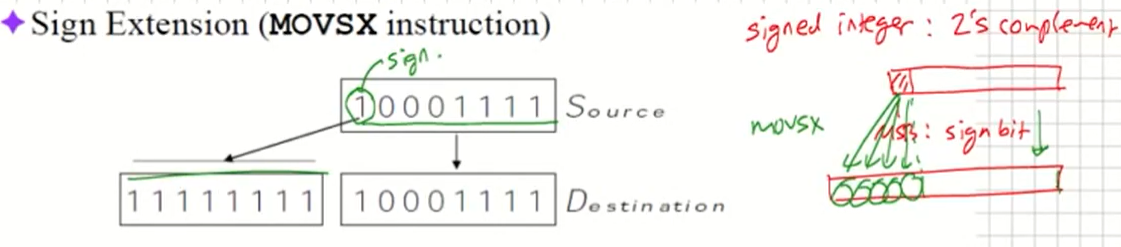
이때도 desination은 반드시 레지스터여야한다.
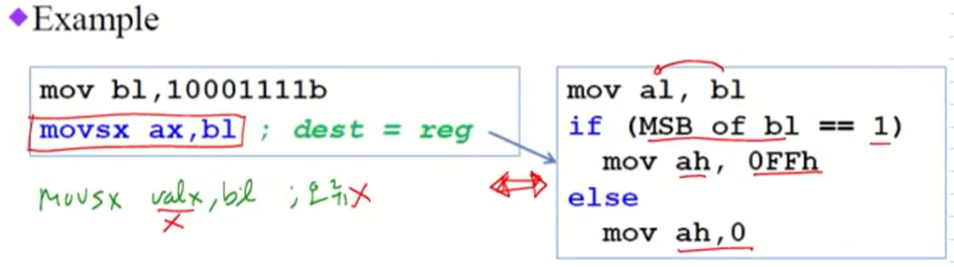
XCHG Instruction
-
Exchange the values of two operands. : 두개의 operand를 바꾸는 것
-
At least one operand must be a register. : 최소 1개는 레지스터여야 한다.
-
No immediate operands are permitted. : immediate operand 사용 불가
-
Example
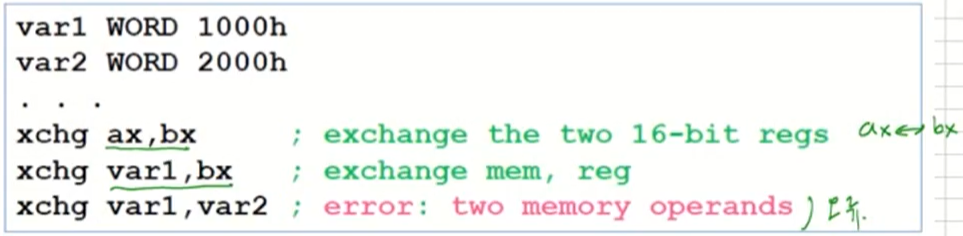
-
Programming 연습
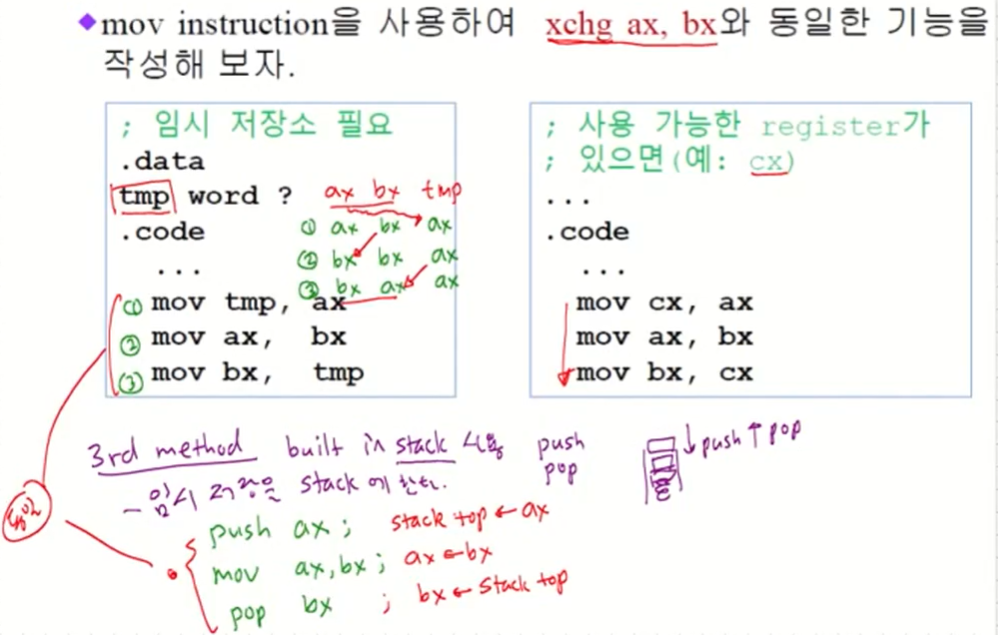
-
16 bit 메모리 변수 var1과 var2 값을 서로 바꾸려면?
