2가지만 기억하면 어느 프레임워크든 사용 가능하다.
express는 서버사이드의 프레임 워크의 일종이다.
npm (Node Package Manager)
- JavaScript 패키지 및 모듈의 설치, 관리, 공유, 및 실행을 담당하는 패키지 관리자
- What is Package?
- 패키지는 JavaScript 모듈, 라이브러리, 프레임워크, 플러그인 등의 코드 묶음
- 코드를 다른 프로젝트에서 사용하기 위해 패키징함
- Roles of npm
- Package Installation: npm allows easy installation of JavaScript packages created by other developers. (npm install package-name)
- Dependency Management: npm is responsible for managing project dependencies. You can specify these dependencies in your package.json file to precisely control your project's dependencies.
- Script Execution: You can define and run scripts in your project's package.json file, automating common tasks such as building, testing, and running a server.
- Package Publishing: npm enables you to publish your own packages, either publicly or privately, making them available for sharing and reuse with other developers.
npm command and package.json
- npm install package-name: Install a package.
- npm uninstall package-name: Remove a package.
- npm update package-name: Update a package.
- npm init: Create a package.json file for your project.
- npm start, npm test, npm run script-name: Execute project scripts.
- npm search package-name: Search for packages.
- npm publish: Publish a package.
- The package.json file stores configuration information for your project, including the project's name, version, a list of dependent packages, scripts, and more.
- ‘npx’ allows you to run an arbitrary command from a npm package (either one installed locally or fetched remotely)
Express JS Overview
Overview
-
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
-
Express provides a thin layer of fundamental web application features,
without obscuring Node.js features that you know and love.
- Request routing
- Static file server
- View engine integration
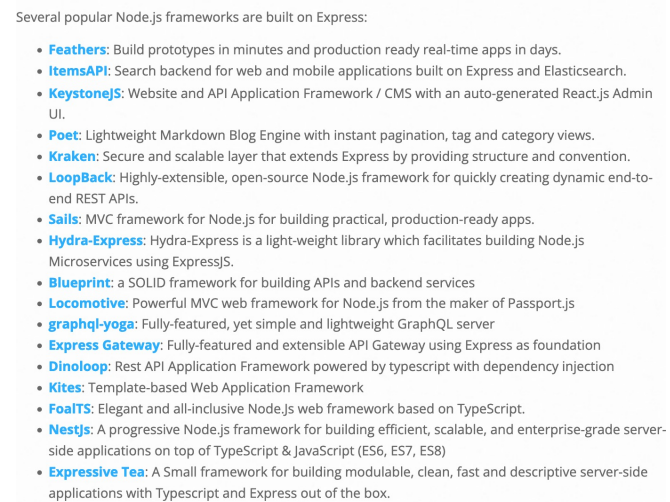
Working with Node
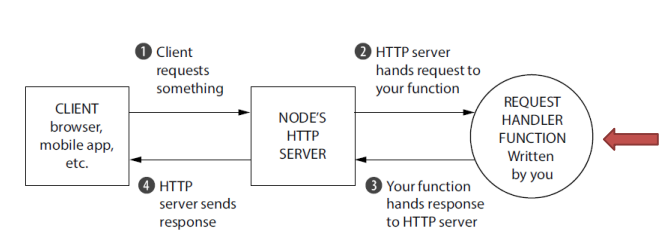
Working with Express
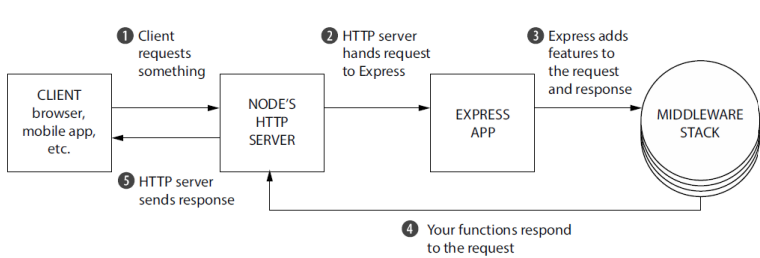
Express JS Introduction
Install Express and Express-generator
// install Express
$ mkdir myapp
$ cd myapp
$ npm init
$ npm install express
// install Express-generator
// for quickly creating an app skeleton
$ npx express-generator
// or
$ npm install–g express-generator
$ express
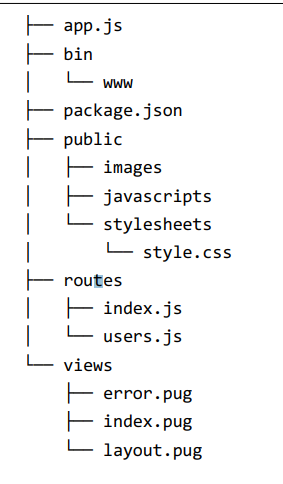
Generate Express Application Skeleton
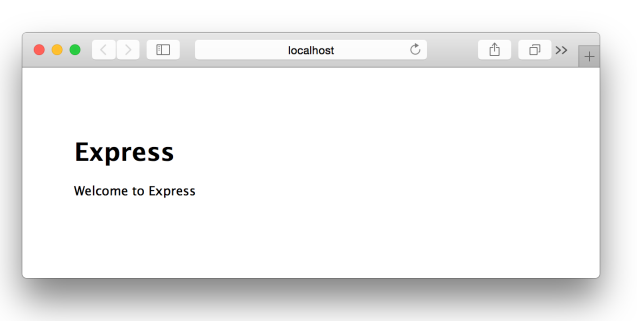
Creating Express App
- (Express) Generator creates for us.
- Creating several folders and files, giving developers a reasonable and predictable project structure to work in.
- Also creates a package.json file with some dependencies pre-filled in
- app.js is the main entry point for the application
