코드의 상수값을 알고싶을때 사용하게 된다.
- OFFSET Operator
- PTR Operator
- TYPE Operator
- LENGTHOF Operator
- SIZEOF Operator : C에서 sizeof 함수(byte 수 return)
- LABEL Directive : Directive
OFFSET Operator
-
OFFSET returns the distance in bytes, of a label from the beginning of its enclosing segment : 기준점을 중심으로 얼마나 떨어져 있는가. offset이기도 하고 index이기도 하고.
- Protected mode: 32 bits : 4GB 까지 가능
- Real address mode: 16 bits : 64K 까지 가능
모든 것이 다 seg:offset에 의해 결정이 된다.
-
The Protected-mode programs we write only have a single
segment (we use the flat memory model). : protected-mode 에서는 flat memory model이기 때문에 세그먼트 시작에서부터 얼마만큼. 그래서 memory address는 상대주소를 의미한다. 하지만 실제주소는 VS에서 &(variable)을 이용해서 실제주소를 알 수 있다.
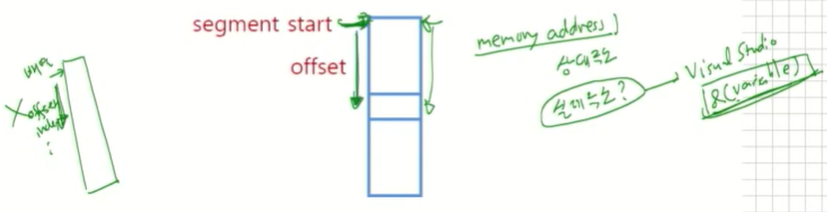
-
Assemble 후 프로그램 시작은 0이라고 간주(~.lst 파일).
-
Memory에 load하면 실제 메모리 주소로 재배치된다.
- Offset 값도 이에 따라 실제 메모리 주소로 변경된다.
- 실행할 때마다 해당 segment가 load되는 위치가 다를 수 있기 때문에, offset 값은 매번 다를 수 있다.
-
C 언어에서는 pointer value가 offset에 해당된다(아래 코드에
서 p에 저장된 값).
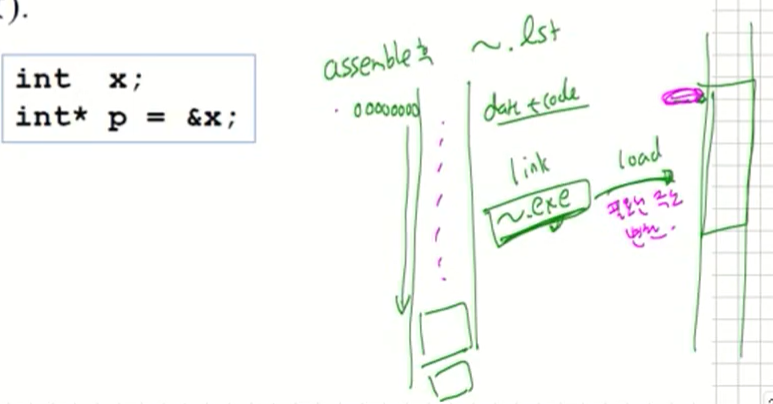
-
Example
- 아래 예에서는 segment 시작 위치가 00404000h라고 가정한다.
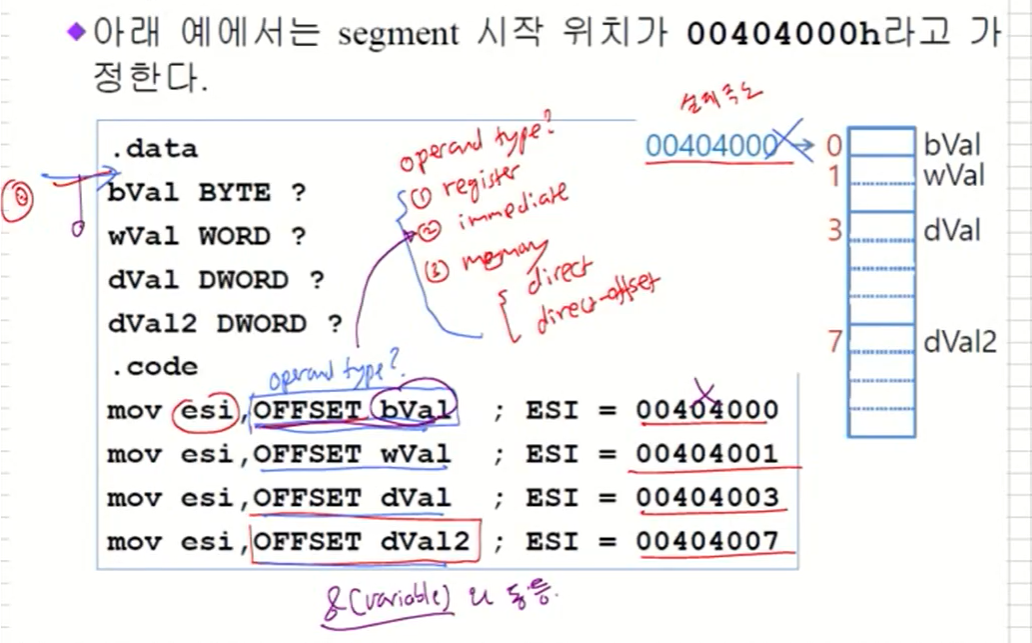
-
Relating to C/C++
- The value returned by OFFSET is a pointer.
- Compare the following two codes written for both C++ and assembly language:
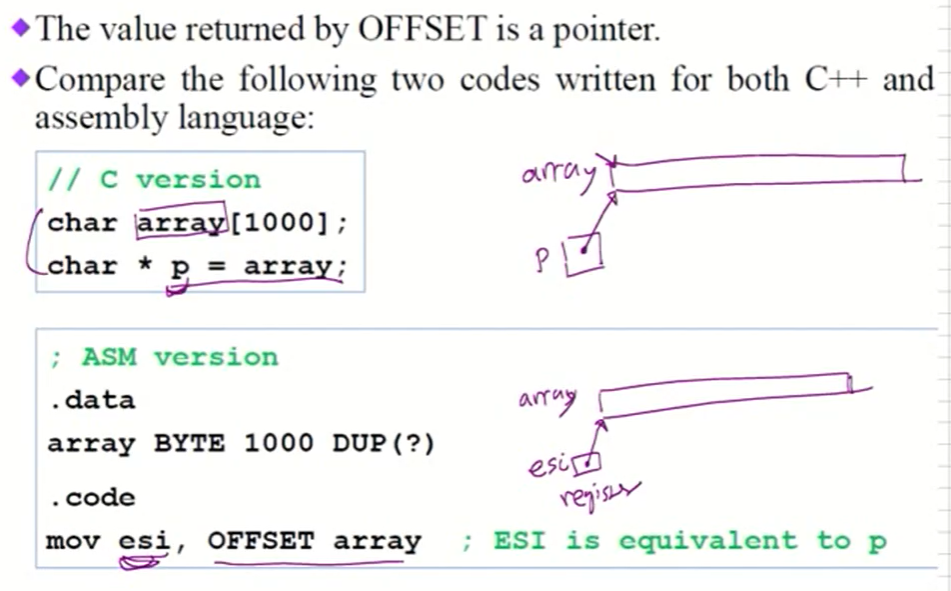
PTR Operator
- Overrides the default type of a label (variable) (=type casting). : type casting operator이라고 볼 수 있다. Size가 다른경우 PTR을 이용해서 아무 사이즈나 받을 수 있게 만들어준다.
- Provides the flexibility to access part of a variable.
