Line Trace
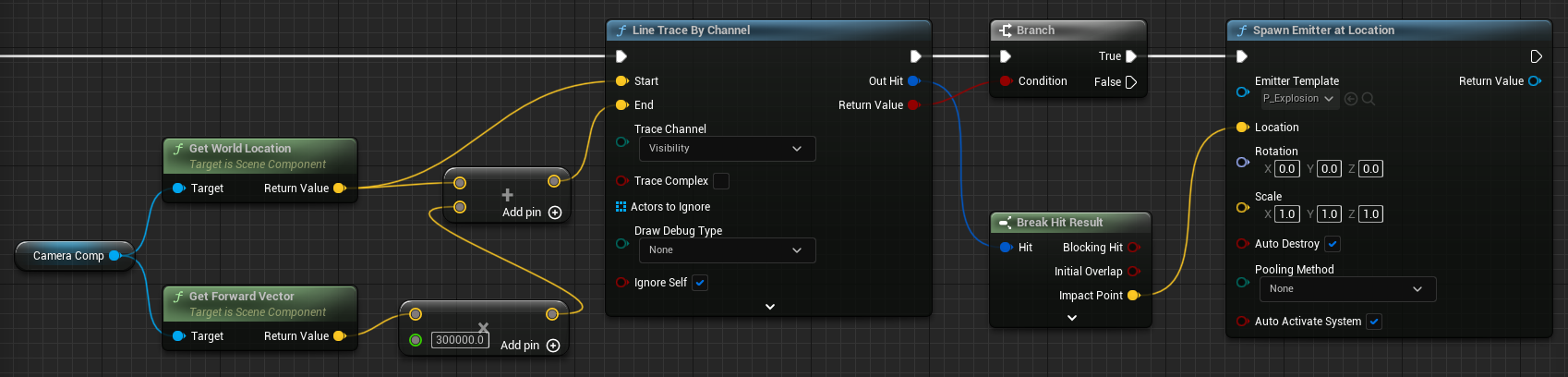
📄 C++
✏️ TPSPlayer.h
UPROPERTY(EditAnywhere)
class UParticleSystem* bulletImpactFactory;
void LineShot();
✏️ TPSPlayer.cpp
void ATPSPlayer::LineShot()
{
FHitResult hitInfo;
FVector start = cameraComp->GetComponentLocation();
FVector end = start + cameraComp->GetForwardVector() * 300000;
FCollisionQueryParams params;
params.AddIgnoredActor(this);
if (GetWorld()->LineTraceSingleByChannel(hitInfo, start, end, ECollisionChannel::ECC_Visibility, params))
{
UGameplayStatics::SpawnEmitterAtLocation(GetWorld(), bulletImpactFactory, hitInfo.ImpactPoint);
}
}
📄 Blueprint
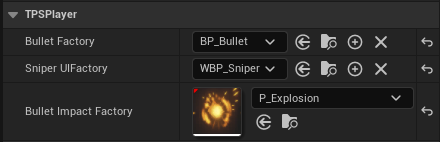
Add Force
📄 C++
✏️ TPSPlayer.cpp
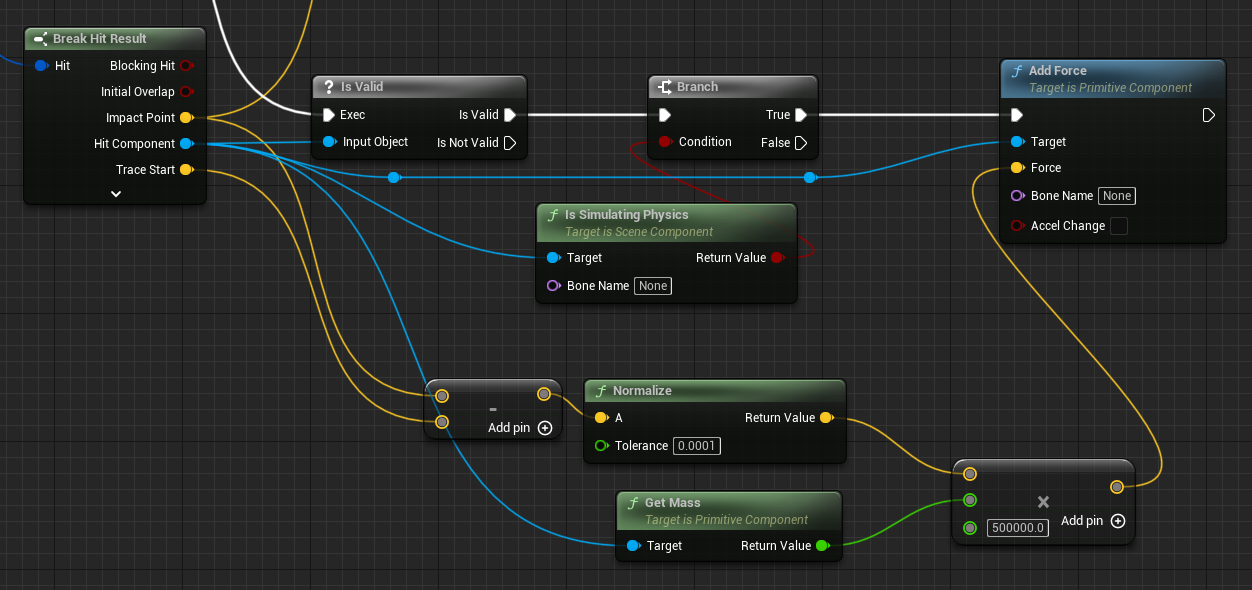
void ATPSPlayer::LineShot()
{
FHitResult hitInfo;
FVector start = cameraComp->GetComponentLocation();
FVector end = start + cameraComp->GetForwardVector() * 300000;
FCollisionQueryParams params;
params.AddIgnoredActor(this);
if (GetWorld()->LineTraceSingleByChannel(hitInfo, start, end, ECollisionChannel::ECC_Visibility, params))
{
UGameplayStatics::SpawnEmitterAtLocation(GetWorld(), bulletImpactFactory, hitInfo.ImpactPoint);
}
auto hitComp = hitInfo.GetComponent();
if (hitComp && hitComp->IsSimulatingPhysics())
{
FVector dir = (hitInfo.ImpactPoint - start).GetSafeNormal();
FVector force = dir * hitComp->GetMass() * 500000;
hitComp->AddForce(force);
}
}