- where logic for the web server resides.
여기에 웹서버에 대한 로직이 있다!
- public folder was created and seeded with subfolders for images, JavaScript files, and style sheets.
- routes folder has several files for declaring and attaching routes to the Express app.
- Since a complete web server there could be thousands of routes, a naïve routing would make the app.js file completely unmaintainable. It would also be difficult to work in a team because that file would constantly be changing
완전한 웹 서버는 수천 개의 루트가 있을 수 있기 때문에, 순진한 라우팅은 app.js 파일을 완전히 유지 관리할 수 없게 만들 것입니다. 또한 그 파일은 계속해서 변경될 것이기 때문에 팀에서 일하기도 어려울 것입니다.
package.json
- package.json is npm’s configuration file located in the root directory of the project
- Manages the version and dependency information for packages installed using npm
- Fields
- Package Name: the name of project or application
- Entry point: The first executed JS file (e.g., index.js or app.js)
Simple express ‘Hello World’
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => res.send('Hello World!'));
app.listen(port, () => console.log(`Example app listening on port ${port}!`))
const http = require('http');
const server = http.createServer((req, res) => {
res.end('Hello World\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log(`Server running at http://localhost:3000/`);
});
Routing
- Routing refers to how an application’s endpoints (URIs) respond to client
requests. (url을 특정 핸들러 함수로 매핑하는 프로세스)
- You define routing using methods of the Express app object that correspond to HTTP methods; for example, app.get() to handle GET requests and app.post to handle POST requests.
- You can also use app.all() to handle all HTTP methods and app.use() to specify middleware as the callback function.
- These routing methods specify a callback function (sometimes called “handler functions”) called when the application receives a request to the specified route (endpoint) and HTTP method.
이러한 라우팅 방법은 응용 프로그램이 지정된 경로(엔드포인트) 및 HTTP 메서드에 대한 요청을 수신할 때 호출되는 콜백 함수를 지정합니다.
- In other words, the application “listens” for requests that match the specified route(s) and method(s), and when it detects a match, it calls the specified callback function.
- In fact, the routing methods can have more than one callback function as arguments. With multiple callback functions, it is important to provide next as an argument to the callback function and then call next() within the body of the function to hand off control to the next callback.
Route Method
- Route definition takes the following structure: app.METHOD(PATH, HANDLER)
- app is an instance of express.
- METHOD is an HTTP request method, in lowercase.
- PATH is a path on the server.
