Three.js Light
- Ambient Light
- Directional Light
- Hemisphere Light
- Point Light
- RectArea Light
- Spot Light
Ambient Light
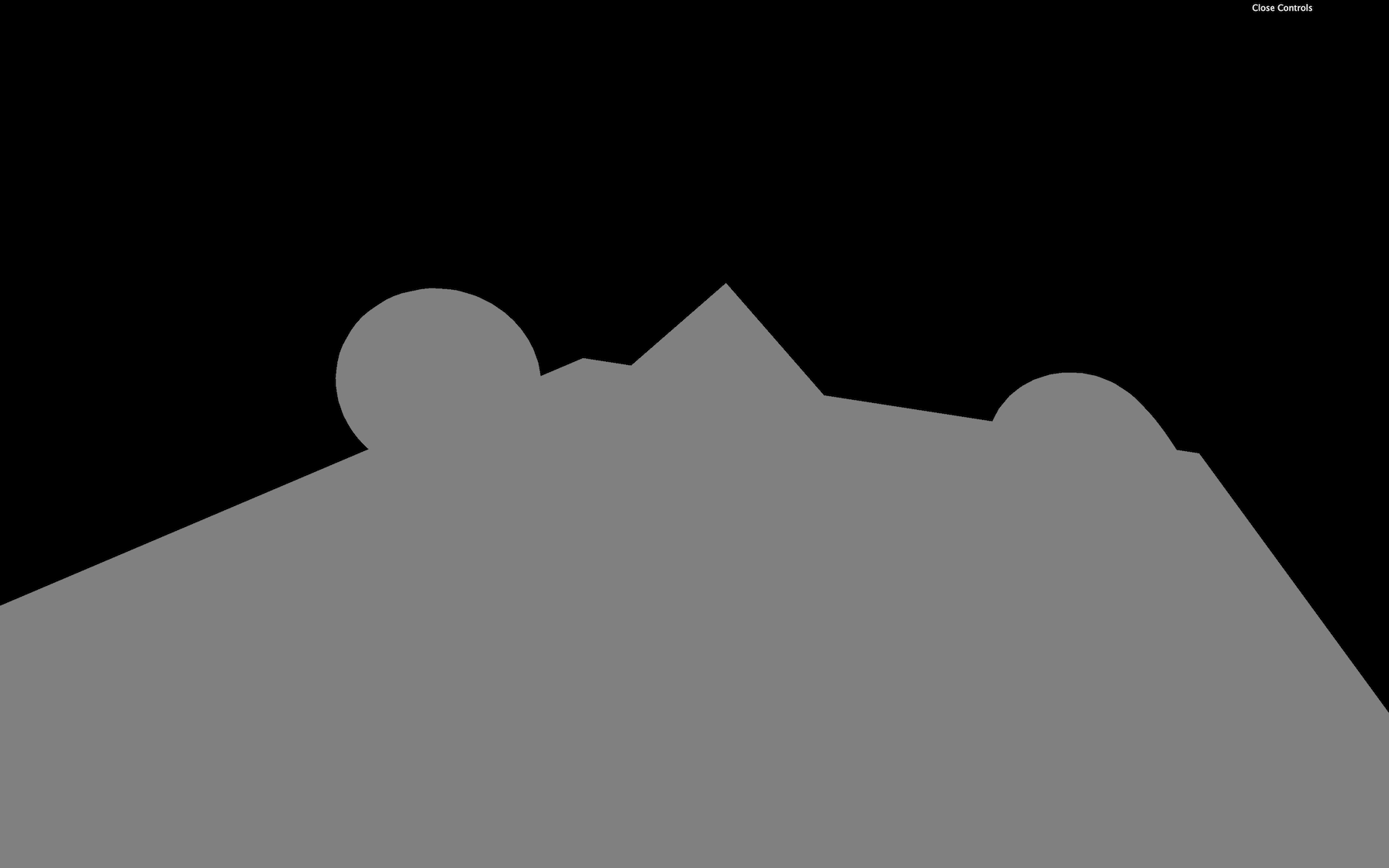
- Ambient Light는 전병향에서 모든 geometry들에 적용하는 Light
- Ambient Light 객체의 첫번째 parameter는 color
- 두번째 parameter는 intensity
const ambientLight = new THREE.AmbientLight(0xffffff, 1)
scene.add(ambientLight)
언제 사용하는가
- MeshBasicMaterial 처럼 모든 geometry들의 모든 면을 전체적으로 밝혀준다.
