서로소 집합
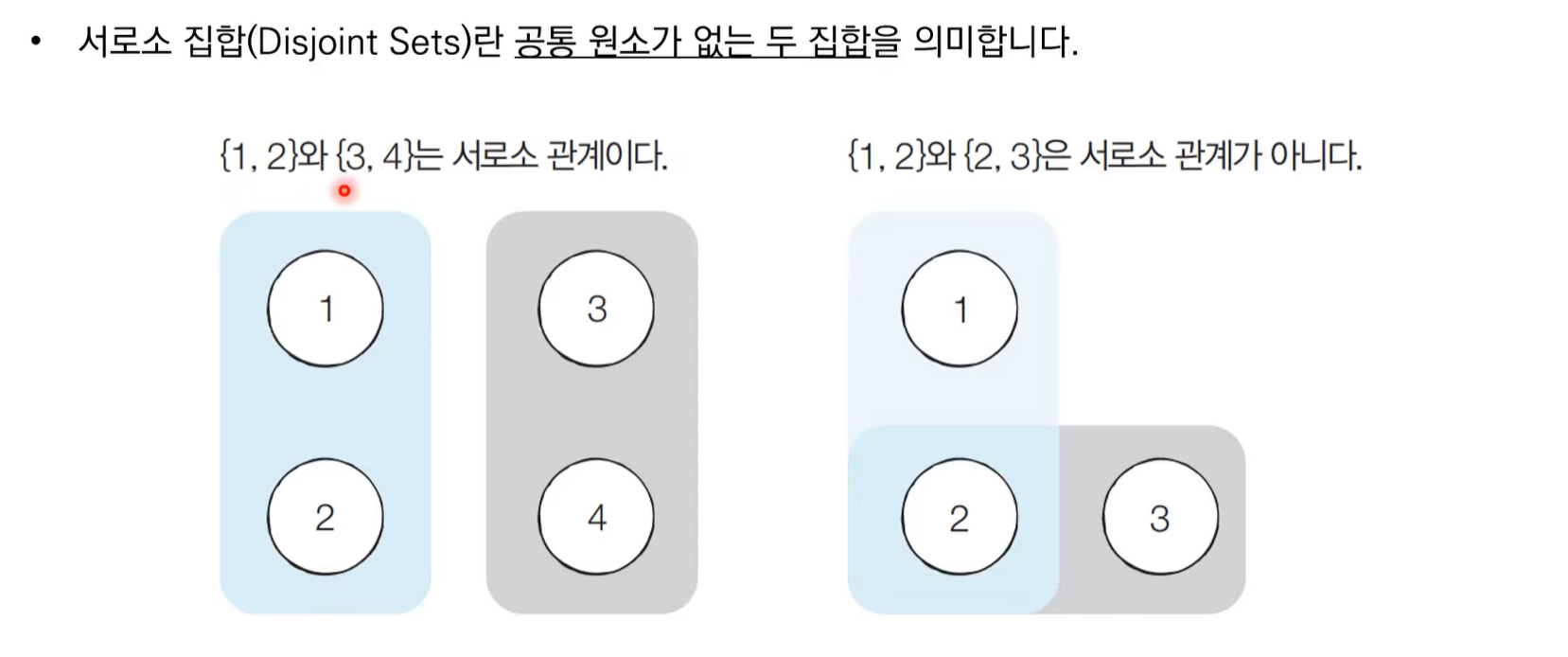
서로소 집합 자료구조
- 서로소 부분 집합들로 나누어진 원소들의 데이터를 처리하기 위한 자료구조
- 두 종류의 연산 지원
- 합집합(Union): 두 개의 원소가 포함된 집합을 하나의 집합으로 합침
- 찾기(Find): 특정한 원소가 속한 집합이 어떤 집합인지 알려주는 연산
- 합집합 찾기(Union Find) 자료구조라고 부르기도 함
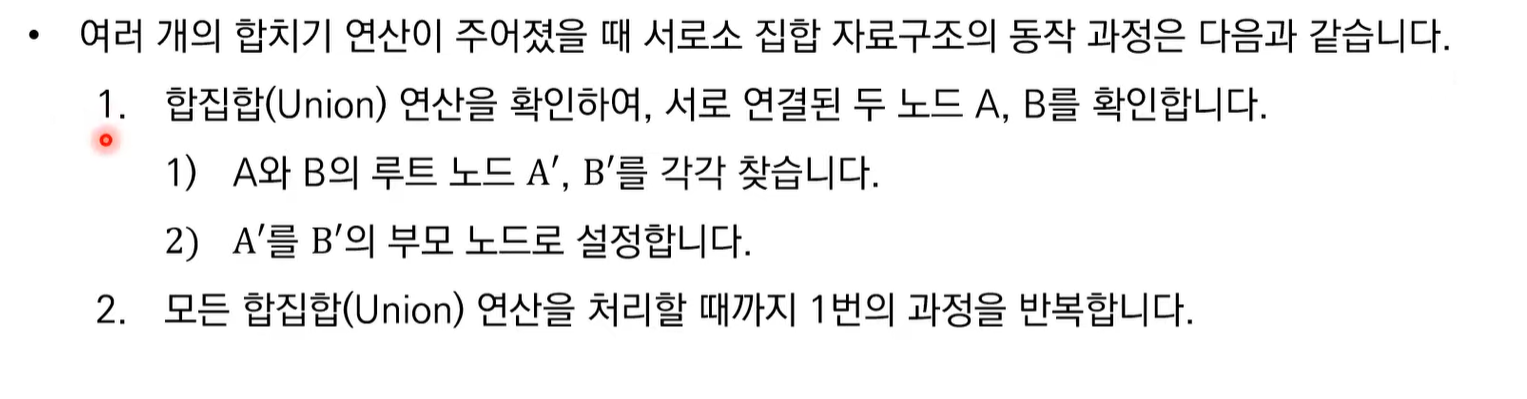
서로소 집합 자료구조: 동작 과정 살펴보기
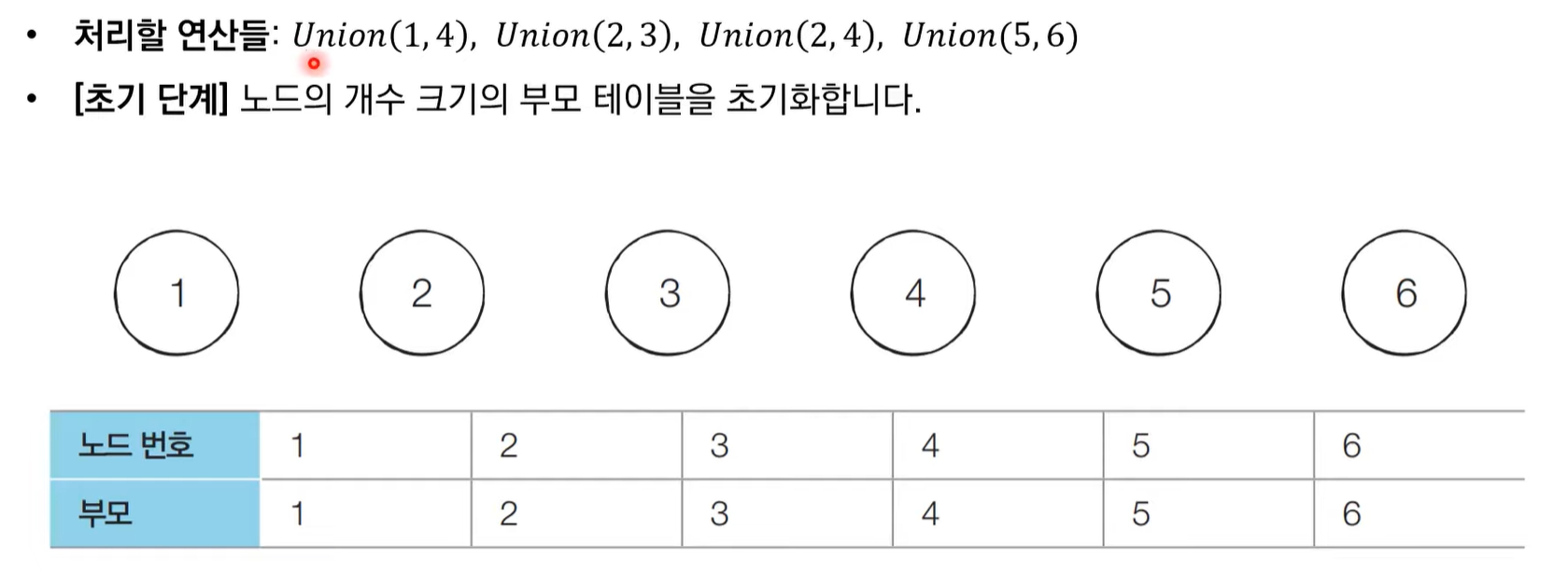
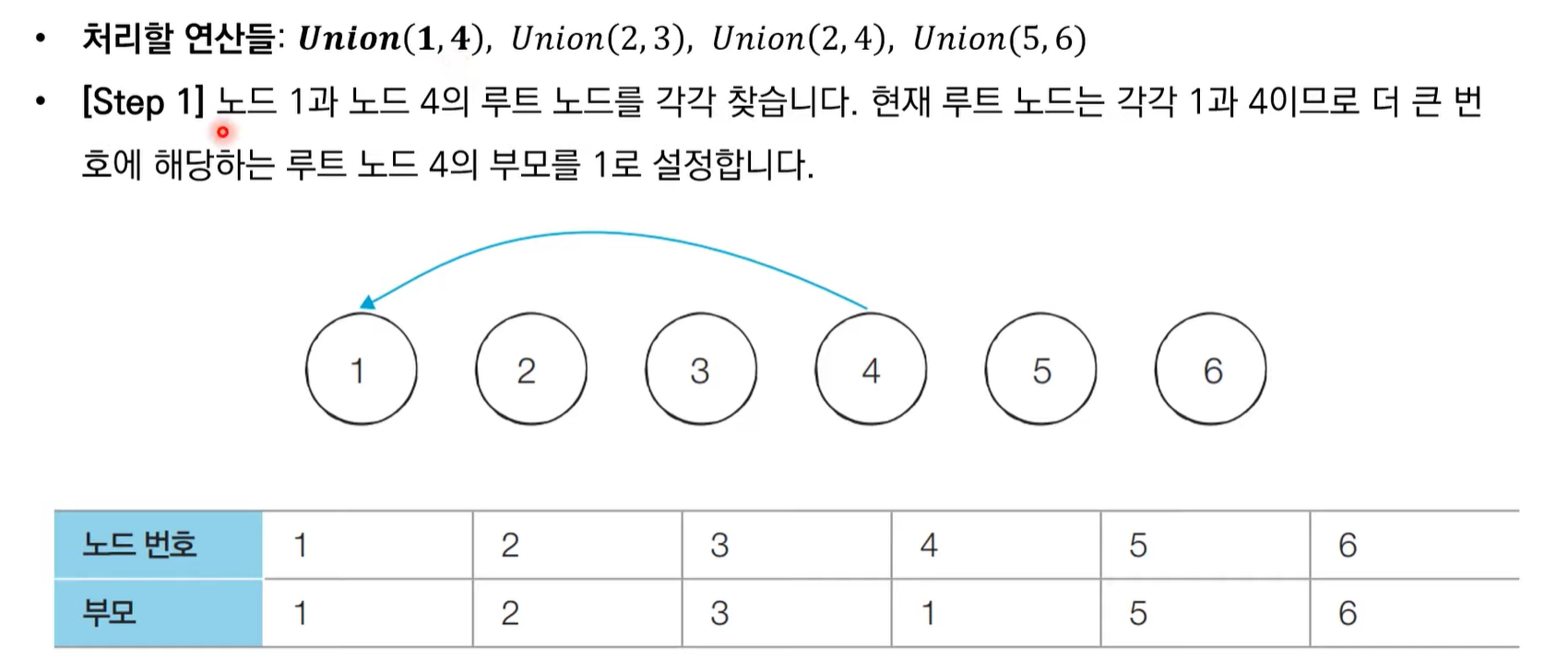
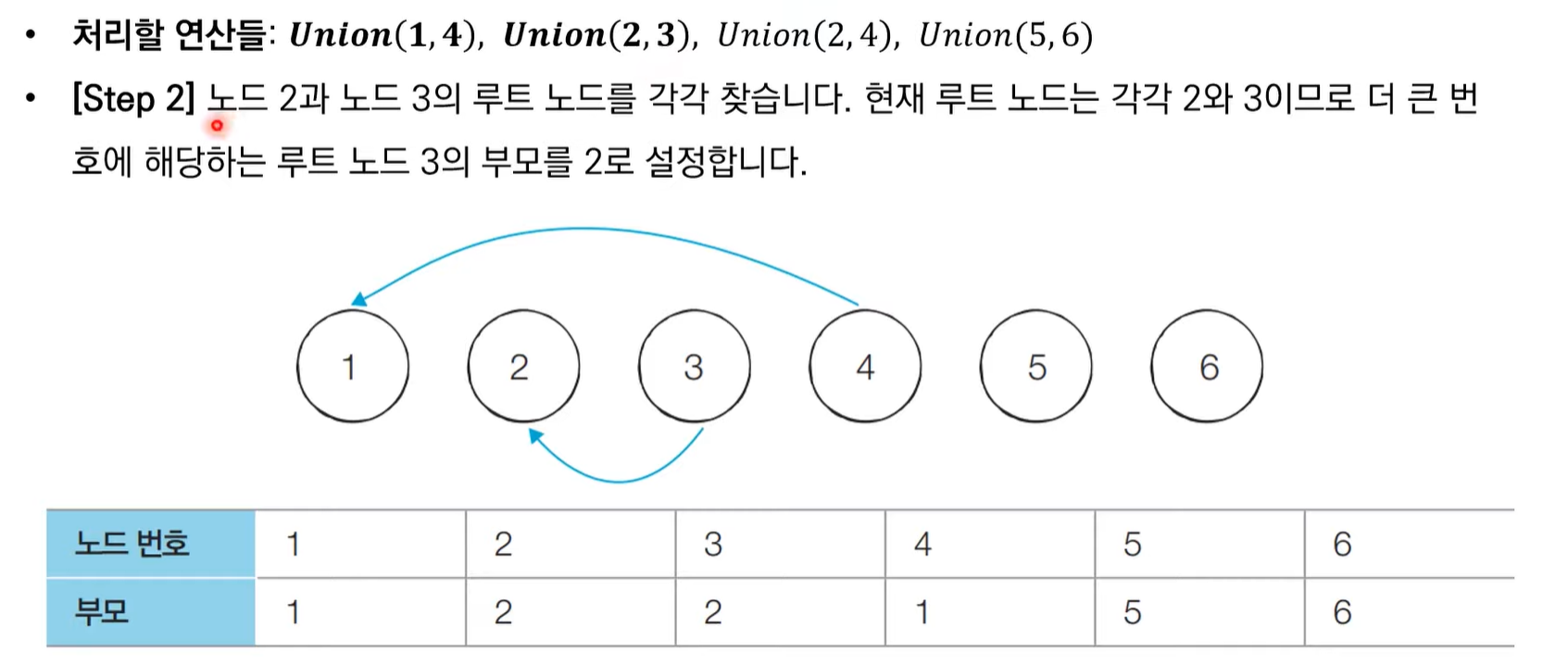
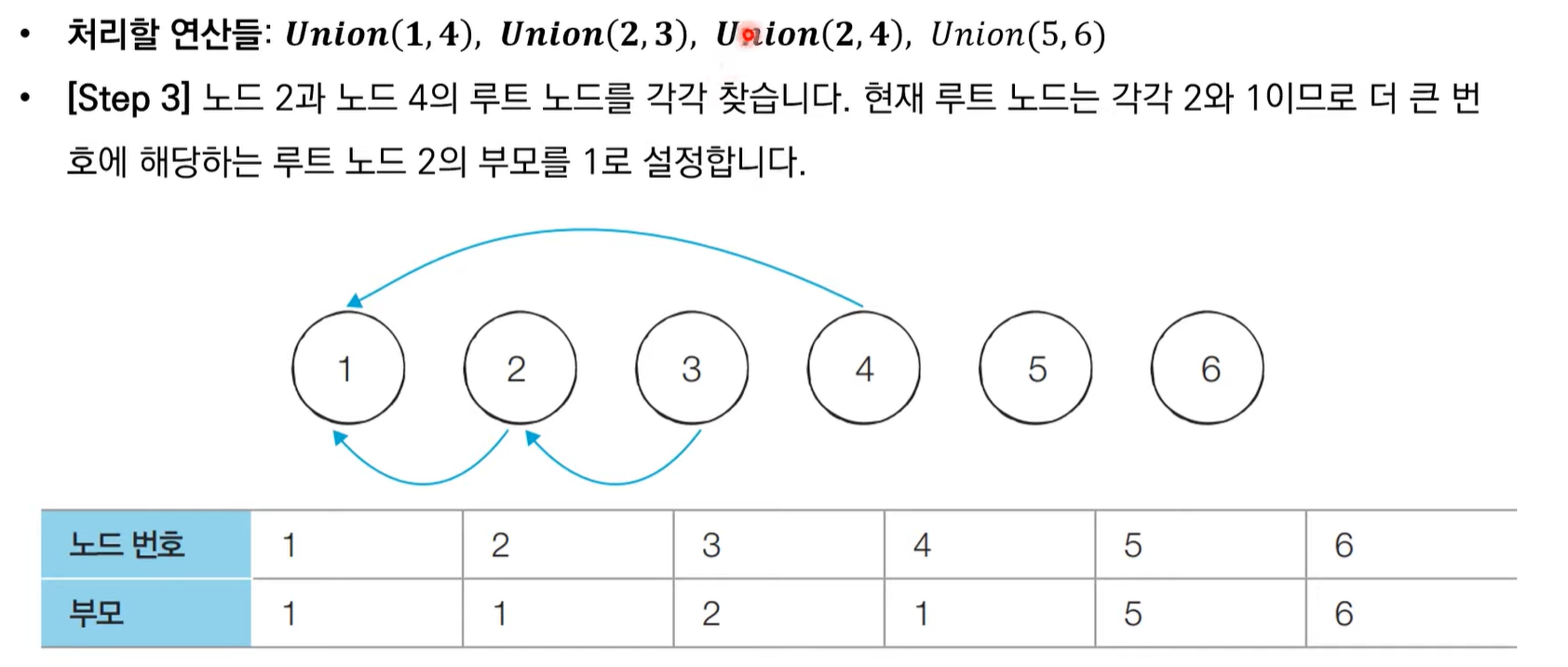
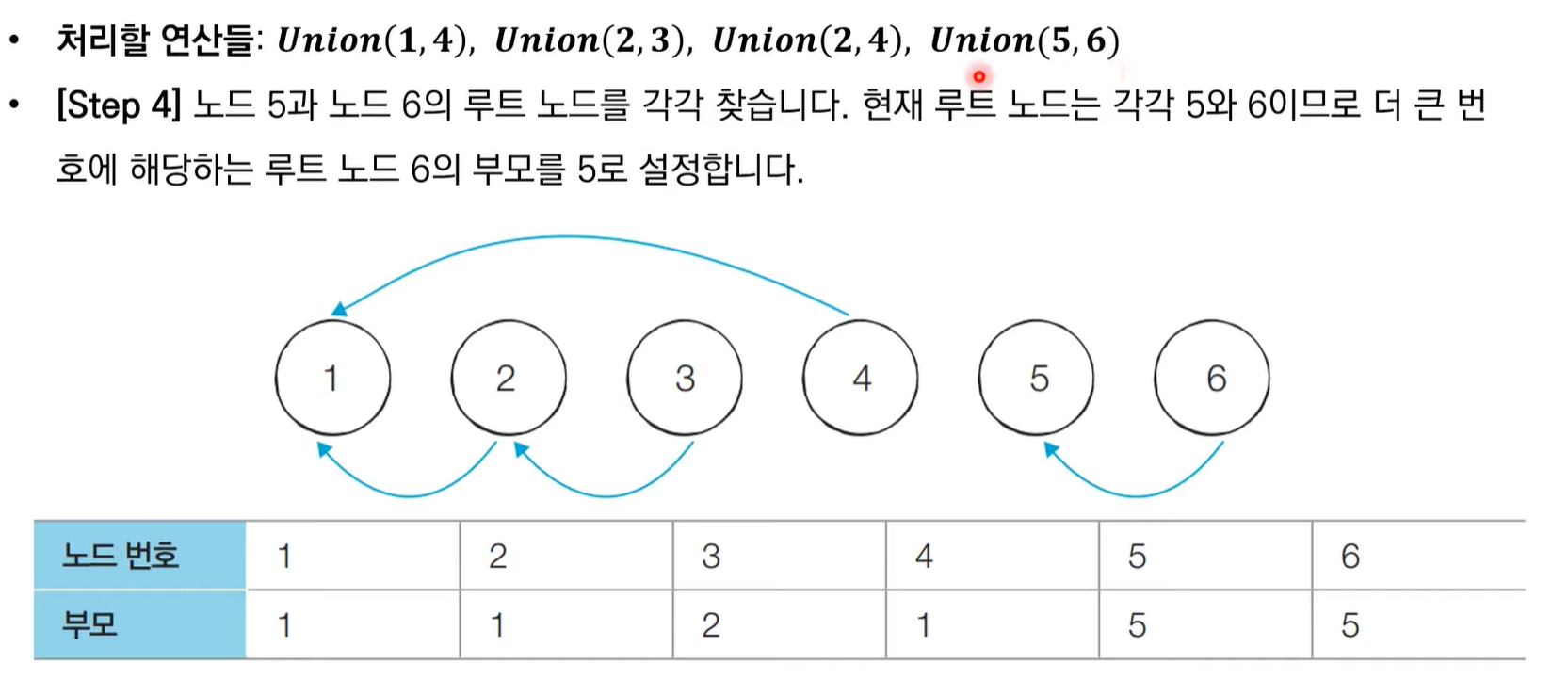
서로소 자료구조:연결성
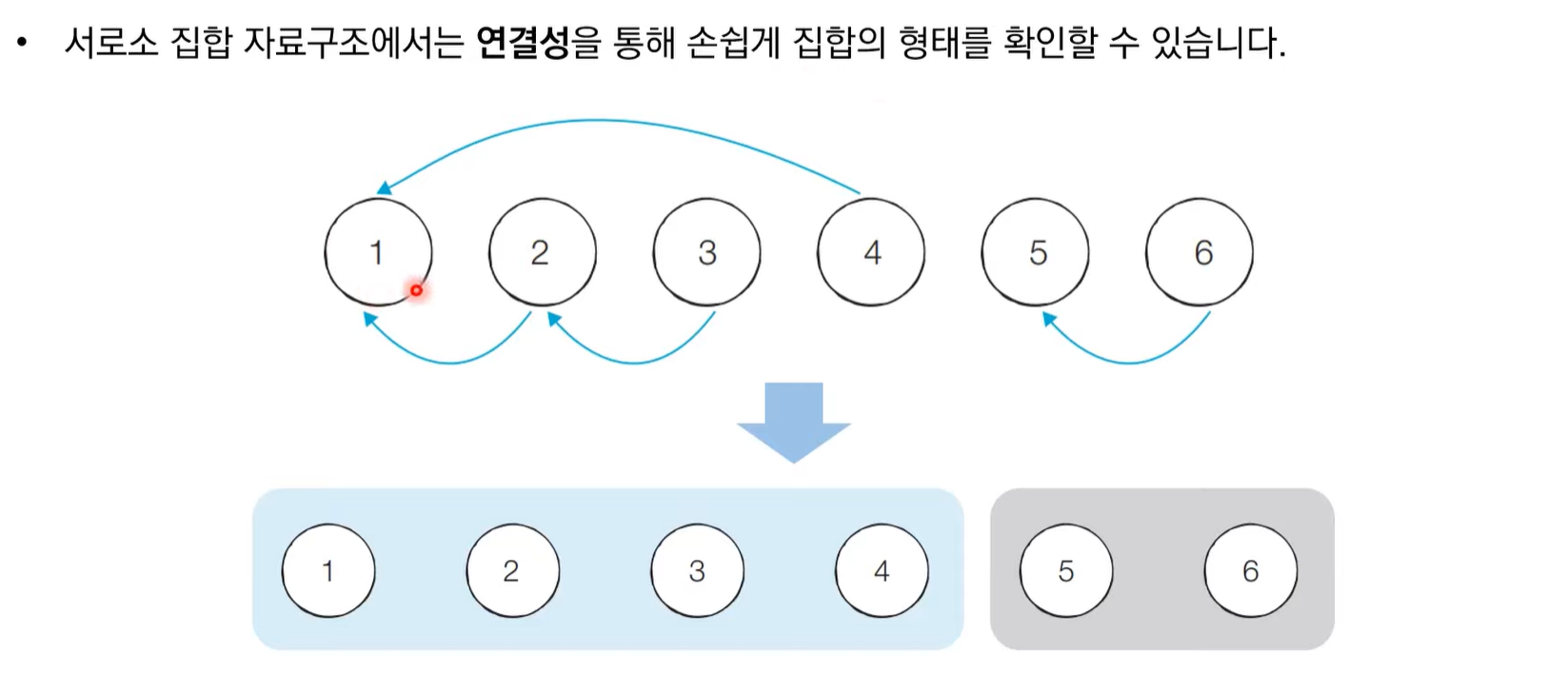
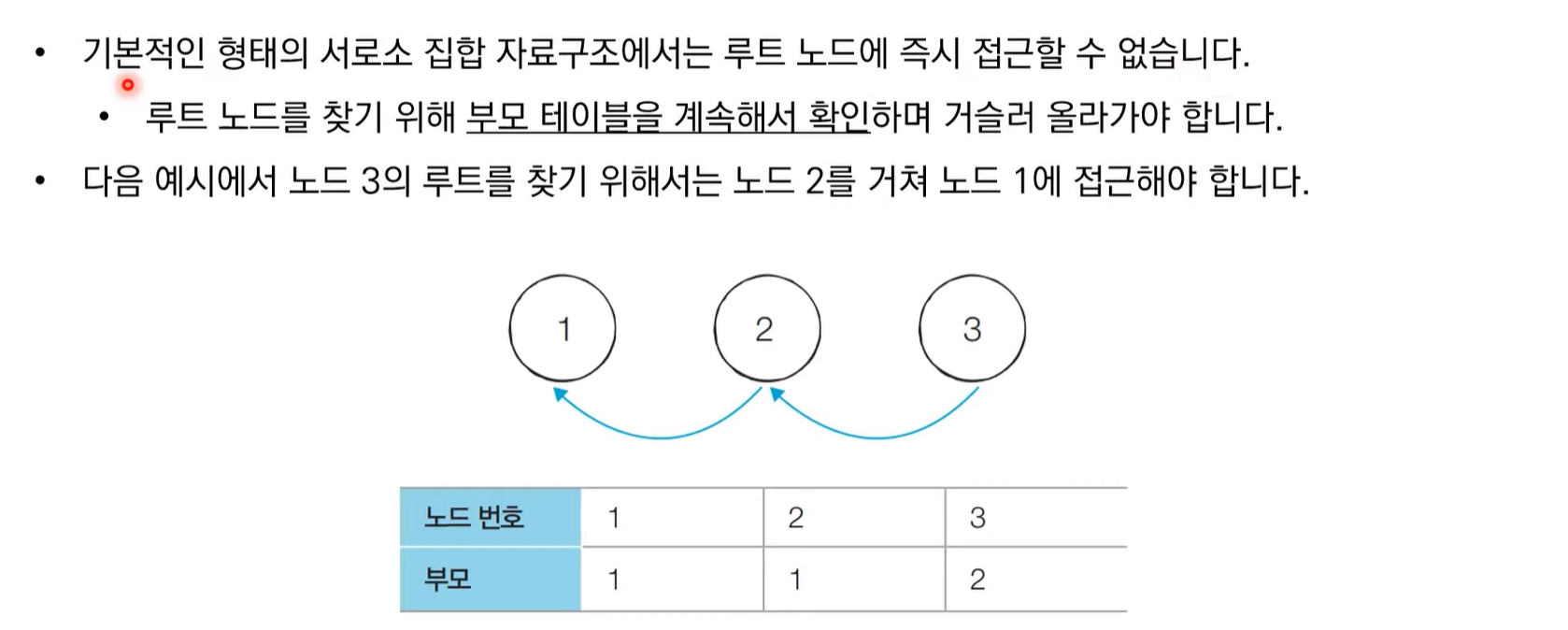
기본적인 구현 방법
def find_parent(parent,x):
if parent[x] != x:
return find_parent(parent,parent[x])
return x
def union_parent(parent,a,b):
a = find_parent(parent,a)
b = find_parent(parent,b)
if a < b:
parent[b] = a
else:
parent[a] = b
v,e = map(int,input().split())
parent = [0] * (v+1)
for i in range(1,v+1):
parent[i] = i