- Example
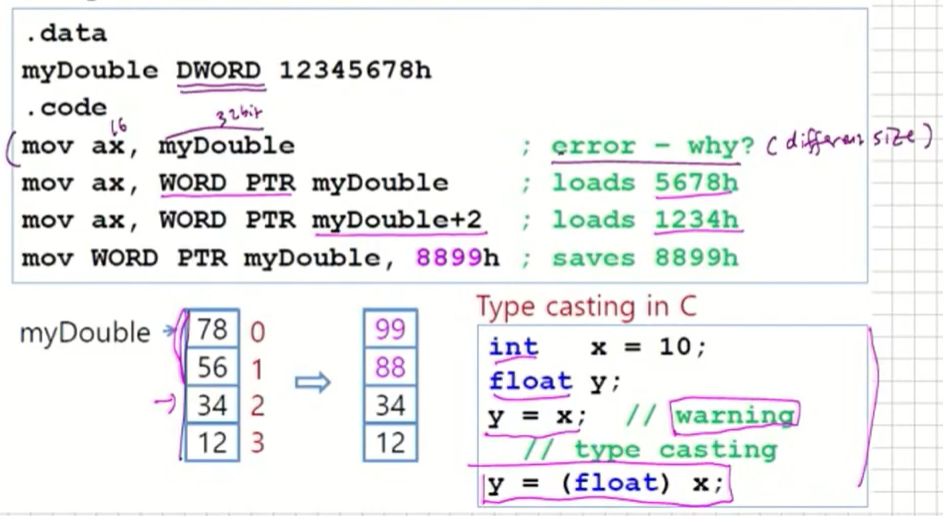
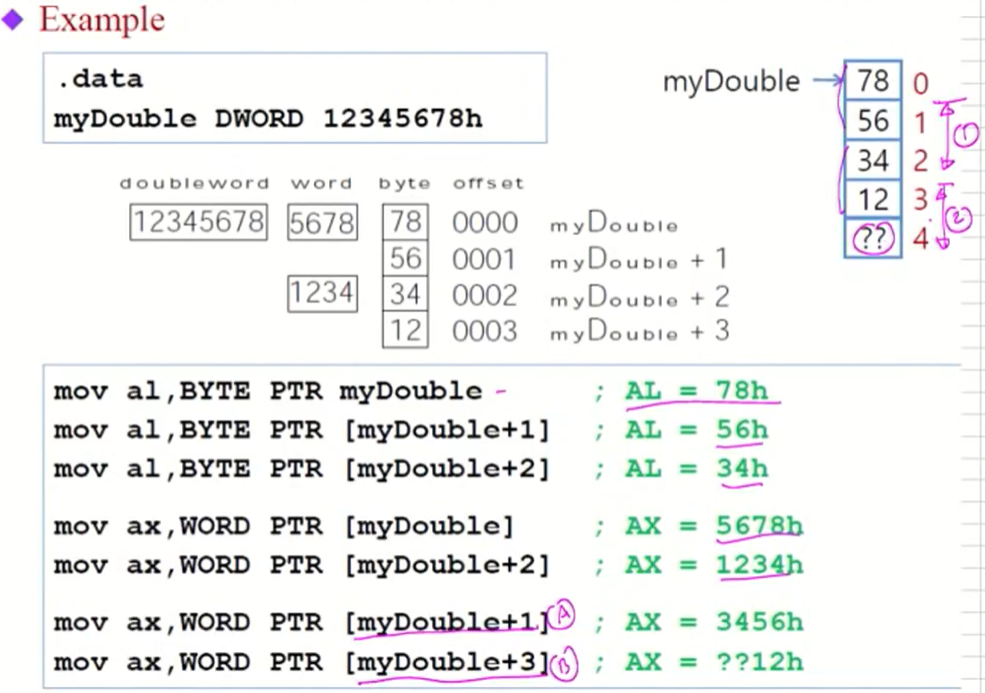
- PTR can also be used to combine elements of a smaller data type and move them into a larger operand.
- The CPU will automatically reverse the bytes.
- Example
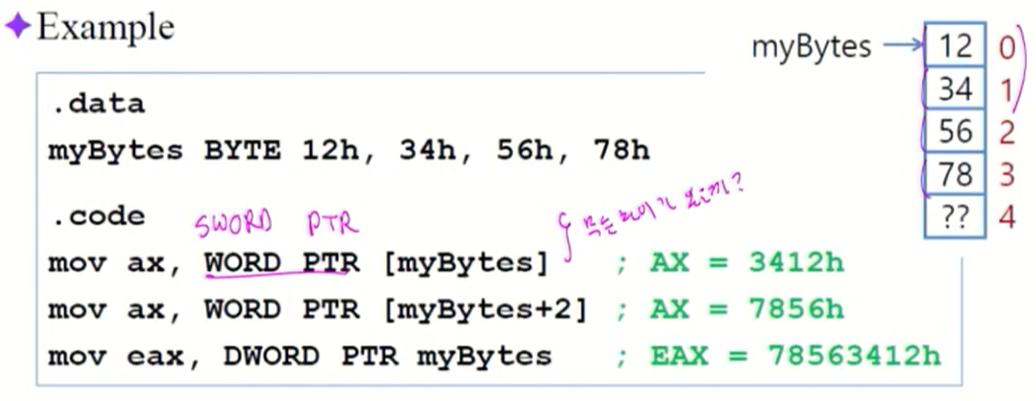
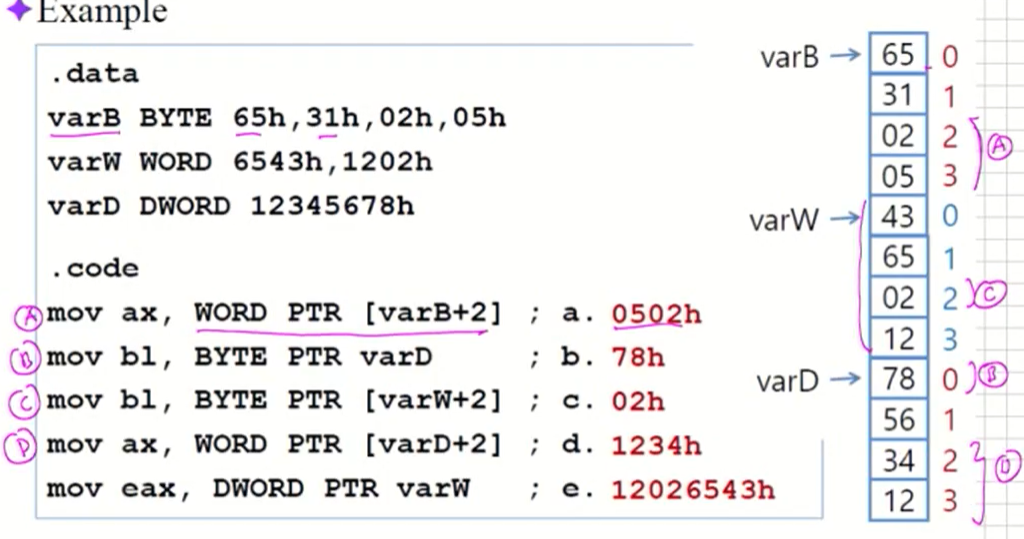
TYPE Operator
- Type Operator returns the size, in bytes, of a single element of adata declaration. : 뒤에 ? 작성시 공간만 할당해준다. 이때 TYPE operand를 사용하면 sizeof(int)와 동일한 효과를 얻을 수 있다.
-Example
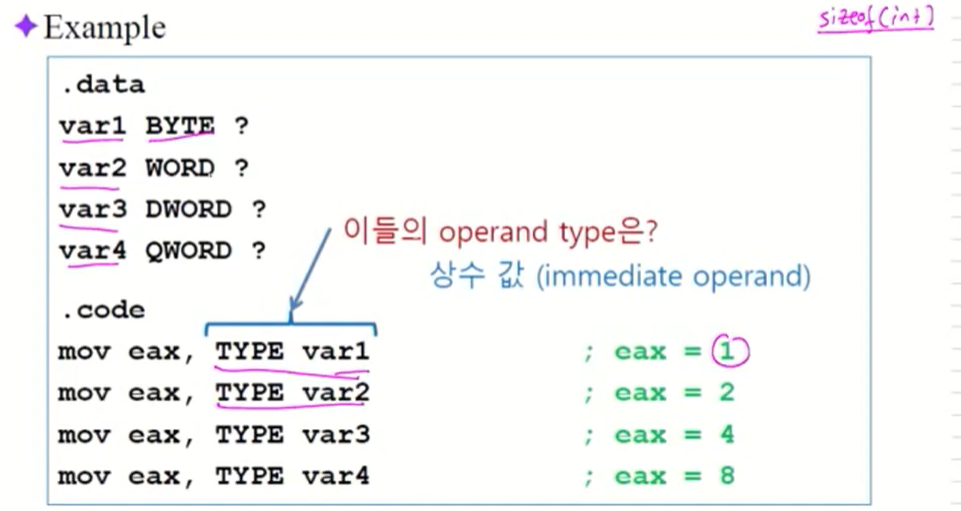
LENGTHOF Operator
- LENGTHOF operator counts the number of elements in a single data declaration. : 데이터의 갯수
- Example
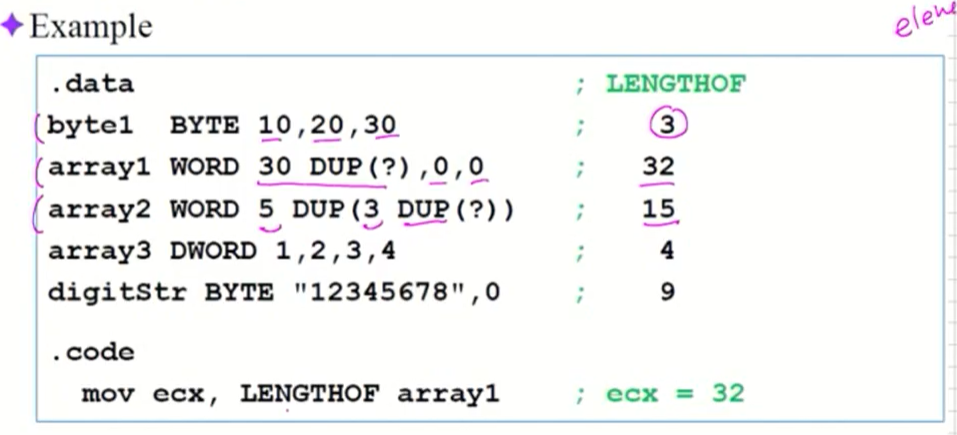
SIZEOF Operator
- SIZEOF operator returns a value that is equivalent to multiplying LENGTHOF by TYPE (size in bytes). : C의 sizeof와 동일. 여기에서 TYPE과의 차이점은 TYPE은 TYPE에 맞는 크기만 주지만 SIZEOF는 TYPE과 LENGTHOF를 곱한 메모리를 차지한 총 크기를 알려준다.
