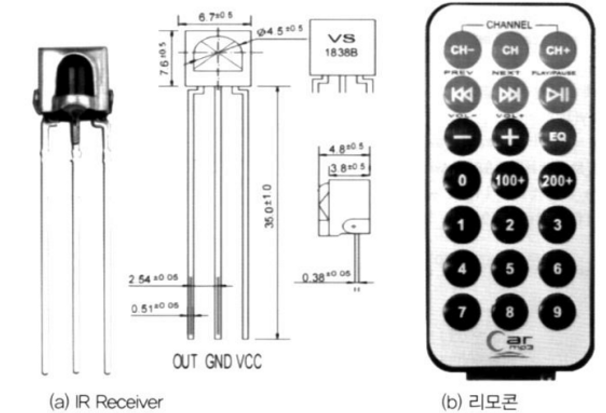
- IR Reciver: (IR 수신기): 송신기로부터 적외선 신호를 수신하고 요청을 해석하는 구성요소
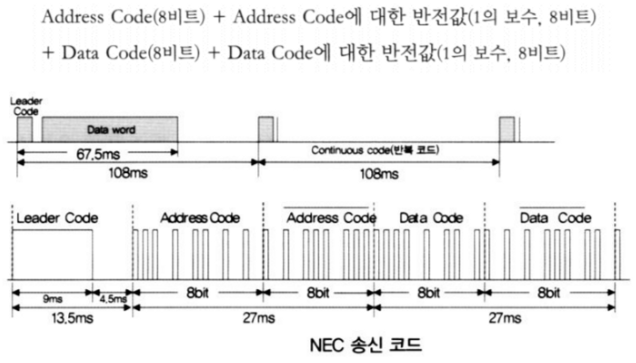
- NFC: 10cm 거리에서 13.56MHz의 주파수로 두 전자 기기가 통신할 수 있는 무선통신 기술
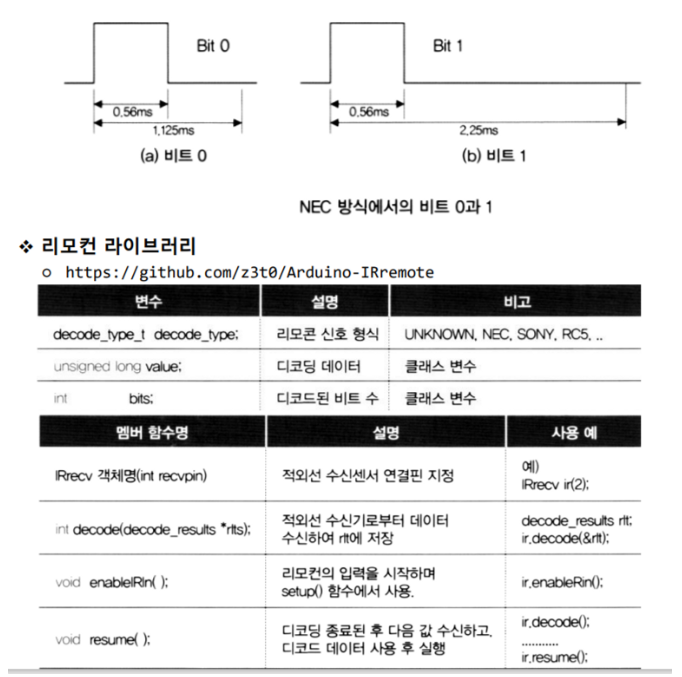
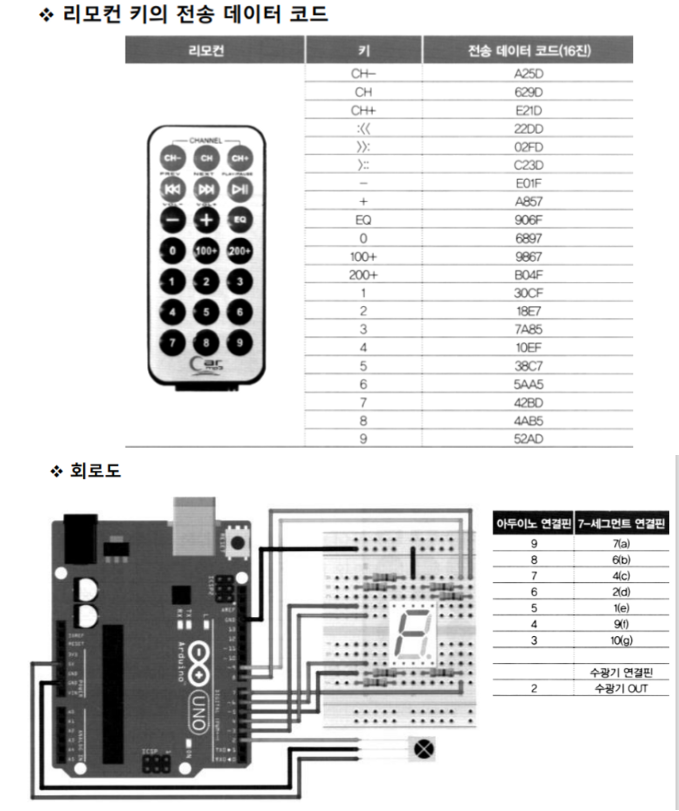
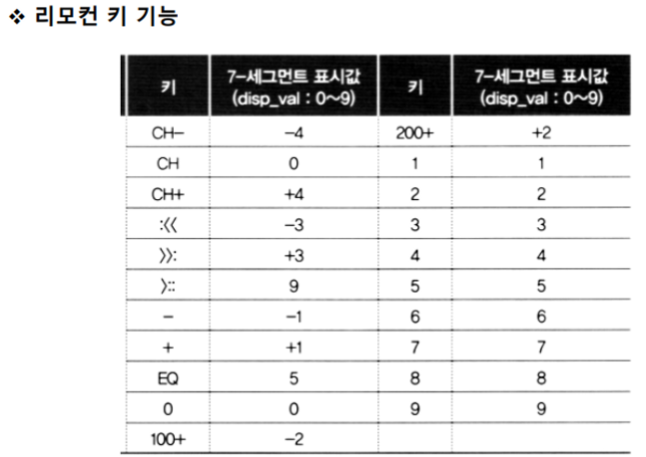
1. 리모컨 눌려진 키 시리얼 모니터 표시하기
#include <IRremote.h>
const int REC_PIN = 2;
IRrecv myIR(REC_PIN);
decode_results rlt;
void setup()
{
Serial.begin(9600);
myIR.enableIRIn();
}
void loop()
{
byte key;
if(!myIR.decode(&rlt)) return;
if(rlt.decode_type == NEC){
key = (unsigned char)rlt.value;
if(key == 0x5D) Serial.println("Press CH-");
else if(key == 0x9D) Serial.println("Press CH");
else if(key == 0x1D) Serial.println("Press CH+");
else if(key == 0xDD) Serial.println("Press :<<");
else if(key == 0xFD) Serial.println("Press >>:");
else if(key == 0x3D) Serial.println("Press >::");
else if(key == 0x1F) Serial.println("Press -");
else if(key == 0x57) Serial.println("Press +");
else if(key == 0x6F) Serial.println("Press EQ");
else if(key == 0x97) Serial.println("Press 0");
else if(key == 0x67) Serial.println("Press 200-");
else if(key == 0x4F) Serial.println("Press 200+");
else if(key == 0xCF) Serial.println("Press 1");
else if(key == 0xE7) Serial.println("Press 2");
else if(key == 0x85) Serial.println("Press 3");
else if(key == 0xEF) Serial.println("Press 4");
else if(key == 0xC7) Serial.println("Press 5");
else if(key == 0xA5) Serial.println("Press 6");
else if(key == 0xBD) Serial.println("Press 7");
else if(key == 0xB5) Serial.println("Press 8");
else if(key == 0xAD) Serial.println("Press 9");
}
myIR.resume();
}
2. 리모컨 키에 따라 7 - 세그먼트 표시 값 설정
