DTO, Util
DTO: User.java
/* import부분 생략 */
public class User {
private int no;
private String id;
private String password;
private String name;
private String tel;
private String email;
private Date createdDate;
public User() {}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Date getCreatedDate() {
return createdDate;
}
public void setCreatedDate(Date createdDate) {
this.createdDate = createdDate;
}
}
ConnectionUtil
/* import부분 생략 */
public class ConnectionUtil {
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String id = "hr";
String pw = "zxcv1234";
static {
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
} catch (ClassNotFoundException ex) {
ex.getStackTrace();
}
}
public static Connection getConnection() throws SQLException{
return DriverManager.getConnection(url, id, pw);
}
}
회원가입
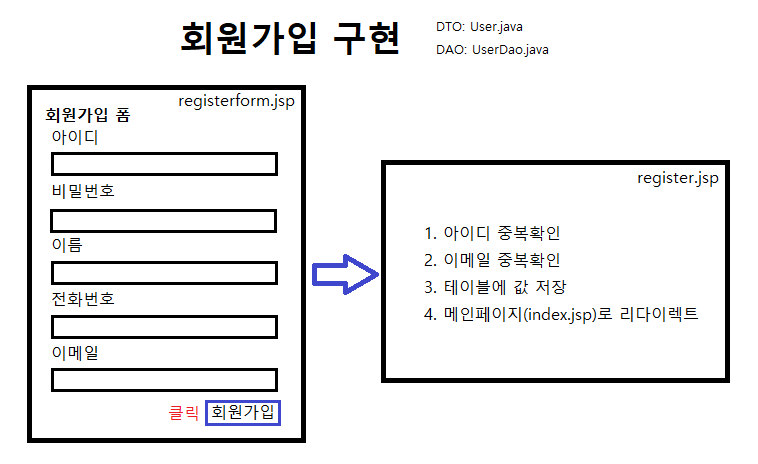
DAO: UserDao.java
/* import부분 생략 */
public class UserDao {
public void insertUser(User user) throws SQLException {
String sql = "insert into tb_comm_users(user_no, user_id, user_password, "
user_name, user_tel, user_email) "
+ "values(comm_user_seq.nextval, ?,?,?,?,?)";
Connection connection = getConnection();
PreparedStatement pstmt = connection.prepareStatement(sql);
pstmt.setString(1, user.getId());
pstmt.setString(2, user.getPassword());
pstmt.setString(3, user.getName());
pstmt.setString(4, user.getTel());
pstmt.setString(5, user.getEmail());
pstmt.executeUpdate();
pstmt.close();
connection.close();
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!doctype html>
<html lang="ko">
<head>
<title>커뮤니티 게시판::가입폼</title>
</head>
<body>
<div class="container">
<div class="row mb-3">
<div class="col">
<h1 class="fs-3">회원가입 폼</h1>
</div>
</div>
<div class="row mb-3">
<div class="col-5">
/* Dao에서 넘겨받을 fail 값을 저장하는 변수 정의 */
<%
String failReason = request.getParameter("fail");
%>
<%
// failReason = (registerform.jsp?fail=id)
if ("id".equals(failReason)){
%>
<div class="alert alert-danger">
<strong>회원가입 실패</strong> 이미 사용중인 아이디입니다.
</div>
<% } %>
<%
// failReason = (registerform.jsp?fail=email)
if ("email".equals(failReason)){
%>
<div class="alert alert-danger">
<strong>회원가입 실패</strong> 이미 사용중인 이메일입니다.
</div>
<% } %>
<form class="border p-3 bg-light" method="post" action="register.jsp">
<div class="mb-3">
<label class="form-label" for="user-id">아이디</label>
<input type="text" class="form-control" name="id" id="user-id">
</div>
<div class="mb-3">
<label class="form-label" for="user-password">비밀번호</label>
<input type="password" class="form-control" name="password" id="user-password">
</div>
<div class="mb-3">
<label class="form-label" for="user-name">이름</label>
<input type="text" class="form-control" name="name" id="user-name">
</div>
<div class="mb-3">
<label class="form-label" for="user-tel">전화번호</label>
<input type="text" class="form-control" name="tel" id="user-tel">
</div>
<div class="mb-3">
<label class="form-label" for="user-email">이메일</label>
<input type="text" class="form-control" name="email" id="user-email">
</div>
<div class="mb-3 text-end">
<button type="submit" class="btn btn-primary">회원가입</button>
</div>
</form>
</div>
</div>
</body>
</html>
- form과 함께 쓰이면 button은 자연적으로 submit기능을 갖게 됨
<button type="button">
으로 정의하면 submit기능 방지 가능
로직: register.jsp
<%@page import="com.sample.board.dao.UserDao"%>
<%@page import="com.sample.board.vo.User"%>
<%@page import="org.apache.commons.codec.digest.DigestUtils"%>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%
String id = request.getParameter("id");
String password = request.getParameter("password");
String name = request.getParameter("name");
String tel = request.getParameter("tel");
String email = request.getParameter("email");
// password를 암호화
String secretPassword = DigestUtils.sha256Hex(password);
User user = new User();
user.setId(id);
user.setPassword(secretPassword);
user.setName(name);
user.setTel(tel);
user.setEmail(email);
UserDao userDao = new UserDao();
// 아이디 중복검사
User savedUser = userDao.getUserById(id);
if (savedUser != null) {
// registerform으로 이동 후 registerform 내의 경고문 출력
response.sendRedirect("registerform.jsp?fail=id");
return;
}
// 이메일 중복 검사
savedUser = userDao.getUserByEmail(email);
if (savedUser != null) {
// registerform으로 이동 후 registerform 내의 경고문 출력
response.sendRedirect("registerform.jsp?fail=email");
return;
}
// 사용자정보를 테이블에 저장시킨다.