- xchg var1, var2는 사용 불가능(오류)
- Memory to memory move도 사용 불가능
- 따라서, 사용 가능한 register가 최소 한 개는 있어야 한다.
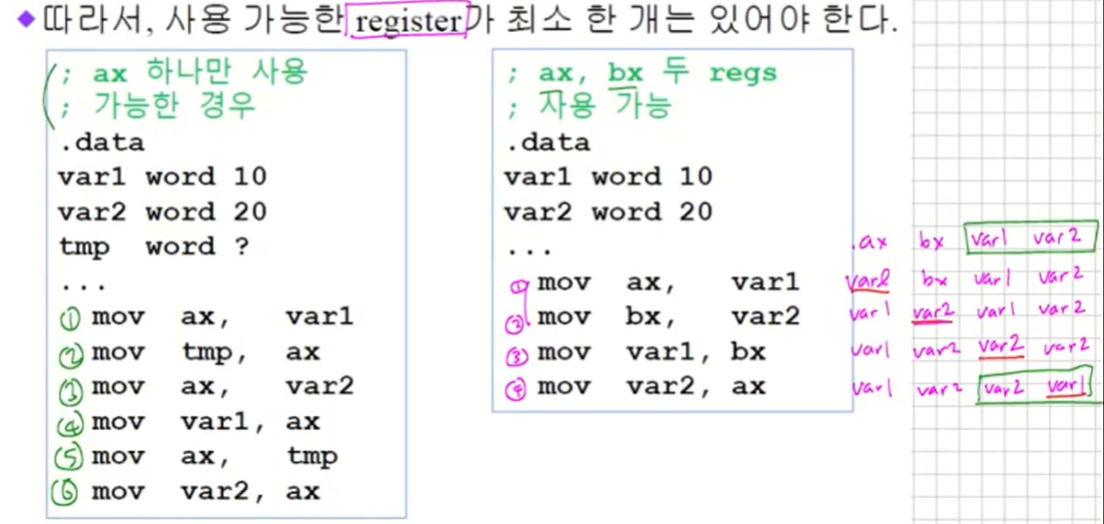
- 사용 가능한 register가 없는 경우, register 하나를 임시 저
장 후 사용.
- 또는 stack을 사용하여 교환할 수 있다.
