1. Addition and Subtraction
덧셈과 뺄셈에 관련된 연산들
- INC and DEC Instructions : ++, --
- ADD and SUB Instructions
- NEG Instruction : -x
- Arithmetic Expressions
- Flags Affected by Arithmetic
- Zero(==0 일때 1로 셋팅 : ZF), Sign(음수인지 확인 : SF), Carry(캐리가 있는지 확인, unsigned 연산에서 overflow : CF), Overflow(오버플로우인지 확인, signed 연산에서 overflow : OF)
- 추가적으로 parity flag(LSB쪽 8bit 1의 갯수가 짝수이면 set : PF), AuxiliaryCarryFlag(하위 4bit의 carry가 올라가게 된다 : AF), Direction Flag(memory copy, move시 address가 increasing/decreasing 하는지 결정 : DF), Interrupt Enable(우리가 set)
Flags
- Flag의 사용
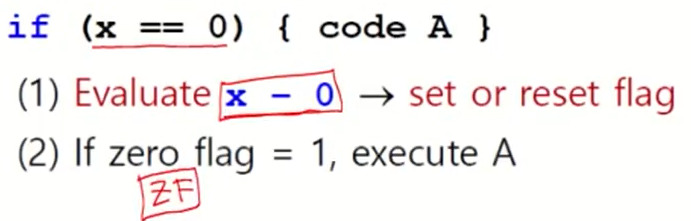
- CPU의 역할?
- 연산 후 side effect로 flag를 설정한다.
- Signed(overflow), unsigned(carry) 연산 모두를 고려하여 flag을 설정한다. : signed 이든 unsigned 이든 구분안하고 flag를 일단 설정