#include <IRremote.h>
const int REC_PIN = 2;
const int segment_pin[8] ={9, 8, 7, 6, 5, 4, 3};
const byte segment_pat[10][7] = {
{1, 1, 1, 1, 1, 1, 0},
{0, 1, 1, 0, 0, 0, 0},
{1, 1, 0, 1, 1, 0, 1},
{1, 1, 1, 1, 0, 0, 1},
{0, 1, 1, 0, 0, 1, 1},
{1, 0, 1, 1, 0, 1, 1},
{1, 0, 1, 1, 1, 1, 1},
{1, 1, 1, 0, 0, 0, 0},
{1, 1, 1, 1, 1, 1, 1},
{1, 1, 1, 0, 0, 1, 1}};
byte disp_val = 0;
void segment_dsp(void);
IRrecv myIR(REC_PIN);
decode_results rlt;
void setup()
{
byte n;
myIR.enableIRIn();
for(n = 0;n < 7;n++)
pinMode(segment_pin[n], OUTPUT);
segment_dsp();
}
void loop()
{
byte key, disp;
if(!myIR.decode(&rlt)) return;
if(rlt.decode_type == NEC){
key = (unsigned char)rlt.value;
if(key == 0x5D) disp_val = (disp_val + 6) % 10;
else if(key == 0x9D) disp_val = 0;
else if(key == 0x1D) disp_val = (disp_val + 4) % 10;
else if(key == 0xDD) disp_val = (disp_val + 7) % 10;
else if(key == 0xFD) disp_val = (disp_val + 3) % 10;
else if(key == 0x3D) disp_val = 9;
else if(key == 0x1F) disp_val = (disp_val + 9) % 10;
else if(key == 0x57) disp_val = (disp_val + 1) % 10;
else if(key == 0x6F) disp_val = 5;
else if(key == 0x67) disp_val = (disp_val + 8) % 10;
else if(key == 0x4F) disp_val = (disp_val + 2) % 10;
else if(key == 0x97) disp_val = 0;
else if(key == 0xCF) disp_val = 1;
else if(key == 0xE7) disp_val = 2;
else if(key == 0x85) disp_val = 3;
else if(key == 0xEF) disp_val = 4;
else if(key == 0xC7) disp_val = 5;
else if(key == 0xA5) disp_val = 6;
else if(key == 0xBD) disp_val = 7;
else if(key == 0xB5) disp_val = 8;
else if(key == 0xAD) disp_val = 9;
segment_dsp();
}
myIR.resume();
}
void segment_dsp() {
byte n;
for(n = 0;n < 7;n++)
digitalWrite(segment_pin[n], segment_pat[disp_val][n]);
}