- Three.js에서는 light bounce를 지원하지 않는다.(performance 이슈) -> 약한 AmbientLight를 사용하면 fake light bounce를 표현 가능하다.
Directional Light
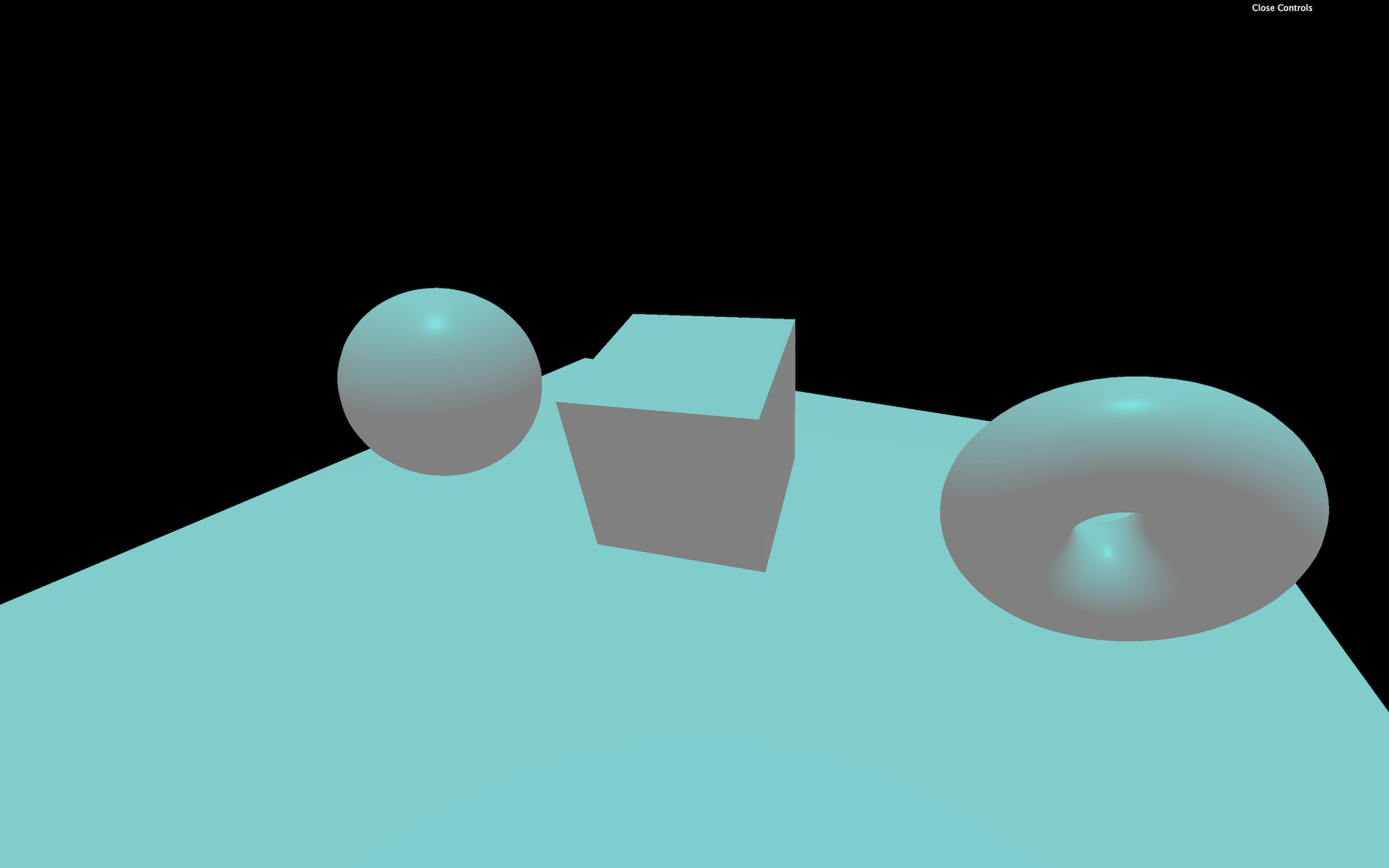
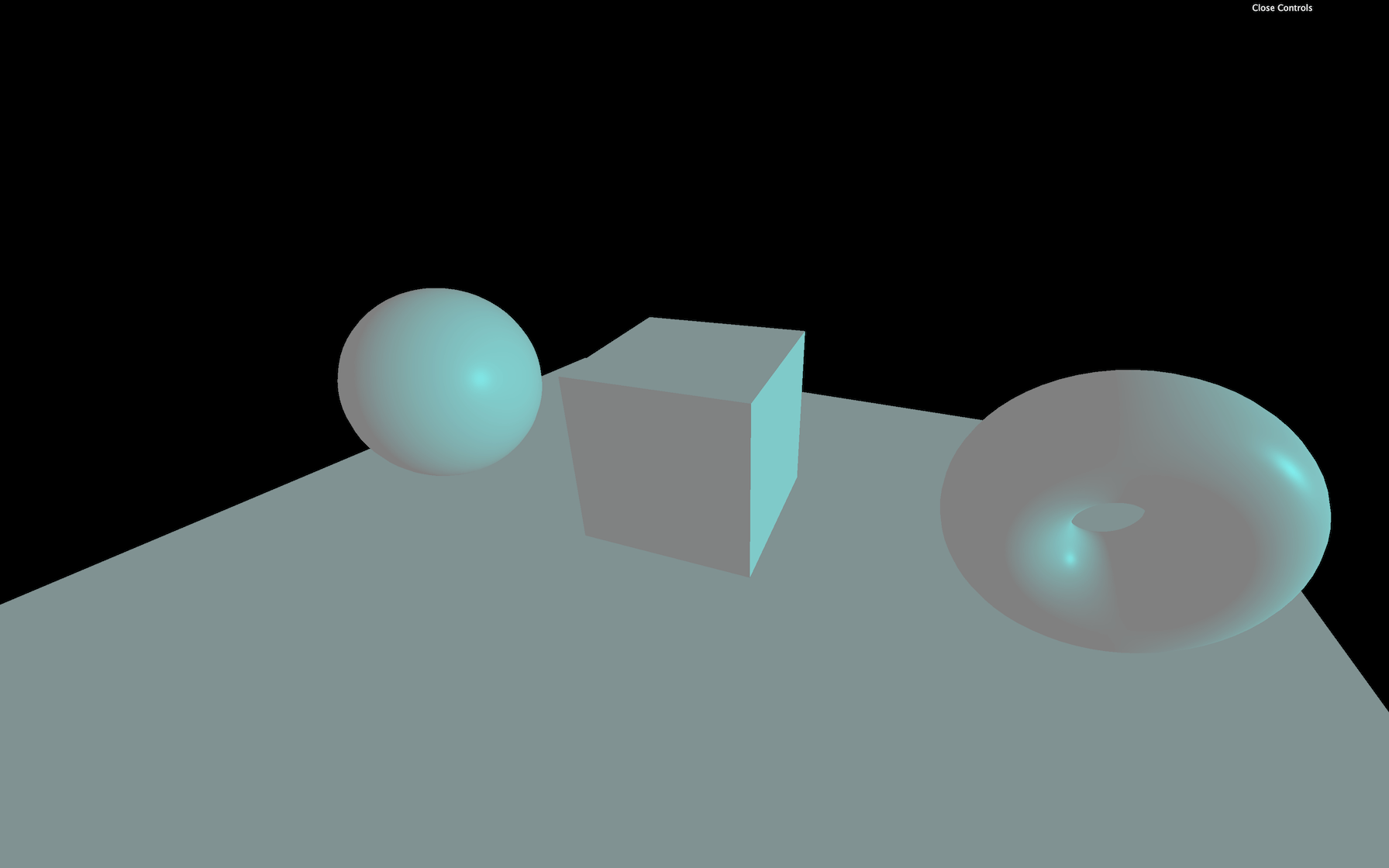
- 태양 빛과 같은 light, 수직으로 빛을 발광하는 효과
- Directional Light 객체의 첫번째 paramter는 color
- 두번째 parameter는 intensity
- Directional Light는 position을 변경할 수 있다.
- 거리는 상관 없고 각도만 신경쓰면 된다. 왜냐하면 Directional light의 거리는 infinite이다.