-Example
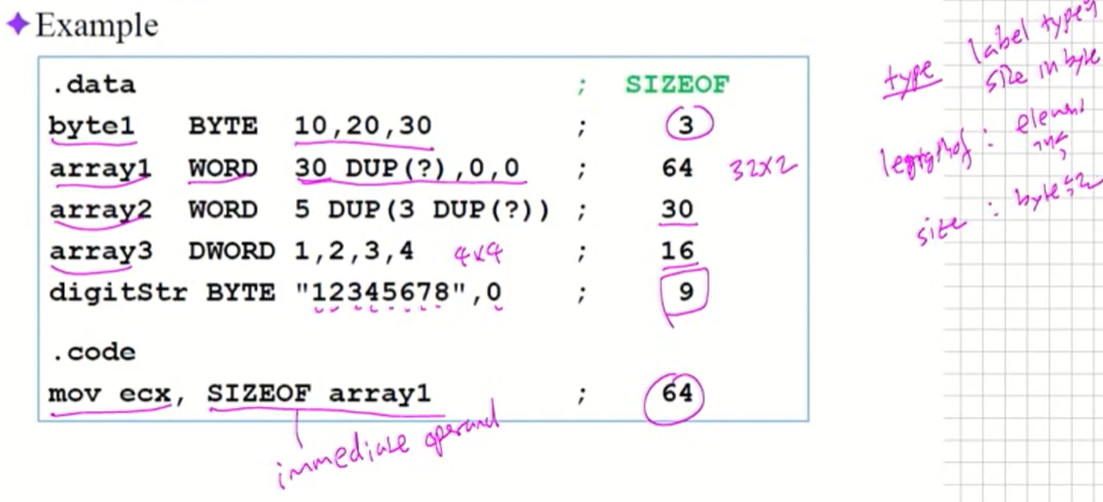
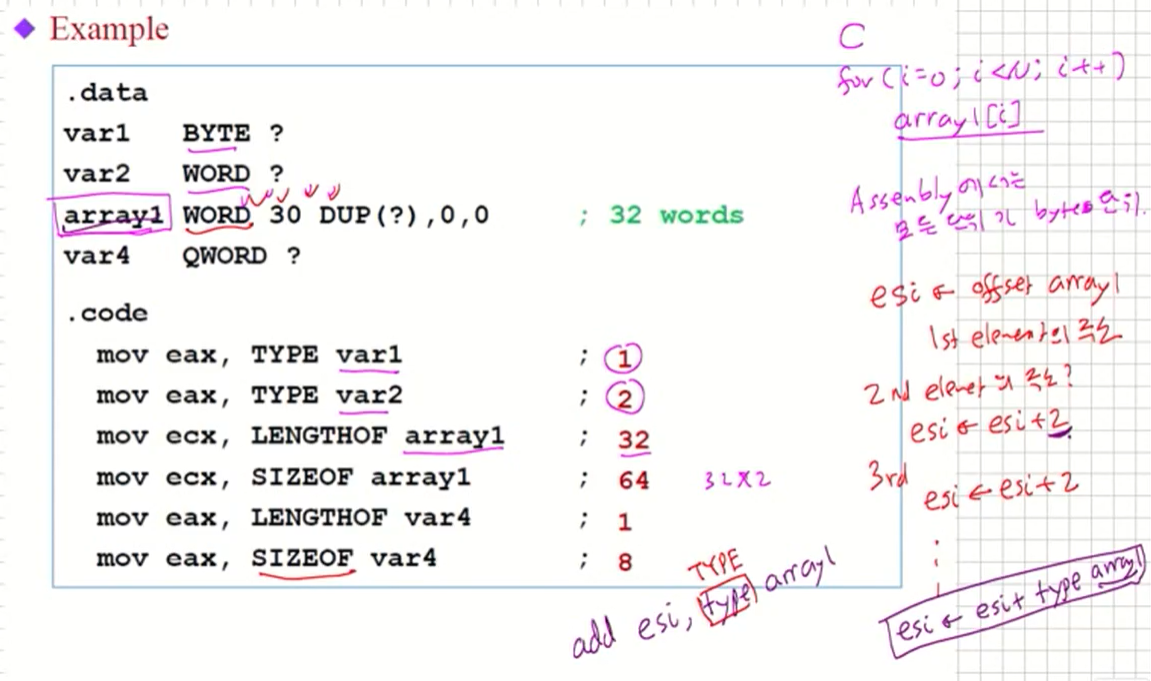
이때 Assembly에서는 모든 단위가 byte 단위이다.
이때 소소한 꿀팁인데 만약에 array1의 위치를 까먹었다면 다음과 같이 하면 된다.
esi <- esi + TYPE array1
add esi, TYPE array1
Spanning Multiple Lines
- A data declaration spans multiple lines if each line (except the last) ends with a comma. : 라인을 여러줄 사용해서 정의하기
- The LENGTHOF and SIZEOF operators include all lines belonging to the declaration.
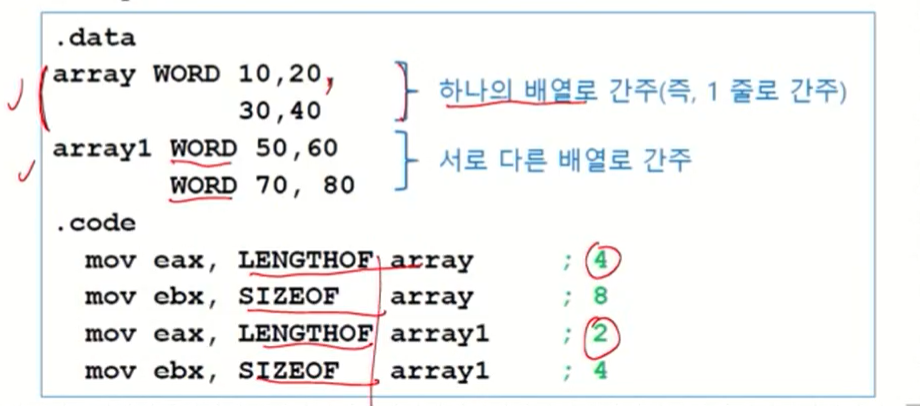
LABEL Directive
- Assigns an alternate label name and type to an existing storage location : 메모리 특정위치에 특정 type의 label을 두어서 원하는 만큼 데이터를 읽어들일 수 있다.
- LABEL does not allocate any storage of its own
- Removes the need for the PTR operator : PTR의 필요성이 줄었다.
- Example
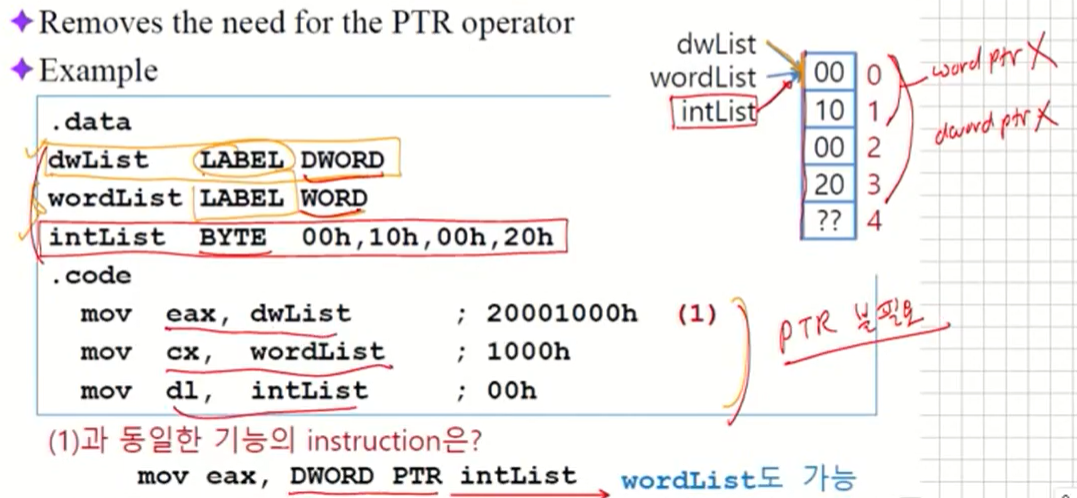
2. Indirect Addressing
memory operands는 direct, direct offset, indirect, indexed 총 4가지가 존재한다.
Indirect Operands (ESI 사용방법)
- An indirect operand holds the address of a variable, usually an array or string. It can be dereferenced (just like a pointer).