- Flag을 이용하는 것은 프로그래머의 몫이다.
INC and DEC Instructions
-
Add one to, subtract one from the destination operand : +1 또는 -1
-
Operand may be register or memory : operand가 레지스터 또는 메모리가 된다.
-
Format
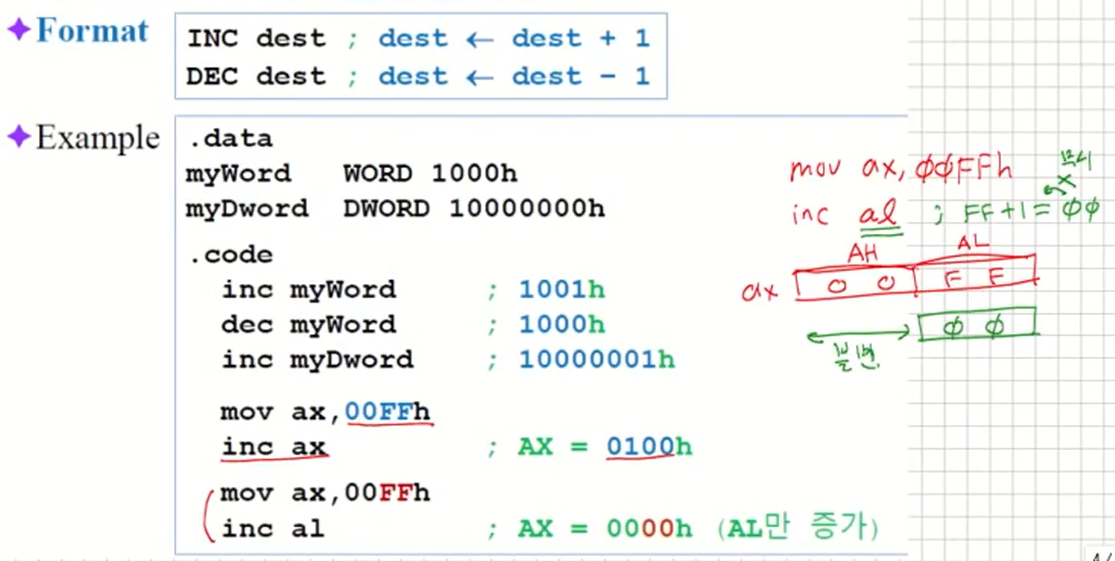
-
Example
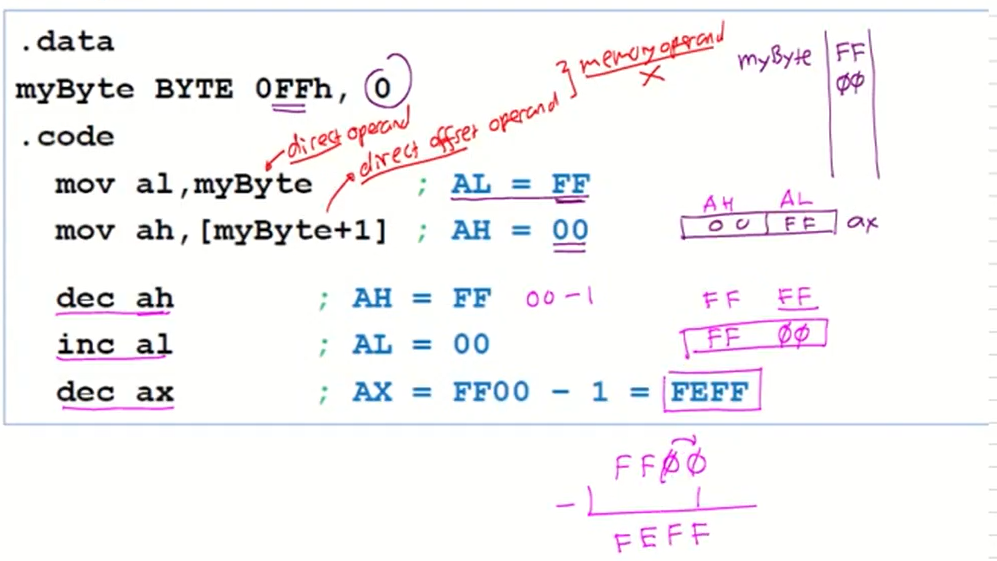
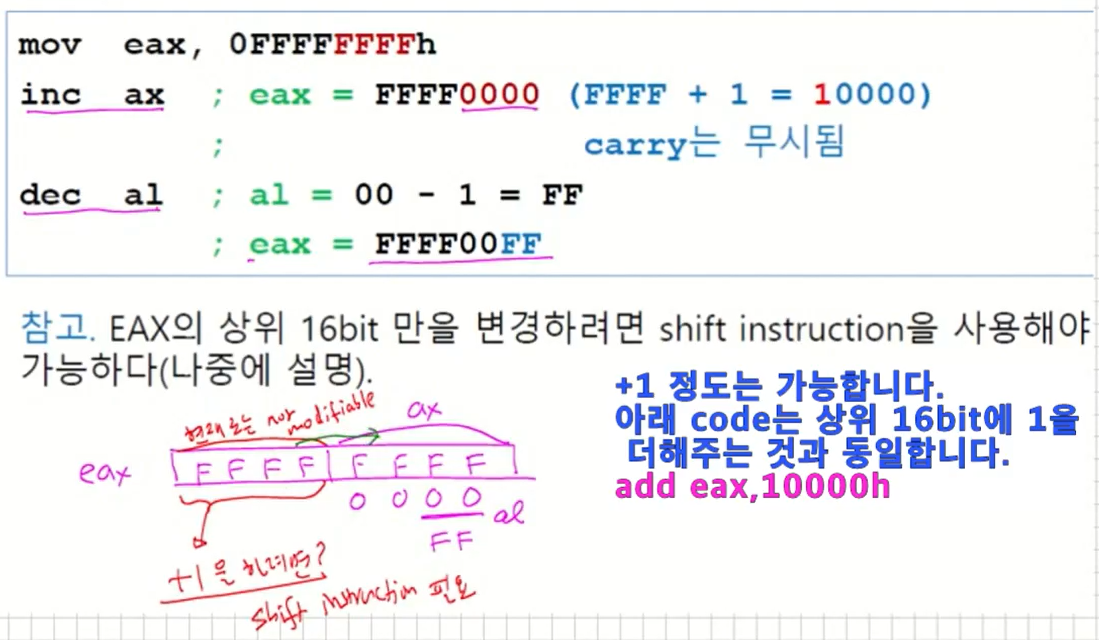
ADD and SUB Instructions
- Format

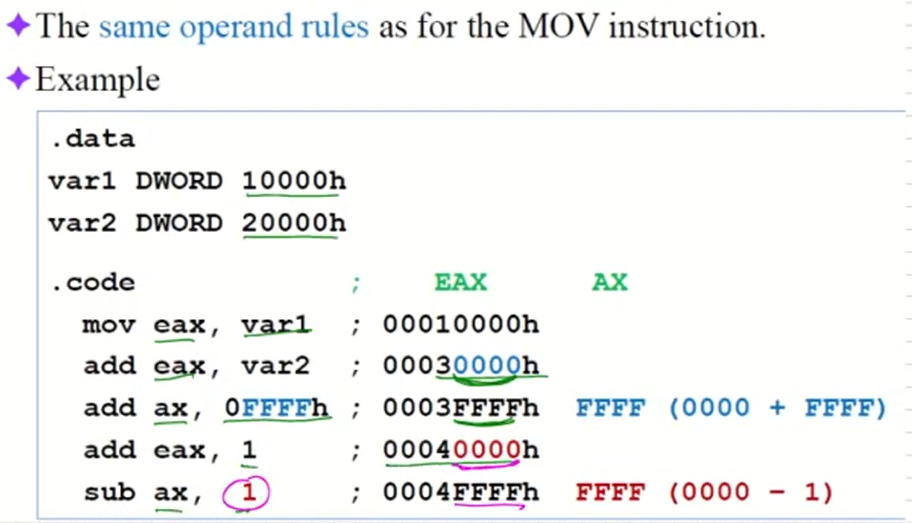
NEG (negate) Instruction : -x
- Reverses the sign of an operand (two’s complement) : 2의 보수를 취한걸로 생각한다.
- Operand can be a register or memory operand
- Internally, SUB 0,operand
- Any nonzero operand causes the Carry flag to be set. : operand가 0이 아니면 CF는 1, 0이면 CF는 0
- Example:
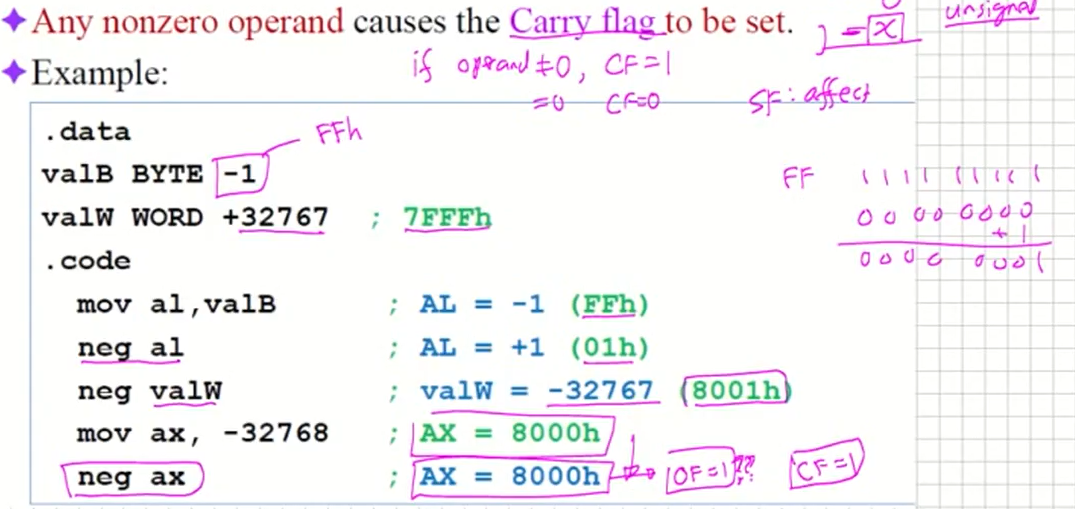
마지막 neg ax는 OF=1, CF=1이 되어 있을 것이다.