const directionalLight = new THREE.DirectionalLight(0x00fffc, 0.9)
scene.add(directionalLight)
directionalLight.position.set(1, 0.25, 0)
언제 사용하는가
- 위에서 내리쬐는 빛을 구현할 때
- 태양과 같은 빛을 구현 할 때
Hemisphere Light
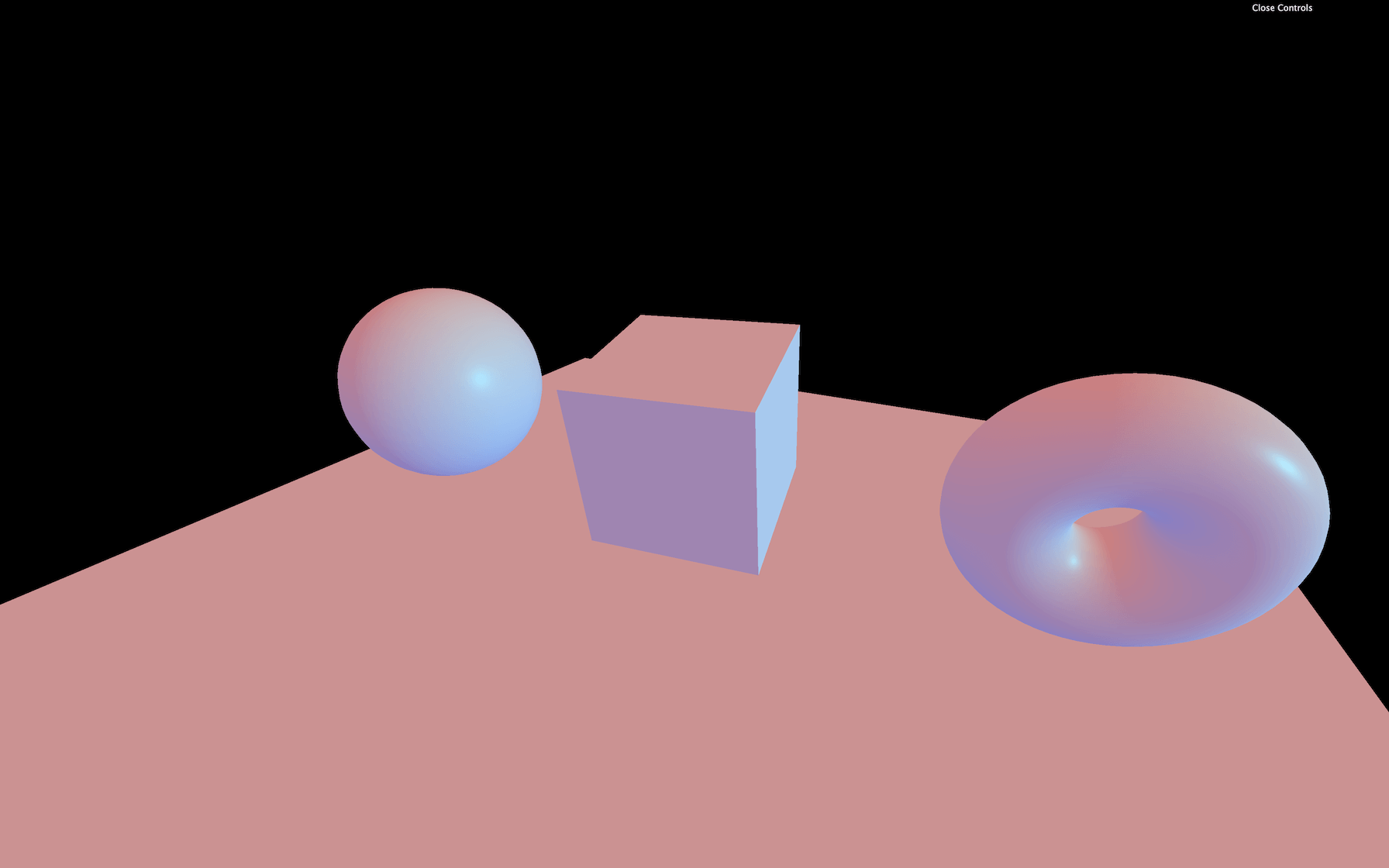
- Ambient Light는 전방휘적인 빛이라면 Hemisphere Light는 위, 아래에서 다른 색상으로 표현이 가능한 빛
- Hemisphere Light 객체의 첫번째 paramter는 sky color
- 두번째 parameter는 ground color
- 세번째 parameter는 intensity
