How to use inits and enums
- 이니셜라이져를 통해 매번 같은 것을 입력하지 않도록 효율적으로 코딩
- 이넘을 이용해서 마찬가지로 더 간단하고 효율적으로 코딩할 수 있다
import SwiftUI
struct InitializerBootcamp: View {
let backgroundColor: Color
let count: Int
let title: String
init(count: Int, fruit: Fruit) {
self.count = count
if fruit == .apple {
self.title = "Apples"
self.backgroundColor = .red
} else {
self.title = "Oranges"
self.backgroundColor = .orange
}
}
enum Fruit {
case apple
case orange
}
var body: some View {
VStack(spacing: 12) {
Text("\(count)")
.font(.largeTitle)
.foregroundColor(.white)
.underline()
Text("\(title)")
.font(.headline)
.foregroundColor(.white)
}
.frame(width: 150, height: 150)
.background(backgroundColor)
.cornerRadius(10)
}
}
#Preview {
HStack {
InitializerBootcamp(count: 100, fruit: .apple)
InitializerBootcamp(count: 46, fruit: .orange)
}
}
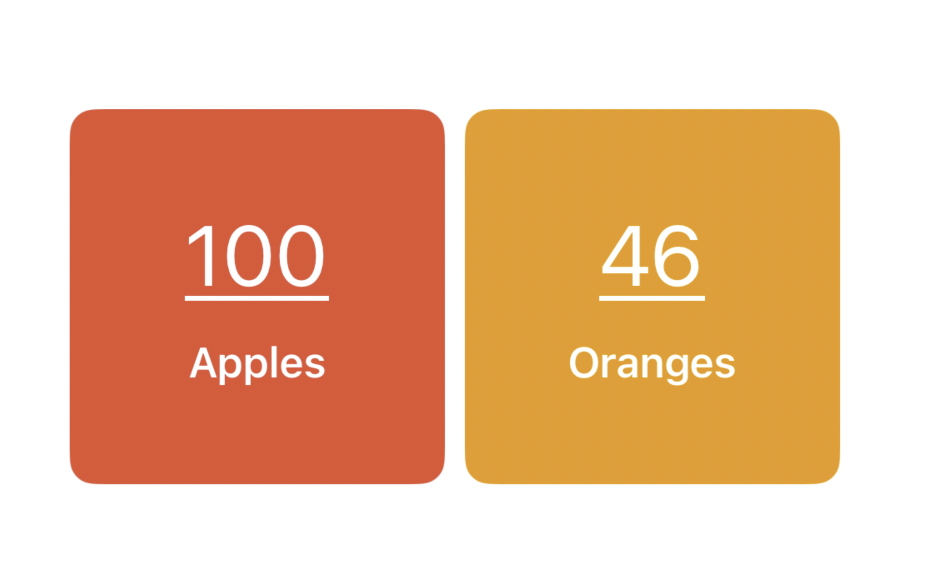