userDao.insertUser(user);
// index.jsp로 redirect
response.sendRedirect("index.jsp?register=completed");
%>
로그인
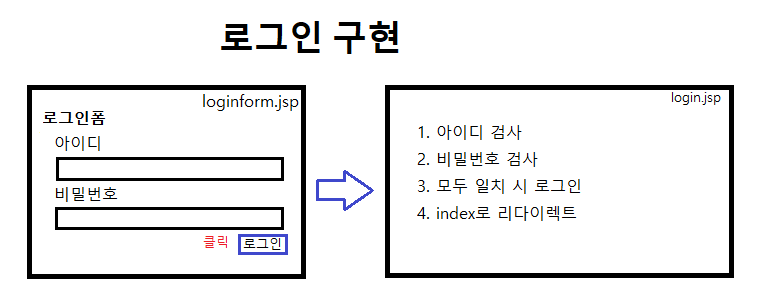
session
- JSP내장객체 중 하나로, 별도로 선언하지 않아도 사용 가능
- 클라이언트와 서버의 관계를 연결된 채로 유지하는 기능
- 주로 클라이언트 정보를 서버측에 저장할 때 사용
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!doctype html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet" >
<title>커뮤니티 게시판::로그인폼</title>
</head>
<body>
<%
pageContext.setAttribute("menu", "login");
%>
<%@ include file="common/navbar.jsp" %>
<div class="container">
<div class="row mb-3">
<div class="col">
<h1 class="fs-3">로그인 폼</h1>
</div>
</div>
<div class="row mb-3">
<div class="col-5">
<%
String fail = request.getParameter("fail");
if("id".equals(fail)){
%>
<div class="alert alert-danger">
<strong>로그인 실패</strong> 회원정보가 존재하지 않습니다.
</div>
<%
}
if("pwd".equals(fail)){
%>
<div class="alert alert-danger">
<strong>로그인 실패</strong> 비밀번호가 일치하지 않습니다.
</div>
<%
}
if("required".equals(fail)){
%>
<div class="alert alert-danger">
<strong>접근 실패</strong> 로그인이 필요합니다.
</div>
<%
}
%>
<form class="border p-3 bg-light" method="post" action="login.jsp">
<div class="mb-3">
<label class="form-label" for="user-id">아이디</label>
<input type="text" class="form-control" name="id" id="user-id">
</div>
<div class="mb-3">
<label class="form-label" for="user-password">비밀번호</label>
<input type="password" class="form-control" name="password" id="user-password">
</div>
<div class="mb-3 text-end">
<button type="submit" class="btn btn-primary">로그인</button>
</div>
</form>
</div>
<div class="col-7">
<img class="img-fluid m-2" src="resources/images/banners/banner1.png">
<img class="img-fluid m-2" src="resources/images/banners/banner2.jpg">
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
로직: login.jsp
<%@page import="org.apache.commons.codec.digest.DigestUtils"%>
<%@page import="com.sample.board.vo.User"%>
<%@page import="com.sample.board.dao.UserDao"%>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%
String id = request.getParameter("id");
String password = request.getParameter("password");
UserDao userDao = UserDao.getInstance();
User user = userDao.getUserById(id);
if (user == null) { // id에 해당하는 회원정보가 존재하지 않음
response.sendRedirect("loginform.jsp?fail=id");
return;
}
String secretPassword = DigestUtils.sha256Hex(password);
if (!user.getPassword().equals(secretPassword)) { // 비밀번호가 일치하지 않음
response.sendRedirect("loginform.jsp?fail=pwd");
return;
}
// 회원정보가 존재하고, 비밀번호도 일치함
session.setAttribute("LOGIN_USER_INFO", user);
response.sendRedirect("index.jsp");
%>