- Example
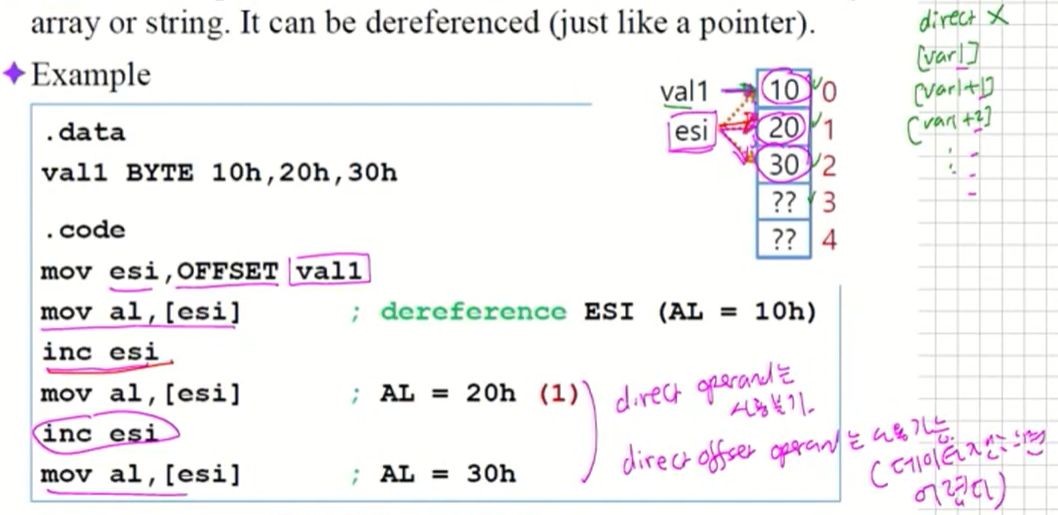
(1) 20h, 30h를 가지고 올때 Direct operand를 사용할 수 있을까? No.
Direct offset operand는 사용 가능( mov al,[val1 + 1] ) 하지만 이것도 데이터가 많으면 어렵다.
만약에 al의 size가 8bit이기 때문에 [esi]의 size는 8bit으로 간주한다.
- Use PTR when the size of a memory operand is ambiguous.
- Example
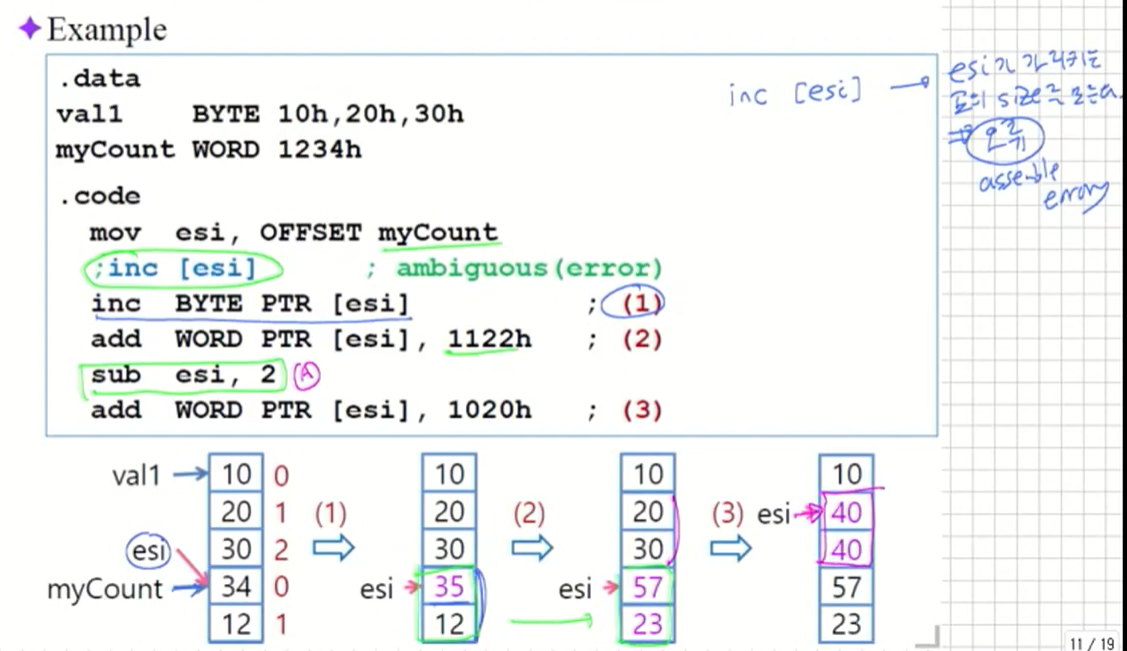
위와 같이 inc [esi]로 하면 esi가 가리키는 곳의 size를 모르기 때문에 에러가 발생한다. 그래서 PTR을 이용해서 esi 가르키는 곳이 얼마인지를 정해주어야 한다.
Indirect Operand가 필요한 경우
-
아래 보인 자료를 모두 더하여 eax에 저장하는 프로그램을 작성하자.
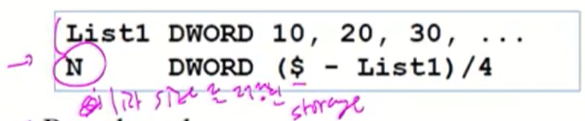
현재 N은 list1의 size를 가지고 있다.
-
Pseudocode
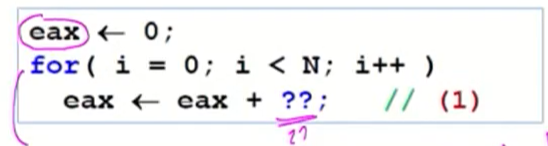
-
(1)을 direct 또는 direct offset operand로 구현 가능할 까?
- Loop의 반복 실행에 따른 주소 변경을 할 수 없다 -> 답은 No.
-
Indirect Operand를 사용하면 구현할 수 있다.
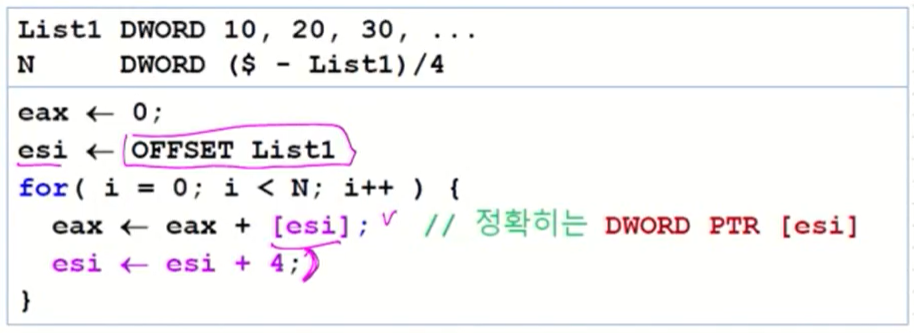
Array Sum Example
- Indirect operands are ideal for traversing an array.
- Note that the register in brackets must be incremented by a value that matches the array type.
- Example
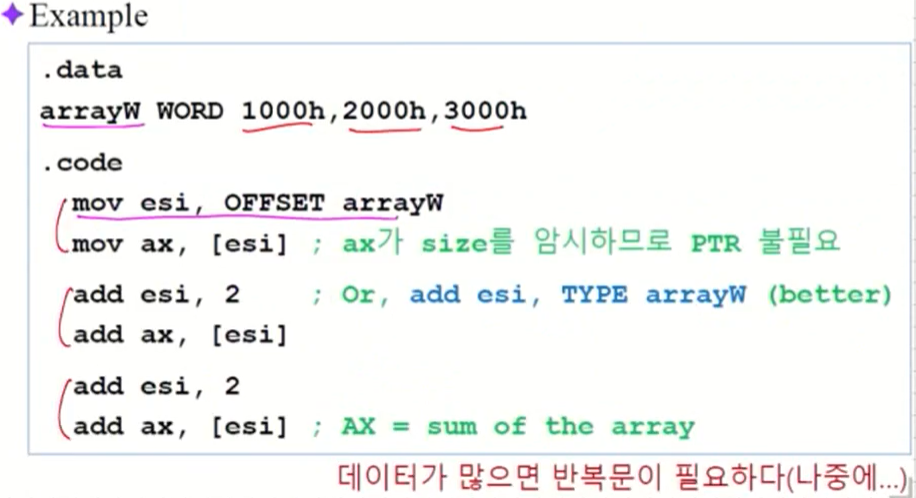
Indexed Operands
arrayW[esi] == [arrayW + esi] 형태로 사용할 수 있다. 이때 arrayW가 WORD이기 때문에 한번에 2byte씩 이동하여 그 다음 인자로 이동하였다.
- arrayW : base address
- esi : index (C와는 달리 0 2, … 등으로 data size의 배수 씩 증가해야 한다).
Pointers
- You can declare a pointer variable that contains the offset of another variable. : C언어의 포인터와 비슷하게 주소를 따로 저장할수도 있다.