for i in range(e):
a,b = map(int,input().split())
union_parent(parent,a,b)
print('각 원소가 속한 집합: ',end = " ")
for i in range(1,v+1):
print(find_parent(parent,i), end = " ")
print()
print('부모 테이블: ',end = " ")
for i in range(1,v+1):
print(parent[i],end = " ")
기본적인 구현 방법의 문제점
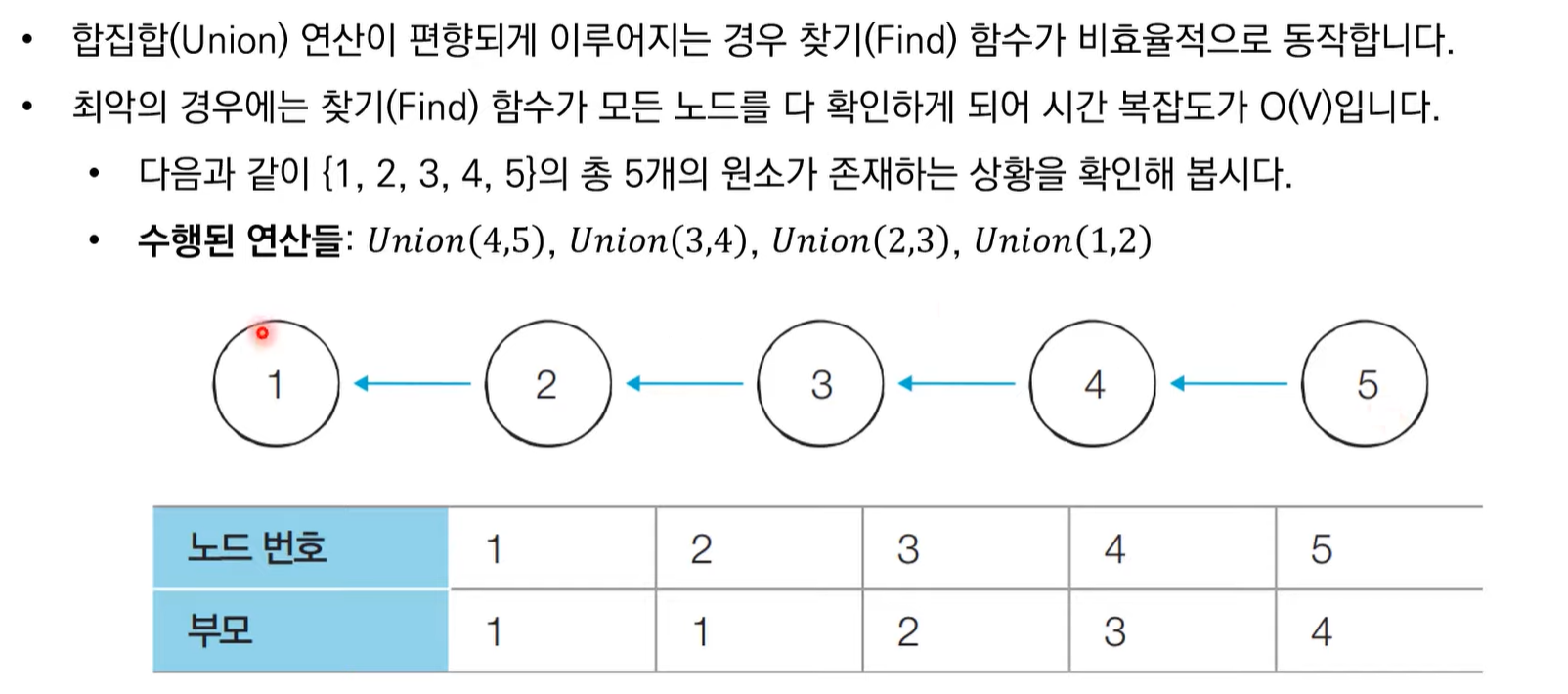
경로 압축(Path Compression)
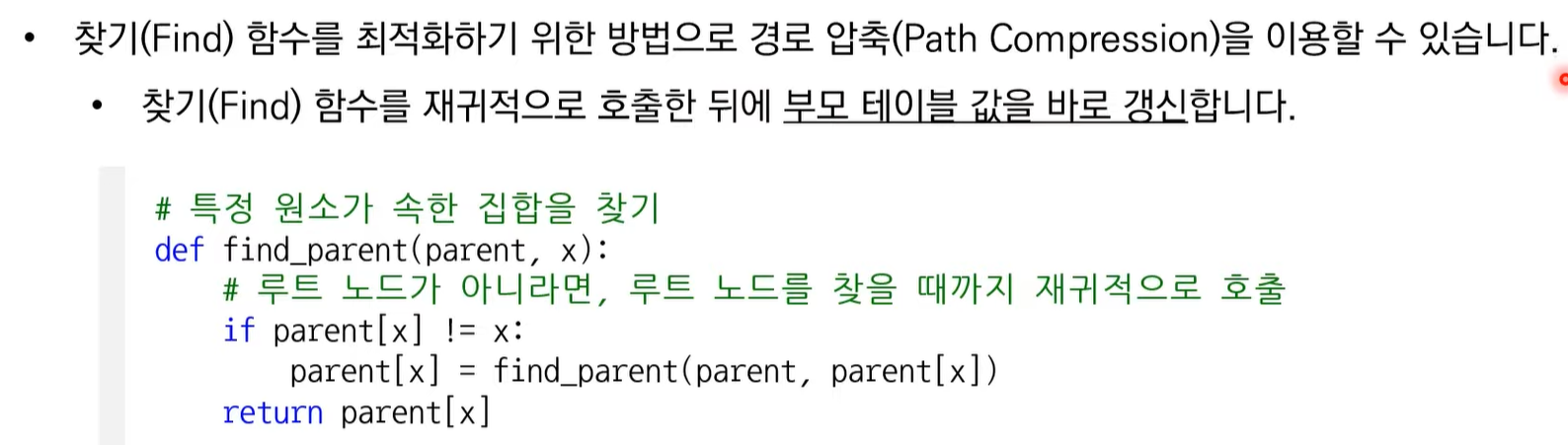
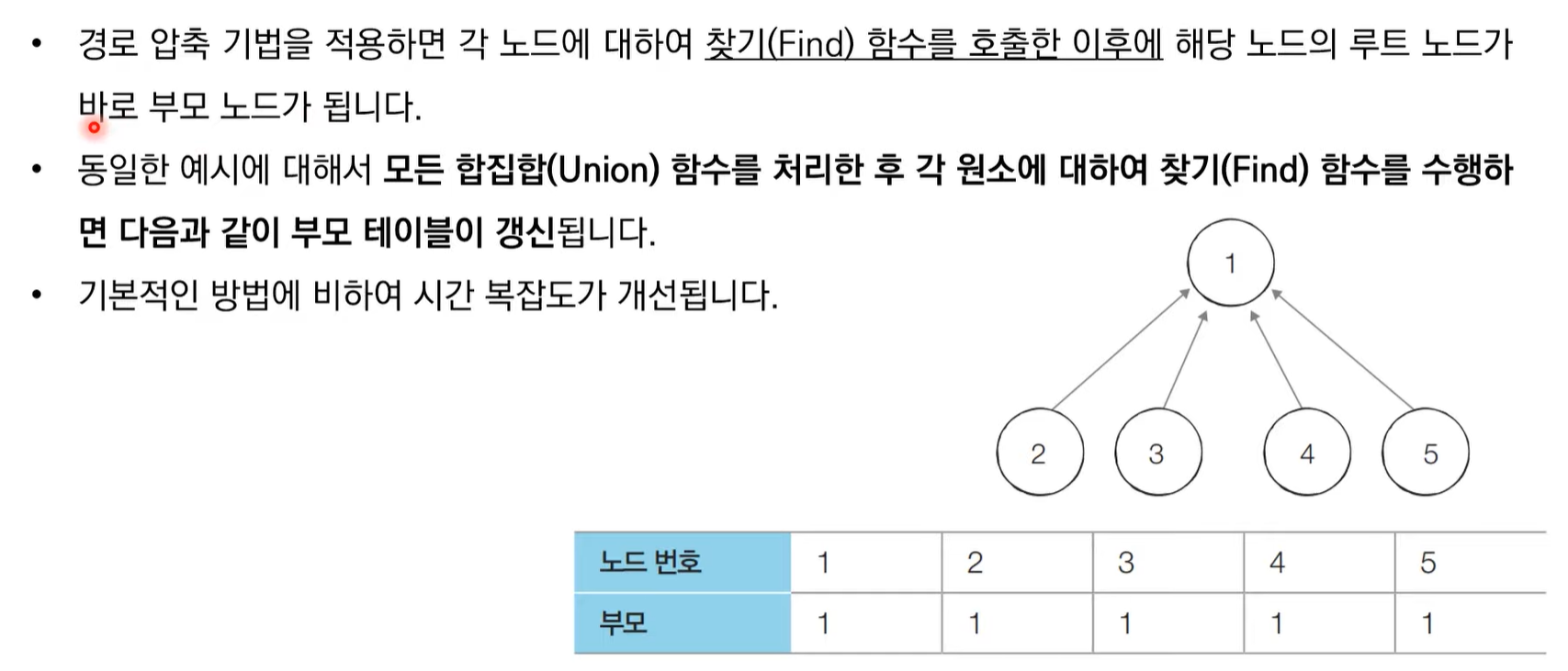
경로 압축 구현
def find_parent(parent,x):
if parent[x] != x:
parent = find_parent(parent,parent[x])
return parent[x]
def union_parent(parent,a,b):
a = find_parent(parent,a)
b = find_parent(parent,b)
if a < b:
parent[b] = a
else:
parent[a] = b
v,e = map(int,input().split())
parent = [0] * (v+1)
for i in range(1,v+1):
parent[i] = i
for i in range(e):
a,b = map(int,input().split())
union_parent(parent,a,b)
print('각 원소가 속한 집합: ',end = " ")
for i in range(1,v+1):
print(find_parent(parent,i), end = " ")
print()