const hemisphereLight = new THREE.HemisphereLight(0xff0000, 0x0000ff, 0.9)
scene.add(hemisphereLight)
언제 사용하는가
- 그림자 및 극적인 효과를 연출할 때
- 연하게 사용해서 자연스러운 그림자
Point Light
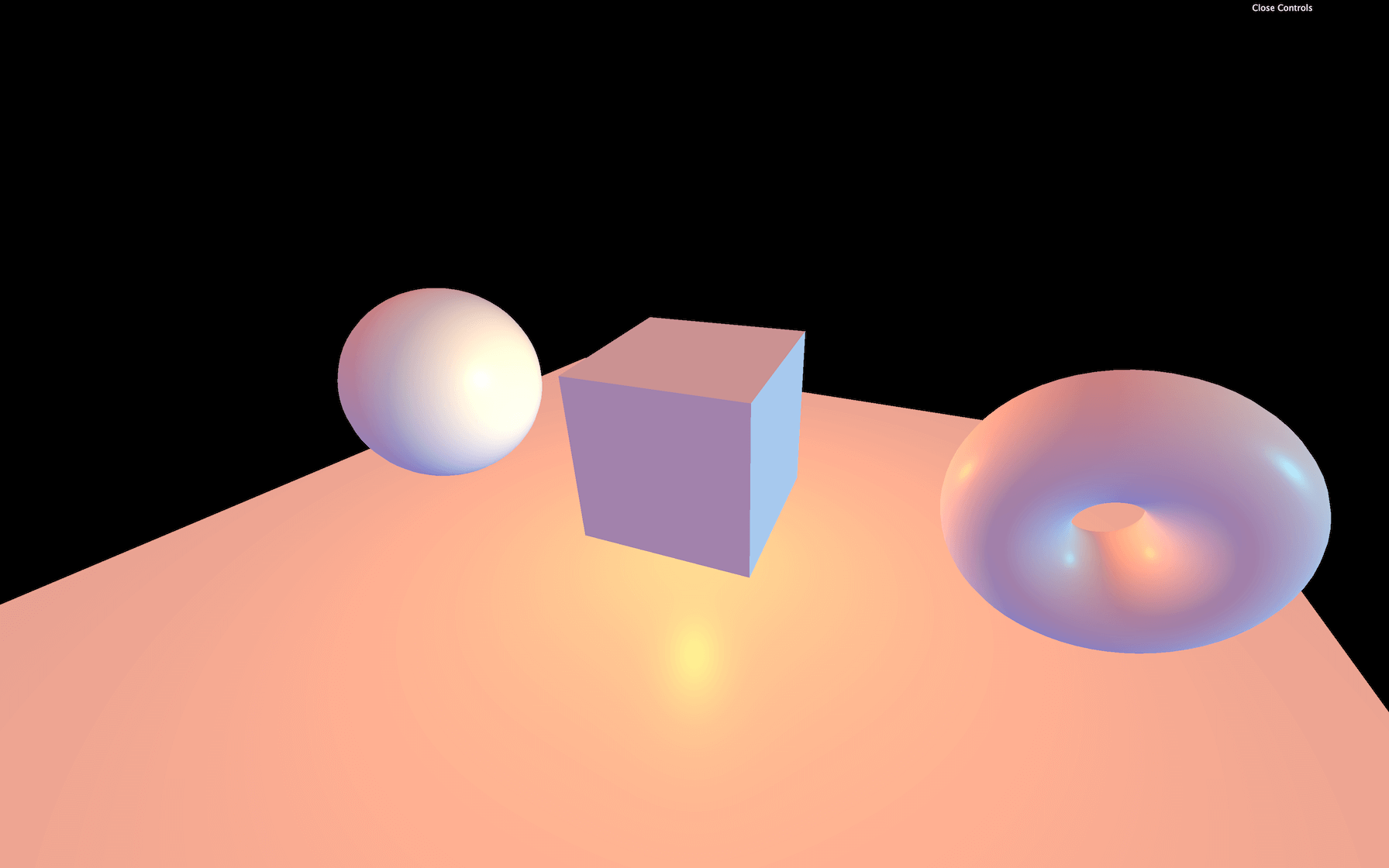
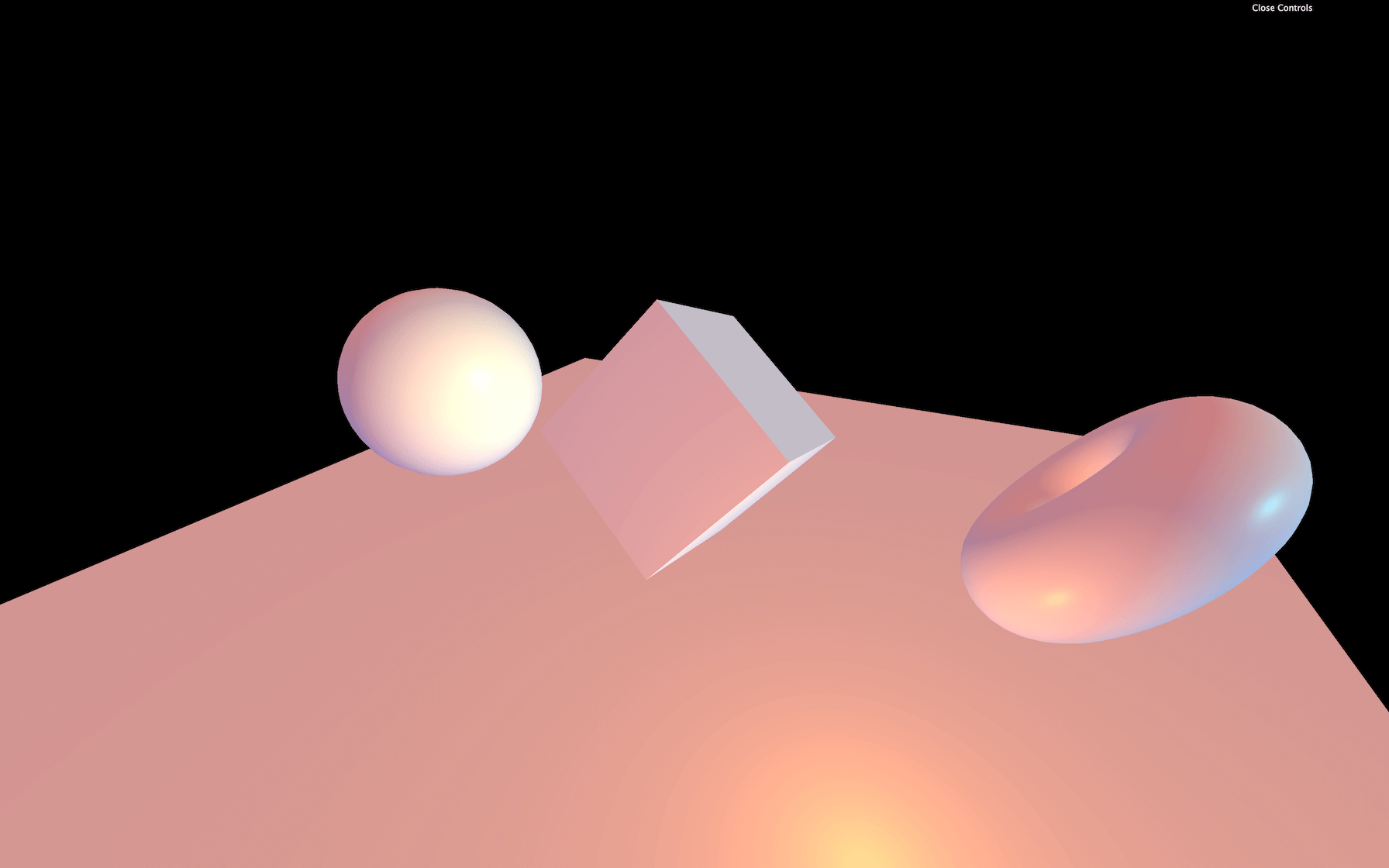
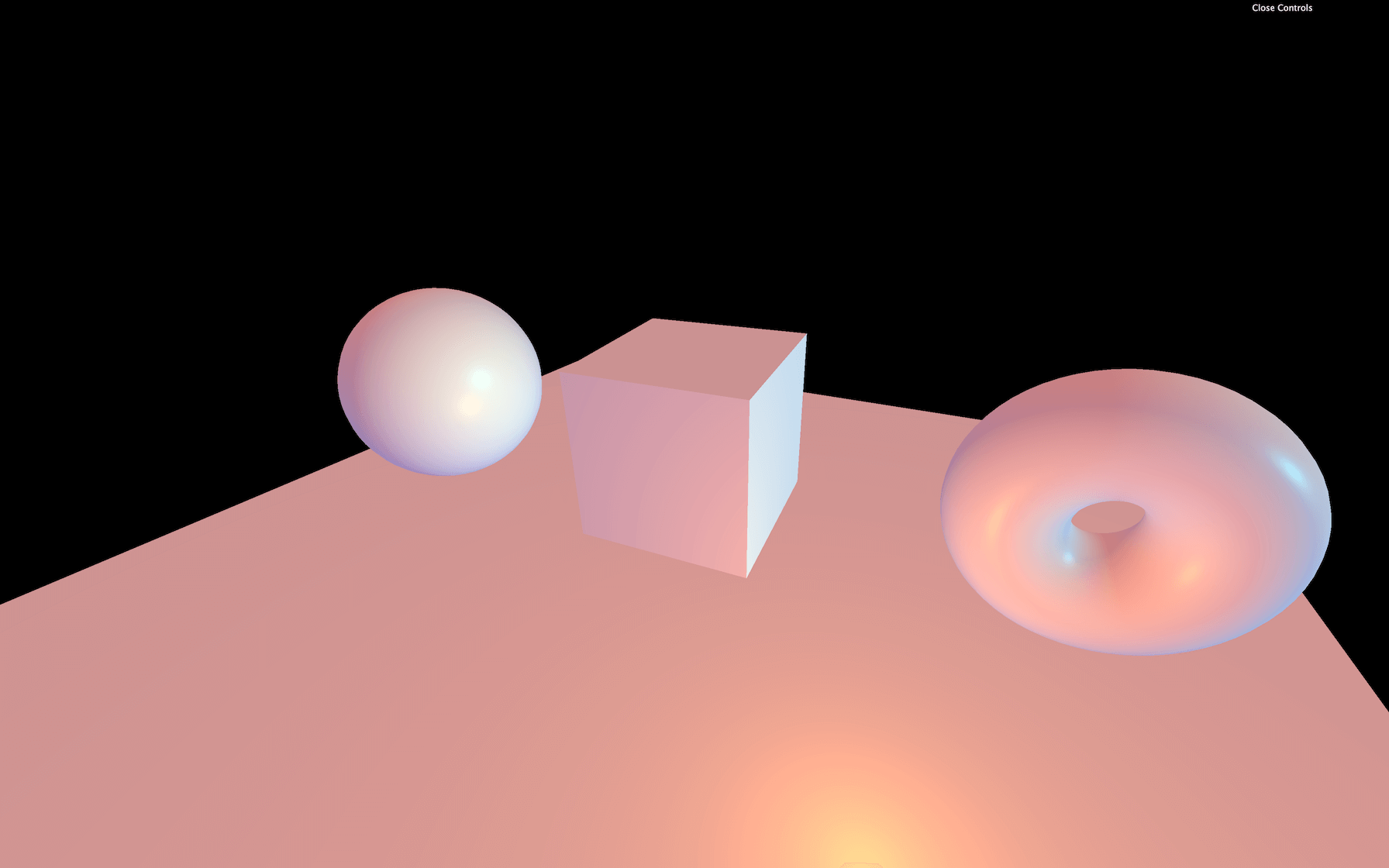
- lighter 형식으로 빛을 발광하는 light
- 모든 방향으로 일정하게 빛을 발광한다
- Point Light 객체의 첫번째 paramter는 color
- 두번째 parameter는 intensity
- Point Light의 위치를 변경할 수 있다.