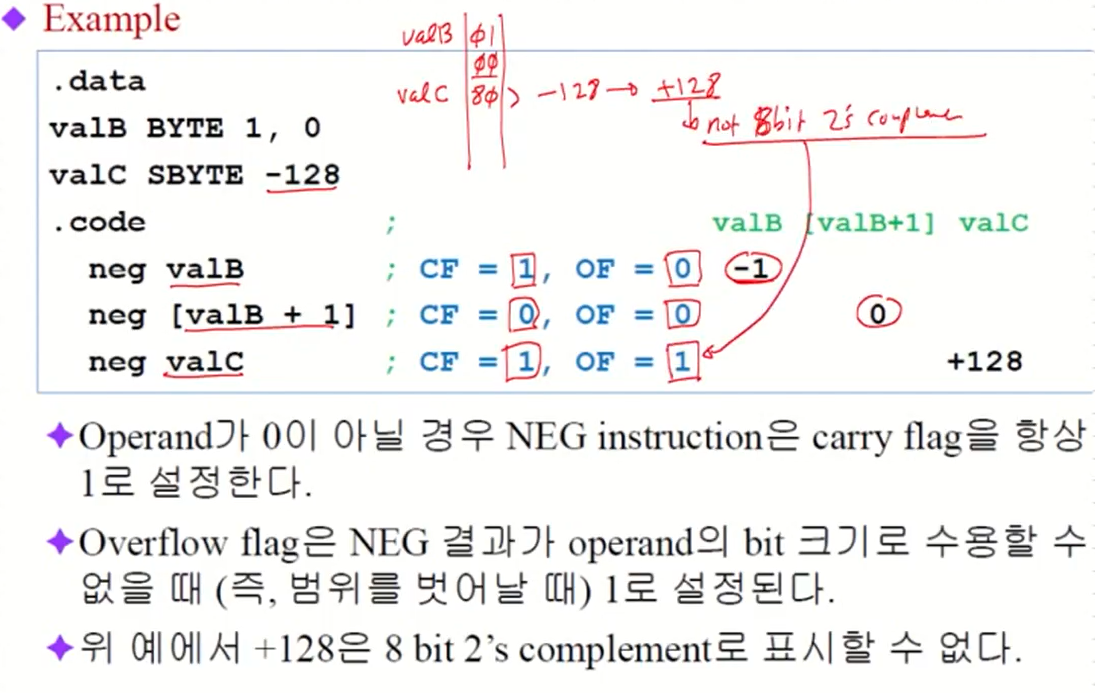
2. Arithmetic Expressions
Implementing Arithmetic Expressions
가정하기를 no overflow라고 하자
-
Example : Rval = -Xval + (Yval – Zval)
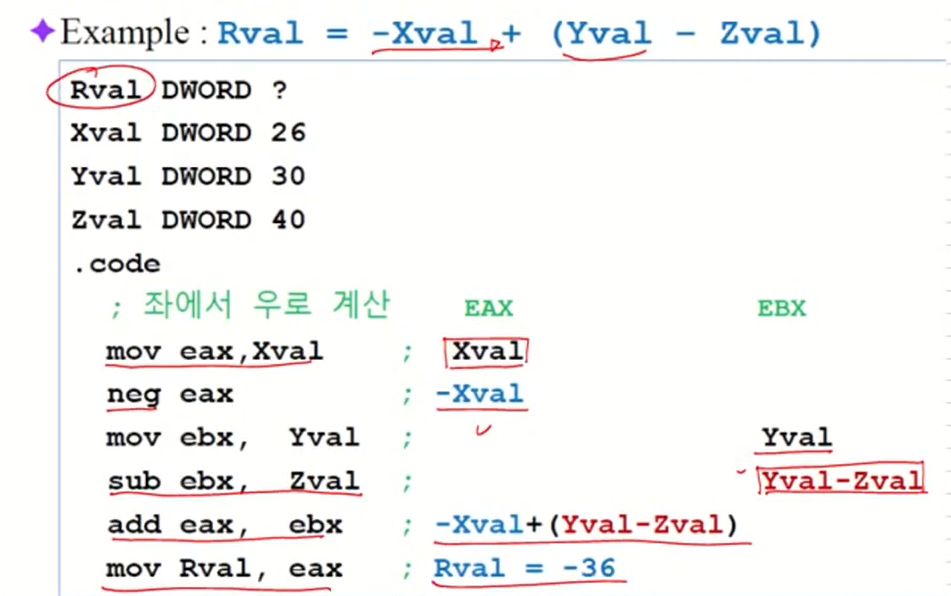
-
Example : Rval = -Xval + (Yval – Zval) (계속)
- 여러 다양한 프로그래밍이 가능하다.
- 레지스터 eax 만 사용할 경우(괄호를 무시해야 한다) : 이 경우 위에서 나온 instruction의 갯수를 줄일 수 있다.
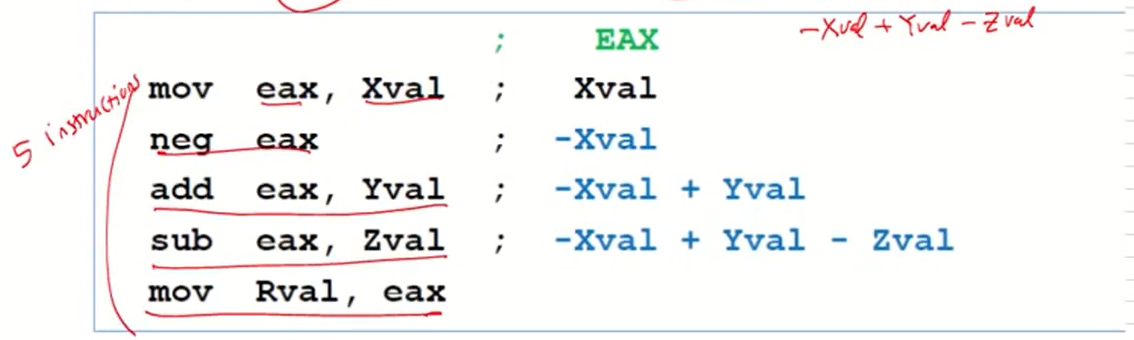
-
Example : Rval = -Xval + (Yval – Zval) (계속)
- 최소 개수의 instruction 만을 사용할 경우(계산 순서를 조정한다) : 즉 뺄샘과 음수는 무조건 마지막에 계산한다.