print('부모 테이블: ',end = " ")
for i in range(1,v+1):
print(parent[i],end = " ")
사이클 판별
- 서로서 집합은 무방향 그래프 내에서의 사이클을 판별할 때 사용 가능
※ 방향 그래프에서의 사이클 여부는 DFS를 이용하여 판별 가능
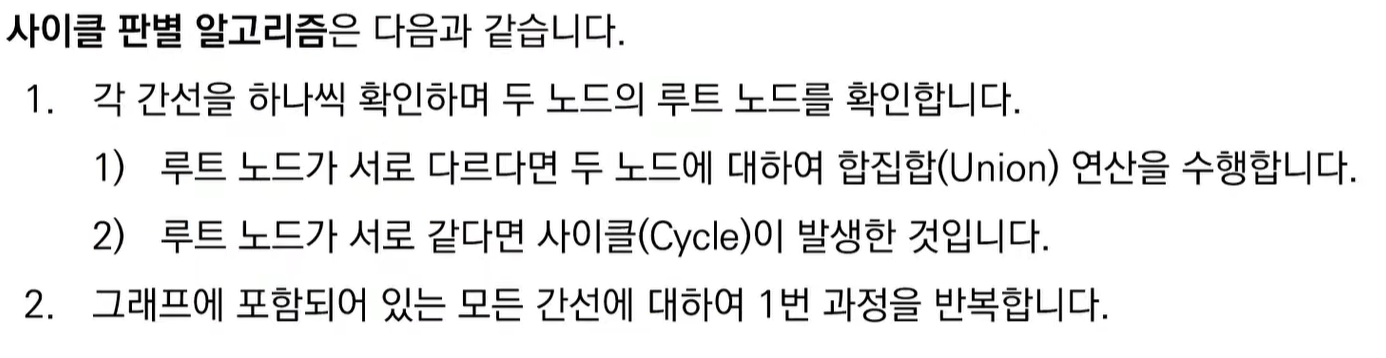
서로소 집합을 활용한 사이클 판별: 동작 과정 살펴보기
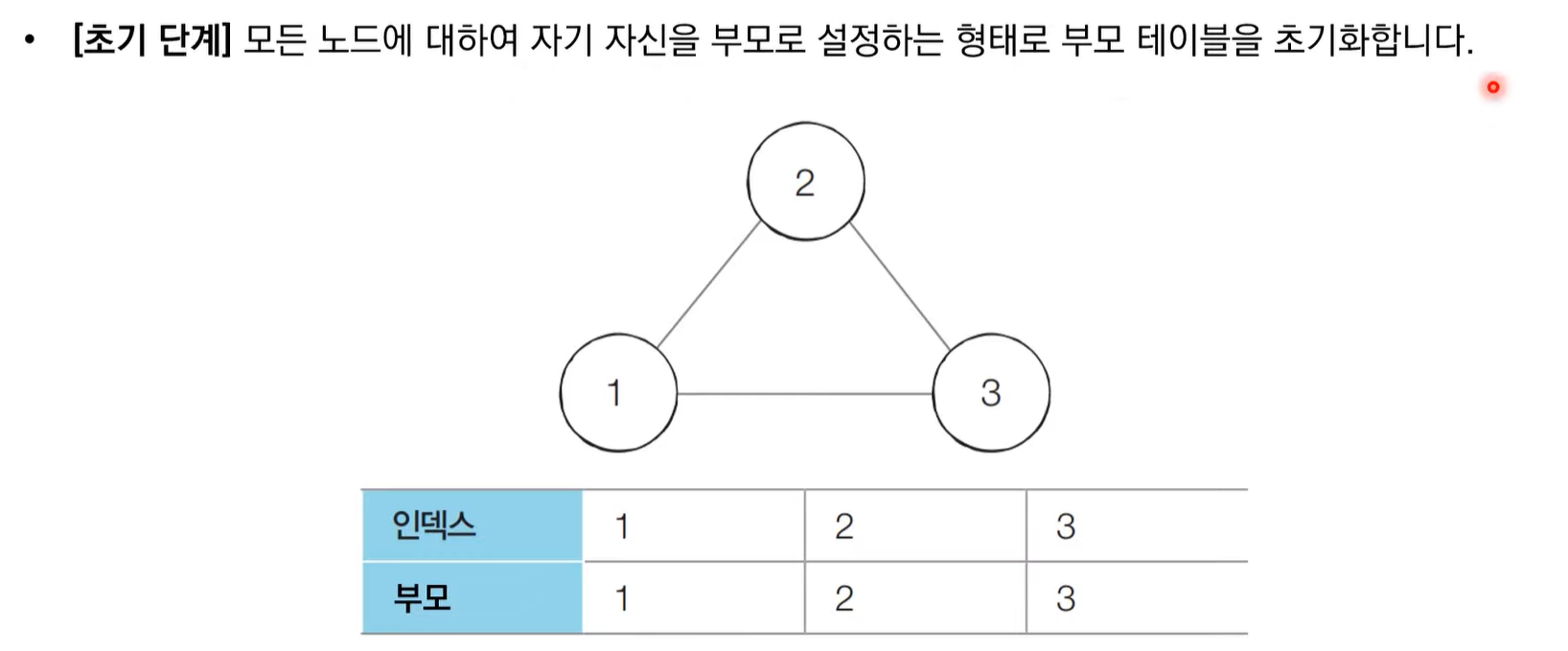
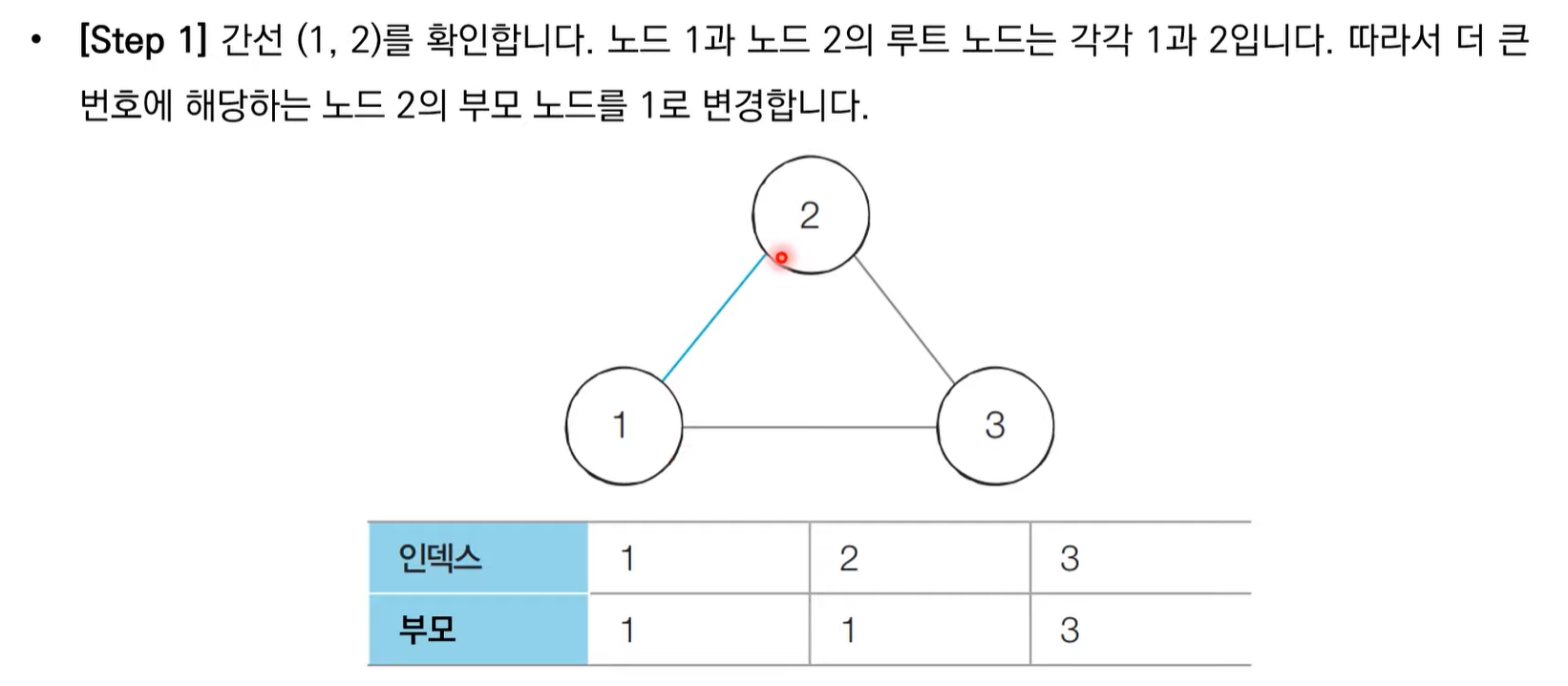
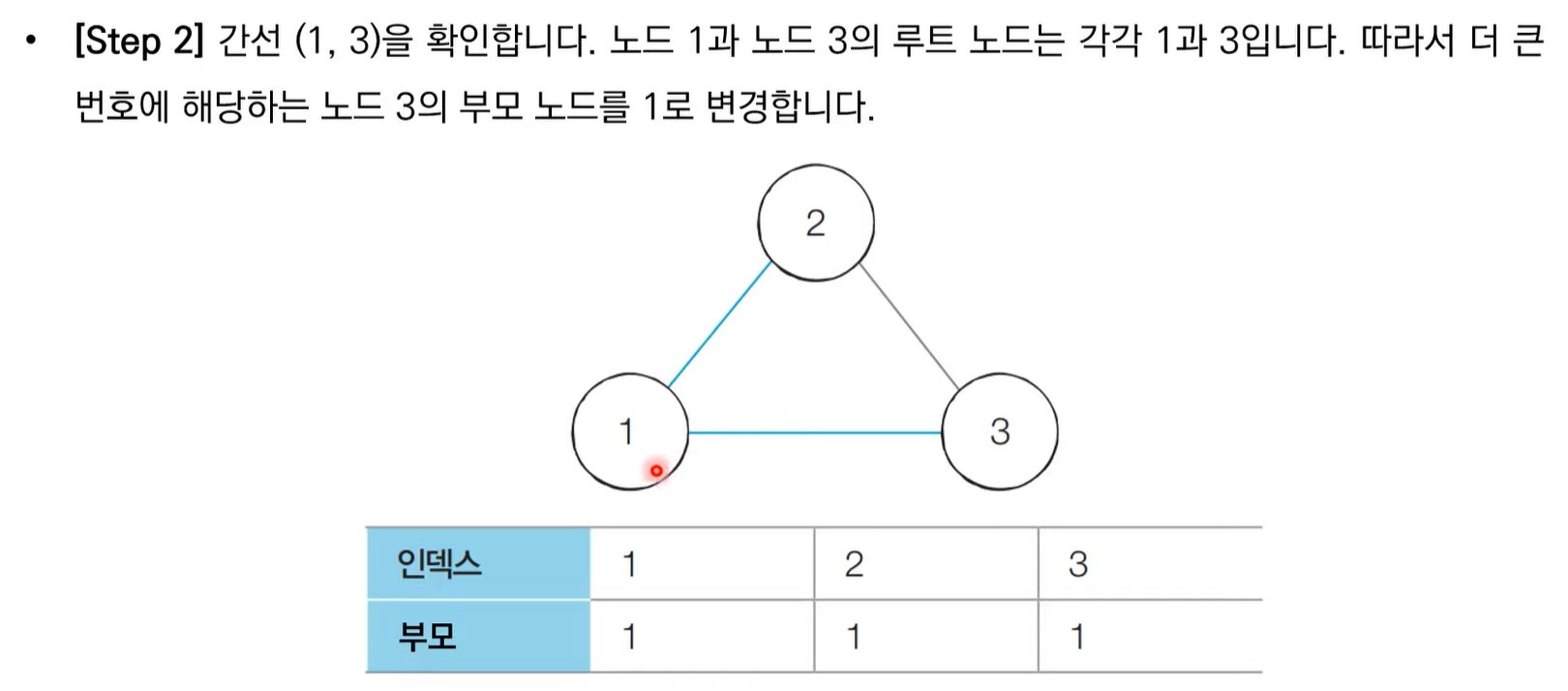
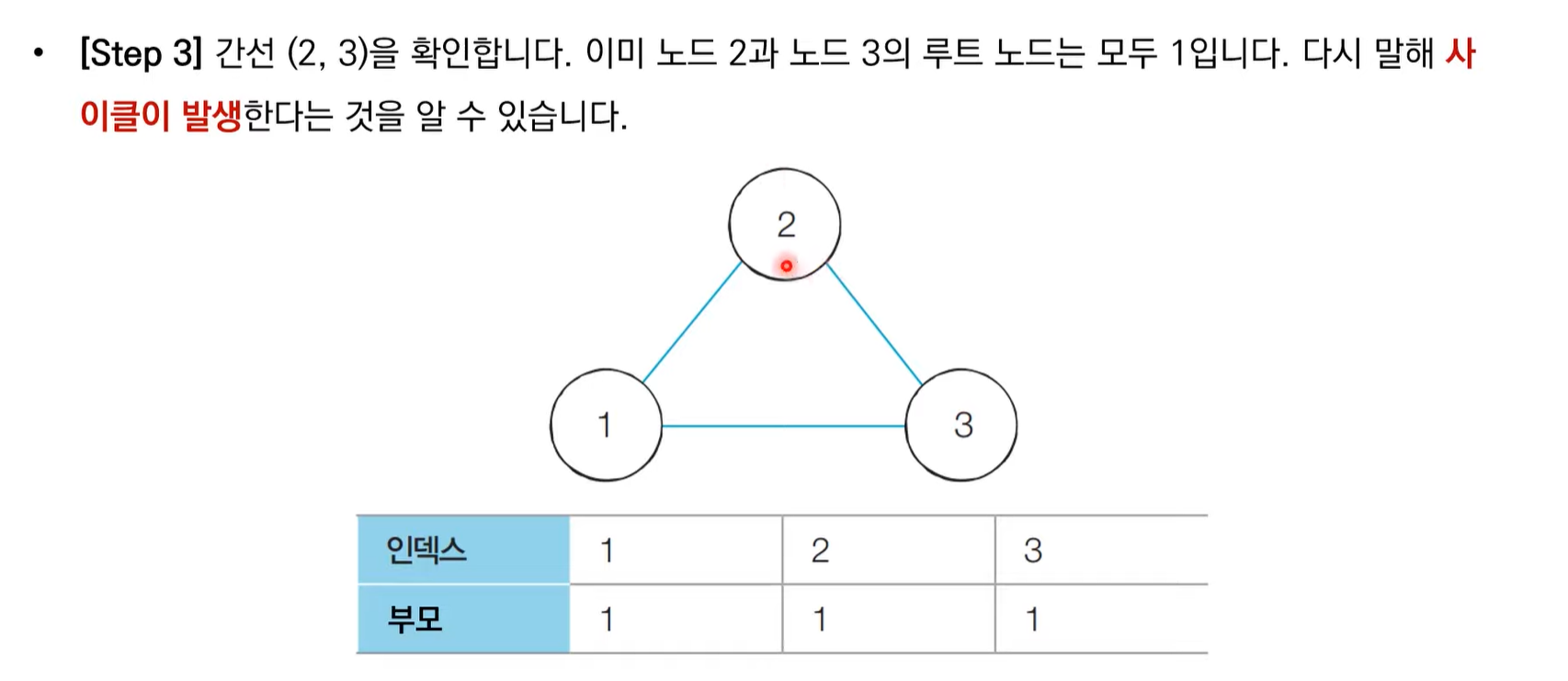
서로소 집합을 활용한 사이클 판별 구현
def find_parent(parent,x):
if parent[x] != x:
parent[x] = find_parent(parent,parent[x])
return parent[x]
def union_parent(parent,a,b):
a = find_parent(parent,a)
b = find_parent(parent,b)
if a < b:
parent[b] = a
else:
parent[a] = b
v,e = map(int,input().split())
parent = [0] * (v+1)
for i in range(1,v+1):
parent[i] = i
cycle = False
for i in range(e):
a,b = map(int,input().split())
if find_parent(parent,a) == find_parent(parent,b):
cycle = True
break
else:
union_parent(parent,a,b)
if cycle:
print("사이클이 발생했습니다.")