- HANDLER is the function executed when the route is matched.
app.get('/', (req, res) => {
res.send('GET request to the homepage')
})
app.post('/', (req, res) => {
res.send('POST request to the homepage')
})
Route Paths and Parameters
- Route paths, in combination with a request method, define the endpoints at which requests can be made. Route paths can be strings, string patterns, or regular expressions
app.get('/about', (req, res) => {
res.send('about')
})
app.get('/random.text', (req, res) => {
res.send('random.text')
})
- Route parameters are named URL segments that are used to capture the values specified at their position in the URL. The captured values are populated in the req.params object, with the name of the route parameter specified in the path as their respective keys.
경로 매개 변수는 URL의 위치에 지정된 값을 캡처하는 데 사용되는 URL 세그먼트로 명명됩니다.
캡처된 값은 req.params 개체에 채워지며 경로에 지정된 경로 매개 변수의 이름이 각각의 키로 표시됩니다.
Route Handlers
- You can provide multiple callback functions that behave like middleware to handle a request. The only exception is that these callbacks might invoke next('route') to bypass the remaining route callbacks. You can use this mechanism to impose pre-conditions on a route, then pass control to subsequent routes if there’s no reason to proceed with the current route.
요청을 처리하기 위해 미들웨어처럼 작동하는 여러 콜백 함수를 제공할 수 있습니다. 유일한 예외는 이러한 콜백이 다음('경로')을 호출하여 나머지 경로 콜백을 우회할 수 있다는 것입니다. 이 메커니즘을 사용하여 경로에 사전 조건을 부여한 다음 현재 경로를 진행할 이유가 없으면 이후 경로에 제어권을 전달할 수 있습니다.
app.get('/example/b', (req, res, next) => {
console.log('the response will be sent by the next function ...')
next()
}, (req, res) => {
res.send('Hello from B!')
})
keyword bypass
Route: Response methods
- The methods on the response object (res) in the following table can send a response to the client, and terminate the request-response cycle.
- If none of these methods are called from a route handler, the client request will be left hanging
이러한 메서드 중 하나도 경로 처리기에서 호출되지 않으면 클라이언트 요청이 보류된 상태로 유지됩니다
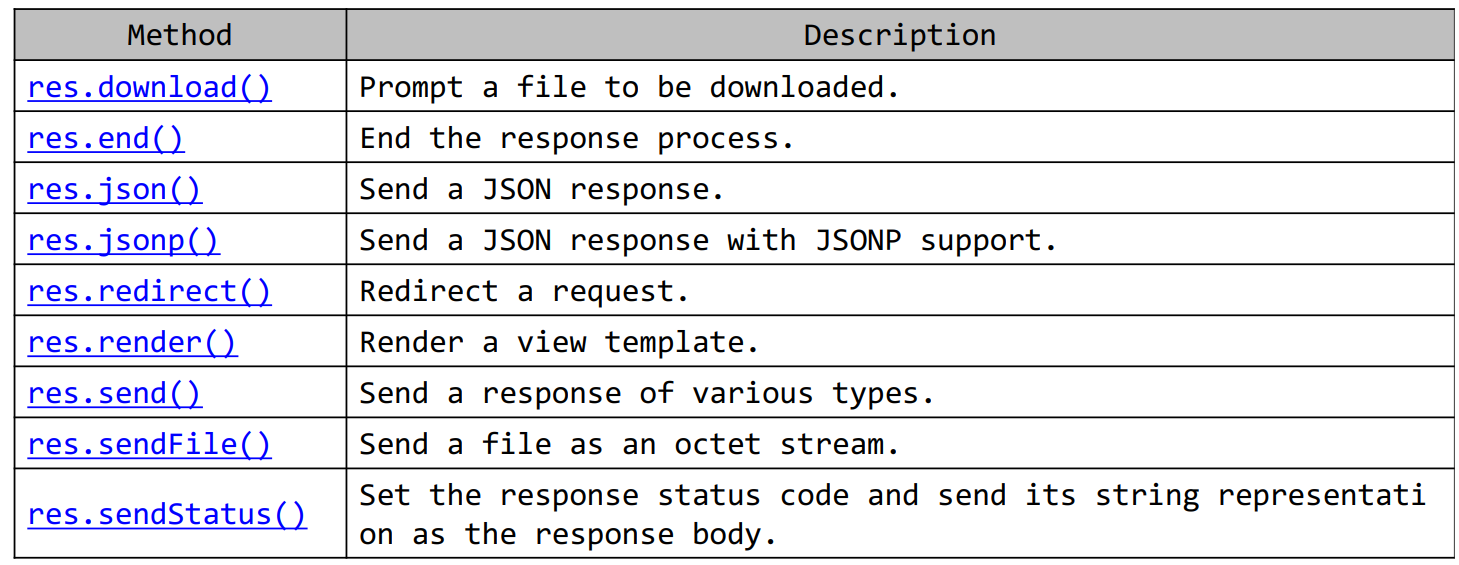
app.route()
- You can create chainable route handlers for a route path by using app.route(). Because the path is specified at a single location, creating modular routes is helpful, as is reducing redundancy and typos.