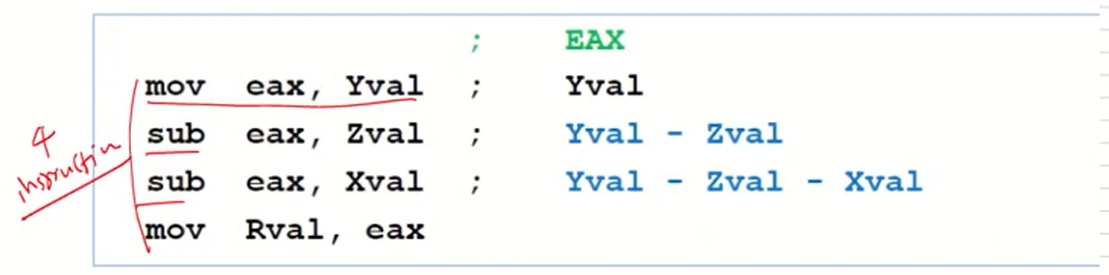
-
Example: Rval = Xval - (-Yval + Zval)
- 괄호 먼저, 계산은 좌에서 우로 진행할 경우
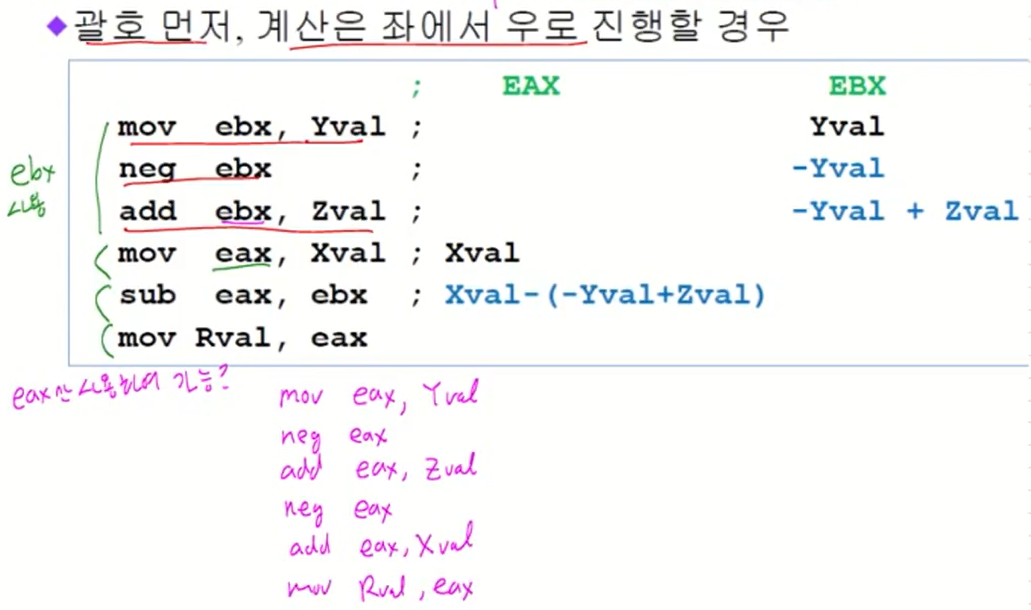
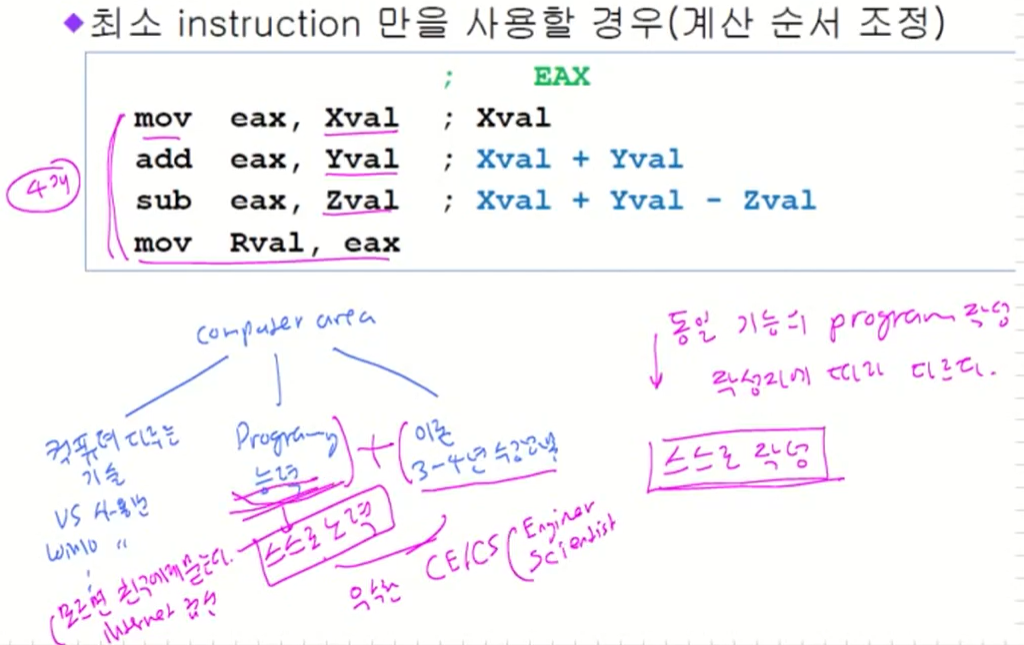
3. Flags
Flags Affected by Arithmetic
- The ALU has a number of status flags that reflect the outcome of arithmetic (and bitwise) operations.
