배열을 이용하여 맵 만들기
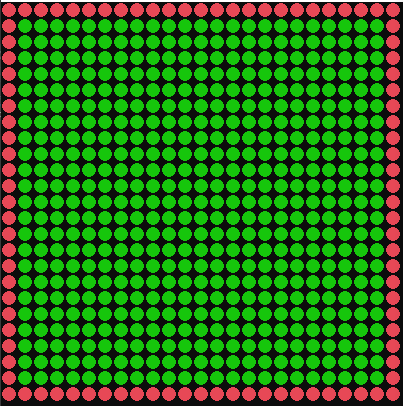
- 벽은 빨간 원으로 비어있는 공간은 초록 원으로 만들기
- 2차원 배열 사용
- 색 추출, 랜더 등의 기능은 함수로 사용
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace Algorithm
{
class Board
{
public TileType[,] _tile;
public int _size;
const char CIRCLE = '\u25cf';
public enum TileType
{
Empty,
Wall
}
public void Initialize(int size)
{
_tile = new TileType[size, size];
_size = size;
for (int y = 0; y < _size; y++)
{
for (int x = 0; x < _size; x++)
{
if (x == 0 || x == _size - 1 || y == 0 || y == _size - 1)
_tile[y, x] = TileType.Wall;
else
_tile[y, x] = TileType.Empty;
}
}
}
public void Render()
{
ConsoleColor prevColor = Console.ForegroundColor;
for (int y = 0; y < _size; y++)
{
for (int x = 0; x < _size; x++)
{
Console.ForegroundColor= GetTileColor(_tile[y, x]);
Console.Write(CIRCLE);
}
Console.WriteLine();
}
Console.ForegroundColor = prevColor;
}
ConsoleColor GetTileColor(TileType type)
{
switch (type)
{
case TileType.Empty:
return ConsoleColor.Green;
case TileType.Wall:
return ConsoleColor.Red;
default:
return ConsoleColor.Green;
}
}
}
}