- default로는 Point light는 거리에 따라서 fade되지 않는다.
- 그러나 fade distance와 decay 값을 활용하면 fade 된다.
- 세번째 parameter는 distance
- 네번째 parameter는 decay
const pointLight = new THREE.PointLight(0xff9000, 1.5)
scene.add(pointLight)
pointLight.position.set(1, - 0.5, 1)
const pointLight = new THREE.PointLight(0xff9000, 1.5, 10, 2)
언제 사용하는가
RectArea Light
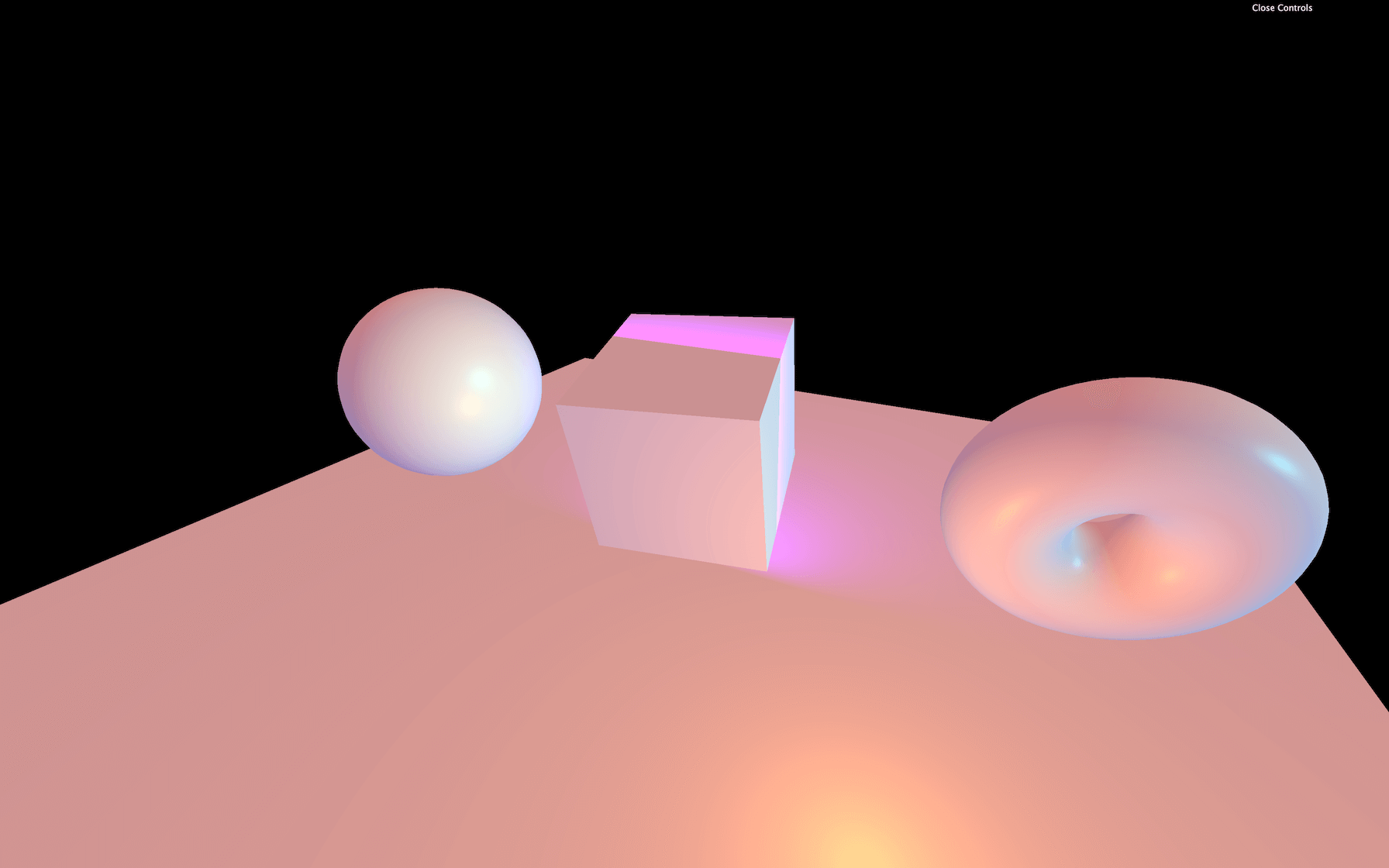
- 커다란 사각형의 light
- 사진 촬영장 빛과 같다
- RectArea Light 객체의 첫번째 paramter는 color
- 두번째 parameter는 intensity
- 세번째 parameter는 width
- 네번째 parameter는 height
- ReactArea Light는 MeshStandard Material과 MeshPhysicalMaterial에만 작용한다.
