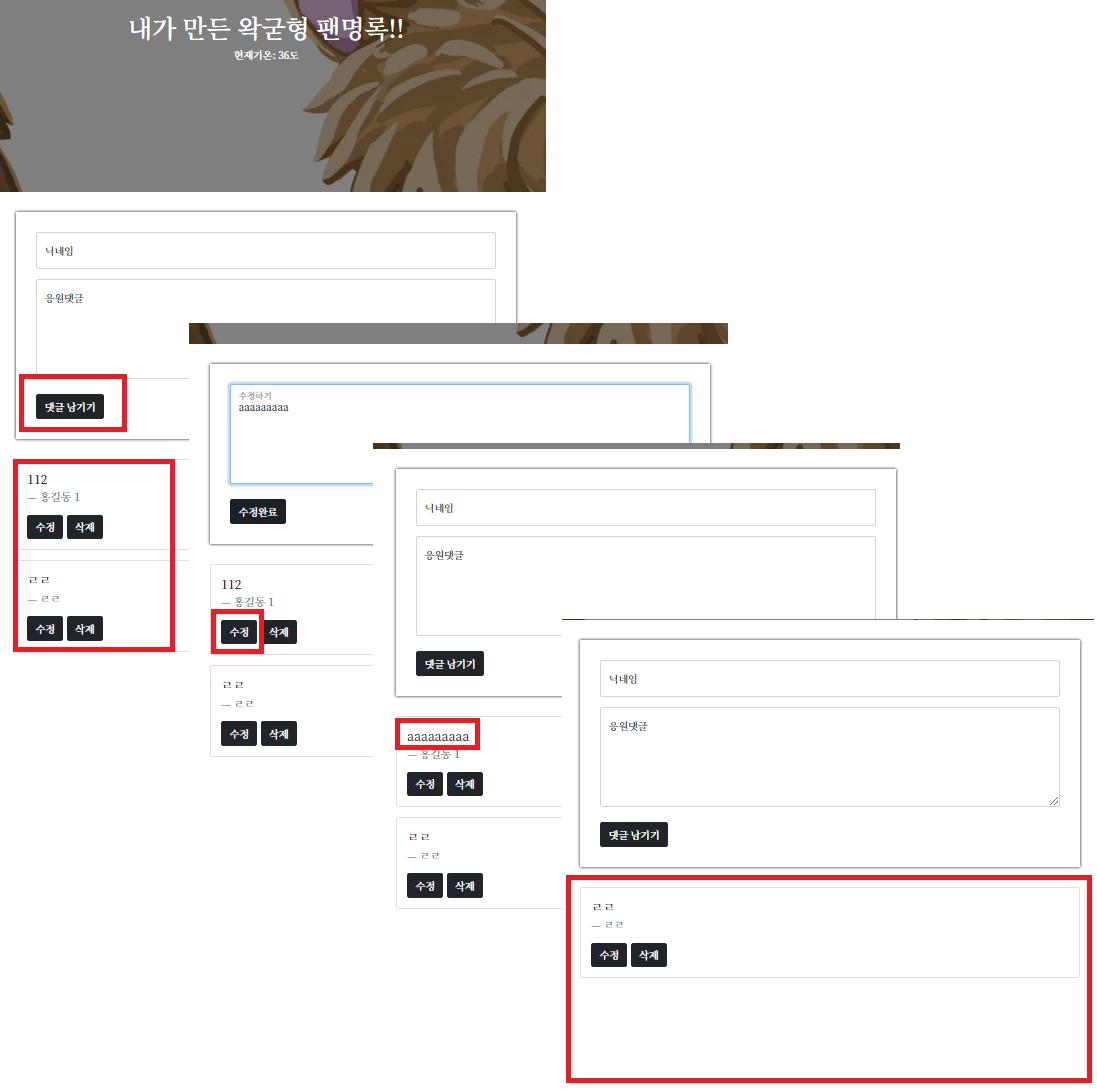
1. 댓글 남기기 버튼
app.py
POST
@app.route("/guestbook", methods=["POST"])
def guestbook_post():
name_receive = request.form['name_give']
comment_receive = request.form['comment_give']
comment_list = list(db.homework2.find({}, {'_id': False}))
count = len(comment_list) + 1
doc = {
'name':name_receive,
'comment':comment_receive,
'num': count
}
db.fan.insert_one(doc)
return jsonify({'msg': '저장 완료!'})
GET
@app.route("/guestbook", methods=["GET"])
def guestbook_get():
all_comments = list(db.fan.find({},{'_id':False}))
return jsonify({'result': all_comments})
index.html
function save_comment() {
let name = $('#name').val()
let comment = $('#comment').val()
let formData = new FormData();
formData.append("name_give", name);
formData.append("comment_give", comment);
fetch('/guestbook', { method: "POST", body: formData, }).then((res) => res.json()).then((data) => {
alert(data["msg"]);
window.location.reload()
});
}
function show_comment() {
fetch('/guestbook').then((res) => res.json()).then((data) => {
let rows = data['result']
$('#comment-list').empty()
rows.forEach((a) => {
let name = a['name']
let comment = a['comment']
let num = a['num']
let temp_html = `<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>${comment}</p>
<footer class="blockquote-footer">${name}</footer>
<button onclick="modify_comment('${num}')" type="button" class="btn btn-dark">수정</button>
<button onclick="delete_comment('${num}')" type="button" class="btn btn-dark">삭제</button>
</blockquote>
</div>
</div>`
$('#comment-list').append(temp_html)
})
})
}
2. 삭제 버튼
app.py
@app.route("/guestbook/delete", methods=["POST"])
def delete_post():
num_receive = request.form['num_give']
db.fan.delete_one({'num': int(num_receive)})
return jsonify({'result': 'success','msg':'삭제 완료!'})
index.html
function delete_comment(num) {
let formData = new FormData();
formData.append("num_give", num);
fetch('/guestbook/delete', { method: "POST", body: formData }).then((response) => response.json()).then((data) => {
alert(data["msg"])
window.location.reload()
});
}
3. 수정 버튼
app.py
@app.route("/guestbook/modify", methods=["POST"])
def modify_comment_post():
comment_receive = request.form['comment_give']
num_receive = request.form['num_give']
db.fan.update_one({'num': int(num_receive)}, {'$set': {'comment': comment_receive}})
return jsonify({'msg':'수정 완료!'})
index.html
function modify_comment(num) {
// mypost 클래스에 적용 (닉네임, 응원댓글, 댓글 남기기) -->
$('.mypost').empty()
let temp_html = `<div class="form-floating">
<textarea class="form-control" placeholder="Leave a comment here" id="modify_comment"
style="height: 100px"></textarea>
<label for="floatingTextarea2">수정하기</label>
</div>
<button onclick="modify_comment_post('${num}')" type="button" class="btn btn-dark">수정완료</button>`
$('.mypost').append(temp_html)
}
function modify_comment_post(num) {
let modify_comment = $('#modify_comment').val()
let formData = new FormData();
formData.append("num_give", num);
formData.append("comment_give", modify_comment);
fetch('/guestbook/modify', { method: "POST", body: formData, }).then((res) => res.json()).then((data) => {
alert(data["msg"]);
window.location.reload()
});
}