📒 Examples
📌 Transparent NavBar
- Header부분 천천히 그라데이션(transition)
<style>
body{
height: calc(100vh + 100px);
margin : 0;
}
#header {
position: fixed;
top : 0;
left : 0;
width : 100vw;
transition: background-color 0.5s;
}
#header p{
text-align : center;
}
.bg-image{
background-image: linear-gradient(to right , #0ef, #0af);
height : 400px;
}
#header.active{
background-color: #fff;
}
</style>
<header id="header">
<p>Transparent Navbar</p>
</header>
<div class="bg-image"></div>
<script>
var header = document.getElementById("header");
document.addEventListener("scroll", function(){
var scrollTop = document.documentElement.scrollTop;
console.log(scrollTop);
if(scrollTop > 50){
header.classList.add("active");
}else{
header.classList.remove("active");
}
})
</script> -->
📌 Side bar
- open 버튼 누르면 링크사이드바나오고 바디 오버레이로 덮인다. 오버레이누르면 사이드바 사라짐(hidden)
<style>
#side-bar{
position : fixed;
top : 0;
left : 0;
width : 16rem;
height : 100vh;
background-color: #fff;
transition: 0.2s;
z-index : 1;
}
#side-bar ul{
margin-top : 4rem;
}
.link{
color : #000;
}
#overlay{
position : fixed;
top : 0; right : 0; bottom : 0; left : 0;
background-color: rgba(0 0 0 /0.2);
}
#side-bar.hidden{
left : -16rem;
}
#overlay.hidden{
display : none;
}
</style>
<h1>Side bar</h1>
<button id="btn">Open</button>
<nav id="side-bar" class="hidden">
<ul>
<li>
<a href="#" class="link">Link</a>
</li>
<li>
<a href="#" class="link">Link</a>
</li>
<li>
<a href="#" class="link">Link</a>
</li>
</ul>
</nav>
<div id="overlay" class="hidden"></div>
<script>
var btn = document.getElementById("btn");
var sidebar = document.getElementById("side-bar");
var overlay = document.getElementById("overlay");
btn.addEventListener("click",function(){
sidebar.classList.remove("hidden");
overlay.classList.remove("hidden");
})
overlay.addEventListener("click",function(){
sidebar.classList.add("hidden");
overlay.classList.add("hidden");
})
</script>
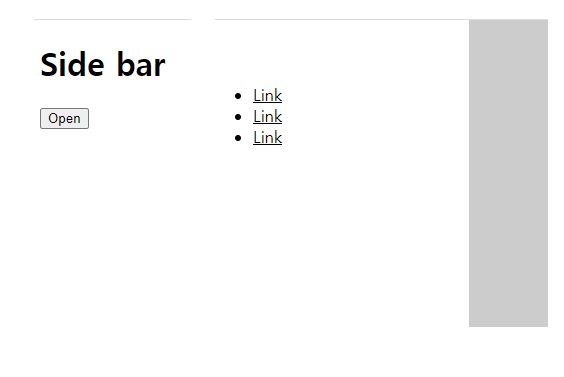
📌 Side bar + x 버튼
- 위 Side bar에서 width : 100vw 로 늘리고 X 버튼 눌렀을때 닫힌다(close).
<style>
#side-bar{
position : fixed;
top : 0;
left : 0;
width : 100vw;
height : 100vh;
background-color: #fff;
transition: 0.2s;
z-index : 1;
}
#side-bar ul{
margin-top : 4rem;
}
.link{
color : #000;
}
#side-bar.hidden{
left : -100vw;
}
#close{
position : absolute;
right : 0; font-size : 2rem;
padding : 1rem;
cursor: pointer;
}
</style>
<h1>Side bar + x 버튼 </h1>
<button id="open">Open</button>
<nav id="side-bar" class="hidden">
<span id="close">×</span>
<ul>
<li>
<a href="#" class="link">Link</a>
</li>
<li>
<a href="#" class="link">Link</a>
</li>
<li>
<a href="#" class="link">Link</a>
</li>
</ul>
</nav>
<script>
var open = document.getElementById("open");
var sidebar = document.getElementById("side-bar");
var close = document.getElementById("close");
open.addEventListener("click",function(){
sidebar.classList.remove("hidden");
})
close.addEventListener("click",function(){
sidebar.classList.add("hidden");
})
</script>
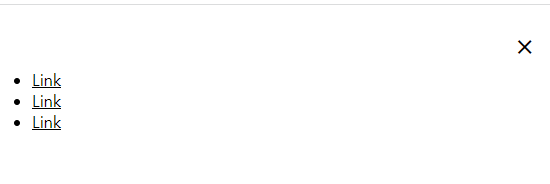
📌 Switch NavBar
- 스크롤 내릴때에는 header hidden되고 올릴때는 보이면서 main blur 처리된다.
