chap 5. ARM Instruction Set
1. Introduction
ARM data types
- word : 32 bits
- floating point data type → ARM core에서는 제공 X
- 6개의 data type을 지원
- {byte, half-word, word} X {signed, unsigned}
- Half-word or byte types
- 레지스터에 저장될 때는 32bit으로 저장됨
⇒ unsigned data는 zero padding
⇒ signed data는 sign extension
- DTI에서만 사용 가능한 data type
Memory Organization
- ARM 주소 : 32bits
- 1 address ⇒ 1 byte (mu0와의 차이점)
- Endianness
- word를 메모리에 넣을 때 어떻게 넣을건지 결정
- 디폴트가 little endian
- ex. 0x12345678일 때
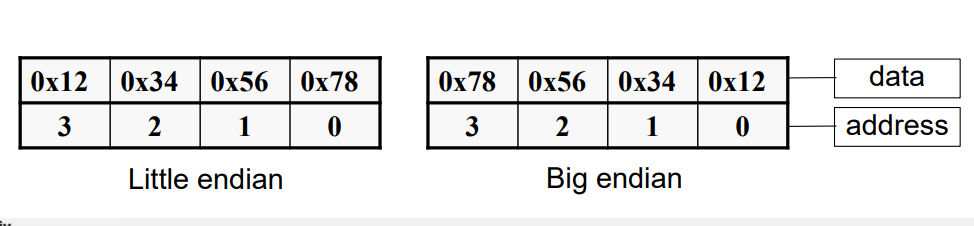
- little endian : 높은 자리수의 data가 높은 address에 저장
- big endian : 높은 자리수의 data가 낮은 address에 저장
- 둘 중 어떤 방식으로 데이터를 저장하던 상관없지만 일관성이 있어야함
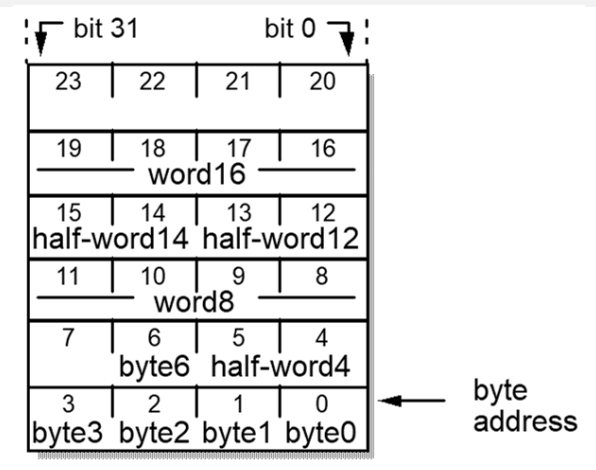
Priviledged mode
- exception과 supervisor calls(software interrupts)를 다루는 mode
- 대부분의 명령어는 user mode에서 실행됨
CPSR : Current Processor Status Register
- condition code : NZCV
- I/F : interrupt(IRQ)와 fast interrupt(FIQ)를 masking
- T : Thumb and ARM mode 결정
- Mode : 어떤 모드인지 알려줌
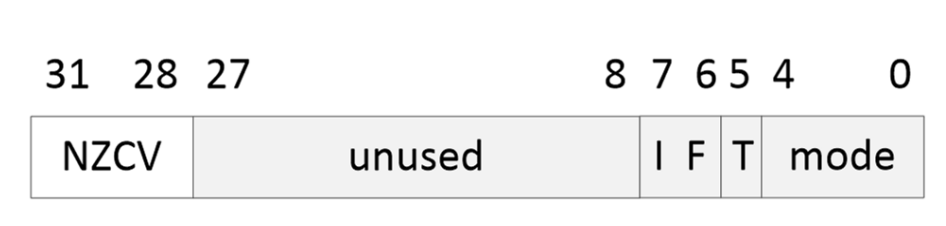
Operating modes
- User mode
- user program 실행
- 보호된 system에 직접 접근 X
- exception이 발생했을 때 privileged mode로 바껴야 함
(OS system program이 protected system resource에 접근)
- Privileged mode : exceptions and supervisor call
- 모든 system resource에 접근 가능
- System mode
- exception mode는 아님
- user mode의 register를 사용하여 모든 system resource에 접근 가능
- Exception modes
- FIQ, IRQ, Supervisor, Abort, Undefined
- 해당하는 exception이 발생했을 때 적절한 exception mode로 바뀜
- 각 exception mode마다 banked register가 존재(공용 레지스터 말고 고유한 레지스터가 존재함)
Register table
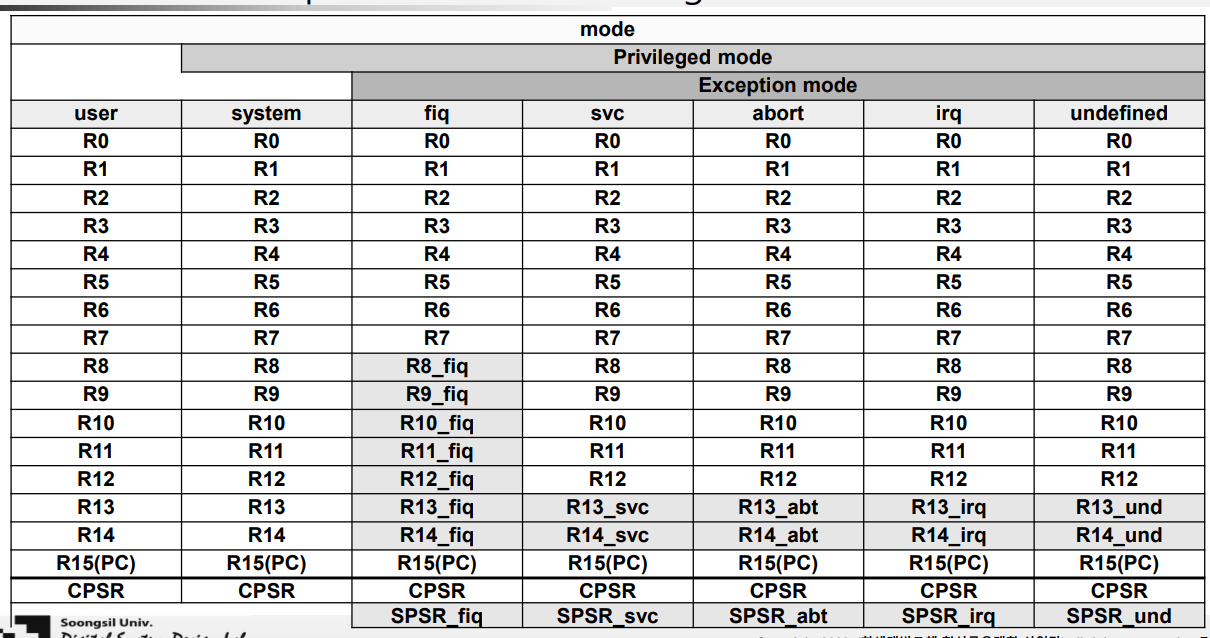
- fast interrupt
- stack에 register 값 저장하는 시간도 짧아야할 만큼 긴급한 상황이 발생할 때의 동작 mode
⇒ r8 - r12의 5개의 전용 register를 이용하여 우선 연산 진행
(추가적인 register 필요 시 레지스터 값을 메모리에 저장 후 사용)