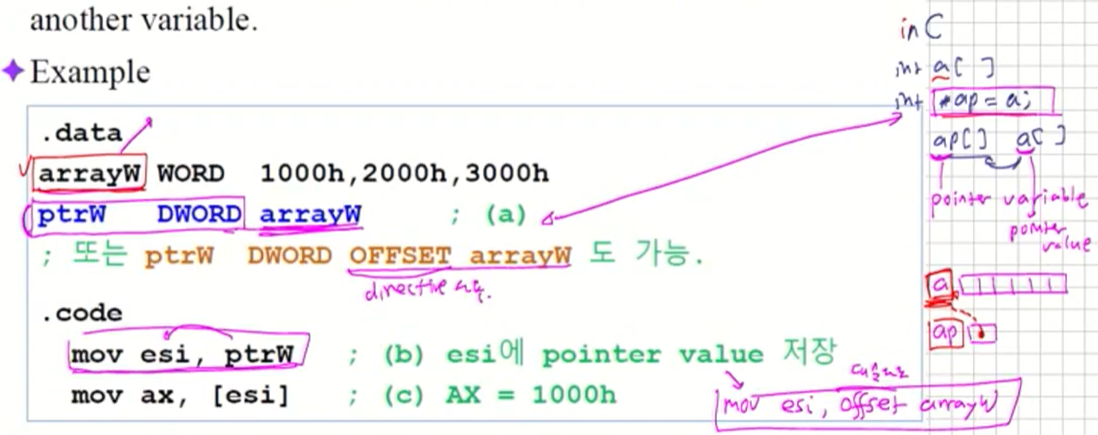
이게 주소값이라는 것을 알려주기 위해서 ptrW DWORD OFFSET arrayW
로도 작성가능하다.
(a) pointer(즉, address)이므로 IA-32에서는 DWORD로 설정해야 한다.
(b) ptrW는 direct operand이다.
(c) [esi]는 indirect opreand이다.
Memory Operand에 관한 추가 설명(1)
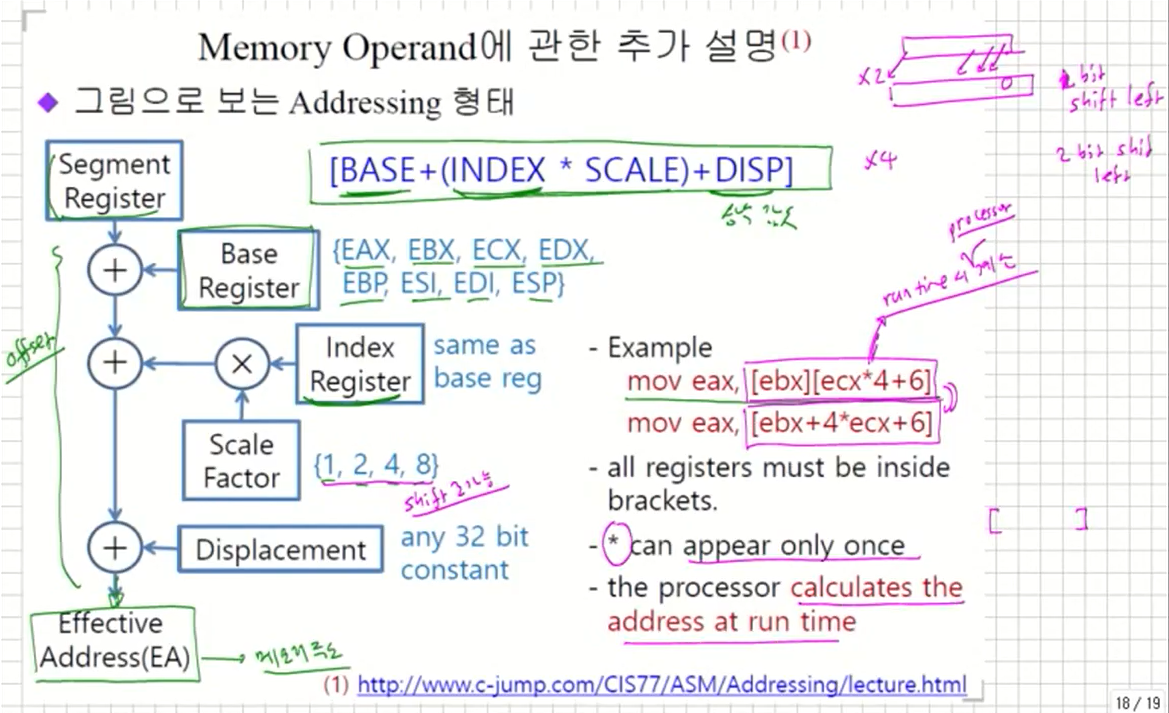
Memory Operand가 규칙이 있다.
EA가 실제 메모리 주소이다.
INDEX Register에 SCALE을 곱하고 DISP까지해서 BASE Address에 더할 수 있다.
run time때 processor가 알아서 값을 게산한다.
CSE3030 어셈블리 프로그래밍 18
그림으로 보는 Addressing 형태
(1) http://www.c-jump.com/CIS77/ASM/Addressing/lecture.html
- Example
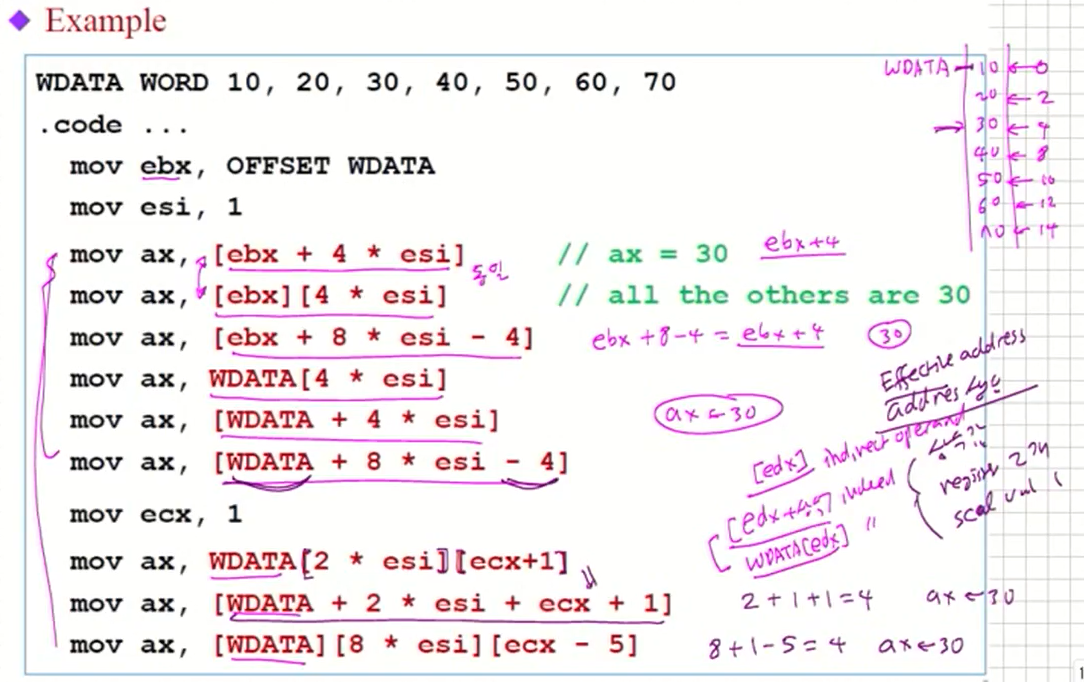
3. JMP and LOOP Instructions
- JMP Instruction
- LOOP Instruction
- LOOP Example
- Summing an Integer Array
- Copying a String
JMP Instruction
- JMP is an unconditional jump to a label that is usually within the same procedure.
- Syntax: JMP target : target에는 label을 사용한다.
- Logic: EIP <- target : EIP 내용을 바꾼다.
- EIP에는 다음 실행할 instruction의 주소가 있다.
- 이 주소를 target으로 바꾸어, target 에서 다음 instruction을 가져온다.
- Example:
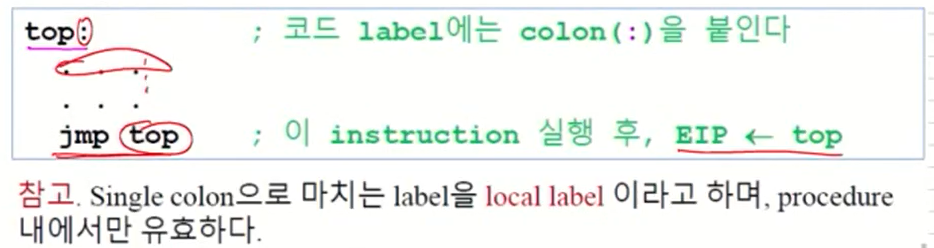
내에서만 유효하다.
:
: local label - 함수내에서만 사용
::
: global label - 모두 볼 수 있다.
-Jmp instruction으로 프로그램 내 어디로든 실행 위치를 바꿀 수 있다.
- 현재 위치에서 전, 후 모두 가능.
