코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Hold Shift to Check Multiple Checkboxes</title>
<link rel="icon" href="https://fav.farm/🔥" />
</head>
<body>
<style>
html {
font-family: sans-serif;
background: #ffc600;
}
.inbox {
max-width: 400px;
margin: 50px auto;
background: white;
border-radius: 5px;
box-shadow: 10px 10px 0 rgba(0, 0, 0, 0.1);
}
.item {
display: flex;
align-items: center;
border-bottom: 1px solid #f1f1f1;
}
.item:last-child {
border-bottom: 0;
}
input:checked + p {
background: #f9f9f9;
text-decoration: line-through;
}
input[type="checkbox"] {
margin: 20px;
}
p {
margin: 0;
padding: 20px;
transition: background 0.2s;
flex: 1;
font-family: "helvetica neue";
font-size: 20px;
font-weight: 200;
border-left: 1px solid #d1e2ff;
}
</style>
<div class="inbox">
<div class="item">
<input type="checkbox" />
<p>This is an inbox layout.</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Check one item</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Hold down your Shift key</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Check a lower item</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Everything in between should also be set to checked</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Try to do it without any libraries</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Just regular JavaScript</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Good Luck!</p>
</div>
<div class="item">
<input type="checkbox" />
<p>Don't forget to tweet your result!</p>
</div>
</div>
<script>
const checkboxes = document.querySelectorAll('input[type="checkbox"]');
let lastChecked;
function handleCheck(e) {
let inBetween = false;
if (e.shiftKey && this.checked) {
checkboxes.forEach((checkbox) => {
if (checkbox === this || checkbox === lastChecked) {
inBetween = !inBetween;
}
if (inBetween) {
checkbox.checked = true;
}
});
}
lastChecked = this;
}
checkboxes.forEach((checkbox) =>
checkbox.addEventListener("click", handleCheck)
);
</script>
</body>
</html>
결과
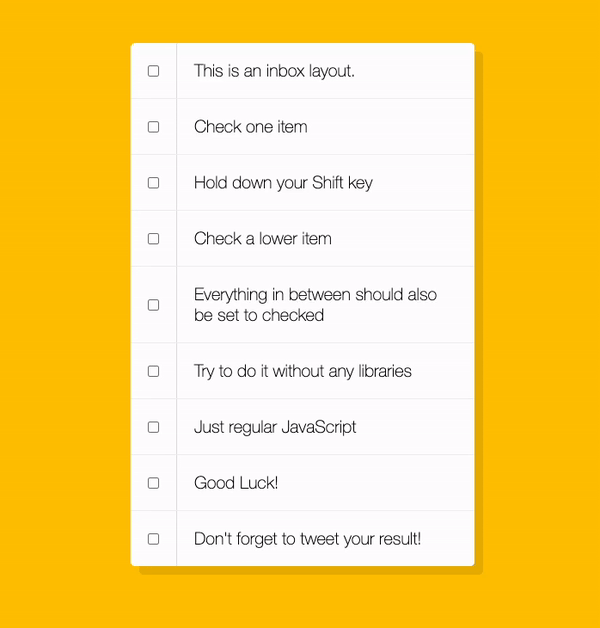