- back up한 register값에 대해서만 restore한 후 fiq mode 빠져나감
- exception mode로 바꿀 때 이전 user or system mode의 r0-r12를 stack에 저장해둬야 함
⇒ stack pointer는 exception모드의 전용 r13에 저장됨
- exception mode의 전용 r14도 존재
⇒ 이전 mode의 명령어를 가리킴
- exception mode의 전용 SPSR
- exception mode로 변환할 때 이전 user or system mode의 CPSR 값을 저장하는 용도 ⇒ 동작을 멈췄던 상태에서 다시 시작할 수 있게 함
- mode를 한번에 여러번 바꿀 때 stack에서 데이터가 섞일 수 있음
⇒ 모든 exception 은 전용 stack을 사용해야 stack에서 data끼리 섞이지 않음
ARM status bits and operating modes
- DPI 명령어 뒤에 'S' 붙이면
arithmetic 명령어들은 모든 flags들을
logic and move 명령어들은 N과 Z를 set
shift 명령어들은 N, Z, C set(left shift 한정)
- ex
-1 + 1 = 0 : NZCV = 0110
2^31 -1 + 1 = -2^31 : NZCV = 1001 (signed에선 표현 불가)
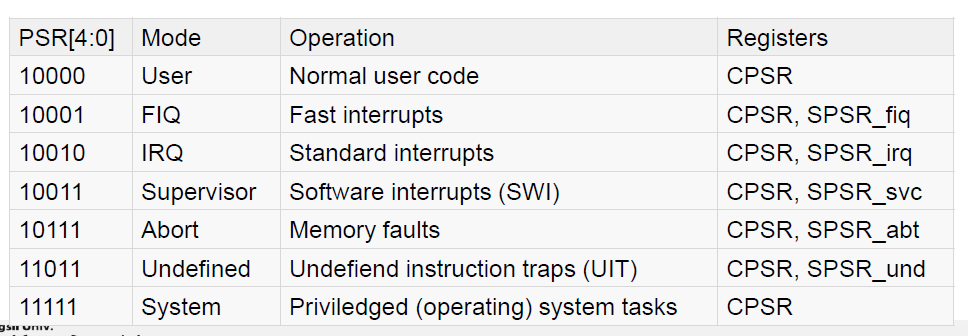
2. Exceptions
- 프로그램 실행 중에 unexpected events들을 handling
1. interrupts or memory faults
- SWI(supervisor) & undefined insturction traps : not unexpected
- Software reset : PC 켜거나 끌 때 → CPSR과 register값 유지 안 해도 괜찮
- memory fault
→ 메모리 결함, readonly인데 write 시도 등
3 groups of exception in ARM
- 명령어를 실행할 때 direct effect 발생하는 경우
⇒ SWI, UIT, prefetch abort
⇒ fetch할 때 memory fault가 발생하는 경우
- prefetch abort : memory fault가 발생해서 명령어를 읽어오지 못하는 경우 ⇒ abort mode에서 처리
- 잘못된 명령어를 읽어와서 memory fault가 발생해도 감지를 못 함 ⇒ undefined instruction trap에서 처리
- 명령어의 side-effect
⇒ data abort(data에 접근할 때 memory abort)
- 실행한 명령어를 제대로 작동 못 함 (명령어 시작은 했는데 완료를 못 함)
- 명령어 flow에 관계 없는 경우(외부에서 프로그램 실행과는 무관하게 발생하는 event)
⇒ Reset, IRQ, FIQ
Exceptions : exception Entry
- exception mode로 들어가는 과정
- exception이 발생하면 대부분의 경우 현재 실행중인 명령어를 완료하고 exception handle
- reset의 경우
현재 실행되고 있는 명령어를 완료할 필요가 없음
- side-effect or external exceptions
exception을 처리하기 위해 fetchm decode에 있는 명령어 버려 ⇒ pipeline 비우기(현재 실행 중인 명령 처리 X)
- Direct exceptions : 예상하는 순서대로 계속 명령어 실행
exception process의 순서
- CPSR change