else:
print("사이클이 발생하지 않았습니다.")
크루스칼 알고리즘
신장 트리
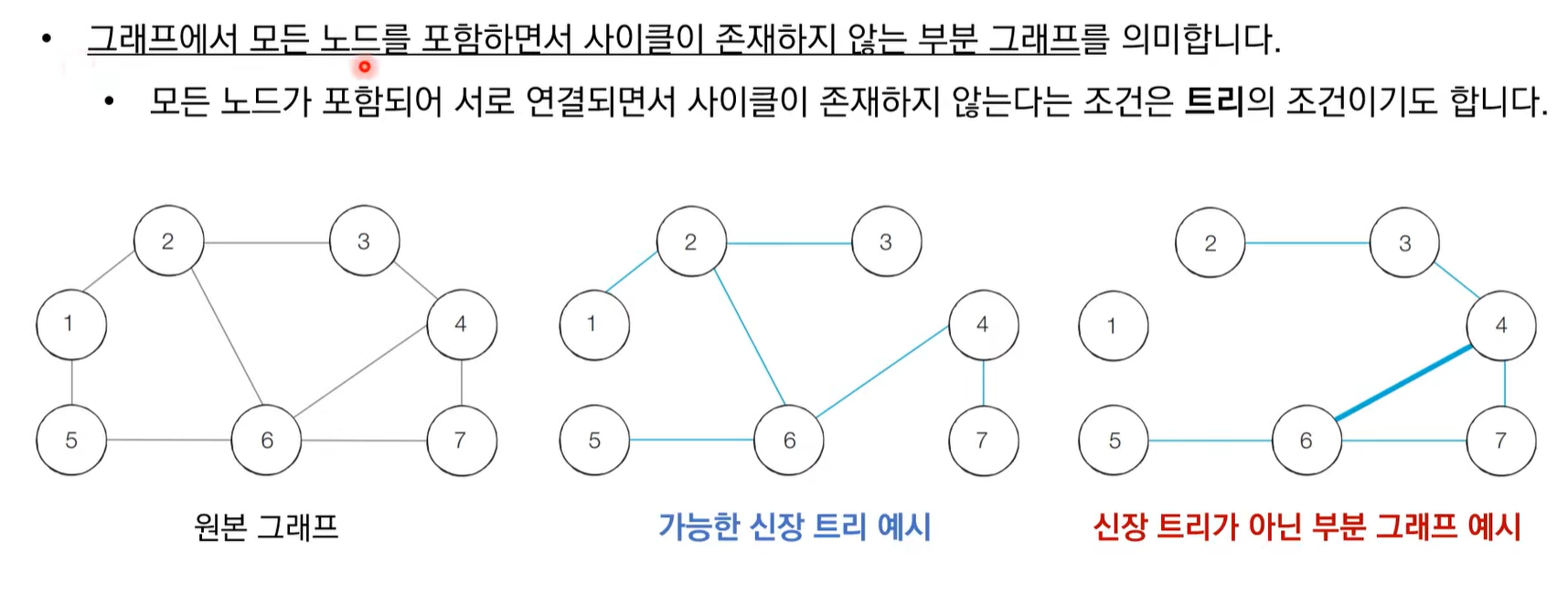
최소 신장 트리(MST,Minimum Spanning Tree)
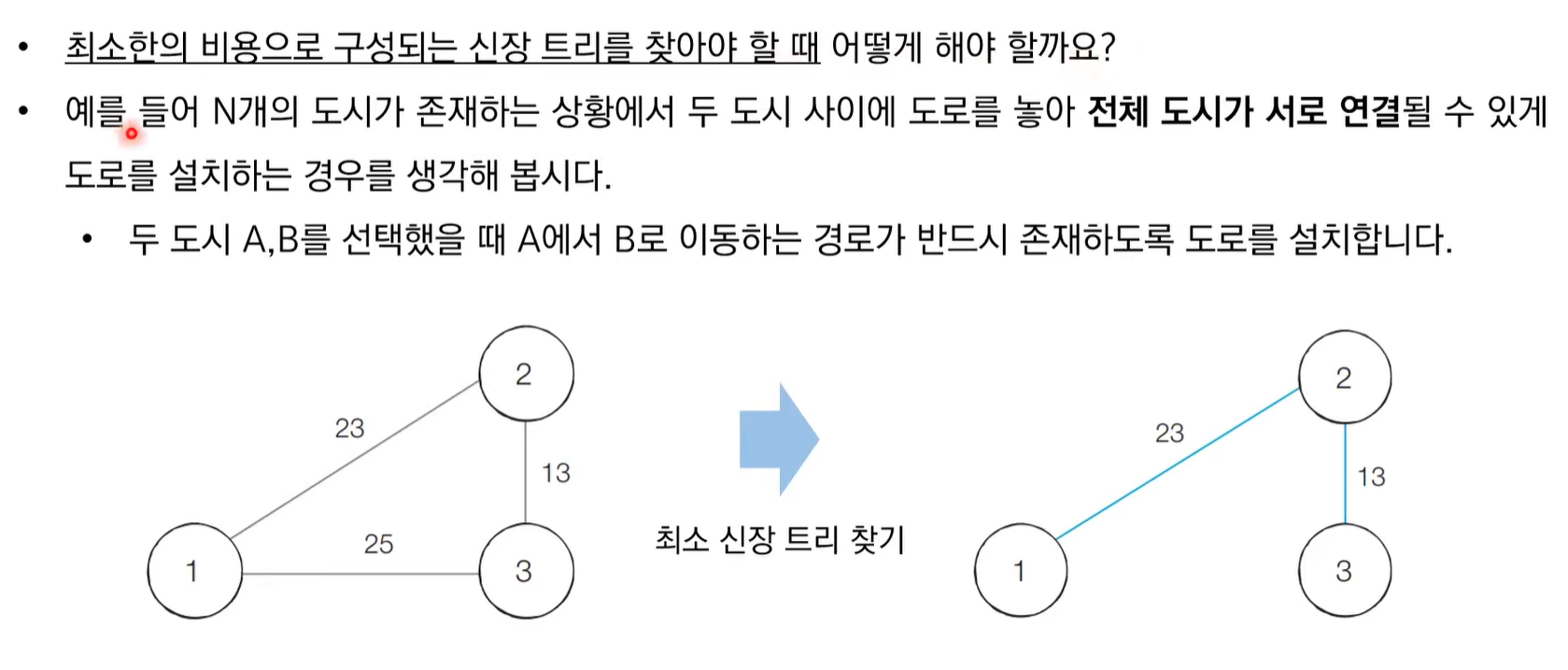
크루스칼 알고리즘
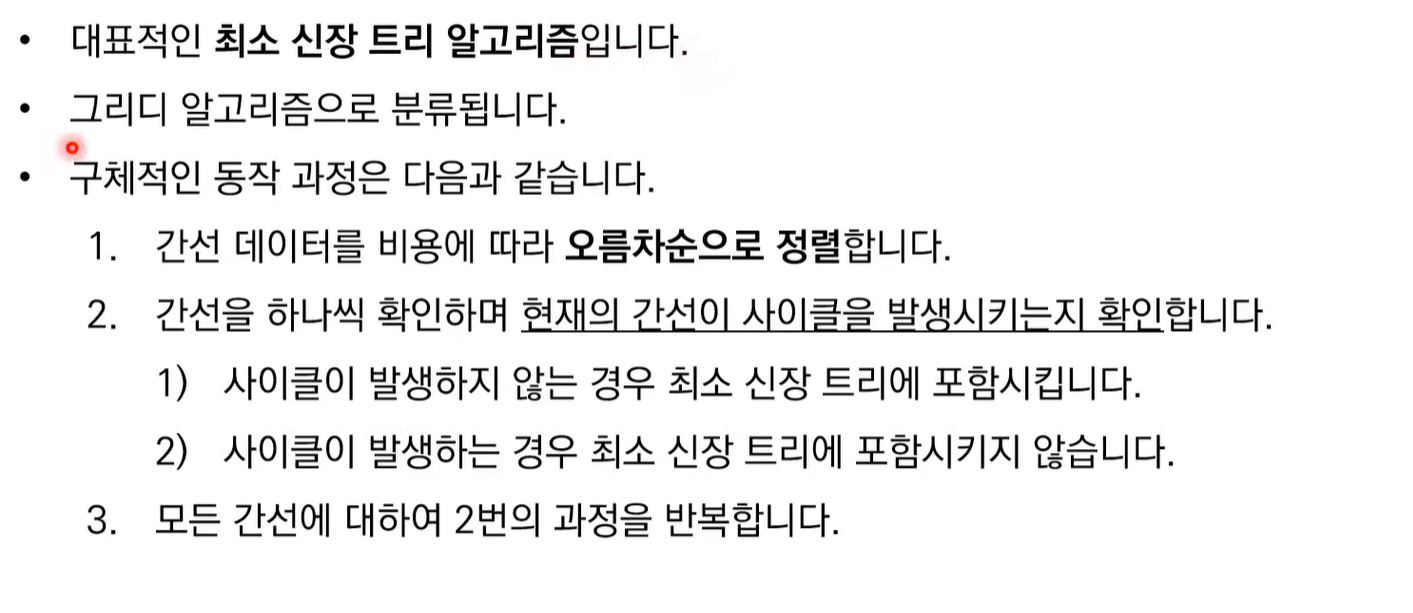
크루스칼 알고리즘: 동작 과정 살펴보기
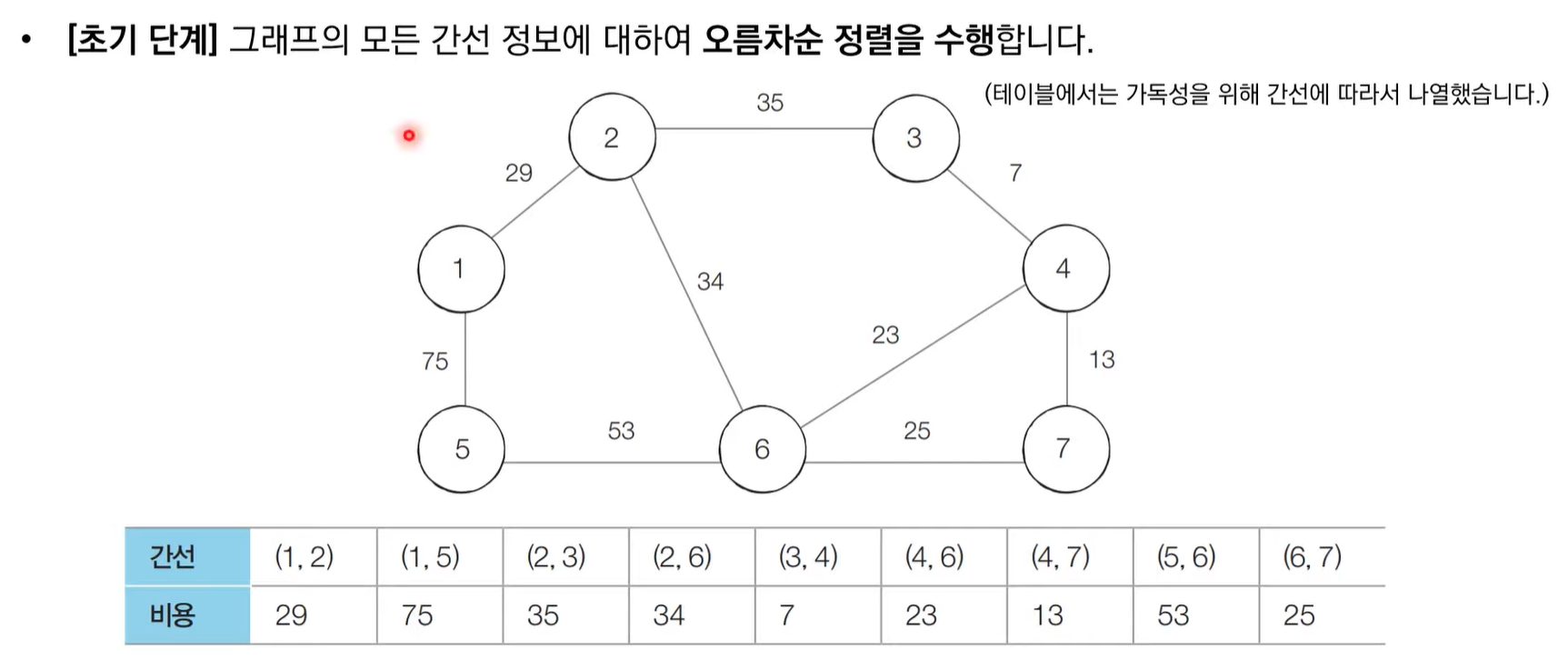
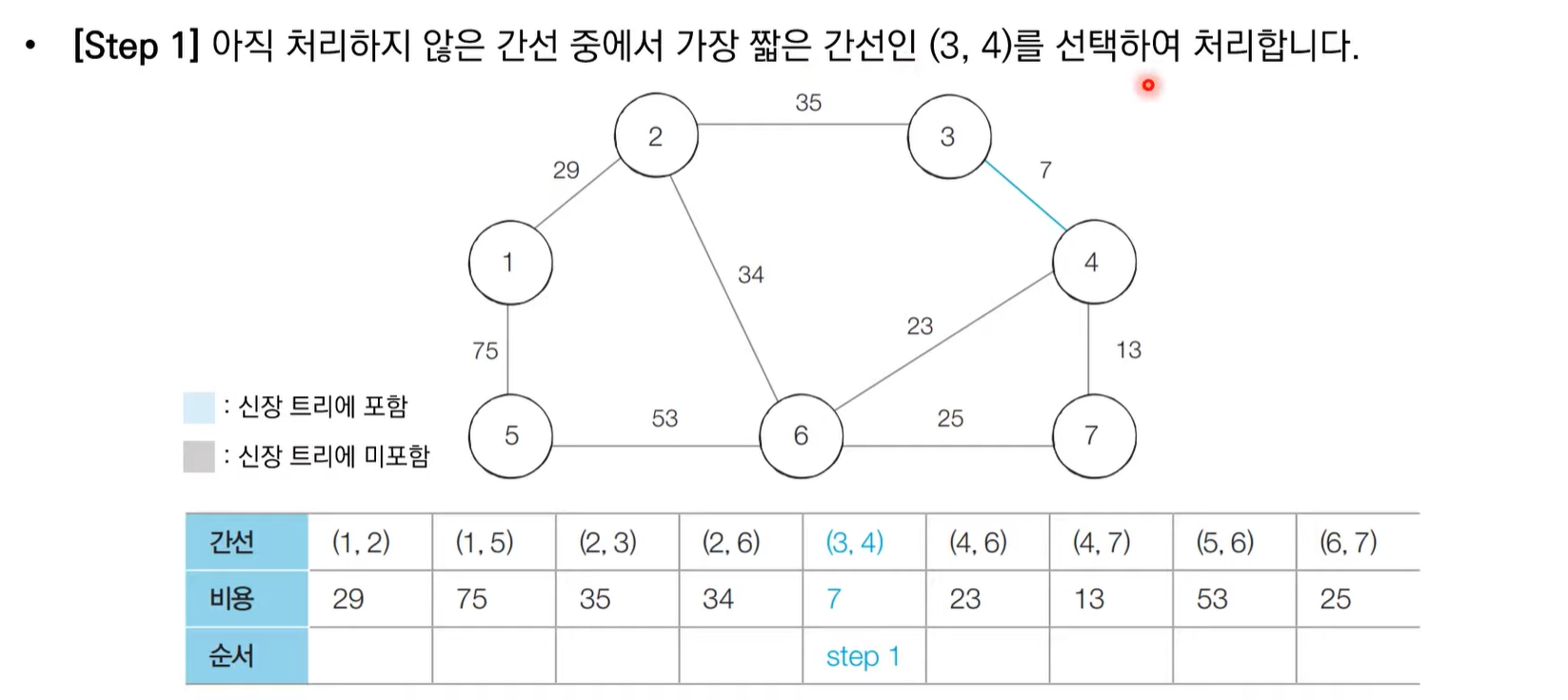
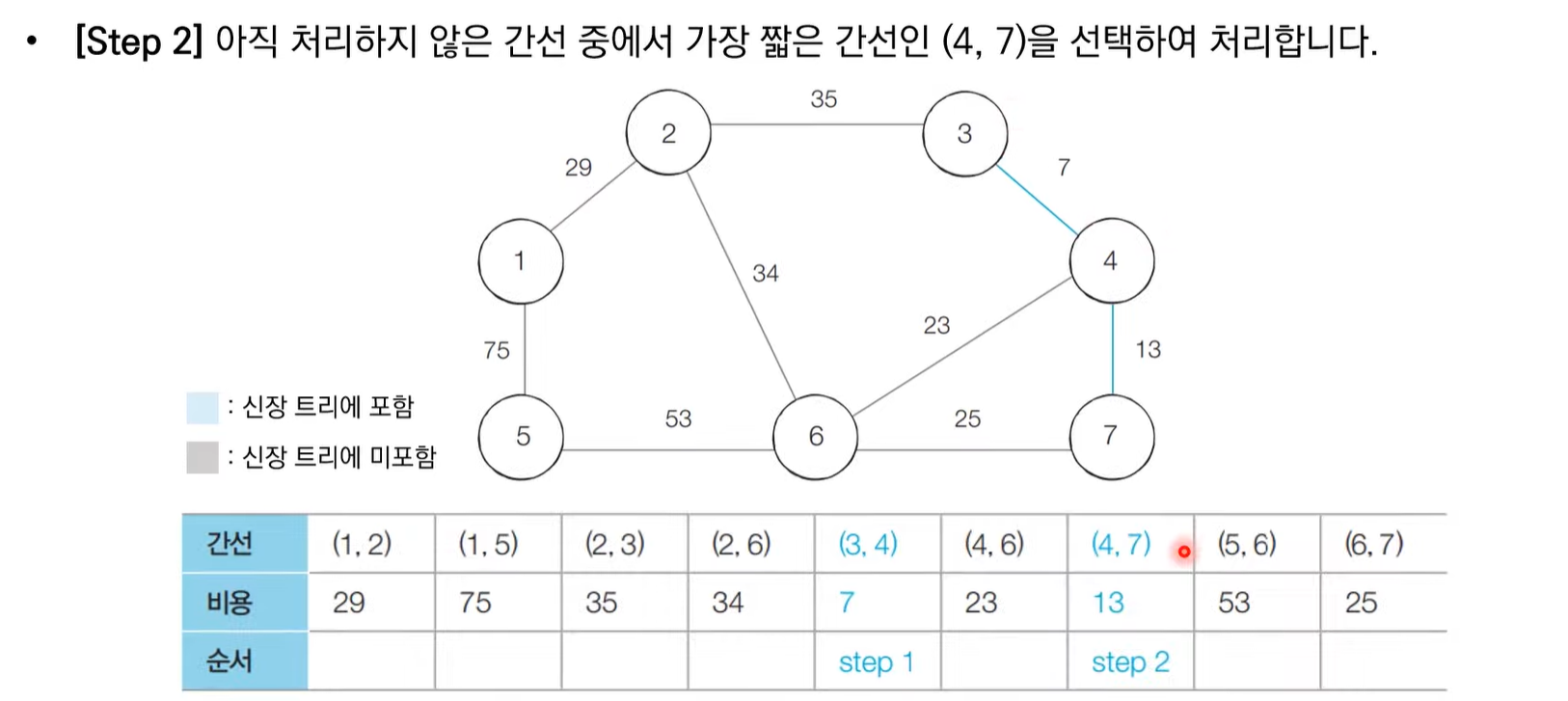