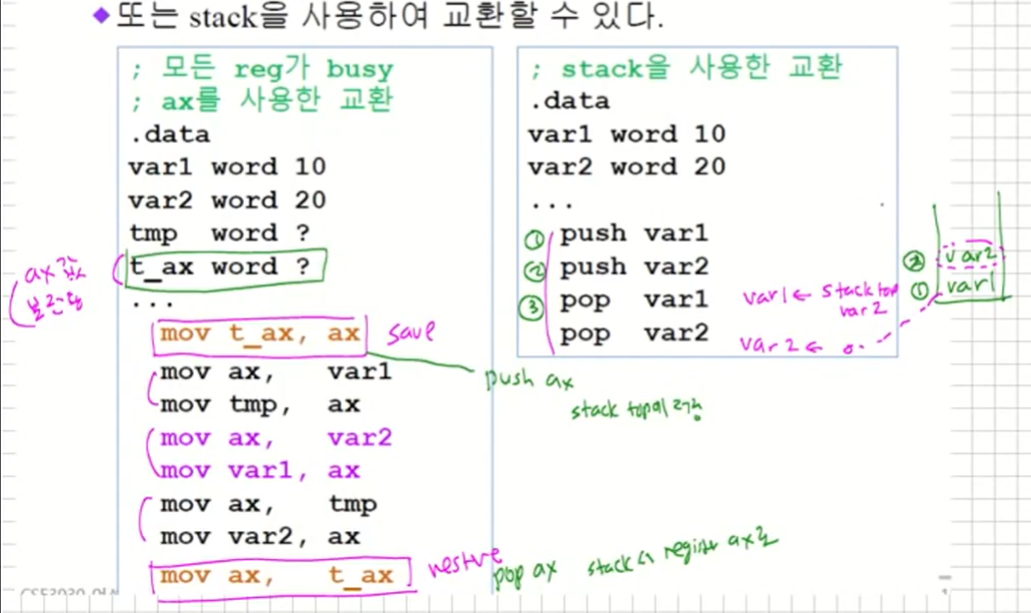
결론은 별로 쉬운 방법이 아니다.
Direct-Offset Operands
- A constant offset is added to a data label to produce an effective address (EA).
- Format: [var + constant(offset)]
- Example
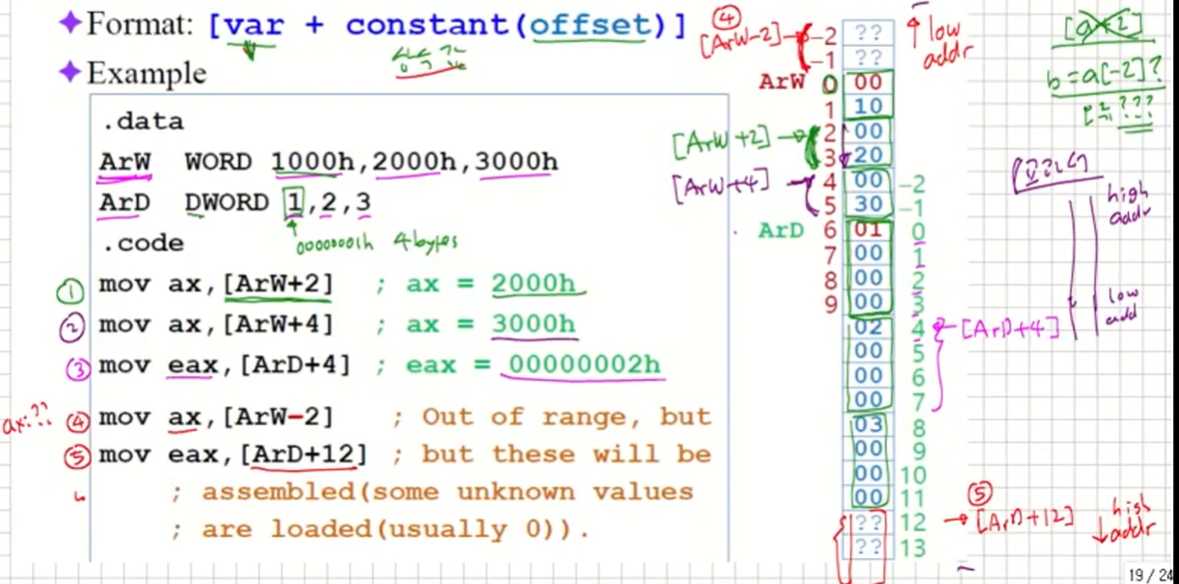
Operand와 register도 같아야 한다. 하지만 WORD PTR이라는 것도 있어서 이것도 강제로 바꿀 수는 있다.
