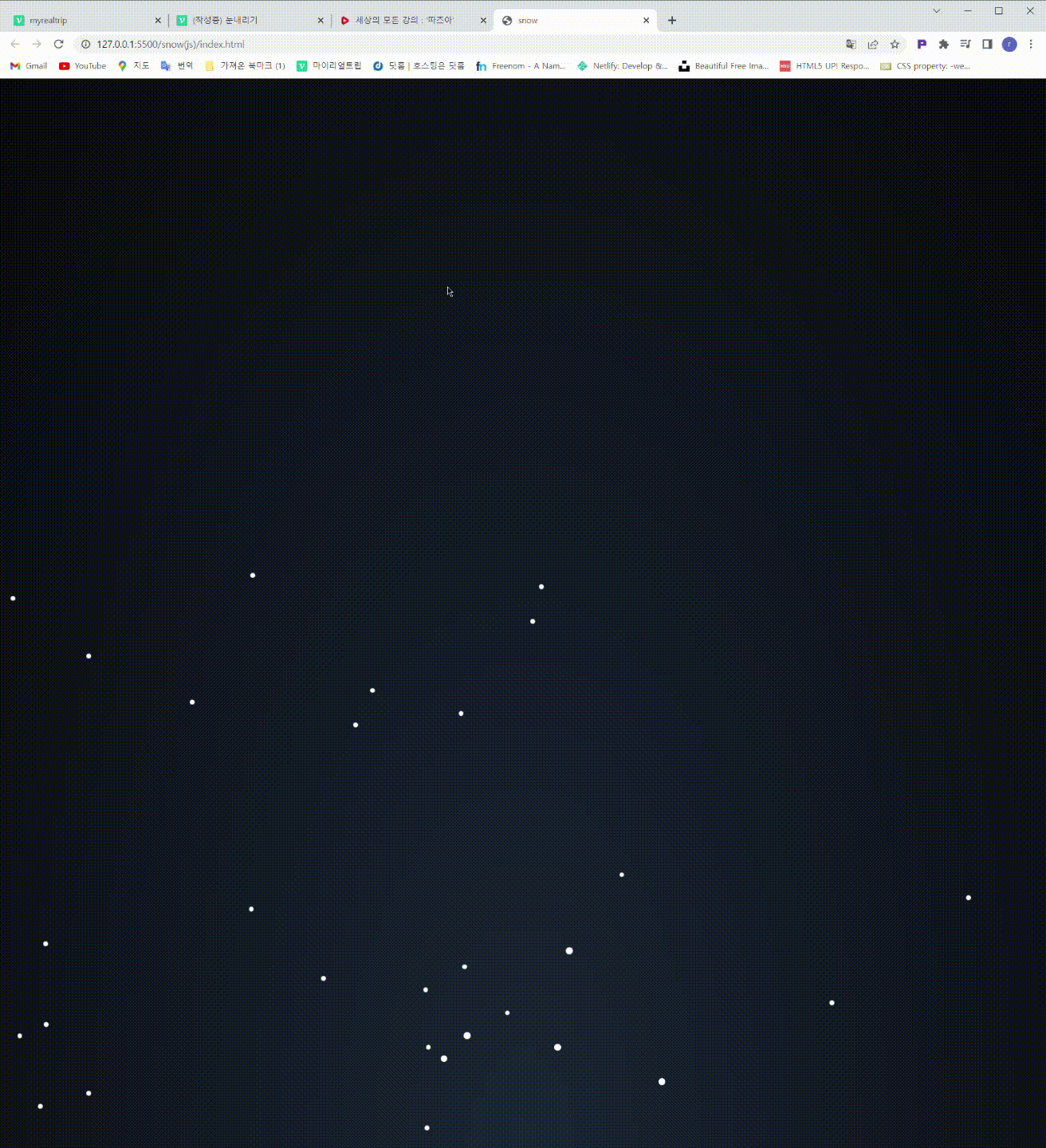
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>snow</title>
<link href="./css/style.css" rel="stylesheet" />
</head>
<body>
<script>
function createSnow() {
const el = document.createElement("div");
el.classList.add("snow");
el.style.marginLeft = randomPostion() + "px";
document.body.appendChild(el);
}
function randomPostion() {
return Math.floor(Math.random() * window.innerWidth);
}
for (let i = 0; i < 300; i++) {
createSnow();
}
</script>
</body>
</html>
body {
height: 100vh;
background: radial-gradient(ellipse at bottom, #1b2735 0%, #090a0f 100%);
overflow: hidden;
}
.snow {
width: 10px;
height: 10px;
background: #fff;
opacity: 0;
border-radius: 50%;
animation: snow 10s linear infinite;
}
.snow:nth-of-type(2n) {
width: 7px;
height: 7px;
animation-duration: 7s;
animation-delay: 1s;
}
.snow:nth-of-type(2n + 1) {
animation-duration: 8s;
animation-delay: 2s;
}
.snow:nth-of-type(9n) {
animation-duration: 9s;
animation-delay: 3s;
}
@keyframes snow {
0% {
opacity: 0;
transform: translateY(0);
}
20% {
opacity: 1;
transform: translate(-15px, 20vh);
}
40% {
opacity: 1;
transform: translate(15px, 40vh);
}
60% {
opacity: 1;
transform: translate(-15px, 60vh);
}
80% {
opacity: 1;
transform: translate(0, 80vh);
}
100% {
opacity: 1;
transform: translateY(100vh);
}
}