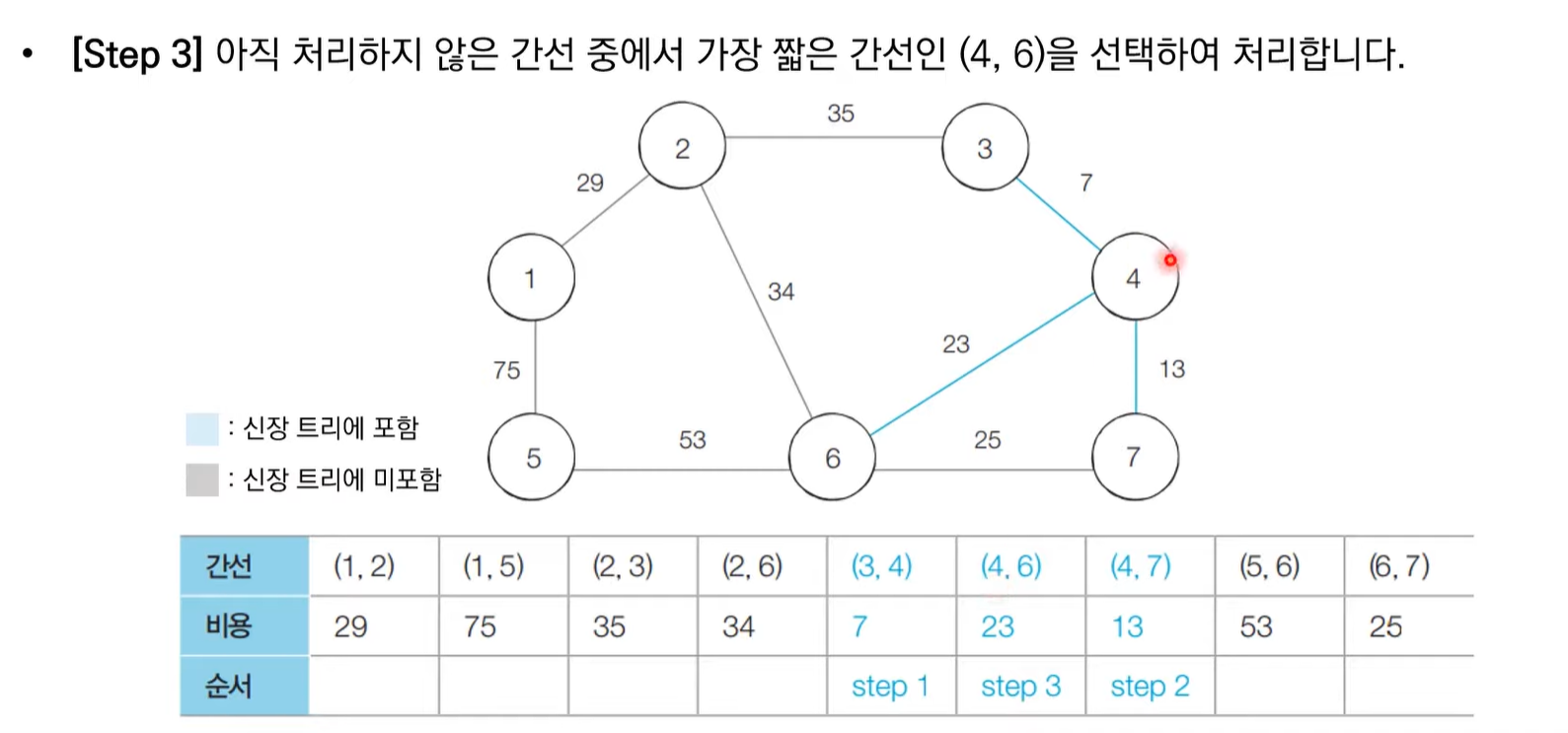
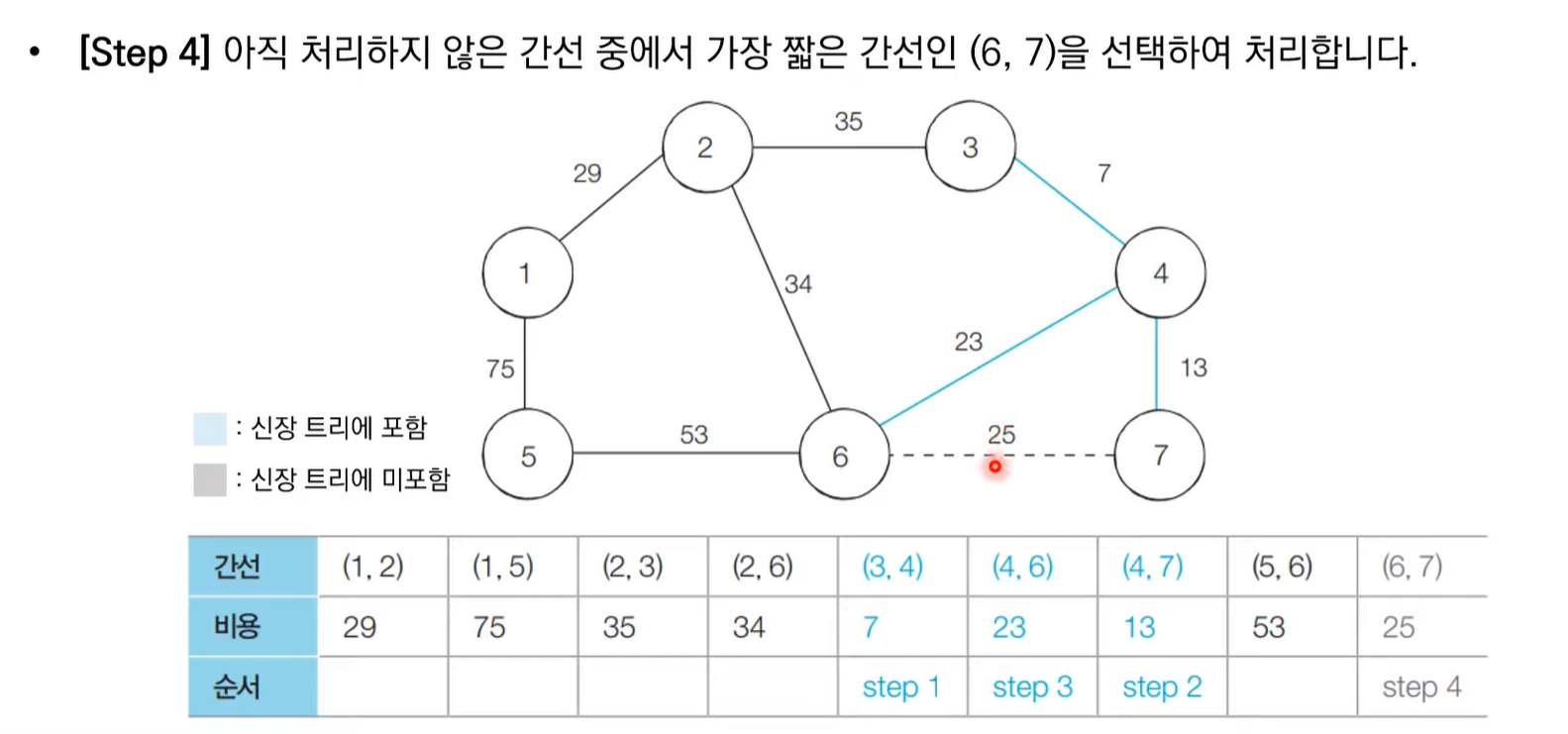
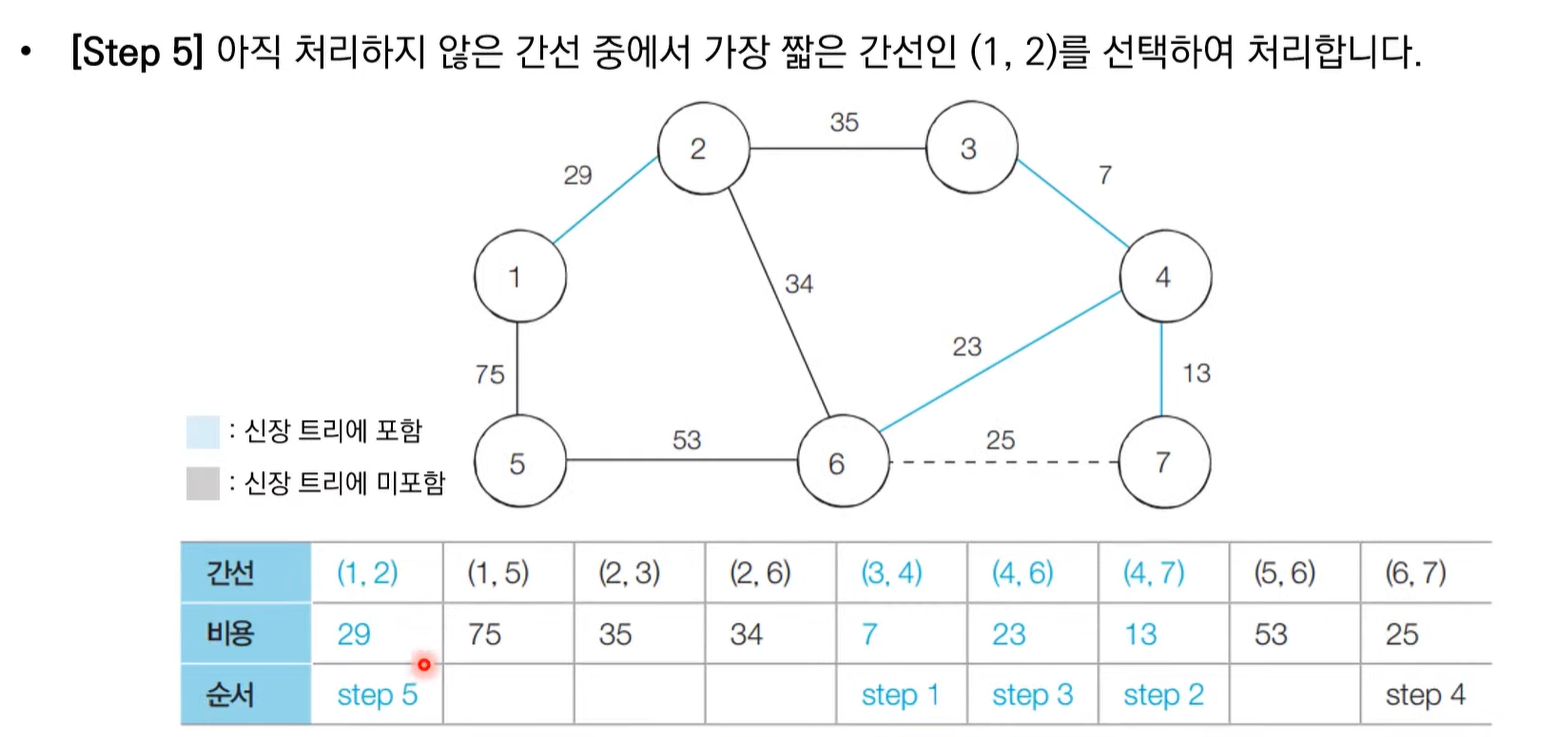
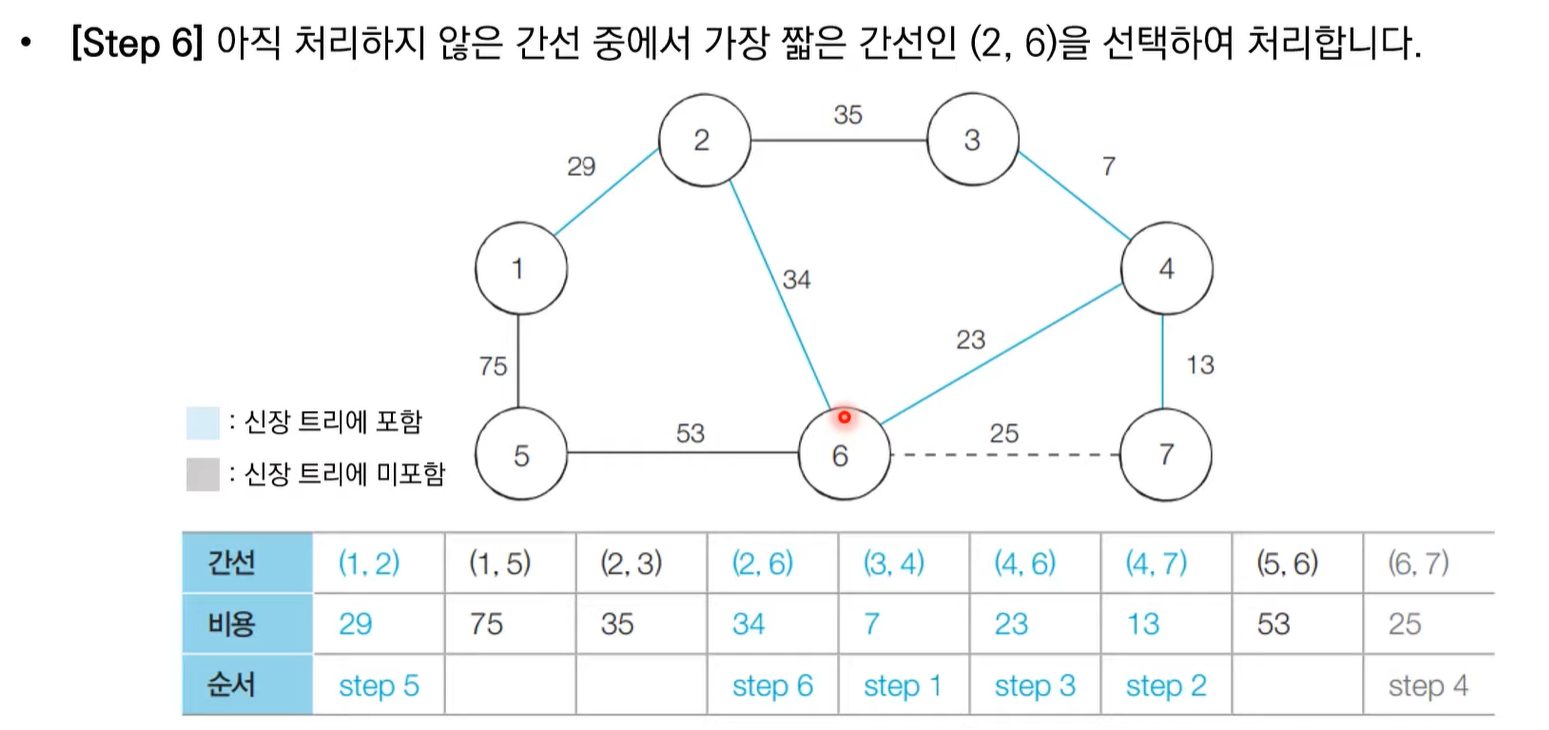
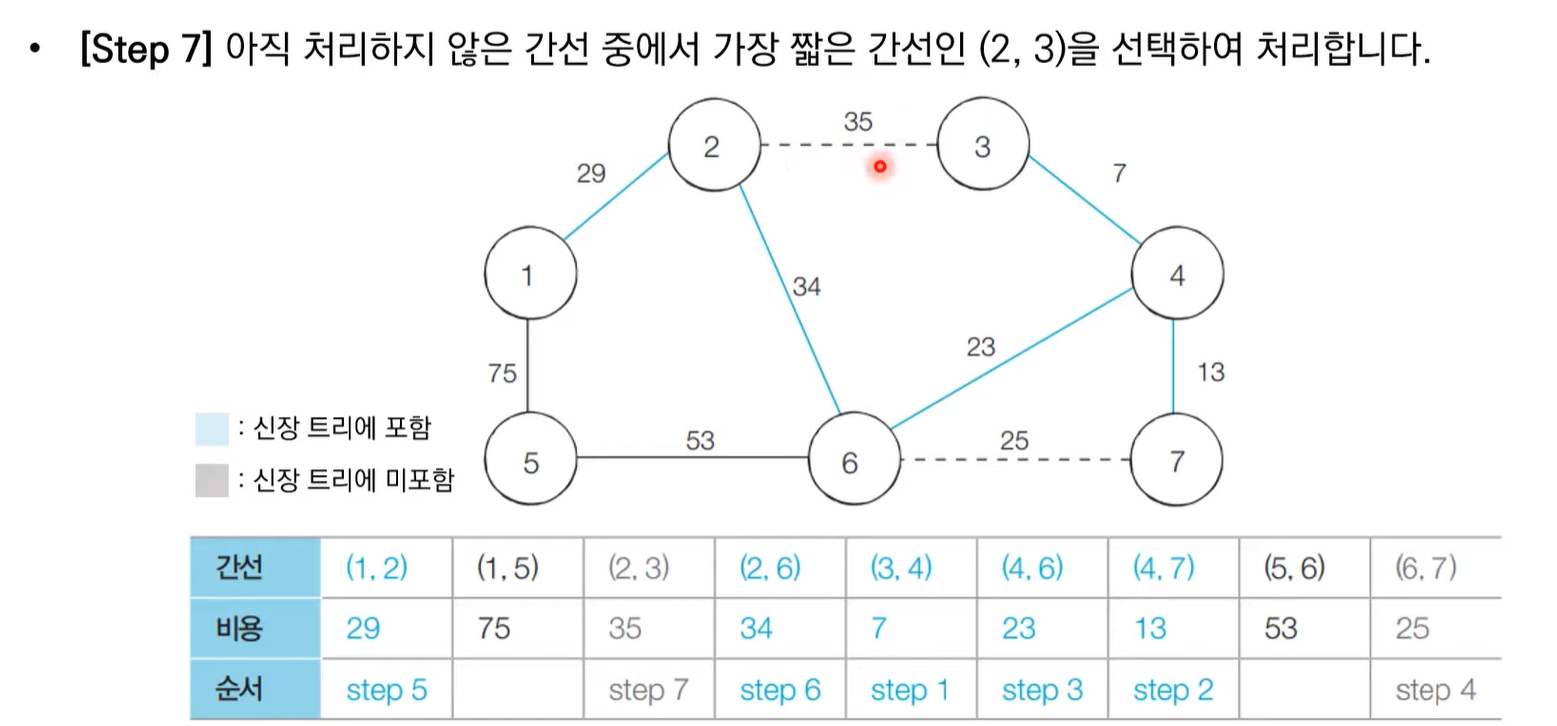
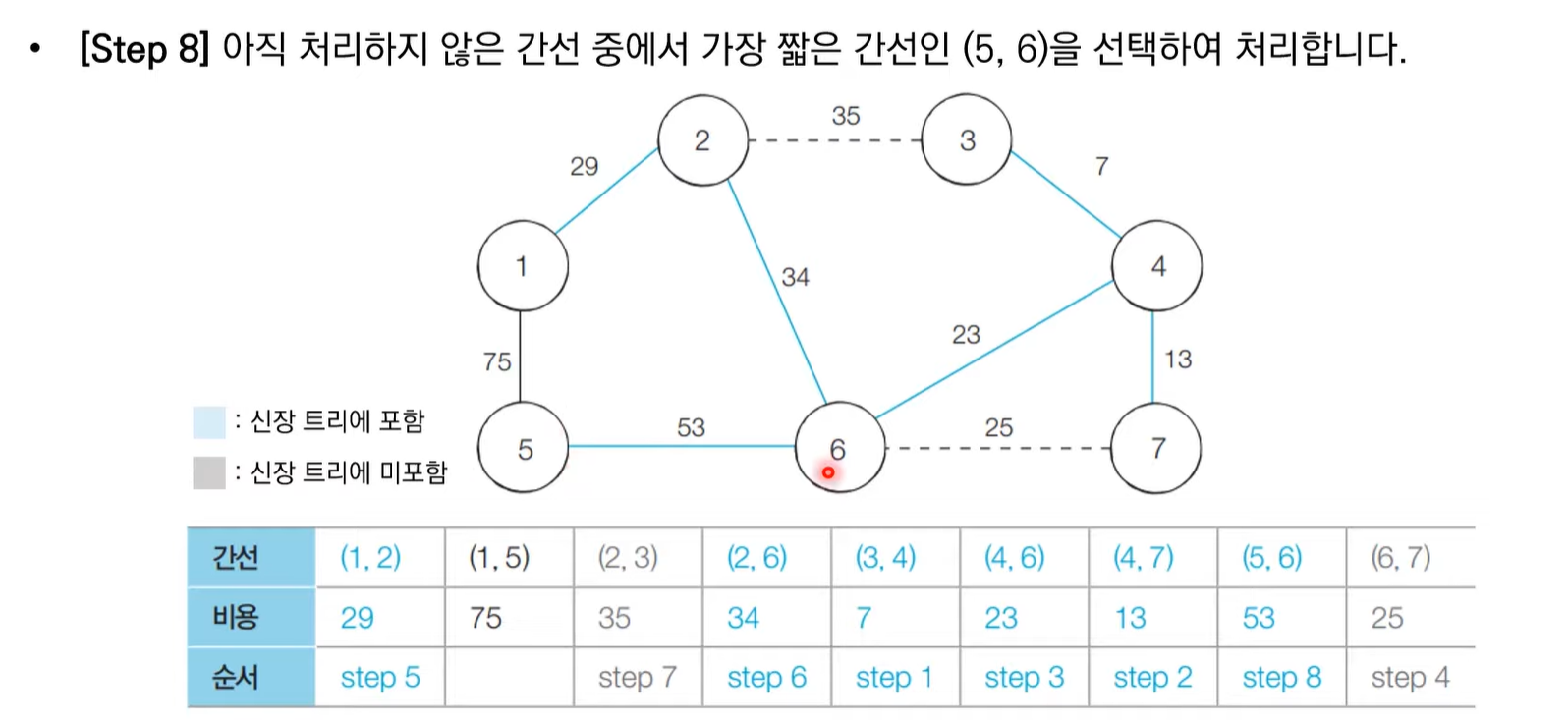
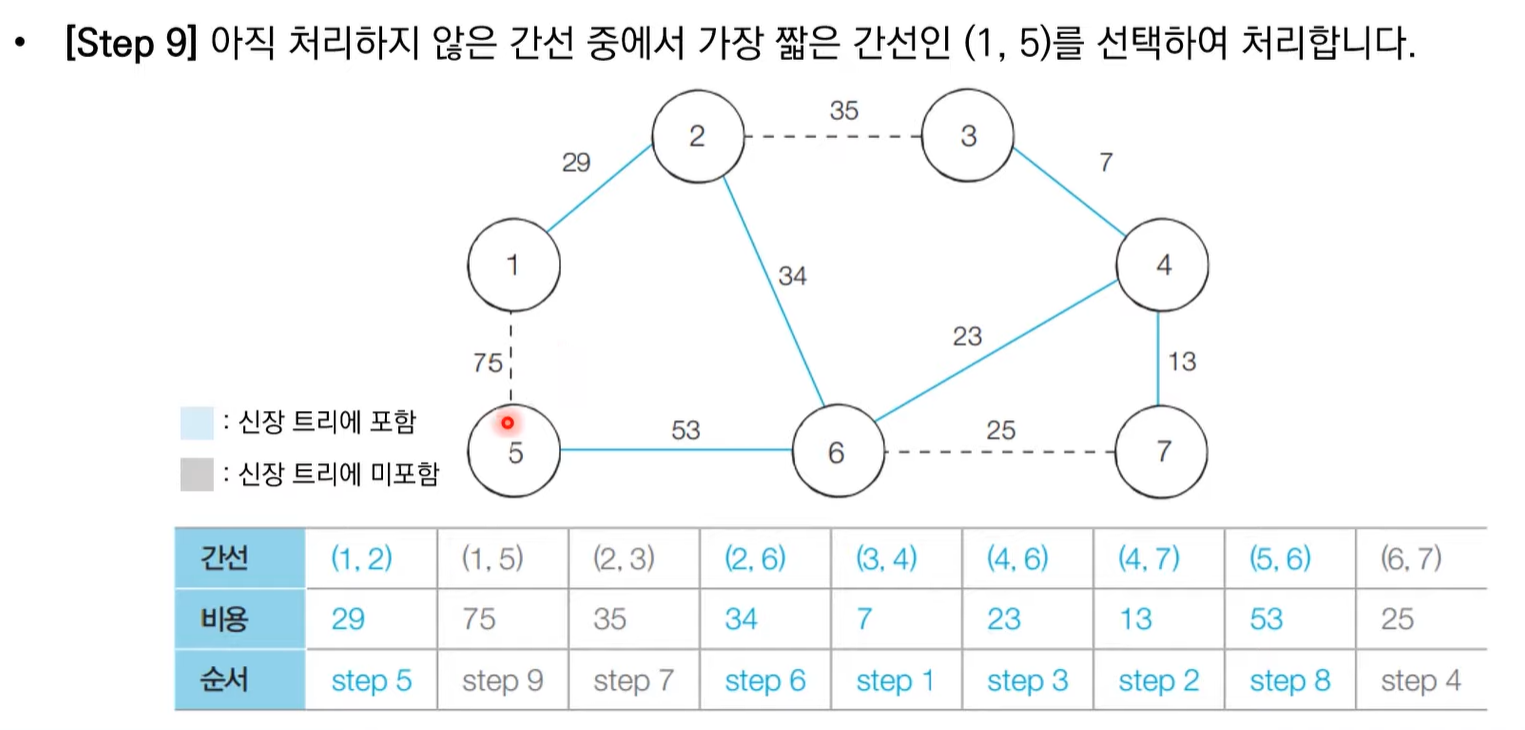
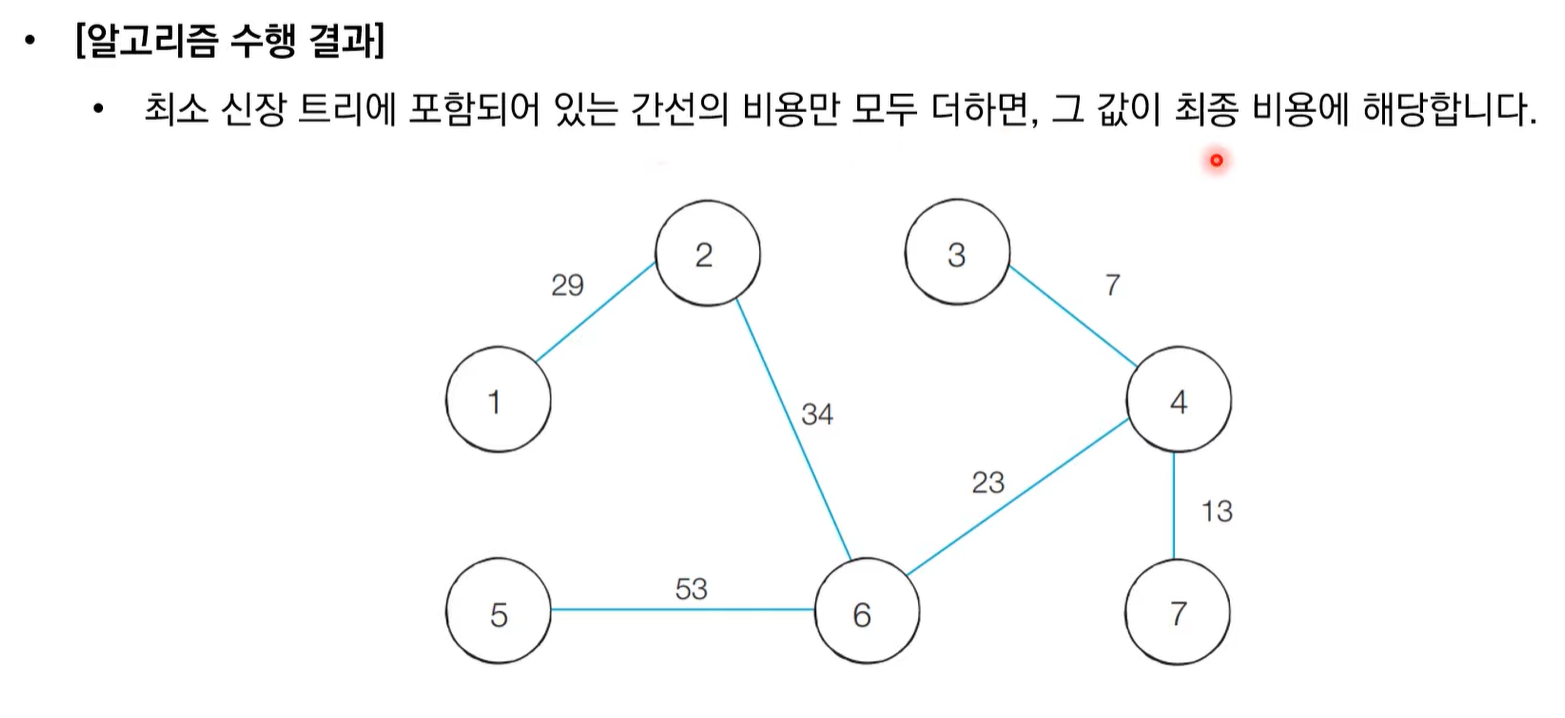
크루스칼 알고리즘 구현
def find_parent(parent,x):
if parent[x] != x:
parent[x] = find_parent(parent,parent[x])
return parent[x]
def union_parent(parent,a,b):
a = find_parent(parent,a)
b = find_parent(parent,b)
if a < b:
parent[b] = a
else:
parent[a] = b
v,e = map(int,input().split())
parent = [0] * (v+1)
edges = []
result = 0
for i in range(1,v+1):
parent[i] = i
for _ in range(e):
a,b,cost = map(int,input().split())
edges.append((cost,a,b))
edges.sort()
for edge in edges:
cost, a, b = edge
if find_parent(parent,a) != find_parent(parent,b):
union_parent(parent,a,b)
result += cost
print(result)
크루스칼 알고리즘 성능 분석

위상 정렬
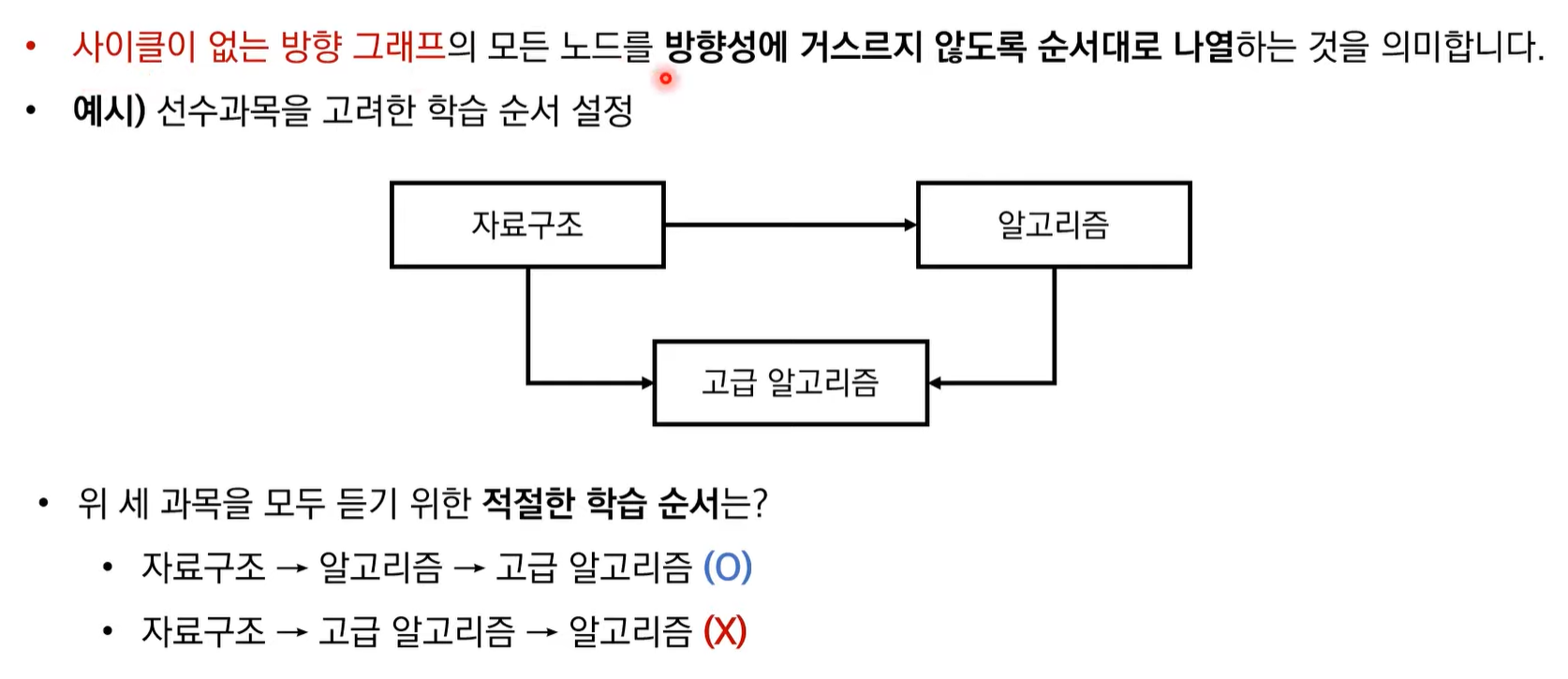
진입차수와 진출차수
- 진입차수(Indegree): 특정한 노드로 들어오는 간선의 개수
- 진출차수(Outdegree): 특정한 노드에서 나가는 간선의 개수
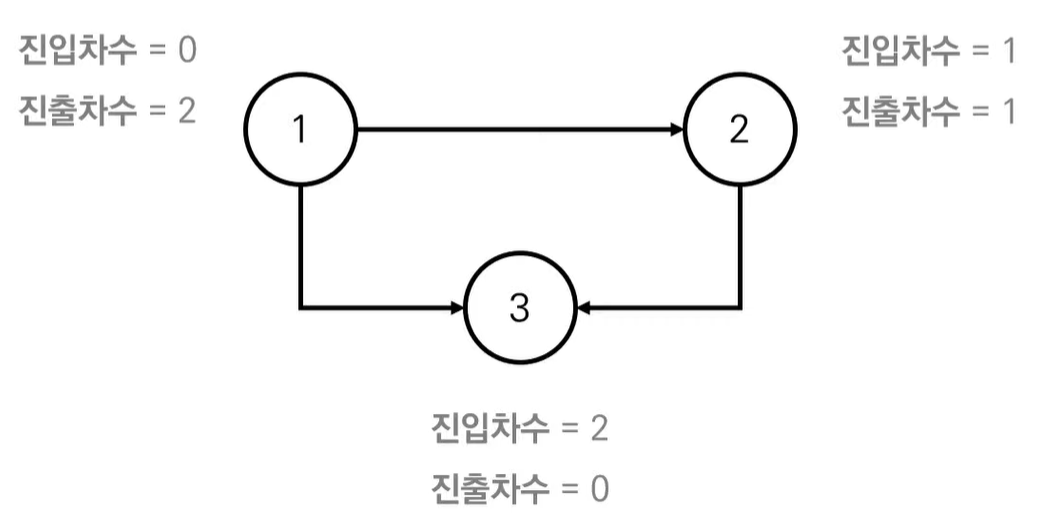