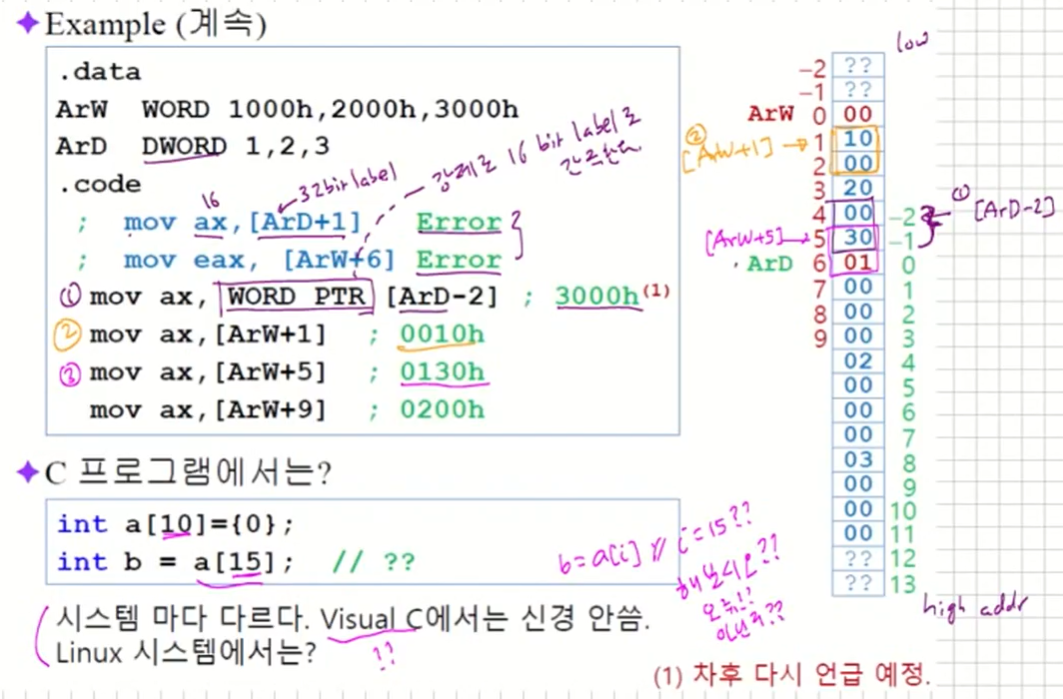
프로그래밍 연습
-
Write a program that rearranges the values of three doubleword values in the following array as: 3, 1, 2.
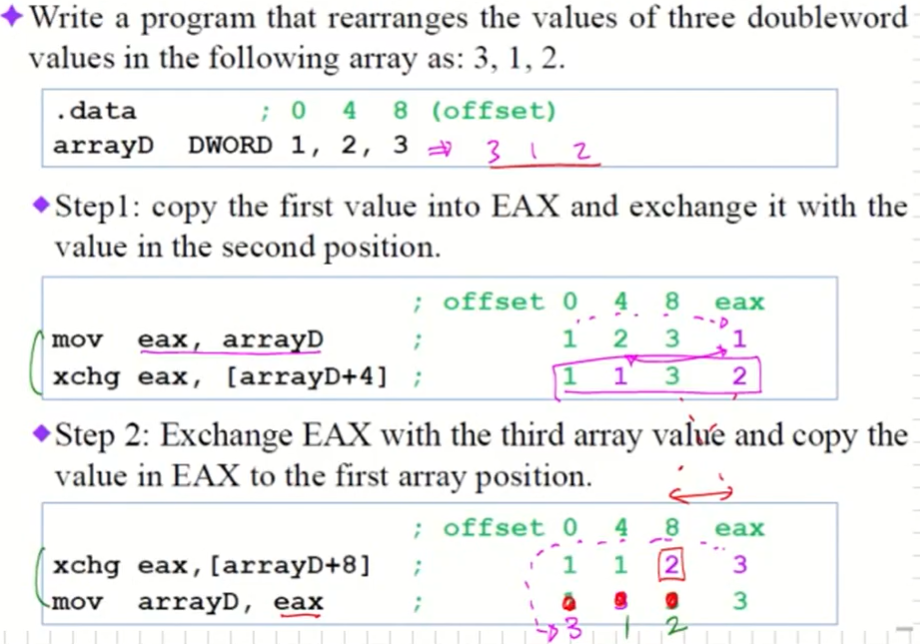
-
Write a program that adds the following three bytes:
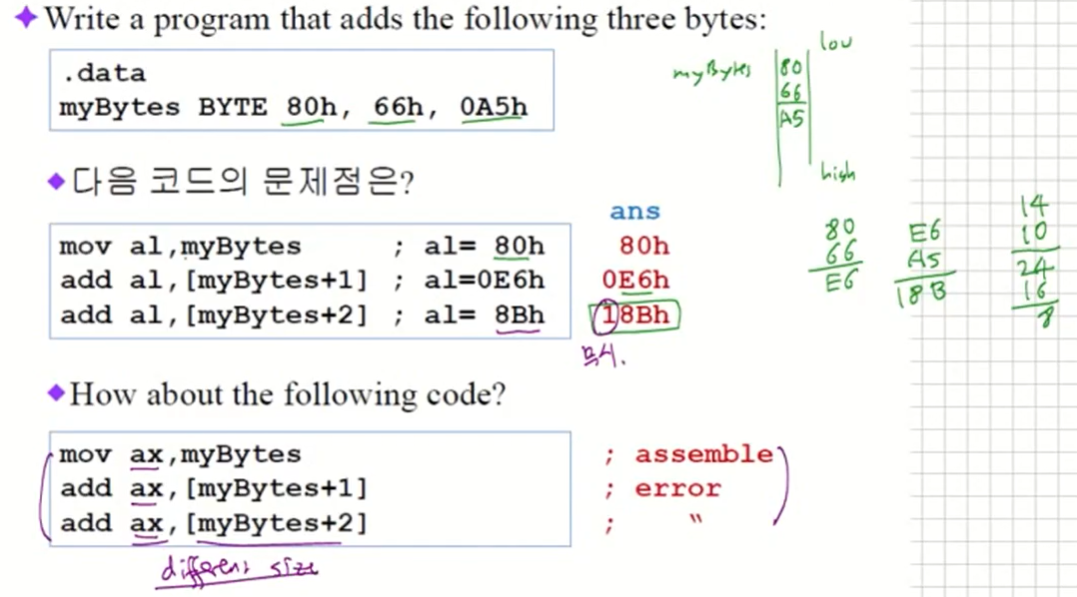
답은 18Bh가 되는데 메모리때문에 가장 앞자리는 무시가 된다. 이런 경우를 보고 overflow났다고 이야기 한다.
그래서 register의 크기를 키우면 이건 assemble 에러가 난다.
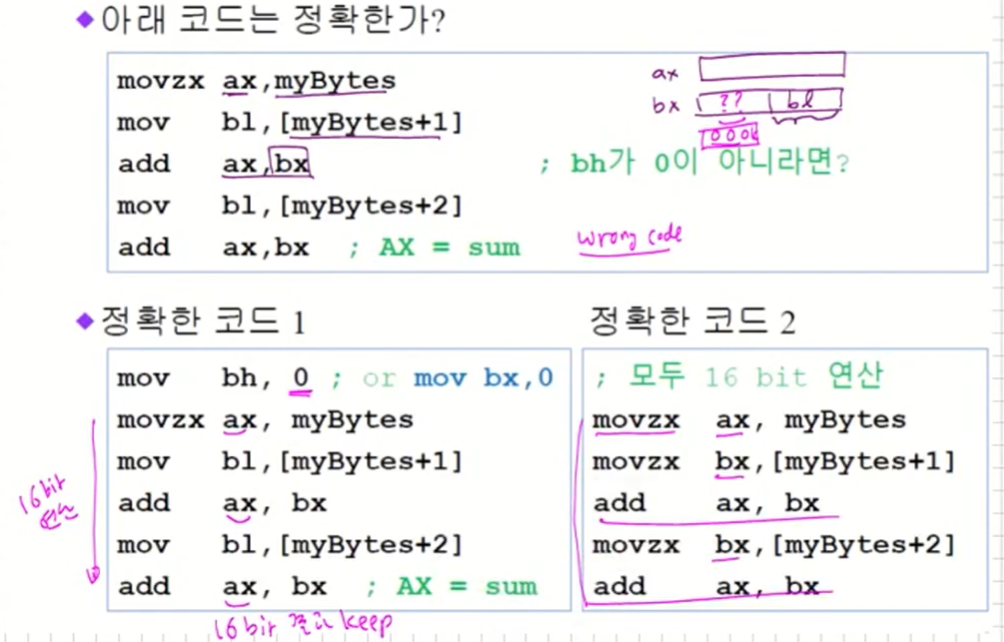
ah값이 어떠한지 모르기 때문에 이 경우에 에러가 날 수 있다.