- based on the contents of the destination operand.
- Essential flags: (아래 4가지가 중요)
- Zero flag – destination equals zero.
- Sign flag – destination is negative (결과 MSB가 1인 경우 set)
- Carry flag – unsigned value out of range.
- Overflow flag – signed value out of range
- Carry flag과 Overflow flag은 동시에 그러나 독립적으로 설
정된다.
- Carry flag은 unsigned 연산에, overflow flag은 signed 연산에 사용한다.
- 이들 flag의 사용은 전적으로 프로그래머의 몫이다.
- The MOV instruction never affects the flags. : MOV instruction은 flag에 영향을 주지 않는다.
Visual Studio
- 프로그램을 debug하면서 flag을 체크하려면 레지스터 창을 열어야 한다(debug mode에서만 가능). : watch window(조사식)
- Debug mode에서 -> 메뉴 바 -> 디버그(D) -> 창(W) -> 클릭 레지스터.
- 레지스터 창이 열리면 창의 위치를 조정.
- 레지스터 창 -> 창 내에서 마우스 우클릭 -> 체크 플래그
- 우측과 같이 flag 정보가 있는 레지스터 창이 보인다.
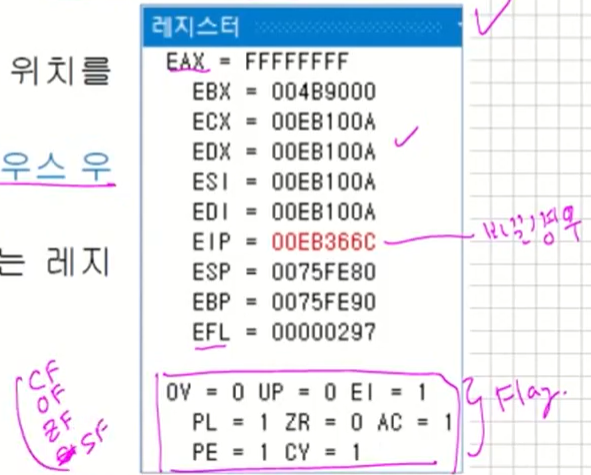
Visual Studio에서 Flag의 약어
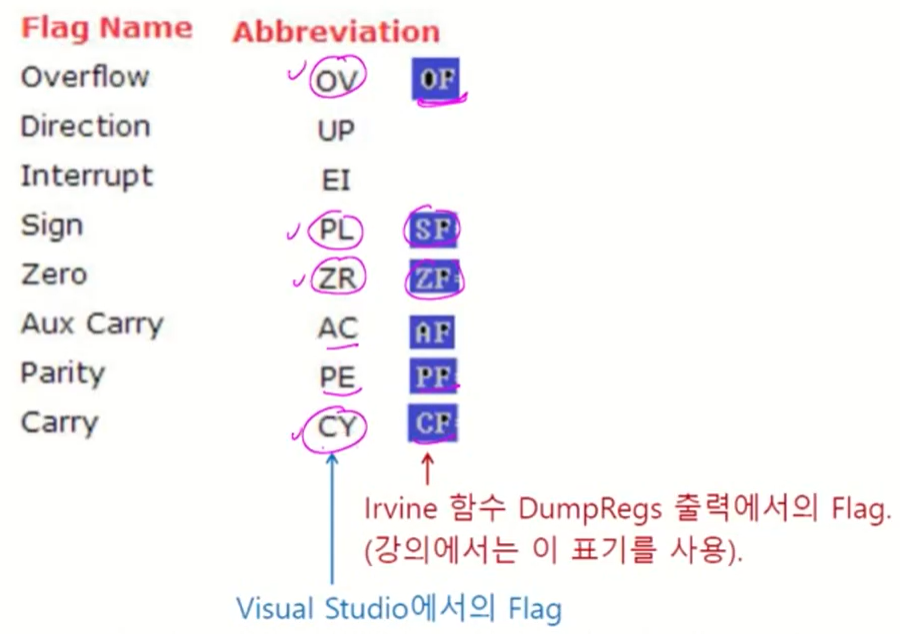
서로 용어가 다르기 때문에 잘알아두자
Concept Map
- 이미 언급하였지만, 연산 결과의 side-effect로 해당 flag을 설
정한다.
- 설정된 flag은 if 등을 구현할 수 있는 conditional jump instruction 등에 사용된다.
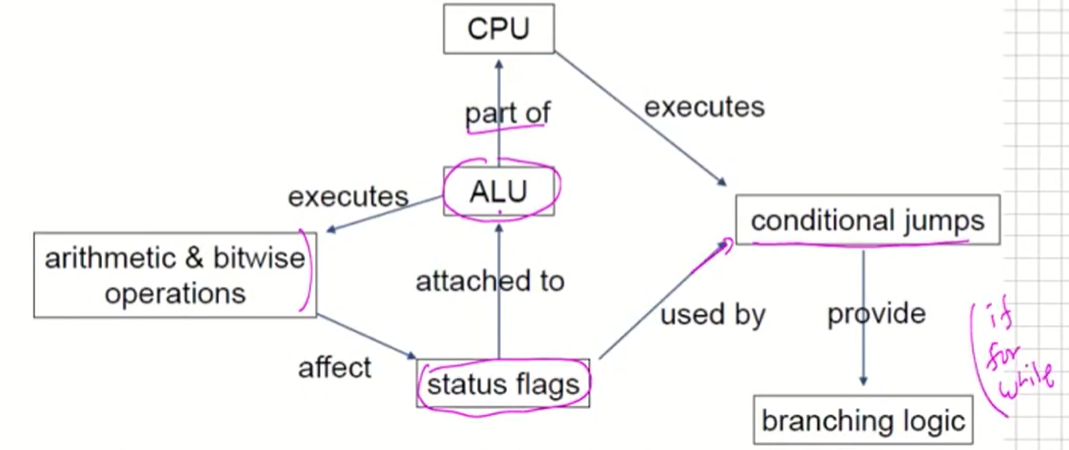
Zero Flag (ZF)
- Whenever the destination operand equals Zero, the Zero flag is set.
