1) html 코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Lotto</title>
<link href="./style.css" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="lotto">
<h3>로또 번호 추첨</h3>
<div class="numbers"></div>
<button id="draw">추첨</button>
<button id="reset">다시</button>
</div>
</div>
<script src="./script.js"></script>
</body>
</html>
2) css 코드
*{
box-sizing: border-box
}
html{
font-size: 32px;
}
.container{
width: 350px;
height: 100vh;
margin: 0 auto;
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
}
.lotto{
text-align: center;
}
.numbers{
display: flex;
justify-content: space-around;
align-items: center;
border: 1px solid black;
border-radius: 10px;
width: 350px;
height: 60px;
}
.eachnum{
font-size: 0.75em;
width: 50px;
height: 50px;
border-radius: 25px;
display: flex;
justify-content: center;
align-items: center;
color: white;
background-color: red;
}
button{
font-size: 0.5em;
width: 100px;
height: 40px;
border: none;
border-radius: 6px;
color: white;
background-color: salmon;
cursor: pointer;
}
button:active{
font-size: 0.6em;
width: 105px;
height: 42px;
}
3) js 코드
const numbersDiv = document.querySelector(".numbers")
const drawButton = document.querySelector("#draw")
const resetButton = document.querySelector("#reset")
const lottoNumbers = []
const colors = ["tomato", "teal", "orange", "purple", "blue"]
function paintNumber(number){
const eachNumDiv = document.createElement("div")
let colorIndex = Math.floor(number / 10)
eachNumDiv.classList.add('eachnum')
eachNumDiv.style.backgroundColor = colors[colorIndex]
eachNumDiv.textContent = number
numbersDiv.appendChild(eachNumDiv)
}
drawButton.addEventListener('click', function(){
while(lottoNumbers.length < 6){
let ran = Math.floor(Math.random() * 45) + 1
if(lottoNumbers.indexOf(ran) === -1){
lottoNumbers.push(ran)
paintNumber(ran)
}
}
})
resetButton.addEventListener('click', function(){
lottoNumbers.splice(0,6)
numbersDiv.innerHTML = ""
})
4) 결과
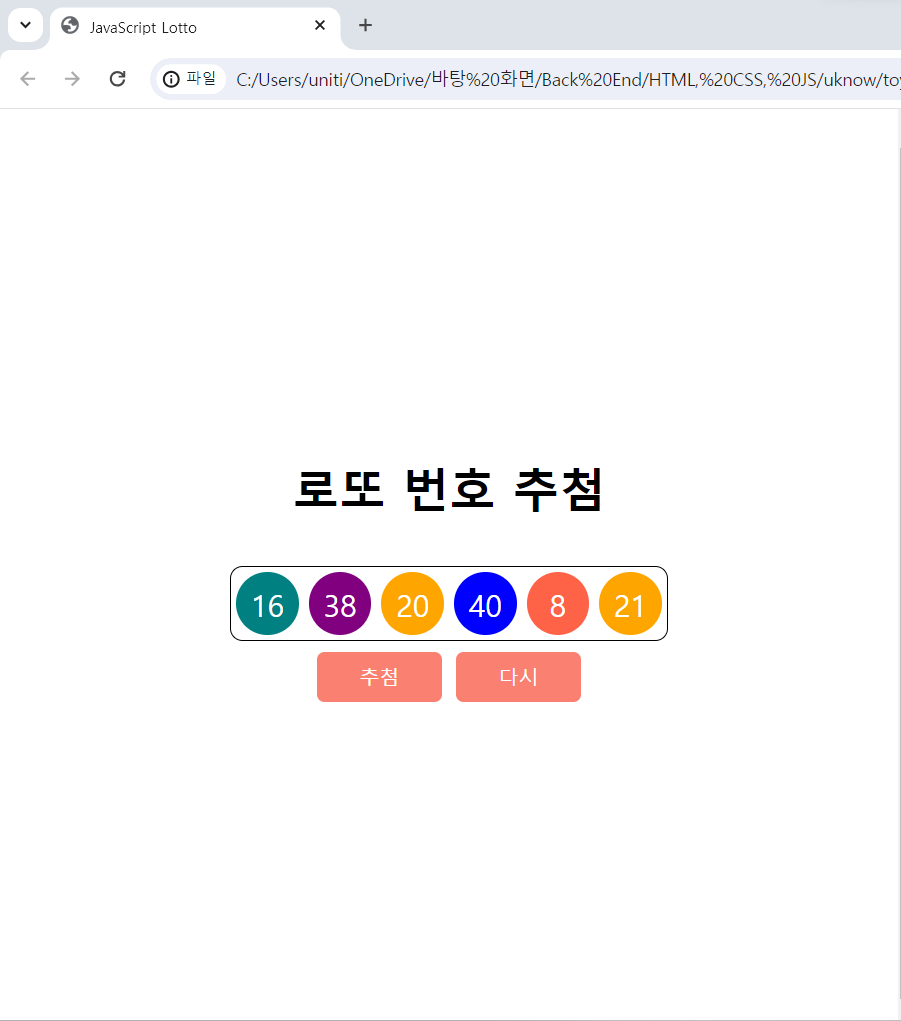