- 위치와 방향을 바꿀 수 있어서 lookAt 함수를 활용할 수 있다.
const rectAreaLight = new THREE.RectAreaLight(0x4e00ff, 6, 1, 1)
scene.add(rectAreaLight)
rectAreaLight.position.set(- 1.5, 0, 1.5)
rectAreaLight.lookAt(new THREE.Vector3())
언제 사용하는가
Spot Light
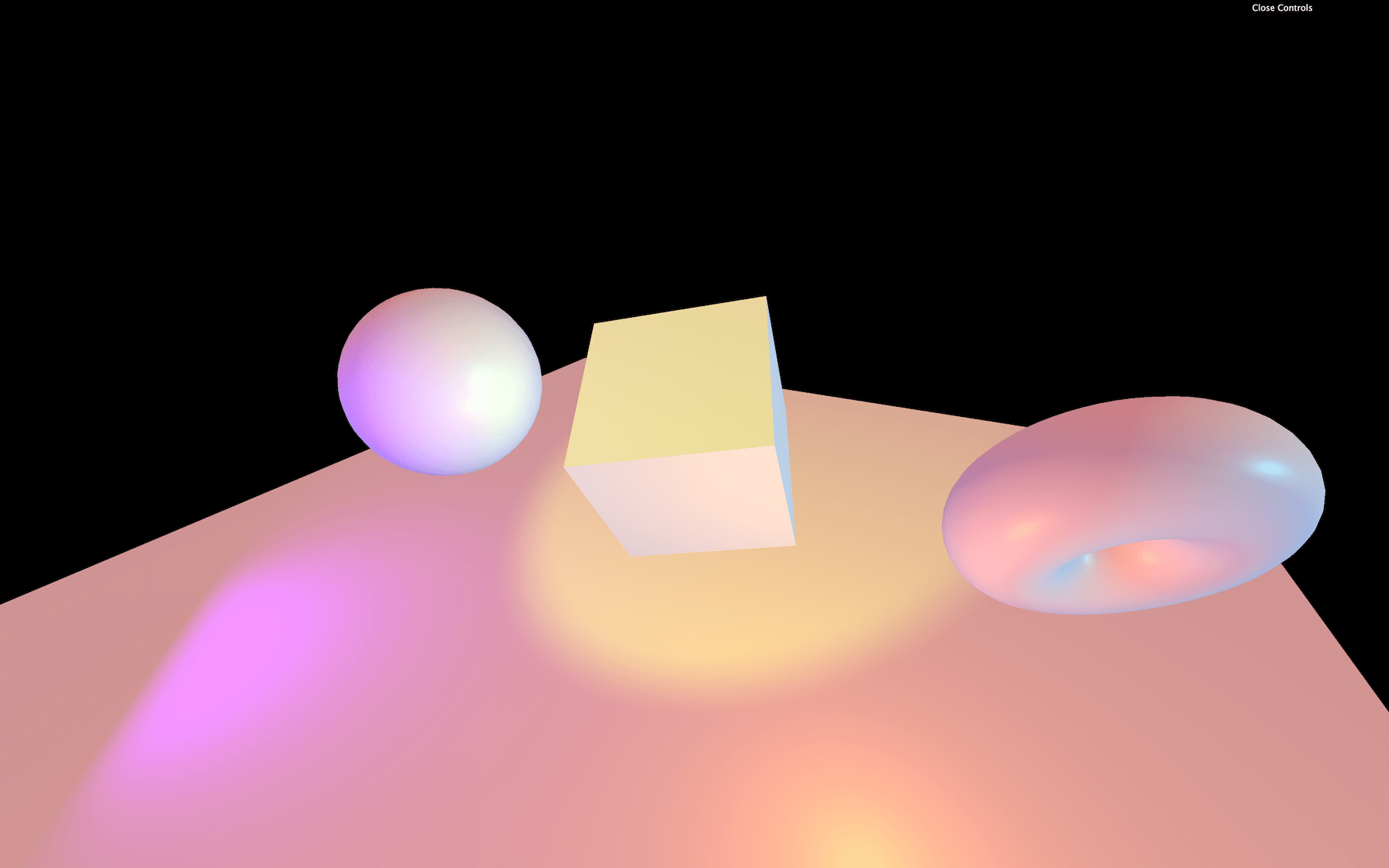
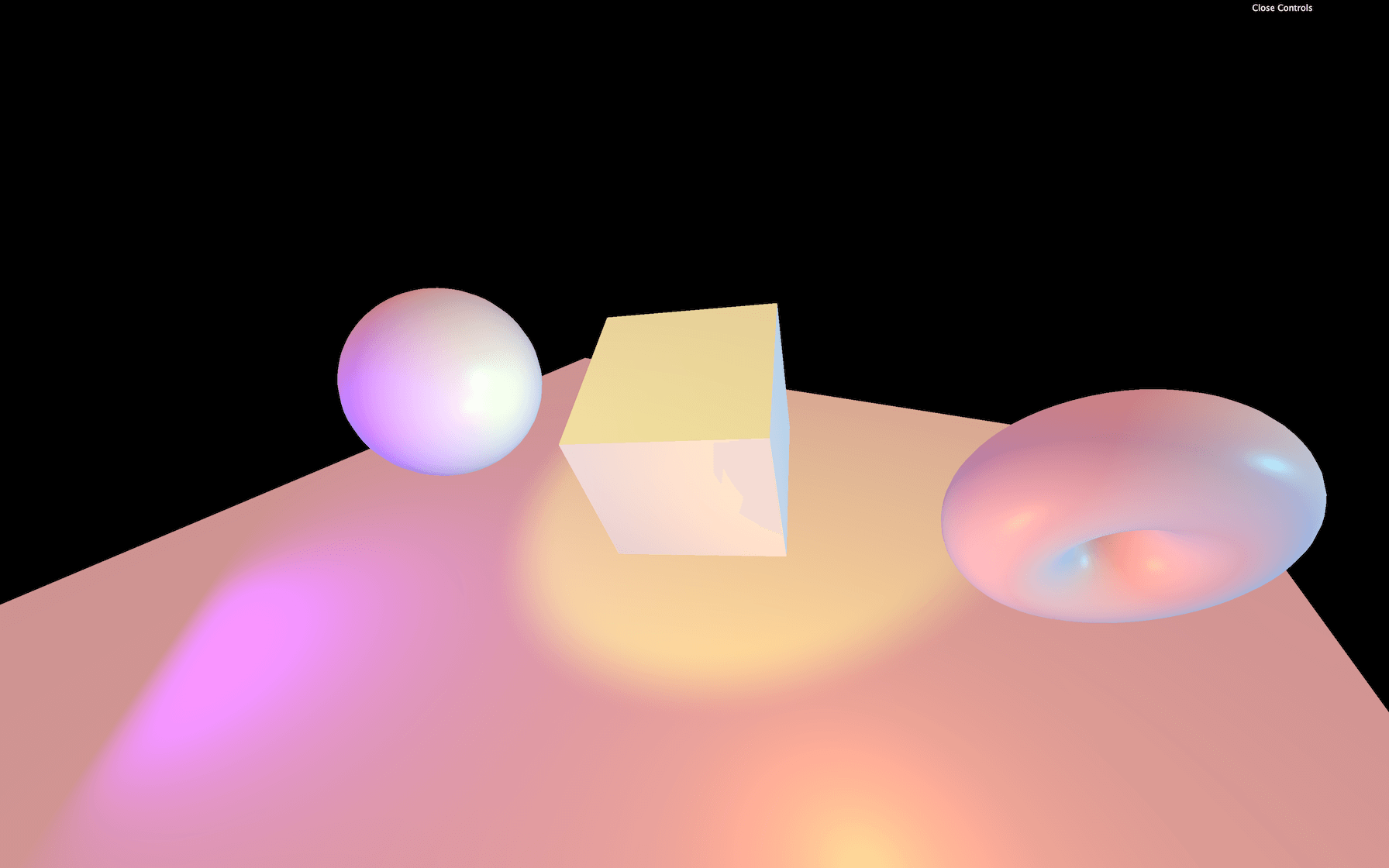
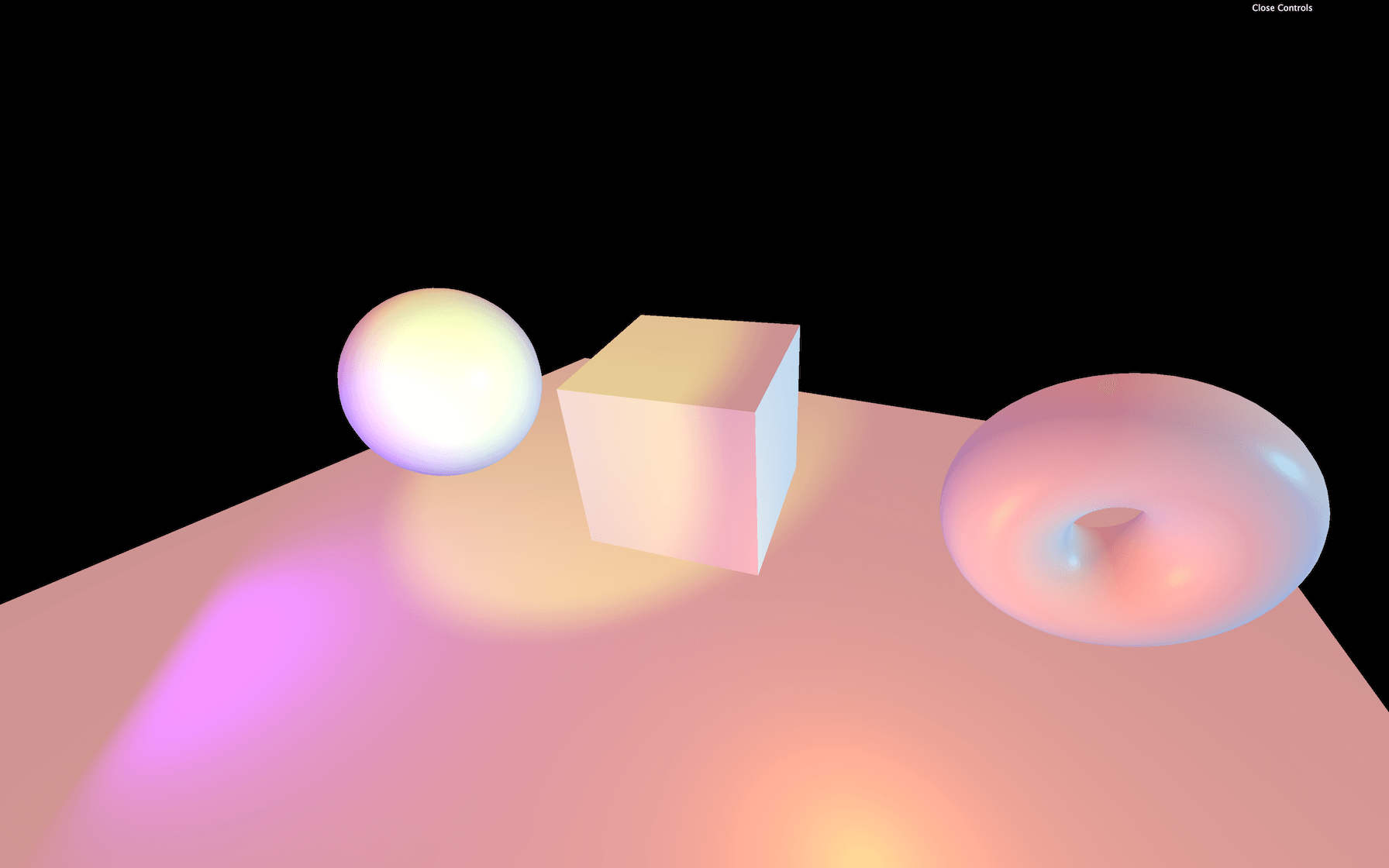
- Spot Light는 flashlight와 같다.
- cone 형식으로 빛을 발광한다.
- Spotlight의 position을 변경하려면 target(Object3D)을 변경해야한다.
- 변경하려면 target 위치를 변경하고, target을 scene에 추가해준다.
parameter
- color
- intensity
- distance
- angle
- penumbra - 반그림자
- decay