<style>
body{
height : calc(100vh + 100px);
}
#header{
position : fixed; top : 0; left : 0;
width : 100vw; border-bottom: 1px solid #ddd;
backdrop-filter: blur(8px);
transition : top 0.2s;
}
.header-inner{
display : flex;
height : 50px;
justify-content : center;
align-items: center;
}
#header.hidden{
top: -50px;
}
main{
margin-top : 4rem;
}
</style>
<header id="header">
<div class="header-inner">
Header
</div>
</header>
<main>
<h1>Switch NavBar</h1>
<p>...</p>
</main>
<script>
var header = document.getElementById("header");
var prevScrollTop = 0;
document.addEventListener("scroll", function(){
var scrollTop = document.documentElement.scrollTop;
console.log("이전 스크롤탑 : ", prevScrollTop);
console.log("현재 스크롤탑 : ", scrollTop);
if(scrollTop > prevScrollTop){
header.classList.add("hidden");
}else{
header.classList.remove("hidden");
}
prevScrollTop = scrollTop;
})
</script>
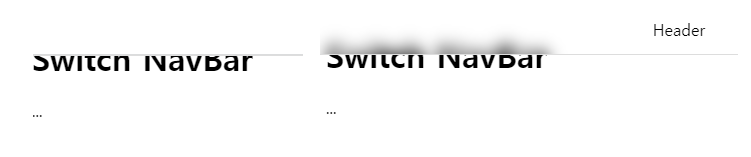
📌 Modal
- Open 버튼누르면 overlay 씌워지고 Modal창이 뜬다.
- Modal부분 제외하고 오버레이 클릭했을 때 overlay를 hidden처리한다.
<h1>Modal</h1>
<style>
#overlay{
position : fixed;
top : 0; left : 0;
width : 100%; height : 100%;
background-color: rgba(0 0 0 / 0.2);
}
.modal{
background-color: white;
box-shadow: 0 0 10px grey;
margin : 100px auto 0;
max-width: 20rem;
padding : 1rem;
border-radius: 1rem;
}
.hidden{
display: none;
}
</style>
<button id="btn">Open</button>
<div id="overlay" class="hidden">
<div class="modal">
<h1>Heading</h1>
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Optio mollitia unde consectetur et ipsam adipisci,
provident distinctio molestiae molestias quo hic incidunt
nulla magni delectus corporis excepturi temporibus
quibusdam labore.
</p>
</div>
</div>
<script>
var overlay = document.getElementById("overlay");
var btn = document.getElementById("btn");
btn.addEventListener("click", function(){
overlay.classList.remove("hidden");
})
overlay.addEventListener("click",function(e){
console.log(e.target);
if(this === e.target){
overlay.classList.add("hidden");
}
})
</script>
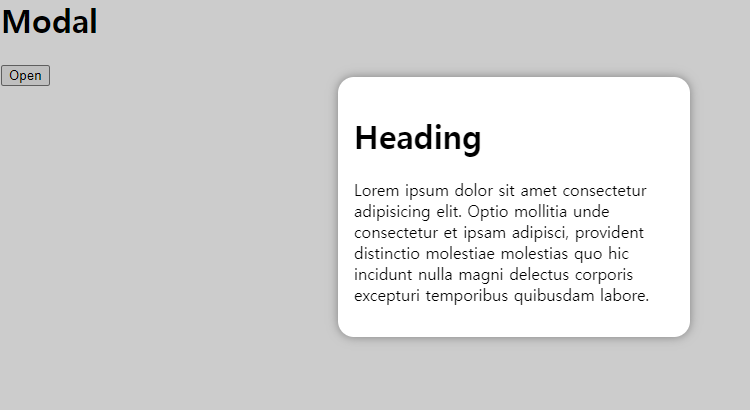