- Example
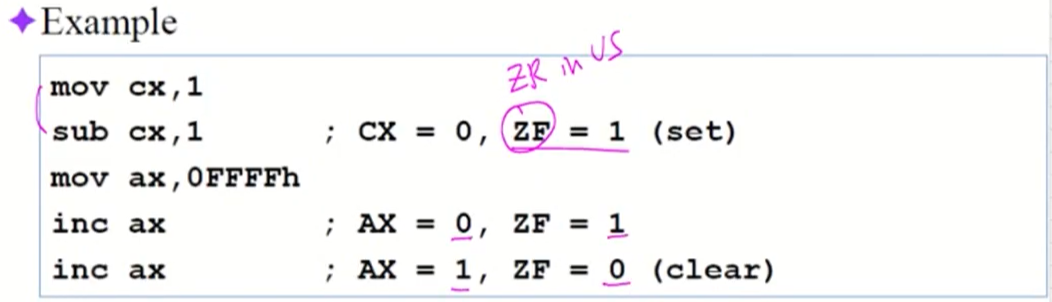
Sign Flag (SF)
- The Sign flag is set when the destination operand is negative.
- The flag is clear when the destination is positive.
- Example
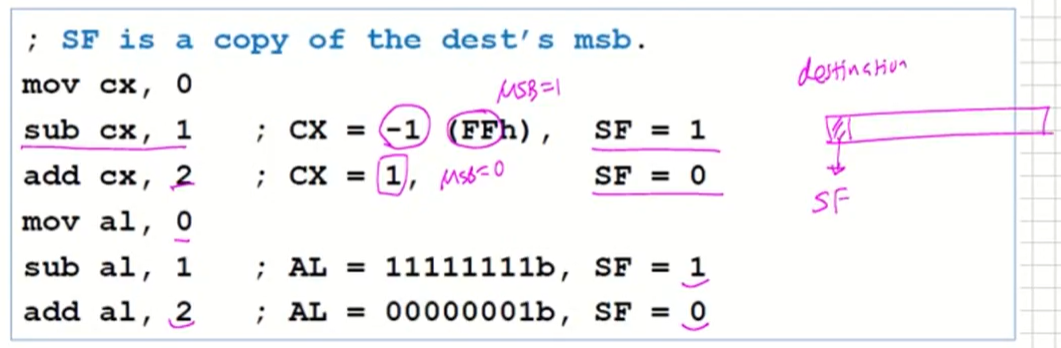
Signed and Unsigned Integers : A Hardware Viewpoint
- All CPU instructions operate exactly the same on signed and unsigned integers. : 모든 CPU instruction은 signed이건 unsigned이건 동일하게 작동한다.
- The CPU cannot distinguish between signed and unsigned integers.
- YOU, the programmer, are solely responsible for using the correct data type with each instruction(반복 강조).
4. Overflow Detection (in Unsigned & Signed)
Overflow Detection in Addition
정리
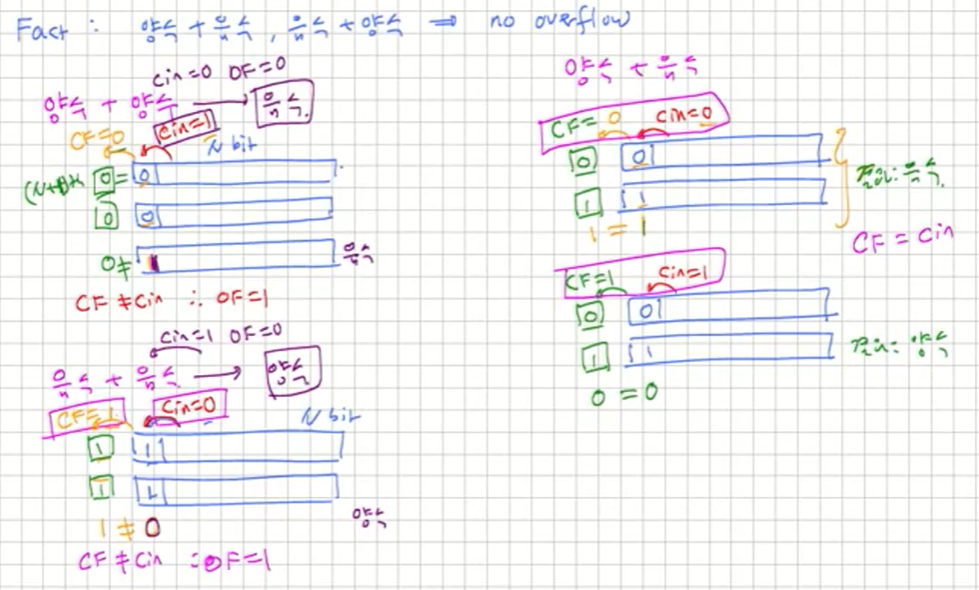
Unsigned Subtraction
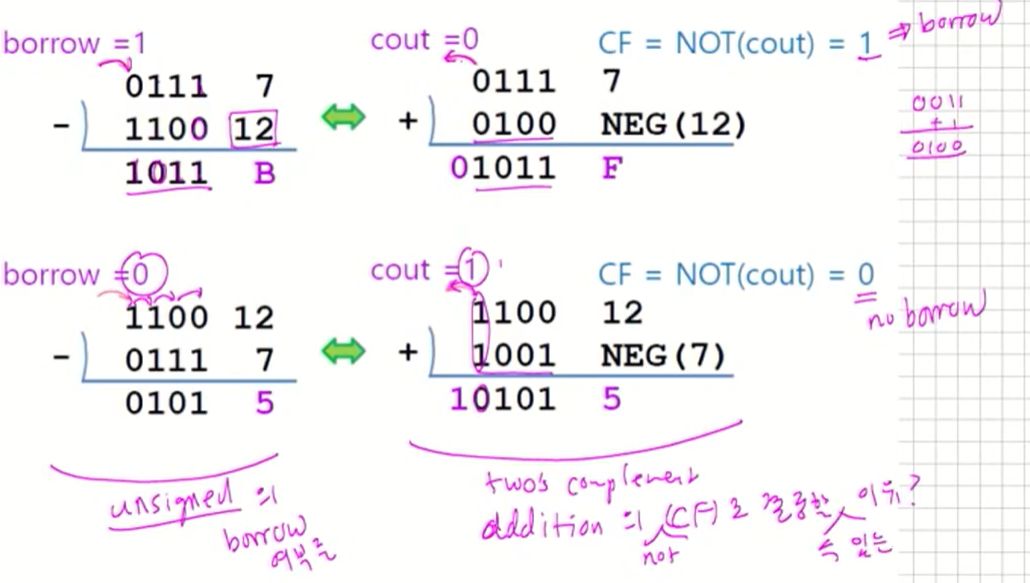
2의 보수를 뺏을때 borrow가 있으면 CF가 1이다. 라고 생각해주면 된다.
