@extend
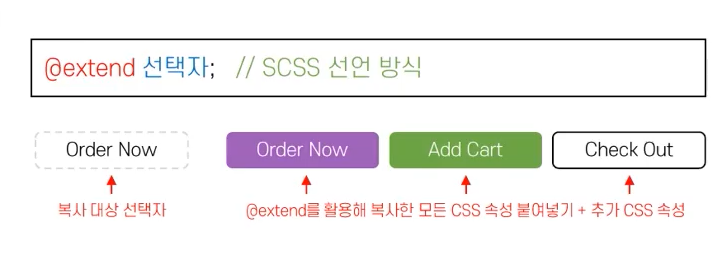
👊 예제
<button class="btn">Initial Button</button>
<div class="buttons">
<button class="order">order</button>
<button class="add">add</button>
<button class="checkout">checkout</button>
</div>
.btn {
width: 200px;
padding: 7px;
background-color: #fff;
font-size: 18px;
text-transform: capitalize;
border-radius: 5px;
border-color: transparent;
outline: none;
cursor: pointer;
color: #fff;
}
- 버튼에 대한 공통적인 속성을 적용했을 때 다른 버튼에도 이 속성을 적용하고 싶다면 @extend를 사용한다.
.order {
@extend .btn;
background-color: purple;
}
.add {
@extend .btn;
background-color: yellowgreen;
}
.checkout {
@extend .btn;
color: #000;
border: 2px solid #000;
}
<div class="buttons">
<button class="btn order">order</button>
<button class="btn add">add</button>
<button class="btn checkout">checkout</button>
</div>
- css에서는 이렇게 했었음
class="btn order"
🙆 RESULT
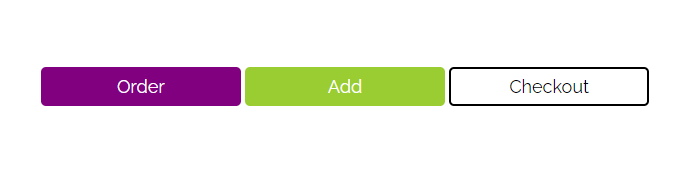
placeholder 선택자 %
👊 예제
<div class="card">
<div class="card-item">Card Item #01</div>
<div class="card-item">Card Item #02</div>
<div class="card-item">Card Item #03</div>
</div>
.shape {
width: 250px;
height: 300px;
border-radius: 5px;
box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
}
.card {
display: flex;
gap: 30px;
&-item {
@extend .shape;
display: flex;
justify-content: center;
align-items: center;
}
}
- 위 html, scss 파일이 있다고 가정했을 때 .card-item은 .shpae를 extend하여 속성을 가져올 수 있다.
- scss의 placeholder 속성 %를 이용해서 다른방식으로 가져올 수 있다.
%shape {
width: 250px;
height: 300px;
border-radius: 5px;
box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
}
.card {
display: flex;
gap: 30px;
&-item {
@extend %shape;
display: flex;
justify-content: center;
align-items: center;
}
}
- 그러나 위, 아래 두방식의 차이점은 컴파일된 css파일을 보면 알 수 있다.
% 사용하지않는 경우
.shape, .card-item {
width: 250px;
height: 300px;
border-radius: 5px;
-webkit-box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
}
% 사용한 경우
.card-item {
width: 250px;
height: 300px;
border-radius: 5px;
-webkit-box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
}
- 컴파일된 css파일을 확인해보면 .shape가 포함되지않는다.
- 깔끔한 코드를 원할때에는 placeholder속성 %를 사용한다.
- 투명선택자라고 할 수 있다.
🙆 RESULT
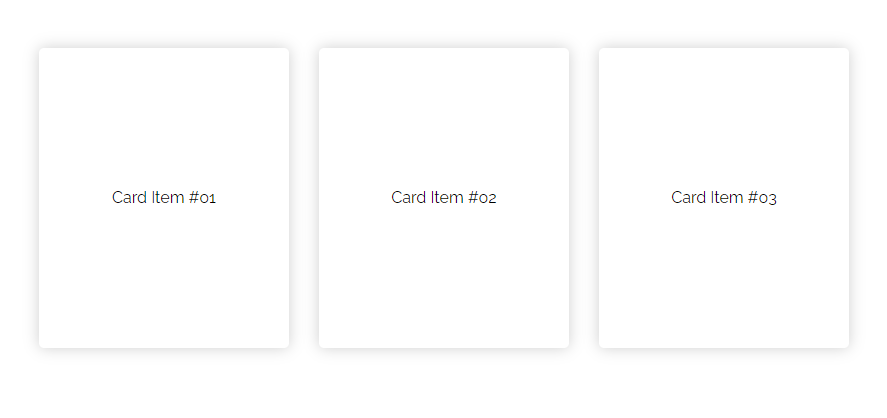