app.route('/book')
.get((req, res) => {
res.send('Get a random book')
})
.post((req, res) => {
res.send('Add a book')
})
.put((req, res) => {
res.send('Update the book')
})
- allows you to group multiple HTTP verb routes (e.g GET, POST,PUT) under a common URL path prefix.
express.Router
- Use the express.Router class to create modular, mountable route handlers.
A Router instance is a complete middleware and routing system; for this reason, it is often referred to as a “mini-app”.
- The following example creates a router as a module, loads a middleware function in it, defines some routes, and mounts the router module on a path in the main app.
modular
const express = require('express')
const router = express.Router()
router.use((req, res, next) => {
console.log('Time: ', Date.now())
next()
})
router.get('/', (req, res) => {
res.send('Birds home page')
});
router.get('/about', (req, res) => {
res.send('About birds')
})
module.exports = router
Middleware (요청과 응답 사이에서 작동하는 중간 소프트웨어)
- 미들웨어 함수는, 요청 오브젝트(req), 응답 오브젝트 (res), 그리고 다음의 미들웨어 함수 대한 액세스 권한을 갖는 함수
- 다음의 미들웨어 함수는 일반적으로 next라는 이름의 변수로 표시됨
- 현재의 미들웨어가 request-response 주기를 마무리하지 않는다면, 반드시 next()를 call 해야 함.
- App 이 처리해야 하는 logic을 여러 middleware 들로 정의하여, 연속하여 수행함 (decompose one large request handler into separate middleware functions.)
- Middleware의 종류
- Application-level middleware // bind to app
- Router-level middleware // bind to router
- Error-handling middleware // app.use(function(err, req, res, next)
- Built-in middleware // express.static
- Third-party middleware (ex. cookie-parser)
Application-Level Middleware of Express (expressjs.com)
- Bind application-level middleware to an instance of the app object by using the app.use(middleware) and app.METHOD(path, middleware) functions
- METHOD is the HTTP method of the request that the middleware function handles (such as GET, PUT, or POST) in lowercase
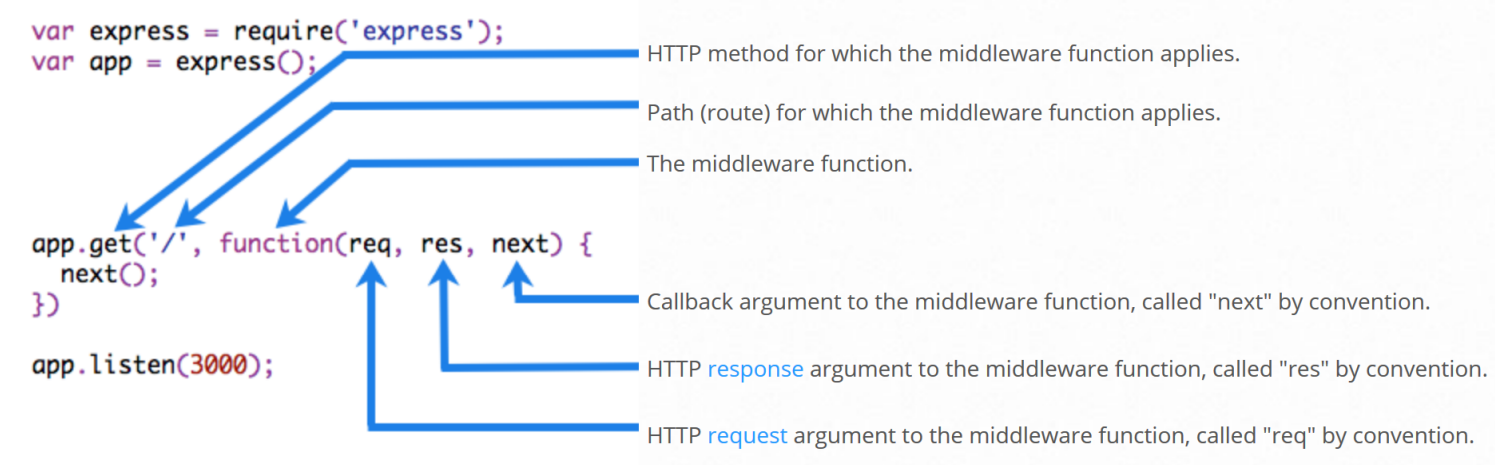
Application-Level Middleware of Express (example) – app_order.js
const express = require('express');
const app = express();
app.use((req, res, next) => {
console.log(`Request is ${req.method} ${req.path}`);
next();
});