- Signed Subtraction
- Signed subtraction z = x – y 계산은 이미 알고 있는 바와 같이 다음과 같이 계산한다.

- Overflow flag OF는 signed addition의 경우와 동일하다.
5. Carry Flag & Overflow Flag
각 Flag에 대한 Example
- 지금까지는 hardware 관점에서 두 flag이 어떻게 설정되는지
알아보았다.
- 이제, 논리적인 관점에서 flag setting에 대해 알아본다.
- Carry Flag (CF)
- The Carry flag is set when the result of an operation generates an unsigned value that is out of range (too big or too small for the destination operand). : CF는 operation이 unsigned value를 생산하는데 범위 밖이면 무조건 생성된다.
- Unsigned Subtraction z = x – y의 경우 CF 설정 방법: unsigned 빼길일때

- Overflow Flag (OF)
- The Overflow flag is set when the signed result of an operation is invalid or out of range. : 결국 범위가 바뀔때 생기게 된다.
- 두 수를 더할 때 OF는 다음과 같은 경우에 set된다.
- 두 양수를 더한 결과가 음수일 때 (MSB = 1) : OF=1
- 두 음수를 더한 결과가 양수일 때 (MSB = 0)
Carry Flag (CF) Examples
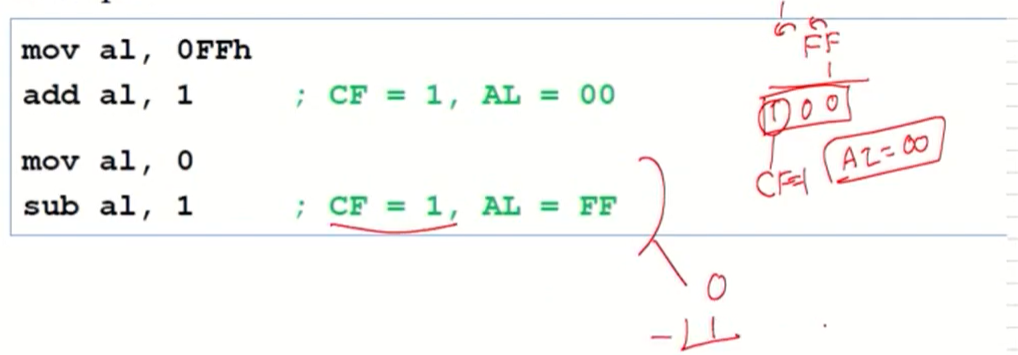
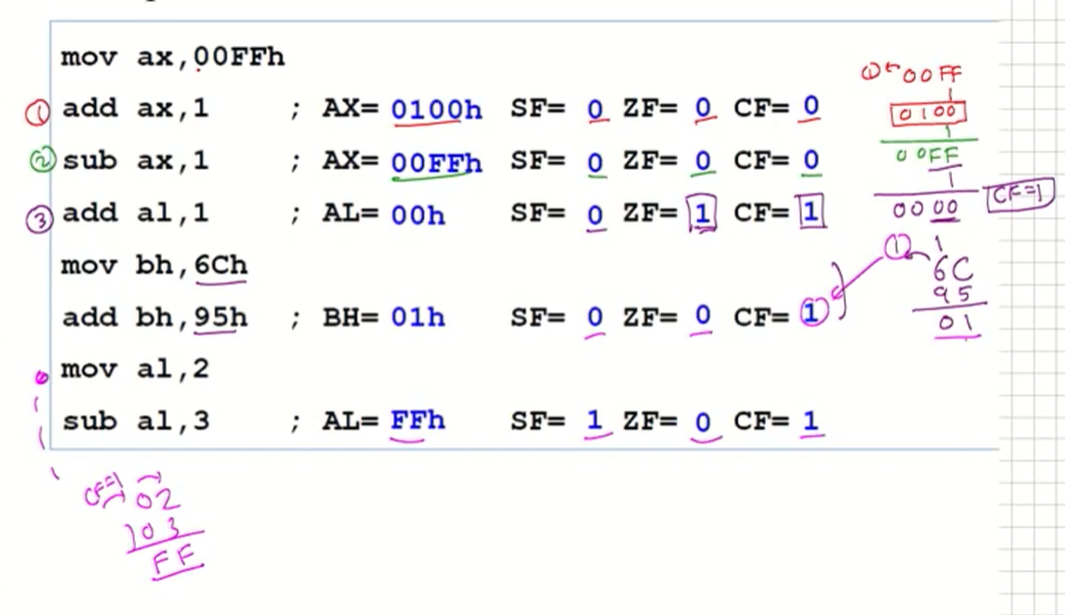
Overflow Flag (OF) Examples
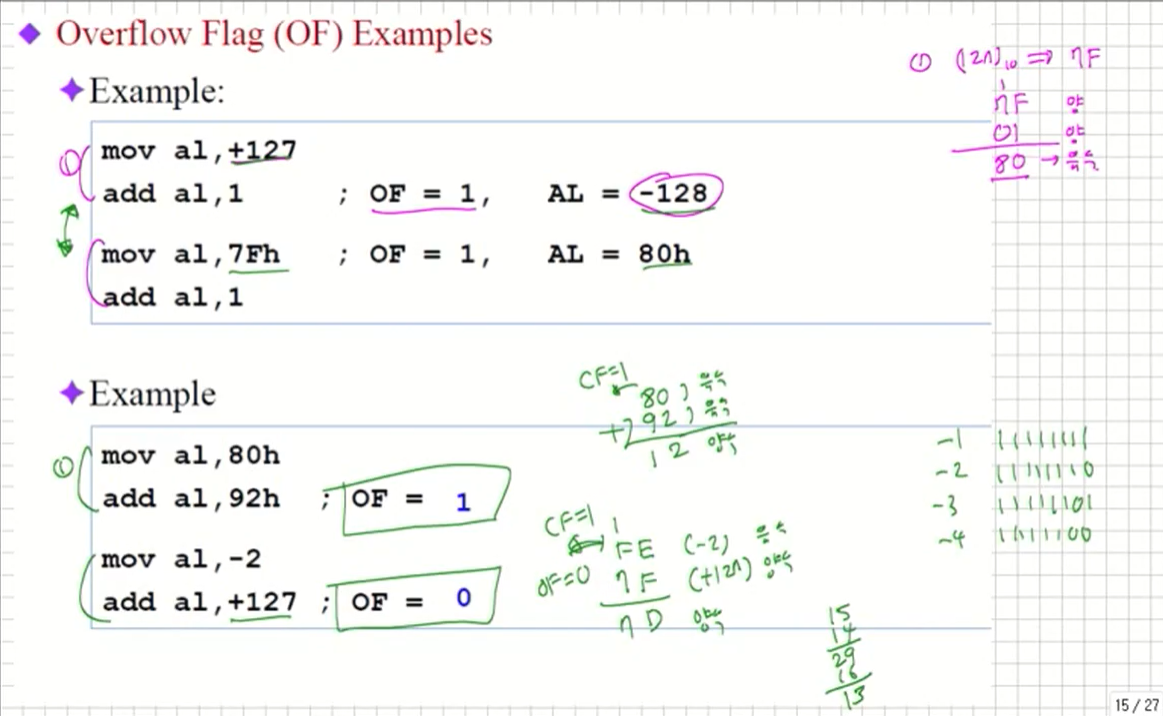
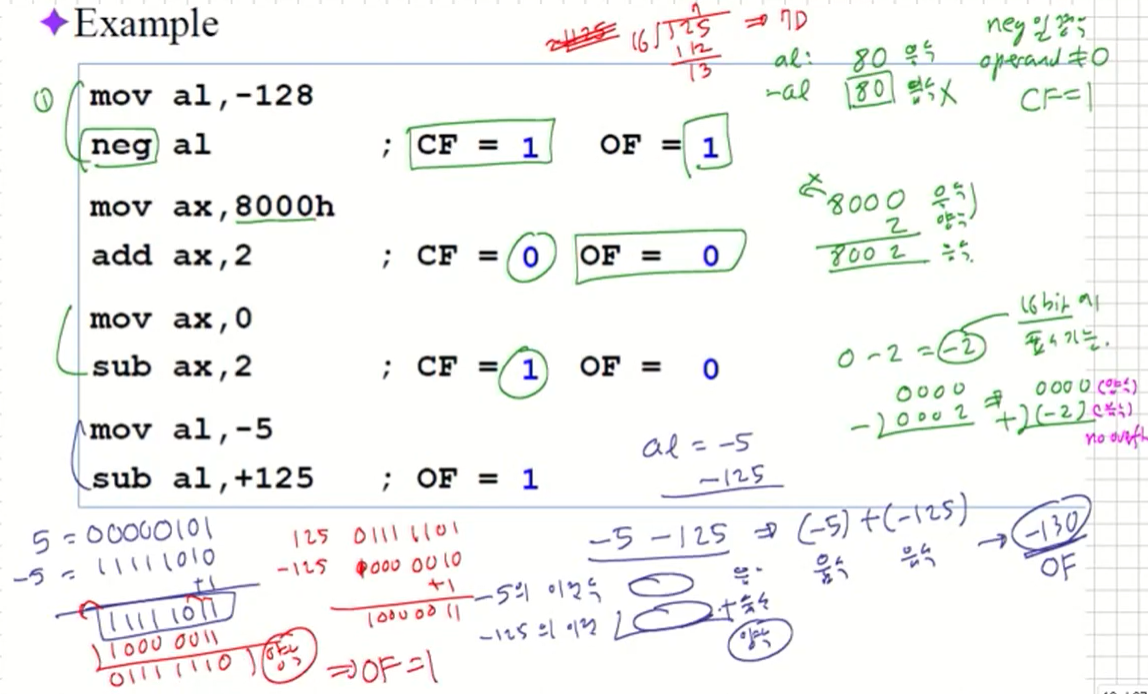
Flags Affected by Instructions
- INC & DEC Instructions
- The Overflow, Sign, Zero, Auxiliary Carry, and Parity flags are changed according to the value of the destination operand.
- Do not affect the Carry flag. : CF는 불변
- ADD, SUB and NEG Instructions
- The Carry, Zero, Sign, Overflow, Auxiliary Carry, and Parity flags are changed according to the value that is placed in the destination operand.