- target은 32bit offset 값이다.
- 현재 실행 중인 procedure 밖으로도 jmp 할 수 있다.
- 이를 위해서는 global label이 필요하다.
- Global label은 label에 double colon(::)을 붙인다.
LOOP Instruction
-
The LOOP instruction creates a counting loop
-
Syntax: LOOP target ; target은 8bit signed relative(eip + target)
-
Logic:
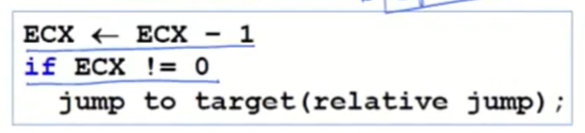
-
Implementation
- The assembler calculates the distance, in bytes, between the offset of the following instruction(2) and the offset of the target.
- The distance is called the relative offset (8 bit signed number).
-
The relative offset is added to EIP
-
즉, 현재 EIP 값에서 -128 ~ +127 사이의 위치로 jump한다.
(1) 어셈블러가 assemble 할 때 계산한다.
(2) 현재 EIP가 갖고 있는 offset(즉, loop 다음 instruction의 offset).
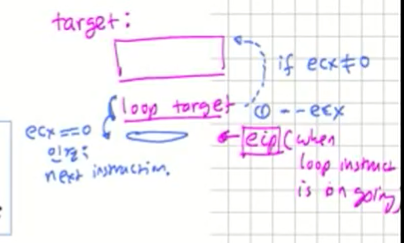
위와 같은 형태로 함수가 진행된다.
-
LOOP Example
-
The following loop calculates 5 + 4 + 3 +2 + 1 :
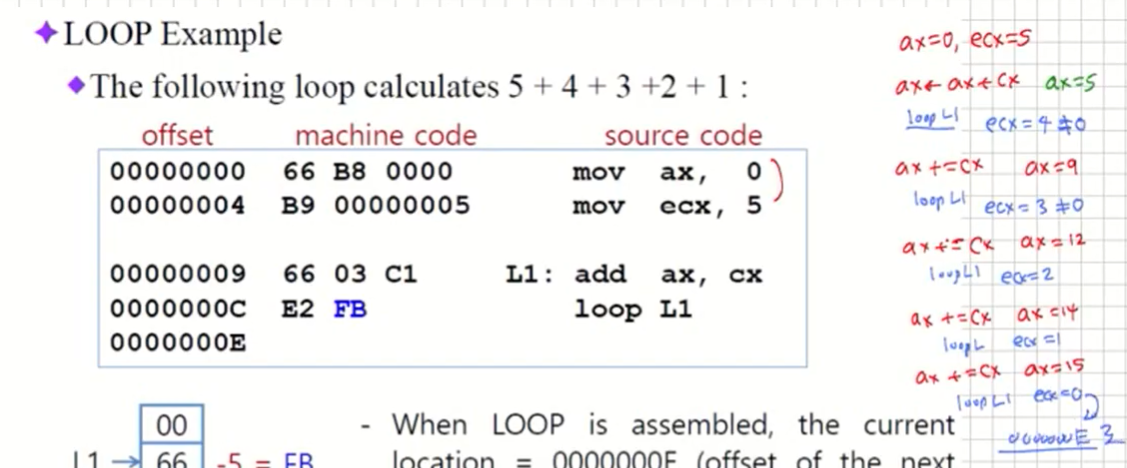
코드의 FB가 relative address이다. 그러면 E+(-5) 로 이동하게 된다.
이때 ecx는 일단 감소하고 체크된다.
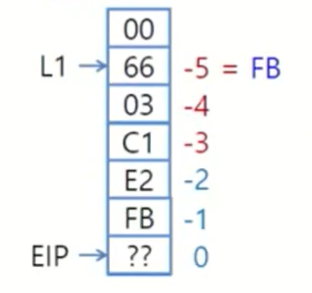
-
When LOOP is assembled, the current location = 0000000E (offset of the next instruction).
-
–5 (FBh) is added to the current location, causing a jump to location 00000009:
00000009 <- 0000000E + FB
-
참고 사항
- The relative offset is encoded in a single signed byte : relative offset은 signed byte 하나가지고 사용한다.
- what is the largest possible backward jump? -128
- what is the largest possible forward jump? +128
- What will be the final value of AX? 10
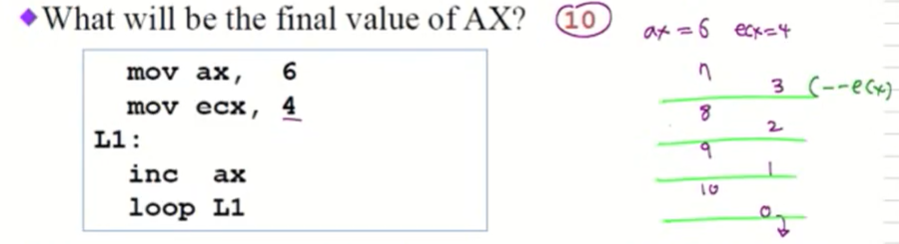
- How many times will the loop execute? 4294967296
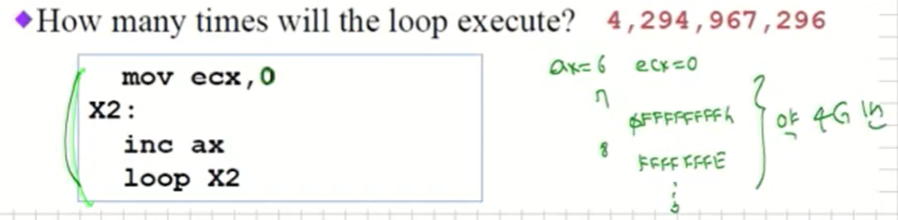
ecx에 0 넣는건 가급적 하지 말아야 한다.
-
Loop 내의 코드 블록 크기가 relative jump의 범위를 벗어날 경우, assemble 할 때 오류로 보고될 것이다.
-
이 경우 해결 방법은 아래 보인 것처럼 jmp instruction을 사용하여 프로그램을 수정할 수 있다.
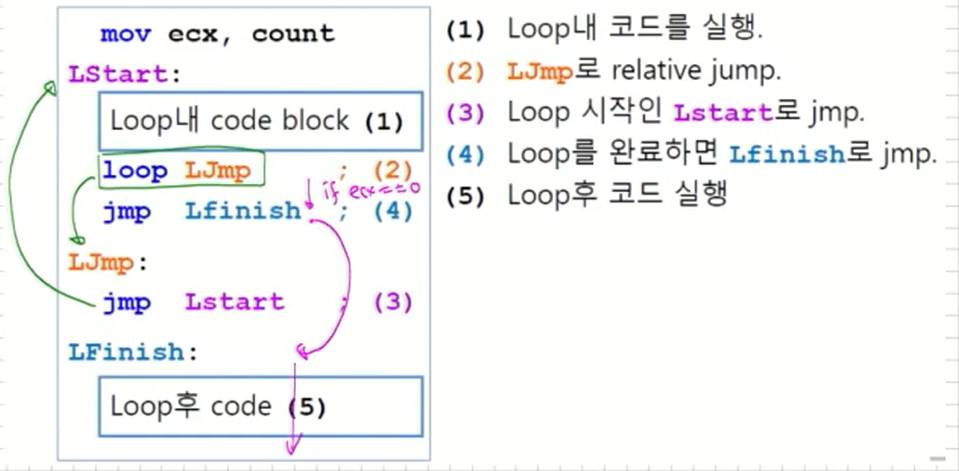
-
Example
-
다음에 보인 가상 코드에서 각 코드 블록의 실행 순서를 알아보자.
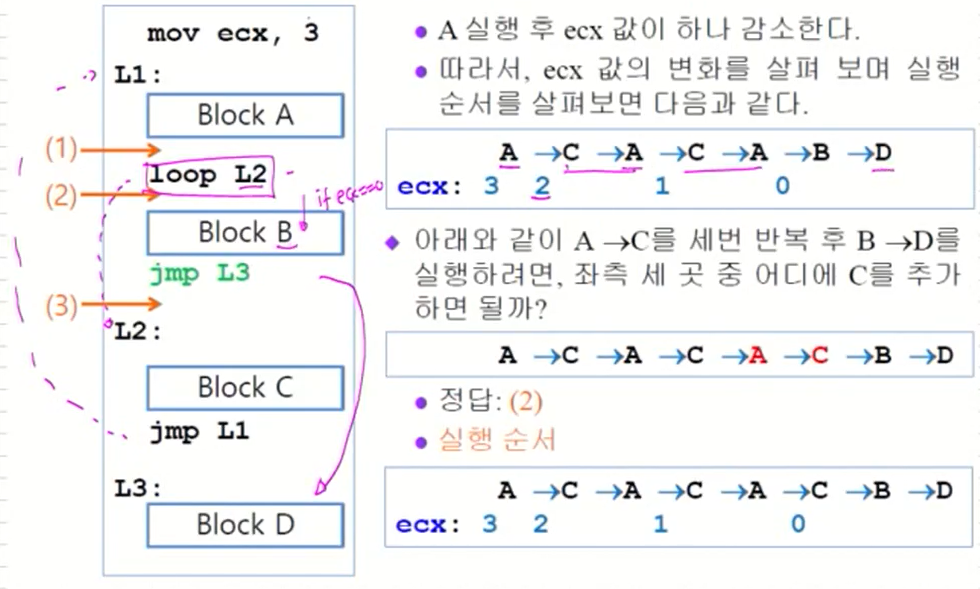
하지만 위의 코드는 A->C를 3번 반복하고자 한다면 마지막 A 뒤에 C를 실행시키어서 끝나게 하면 된다.