const spotLight = new THREE.SpotLight(0x78ff00, 4.5, 10, Math.PI * 0.1, 0.25, 1)
spotLight.position.set(0, 2, 3)
scene.add(spotLight)
spotLight.target.position.x = - 0.75
scene.add(spotLight.target)
- 기본적으로 Light Object는 performance cost가 높다
low cost
- AmbientLight
- HemisphereLight
mid cost
- DirectionalLight
- PointLight
high cost
Baking
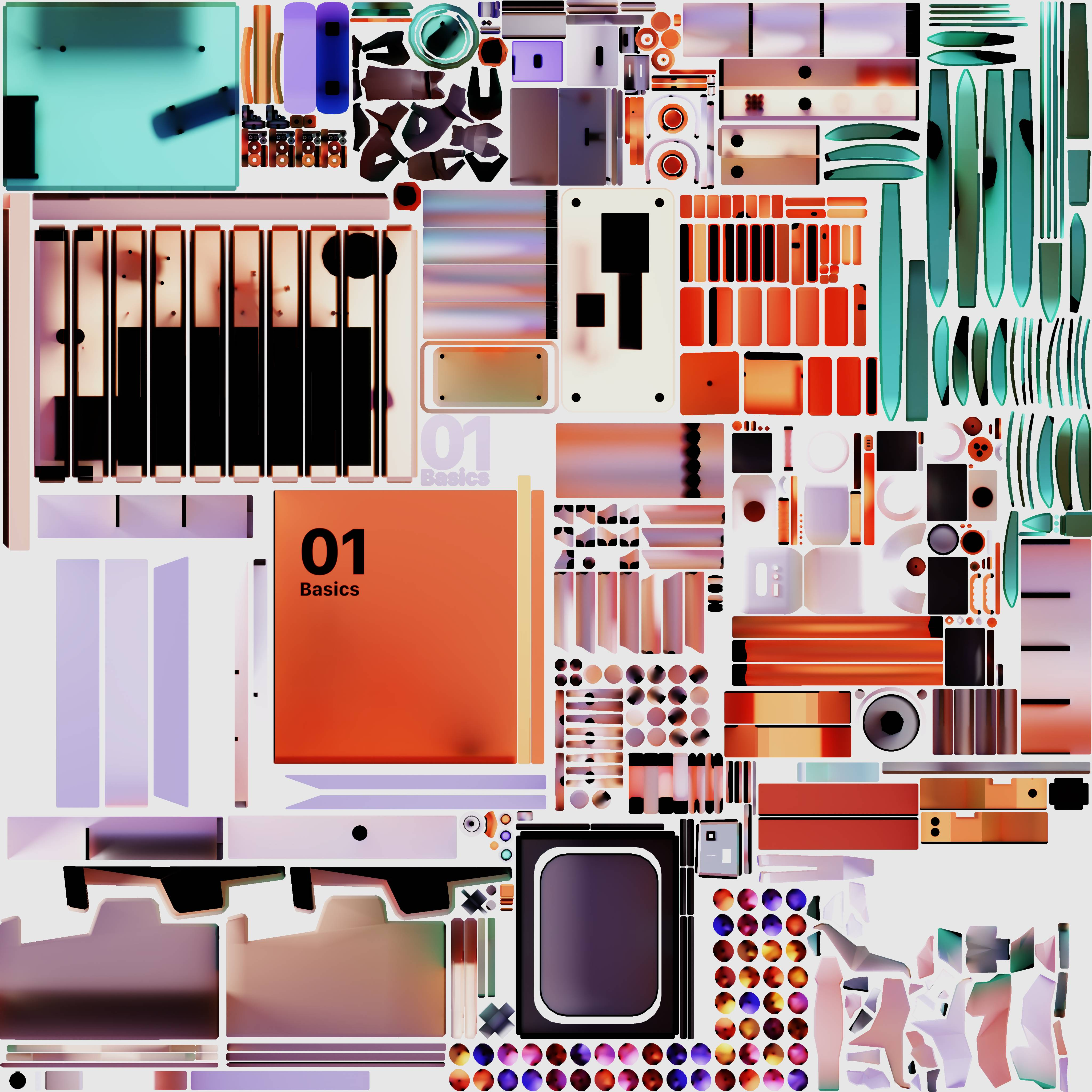
- 위에 cost 때문에 보통은 Baking을 사용한다.
Helper
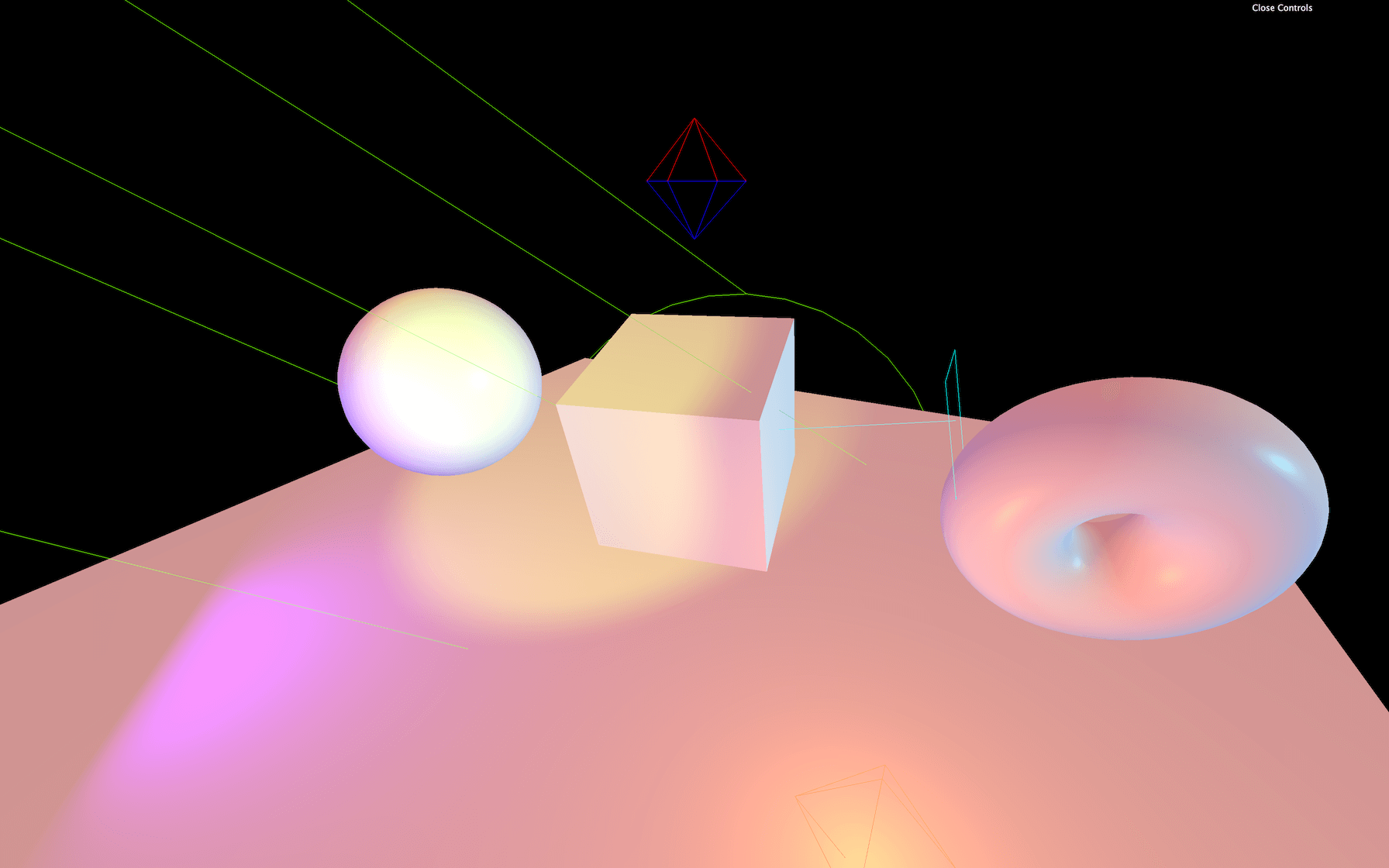
- Light Object는 보이지 않기 때문에 배치하기 어렵다
- 이를 위해서 Helper를 활용해서 배치한다.
- RectAreaLightHelper 는 import해서 사용해야한다.
const hemisphereLightHelper = new THREE.HemisphereLightHelper(hemisphereLight, 0.2)
scene.add(hemisphereLightHelper)
const directionalLightHelper = new THREE.DirectionalLightHelper(directionalLight, 0.2)
scene.add(directionalLightHelper)
const pointLightHelper = new THREE.PointLightHelper(pointLight, 0.2)
scene.add(pointLightHelper)
const spotLightHelper = new THREE.SpotLightHelper(spotLight)
scene.add(spotLightHelper)
import { RectAreaLightHelper } from 'three/examples/jsm/helpers/RectAreaLightHelper.js'
const rectAreaLightHelper = new RectAreaLightHelper(rectAreaLight)
scene.add(rectAreaLightHelper)