✅ Stack
: 상자에 물건을 쌓아 올리듯이 데이터를 쌓는 자료 구조
🌐 특징
- 먼저 들어간 자료가 나중에 나옴 → 후입선출(LIFO, Last In First Out) 구조
- 인터럽트처리, 수식의 계산, 서브루틴의 복귀 번지 저장 등에 쓰임
- 그래프의 깊이 우선 탐색(DFS)에서 사용
- 재귀적(Recursion) 함수를 호출할 때 사용
🌐 기능
push()
: 스택에 데이터를 추가
pop()
: 스택에서 데이터를 회수
isEmpty()
: 스택이 비어있는지 확인
peek()
: 스택의 제일 위에 무슨 자료가 있는지 확인
isFull()
: 스택이 가득 차 있는지 확인
🚧 예제 코드
public class StackEx {
private final int[] arr = new int[10];
priavte int top = -1;
public StackEx() {}
public void push(int data) {
if (arr.length - 1 == top) {
throw new RuntimeException("Stack is full");
}
top++;
arr[top] = data;
}
public int pop() {
if (top == -1) {
throw new RuntimeException("Stack is empty");
}
int temp = arr[top];
top--;
return temp;
public int peek() {
if (top == -1) {
throw new RuntimeException("Stack is empty");
}
return arr[top];
}
public boolean isEmpty() {
return top == -1;
}
public static void main(String[] args) {
StackEx stack = new StackEx();
stack.push(3);
stack.push(5);
stack.push(7);
System.out.println(stack.peek());
System.out.println(stack.pop());
System.out.println(stack.pop());
System.out.println(stack.pop());
System.out.println(stack.isEmpty());
}
}
✅ Stack_DFS
: 깊이 우선 탐색(Depth-First Search), 그래프에서 깊은 부분을 우선적으로 탐색함
🌐 장단점
🔸 장점
- 구현이 너비 우선 탐색(BFS)보다 간단함
- 현재 경로 상의 노드들만 기억하면 되므로, 저장 공간의 수요가 비교적 적음
- 목표 노드가 깊은 단계에 있는 경우 빨리 구할 수 있음
🔹 단점
- 단순 검색 속도는 너비 우선 탐색(BFS)보다 느림
- 답이 없는 경우에 빠질 가능성이 있음
- 구한 답이 최단 경로가 된다는 보장이 없음
💡 동작 방식
- 탐색 시작 노드를 스택에 삽입하고, 방문 처리
- 스택의 최상단 노드에 방문하지 않은 인접 노드가 있으면 그 인접 노드를 스택에 넣고 방문 처리하고, 방문하지 않은 인접 노드가 없으면 스택에서 최상단 노드를 꺼냄
- 위의 1번과 2번 과정을 더 이상 수행할 수 없을 때까지 반복
🚧 예제 코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.Stack;
public class DepthFirstSearch {
public void solution() throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
int maxNodes = Integer.parseInt(reader.readLine());
int[][] edgeMap = new int[maxNodes + 1][maxNodes + 1];
String[] edges = reader.readLine().split(" ");
for (int i = 0; i < edges.length / 2; i++) {
int leftNode = Integer.parseInt(edges[i * 2]);
int rightNode = Integer.parseInt(edges[i * 2 + 1]);
edgeMap[leftNode][rightNode] = 1;
edgeMap[rightNode][leftNode] = 1;
}
Stack<Integer> toVisit = new Stack<>();
boolean[] visited = new boolean[maxNodes + 1];
List<Integer> visitedOrder = new ArrayList<>();
int next = 1;
toVisit.push(next);
while (!toVisit.empty()) {
next = toVisit.pop();
if (visited[next]) continue;
visited[next] = true;
visitedOrder.add(next);
for (int i = maxNodes; i > 0; i--) {
if (edgeMap[next][i] == 1 && !visited[i]) {
toVisit.push(i);
}
}
}
System.out.println(visitedOrder);
}
public static void main(String[] args) throws IOException {
new DepthFirstSearch().solution();
}
}
✅ Queue_BFS
: 너비 우선 탐색(Breadth-First Search),기준노드에서 인접한 노드를 먼저 탐색
👉 주로 두 지점의 최단경로 및 임의의 경로를 찾고싶을 때 사용
🌐 특징
- 직관적이지 않음
- 어떤 노드를 방문했는지 반드시 확인해야함 → 무한 루프 방지
- 방문한 노드를 차례로 저장한 후 꺼내는 Queue 자료구조 사용
💡 동작 방식
- 방문한 점에서 도달할 수 있는 점들을 살펴보고, 아직 방문하지 않은 점들을 큐에 enQueue함
- 큐에서 점의 정보를 deQueue하고 방문하고, 이후 다시 1번으로 돌아감
- 큐가 빌때까지 반복
🚧 예제 코드
public BFS {
public void solution() throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
int maxNodes = Integer.parseInt(reader.readLine());
int[][] adjMap = new int[maxNodes + 1][maxNodes + 1];
String[] edges = reader.readLine().split(" ");
for(int i = 0; i < edges.length / 2; i++) {
int leftNode = Integer.parseInt(edge[i * 2]);
int rightNode = Integer.parseInt(edge[i * 2 + 1]);
adjMap[leftNode][rightNode] = 1;
adjMap[rightNode][leftNode] = 1;
}
Queue<Integer> toVisit = new LinkedList<>();
List<Integer> visitedOrder = new ArrayList<>();
boolean[] visited = new boolean[maxNodes + 1];
int next = 1;
toVisit.offer(next);
while(!toVisit.isEmpty()) {
next = toVisit.poll();
if (visited[next]) continue;
visited[next] = true;
visitOrder.add(next);
for (int i = 0; i < maxNodes + 1; i++) {
if (adjMap[next][i] == 1 && !visited[i])
toVisit.offer(i);
}
}
System.out.println(visitedOrder);
}
public static void main(String[] args) throws IOException {
new BFS().solution();
}
}
👉 결과
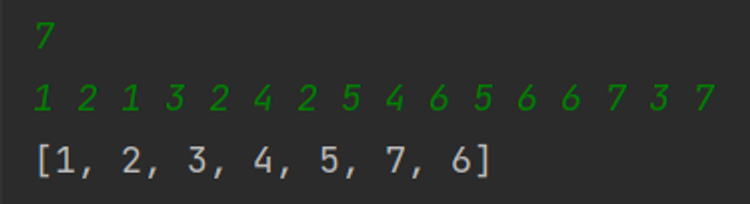
좋은 글 잘 읽었습니다, 감사합니다.