-
Example (계속)
-
아래 보인 가상 코드에서 각 코드 블록의 실행 순서는?
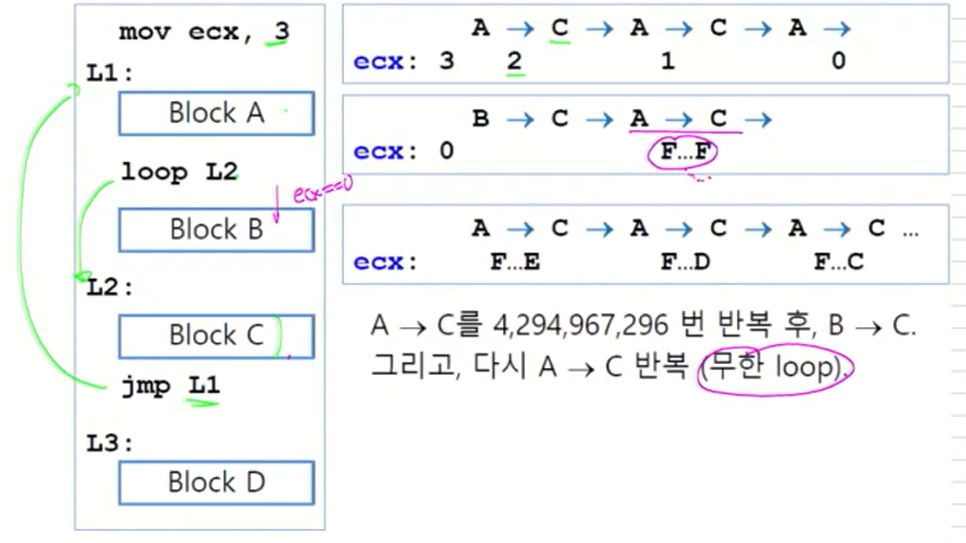
위와 같은 경우는 무한 loop에 걸릴수도 있다. 그렇기 때문에 ecx를 잘 생각해서 설정해야 한다.
Nested Loop
- In order to code a loop within a loop, the outer loop counter’s ECX value must be saved(1) : loop가 두개인 경우 ecx를 미리 따로 저장해놓을 필요가 있다.
- In the following example, the outer loop executes 100 times, and the inner loop 20 times.
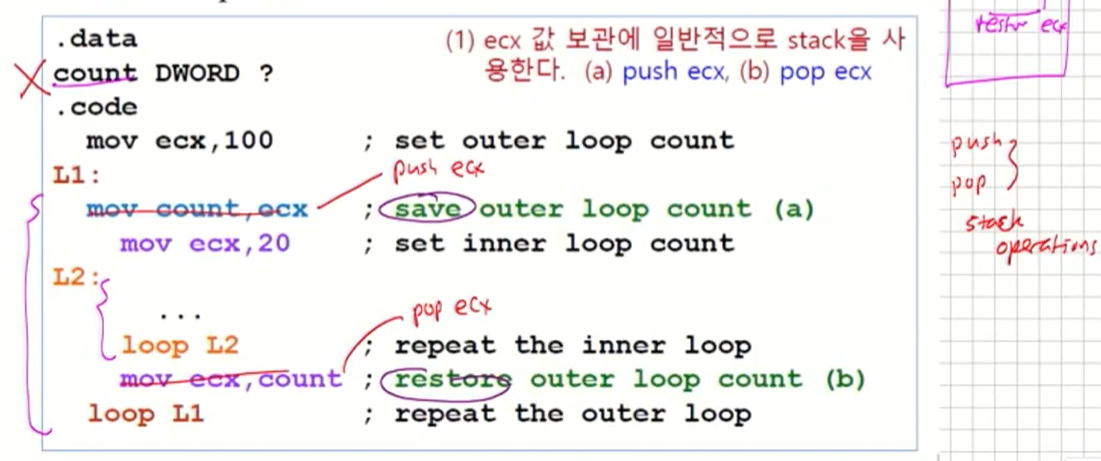
Summing an Integer Array
-
The following code calculates the sum of an array of 16-bit integers.
(참고. OFFSET, PTR, TYPE, LENGTHOF, SIZEOF 등은 operator이다. 반면 LAVEL은 directive이다.)
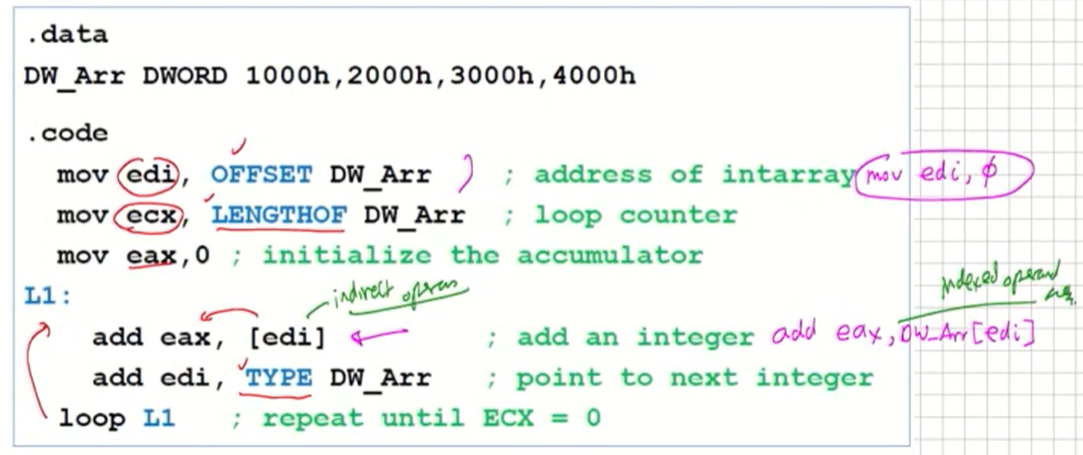
-
앞에 보인 배열 덧셈 문제를 indirect operand가 아닌 indexed operand를 사용하여 프로그램을 작성하시오.
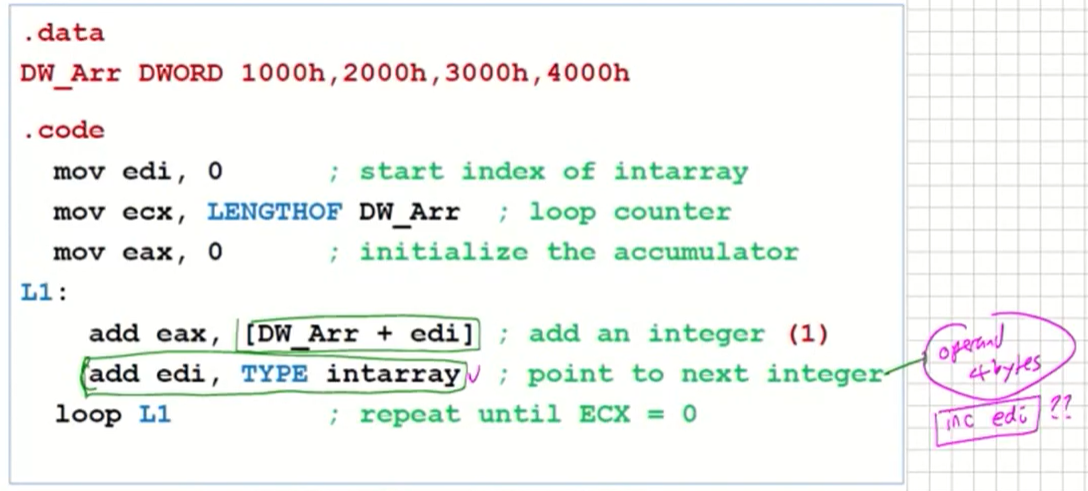
(1) [DW_Arr + edi] 대신 DW_Arr[edi]를 사용해도 된다.
하지만 위엫서 전에 안보이던 TYPE intarray가 나온것을 확인할 수 있다. 문제는 이게 4byte이다. 그렇다보니 기존과는 조금 다른 방식으로 코딩이 되었다고 보이기도 한다. 이를 해결하기 위해 scale Factor을 사용한다.
64-Bit Programming
- 지금까지 32 bit 프로그래밍을 위하여 기본적으로 알아야할 사항을 설명하였다.
- 여기서는 64 bit 프로그래밍을 위하여 추가로 알아야할 사항을 기술한다.