app.get('/', (req, res) => {
res.end('Hello World!');
});
app.listen(8000, (err) => {
console.log('Server is running at 8000');
});
Order of Middleware
- the order in which they are written/included in your file is the order in which they are executed (the route matches)
var express = require('express');
var app = express();
app.use(function(req, res, next){
console.log("Start");
next();
});
app.get('/', function(req, res, next){
res.send(“Hello World!");
console.log("Middle");
next();
});
app.use('/', function(req, res){
console.log('End');
}); app.listen(3000);
Express Web Server with three routes
var express = require('express');
var app = express();
app.get('/teams/:teamName/employees/:employeeId', function (req, res, next) {
console.log('teamName = ' + req.params.teamName);
console.log('employeeId = ' + req.params.employeeId);
res.send('path one');
});
app.get('/teams/:teamName/employees', function (req, res, next) {
console.log('setting content type');
res.set('Content-Type', 'application/json');
res.locals.data = 100 ;
next();
}, function (req, res, next) {
console.log('teamName = ' + req.params.teamName);
console.log(res.locals.data);
res.send('path two');
});
app.get(/^\/groups\/(\w+)\/(\d+)$/, function (req, res, next) {
console.log('groupname = ' + req.params[0]);
console.log('groupId = ' + req.params[1]);
res.send('path three');
});
var server = app.listen(1337, function() {
console.log('Server started on port 1337');
});
Route matching with regular expression
- Route three illustrates using regular expression parameters.
/^\/groups\/(\w+)\/(\d+)$/
- \w+ : matches any word character
- \d+ : matches a digit(equal to [0-9])
- ^ : matches beginning of the input
- $ : matches end of input
- ^: 정규 표현식에서 문자열의 시작을 나타냅니다.
\/groups\/
: /groups/ 문자열과 정확히 일치해야 합니다.
(\w+)
: 경로에서 추출하고자 하는 그룹, 여기서는 그룹 이름(groupname)을 의미하며, \w+는 하나 이상의 단어 문자를 나타냅니다.
\/
: / 문자와 정확히 일치해야 합니다.
(\d+)
: 두 번째 그룹, 여기서는 그룹 ID(groupId)를 의미하며, \d+는 하나 이상의 숫자를 나타냅니다.
$
: 정규 표현식에서 문자열의 끝을 나타냅니다.
즉, 이 경로 패턴은 /groups/ 다음에 나오는 단어 문자로 이루어진 그룹 이름과 숫자로 이루어진 그룹 ID를 추출하는 역할을 합니다.
해당 라우트 핸들러에서는 추출된 그룹 이름과 그룹 ID를 콘솔에 출력하고, 클라이언트에게 'path three'라는 응답을 보내는 역할을 합니다.