참고로 여기에서부터 레지스터 이름 앞에 r이 붙은 놈들이 나오는데 e로 시작하는 것의 2배 크기라고 생각하면 된다.
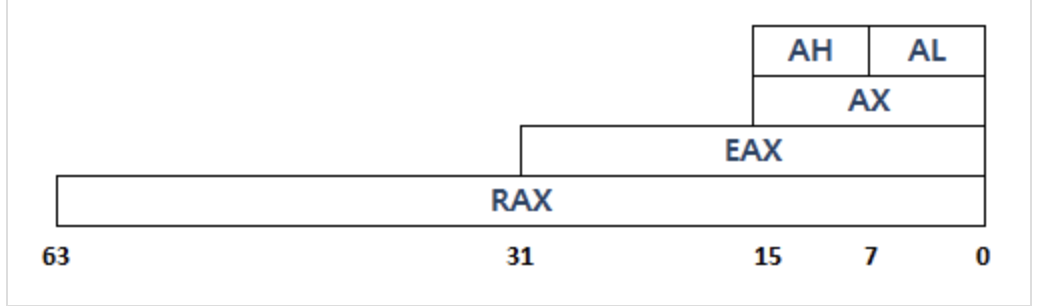
MOV Instruction
-
64-bit register에 32, 16, or 8 bit 상수 값을 쓰면, upper 32, 48, 56 bits는 0으로 clear 된다 (당연?).

-
32-bit register에 값을 쓰면, 이 register에 대응하는 64 bit
register의 upper 32 bit는 clear 된다.

-
그러나 16, 8 bit register에 쓰는 것은 영향을 주지 않는다
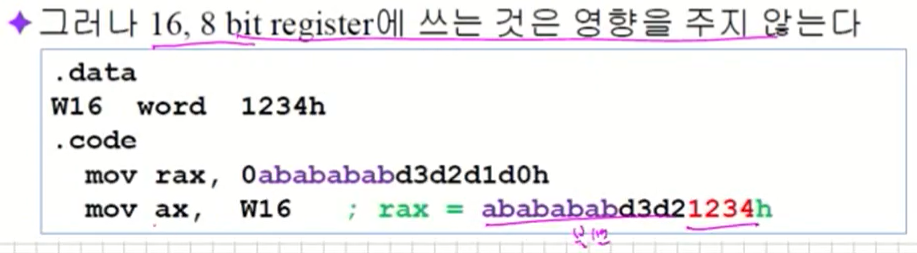
위와 같이 같은 accumulte register이지만 rax값은 바뀌지 않았다.
-
MOVSXD instruction (move with sign-extension)
- Permits the source operand to be a 32-bit register or memory operand. : 64bit에 32bit를 삽입하고 싶을때
- MOVZXD는?
- Instruction이 없다(사실 불필요).
- 64 bit register <- 32 bit operand (upper 32 bit는 자동으로 0)
- 8, 16 bit operand를 64 bit register에 저장하는 것은 movsx, movzx를 사용한다. (비트에 따라 쓰는 함수가 다르다)
-
Example
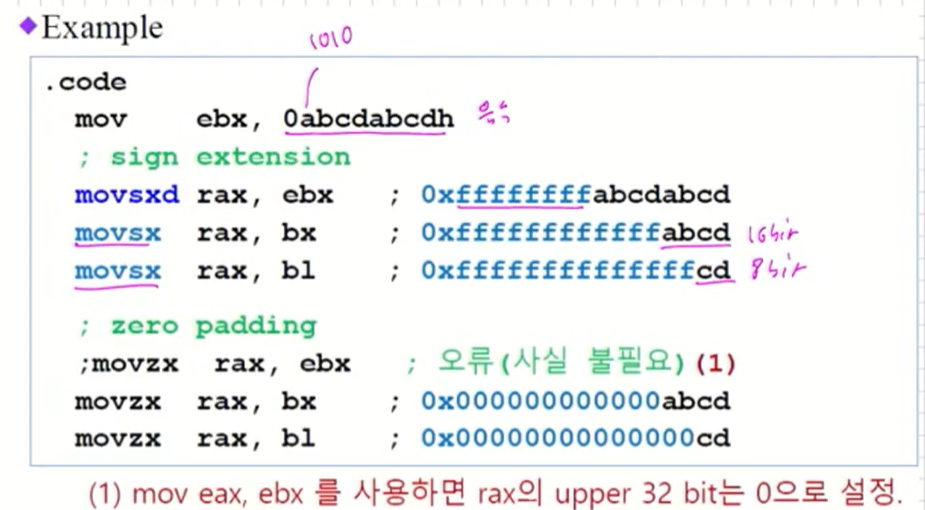
-
OFFSET operator
- Generates a 64-bit address.
- RSI and RDI are the most common 64-bit index registers for accessing arrays. : RSI, RDI는 index register이다. 주로 64bit에서 사용하는 레지스터이다.
-
LOOP uses the RCX register as the loop counter. : LOOP는 RCX register을 사용한다.(jump 범위는?)
-
When a partial register operands are used in ADD, SUB, INC, DEC, the remainder of the register is not modified.
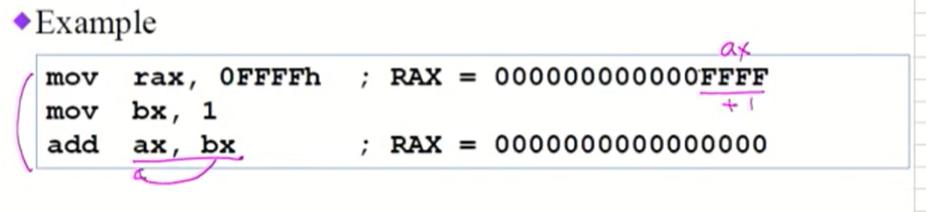
-
Indirect operand를 사용할 경우 그의 data 크기가 애매할 경우 PTR opeartor를 사용한다(32 bit와 동일)

-
64 bit data array를 사용할 경우, 주소는 8씩 증가 또는 감소
해야한다(1)
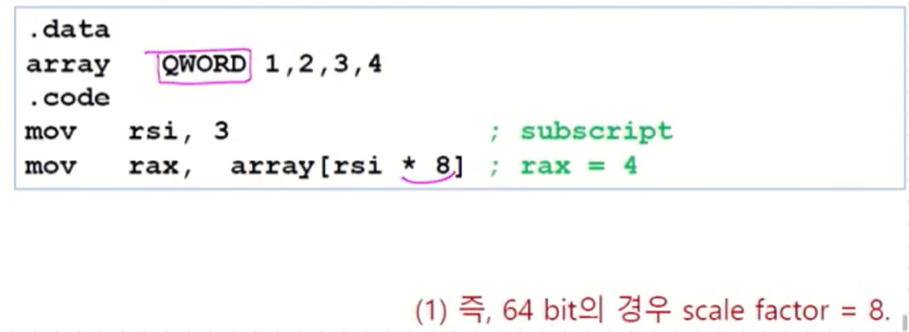
-
Example: 64 bit Data Array Addition
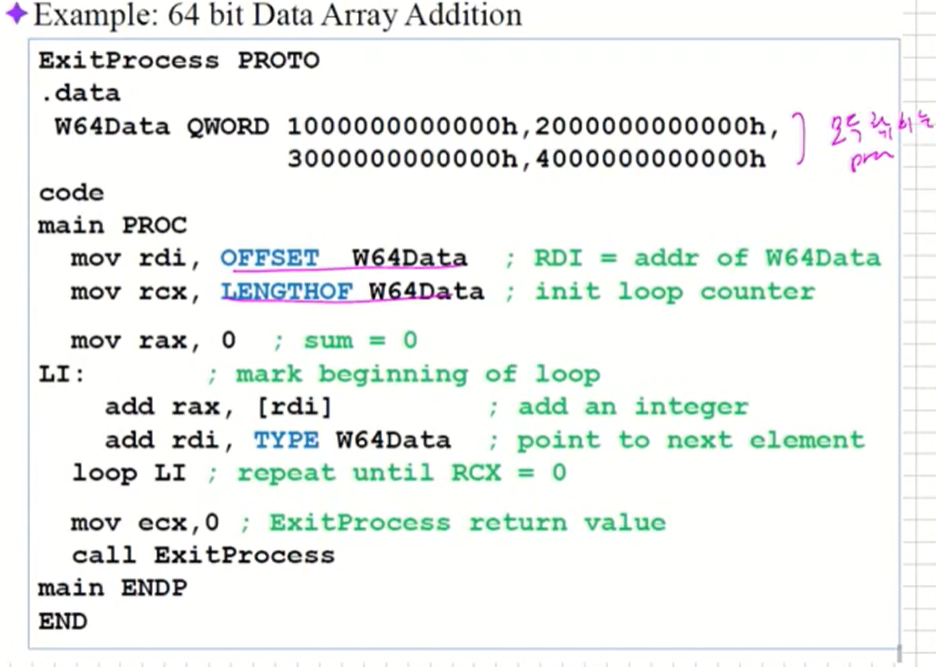