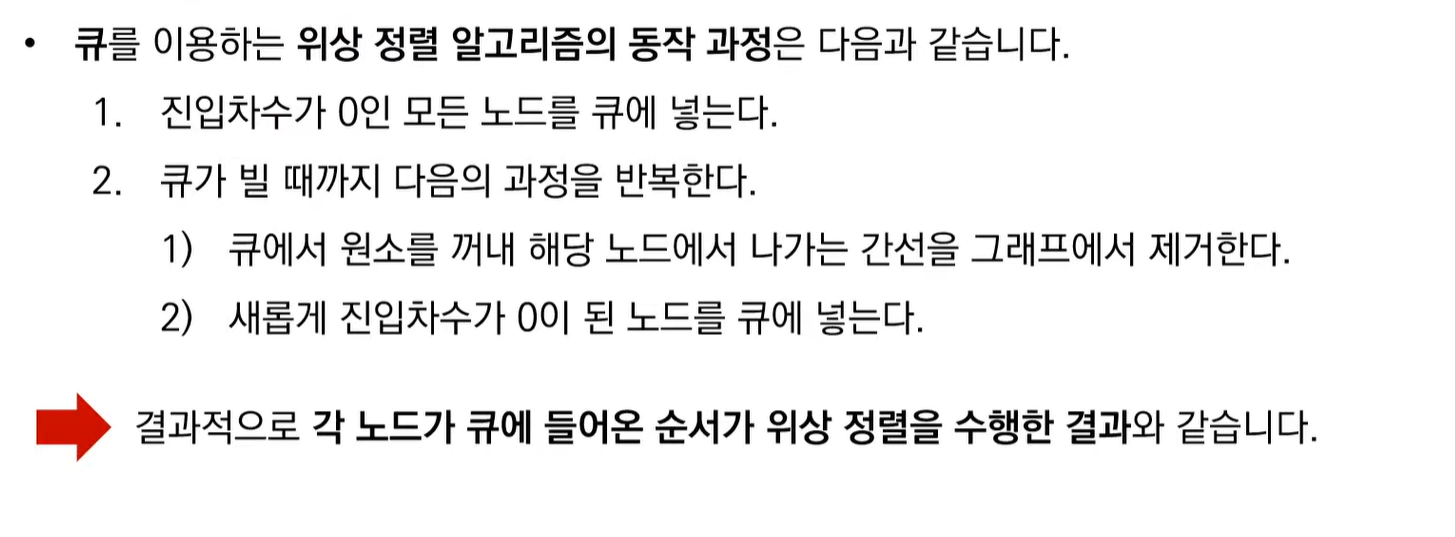
위상 정렬 동작 예시
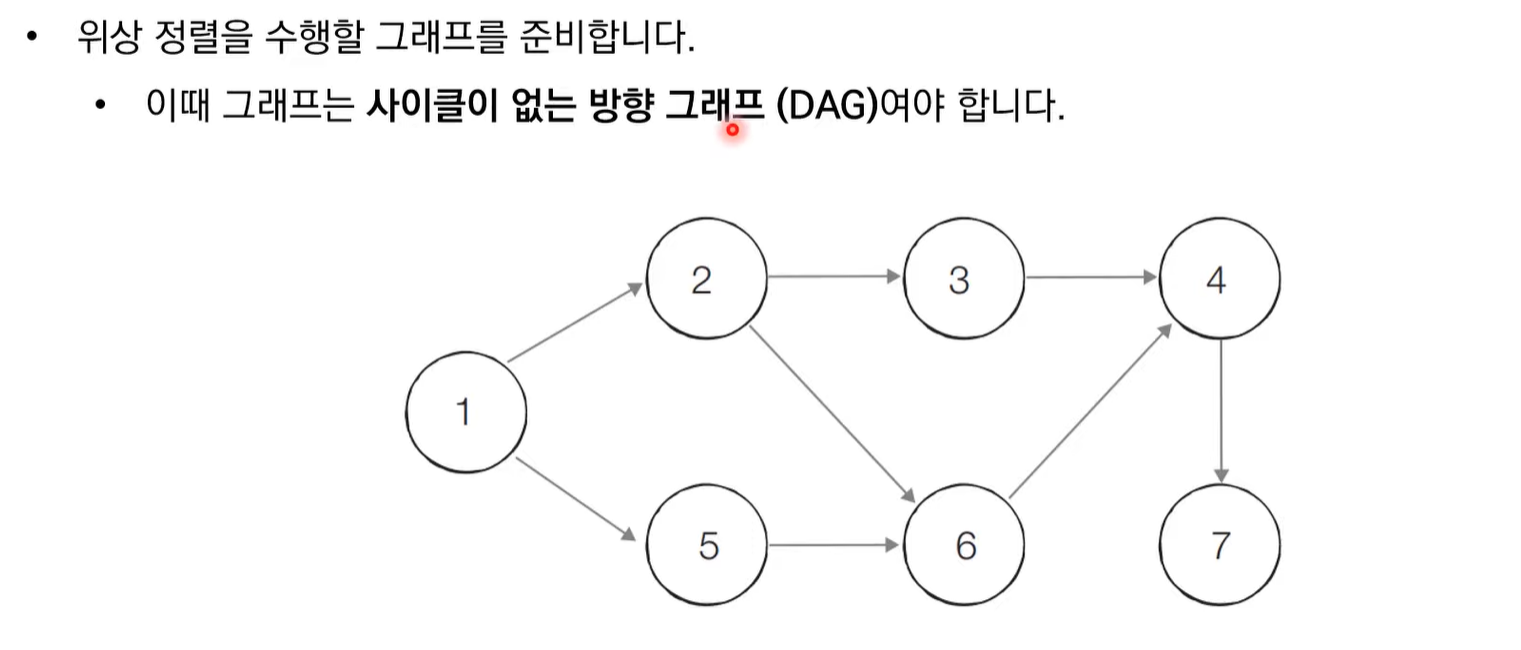
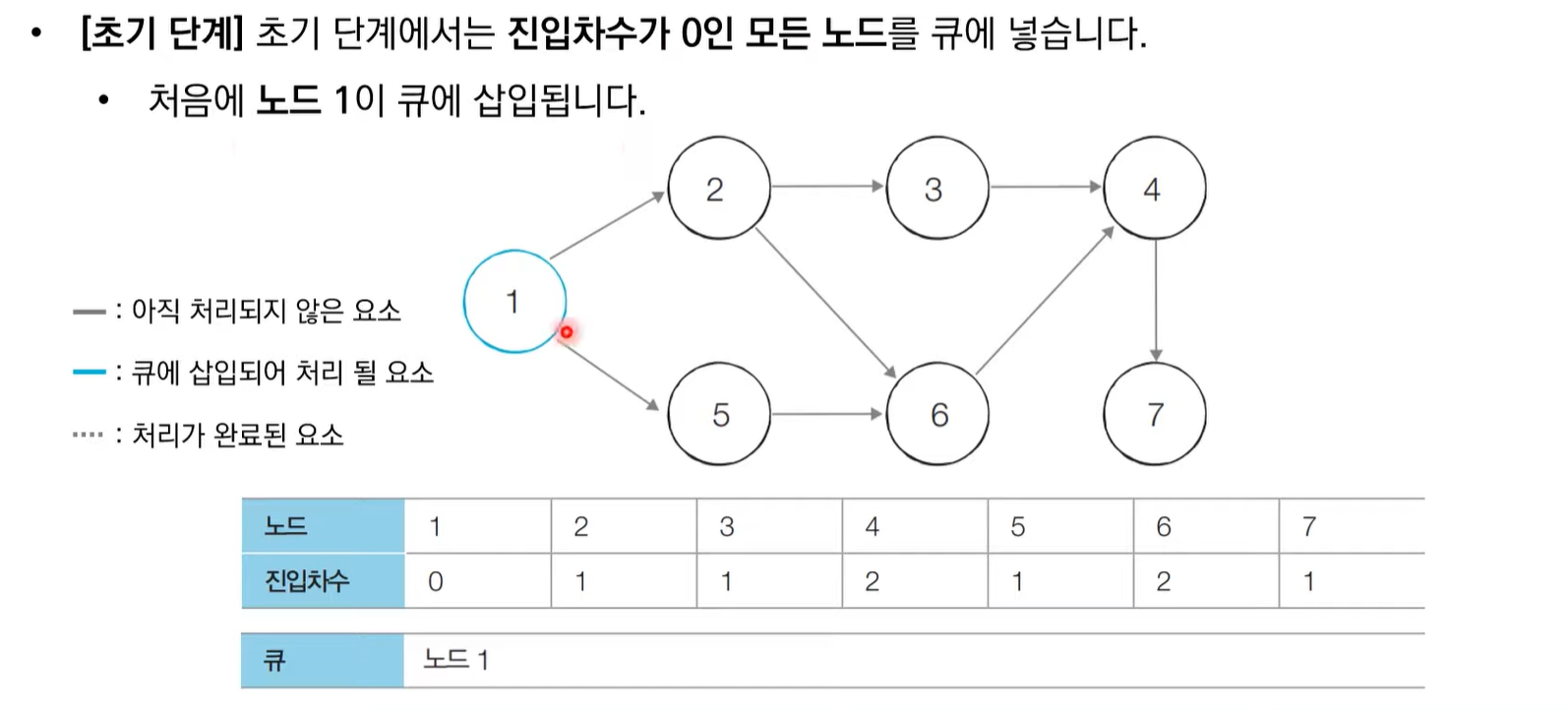
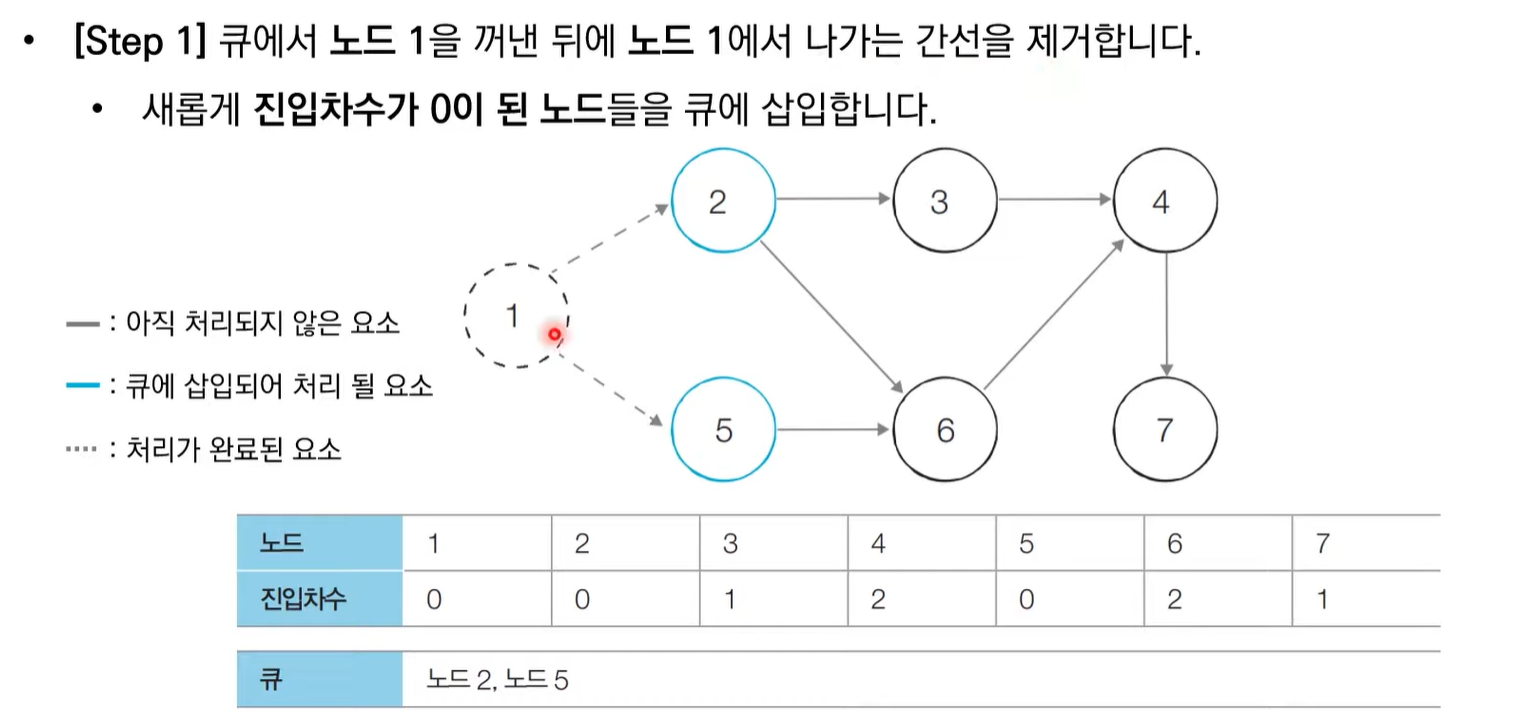
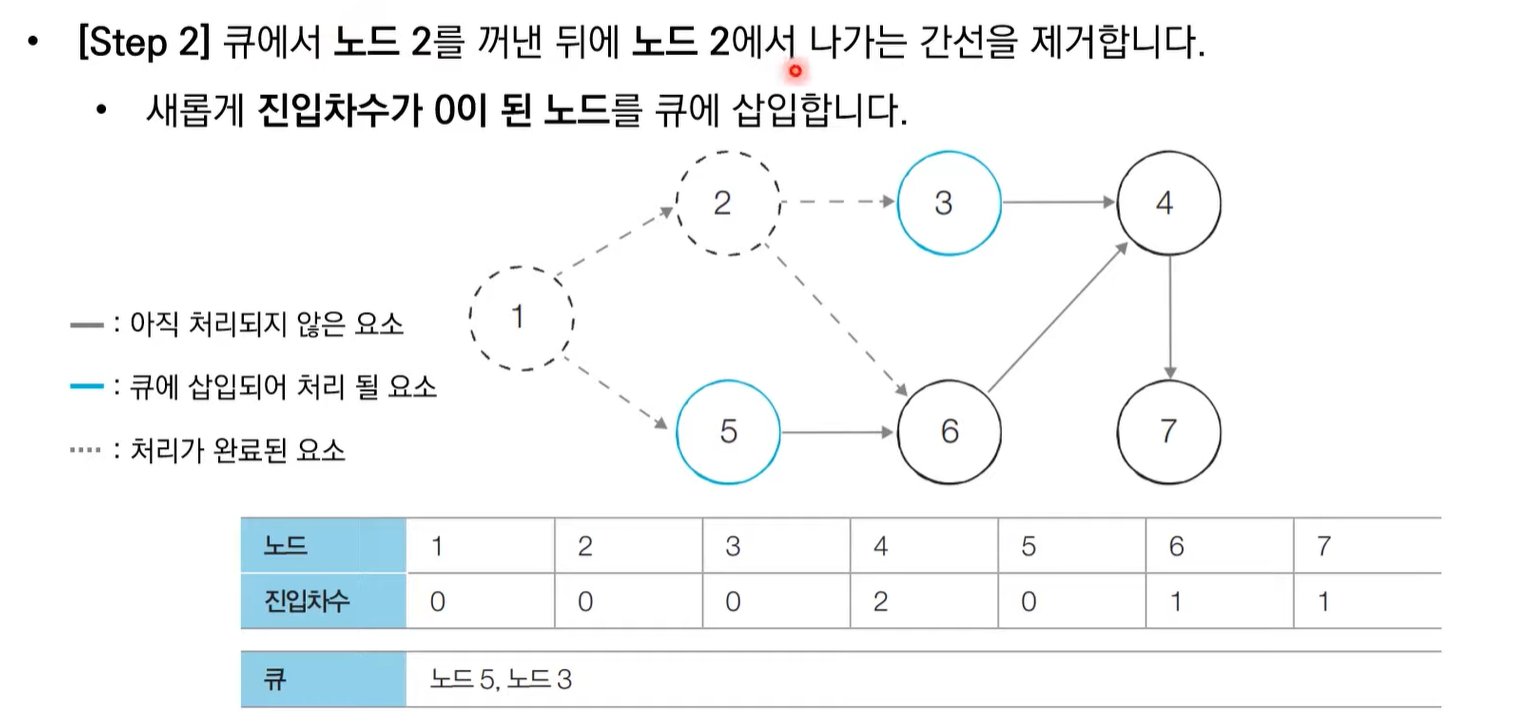
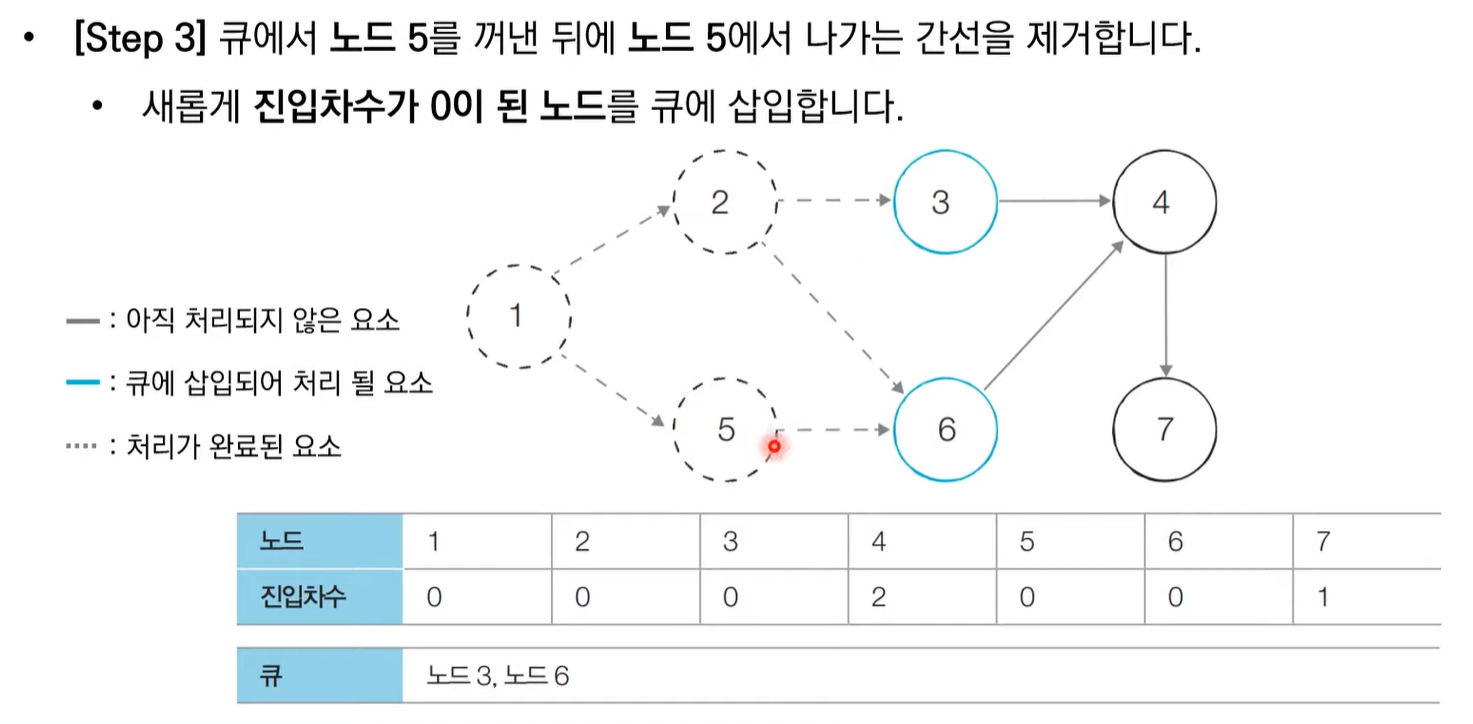
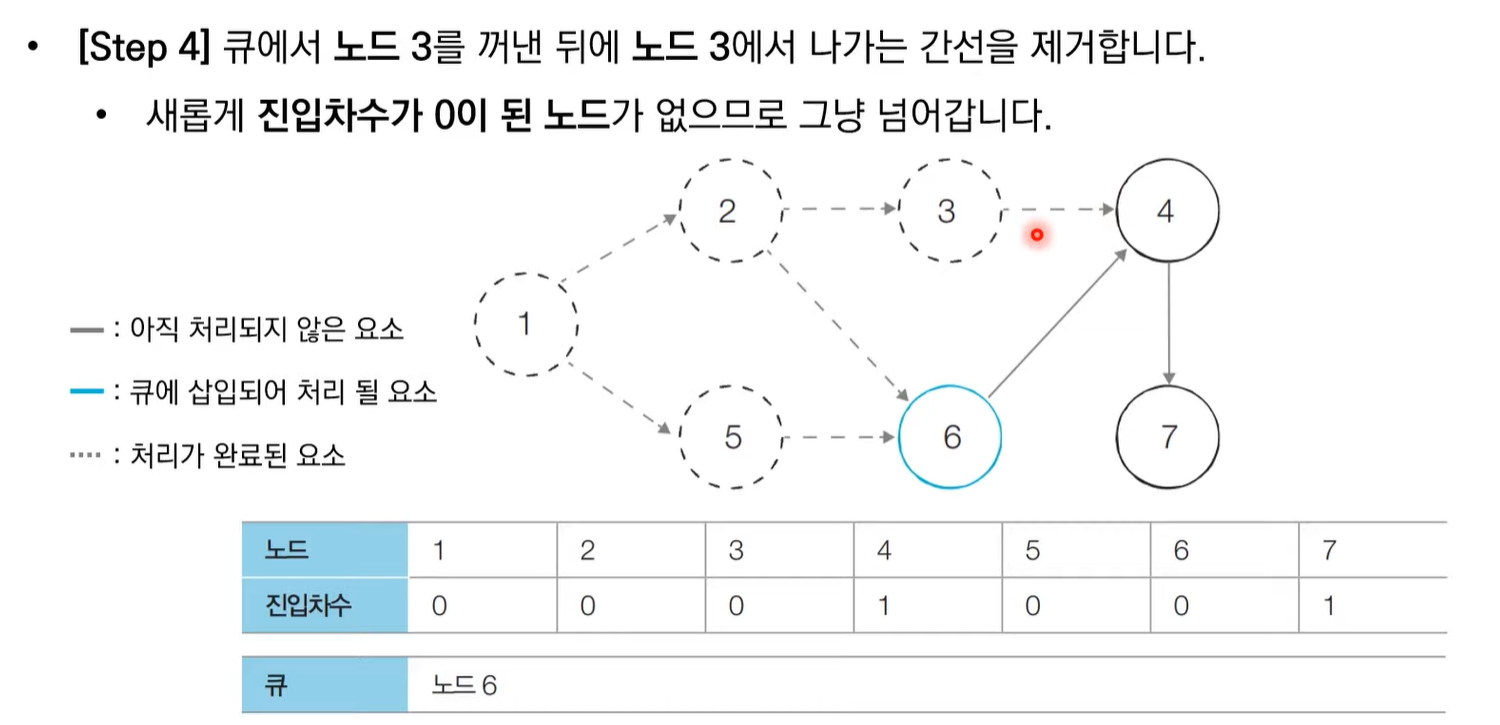
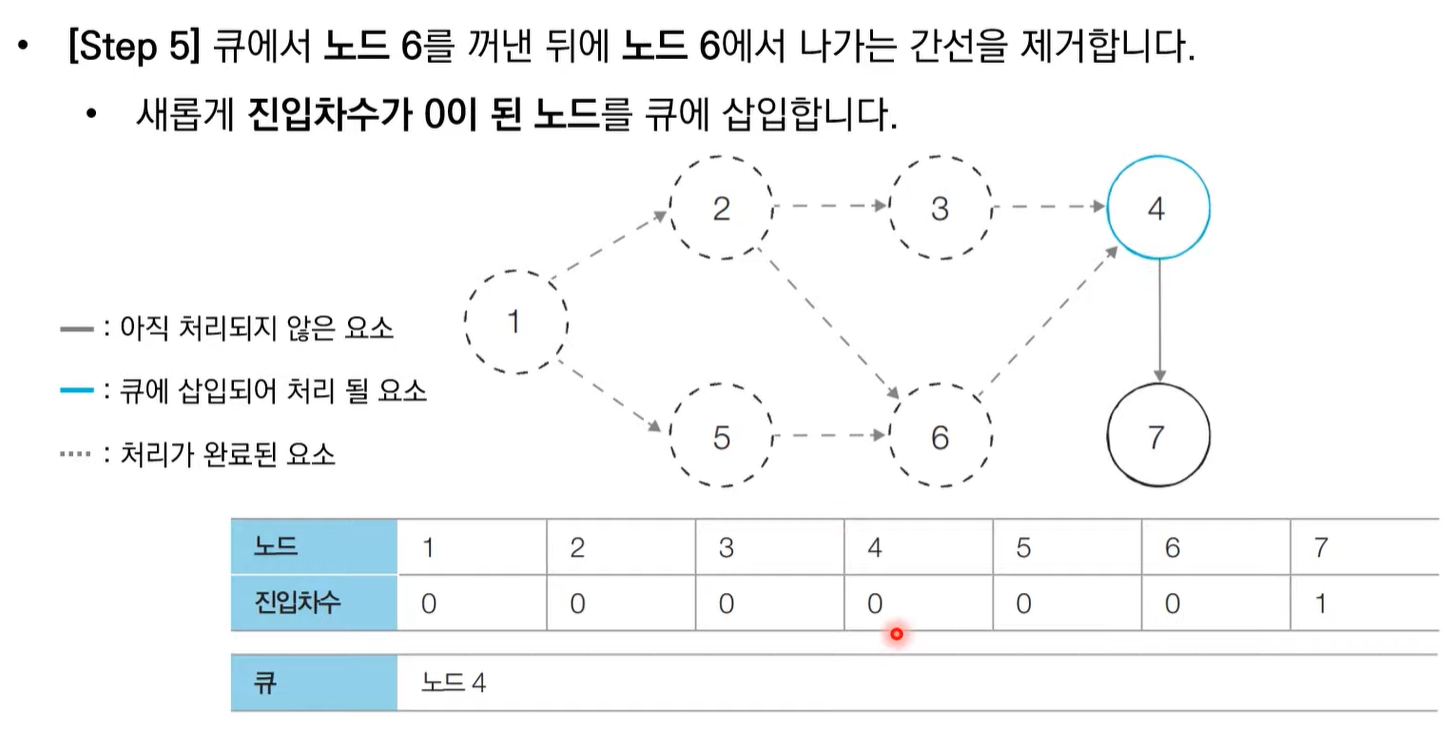
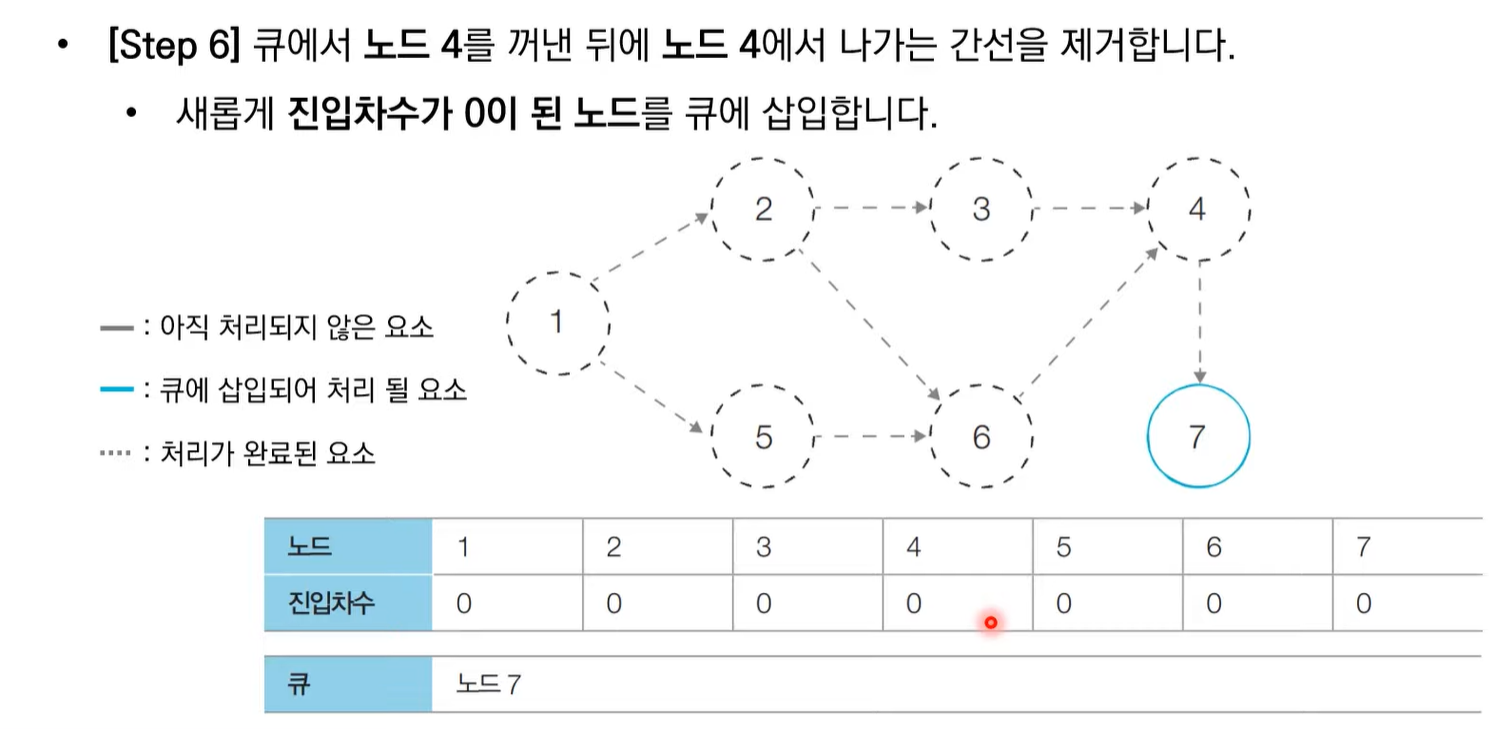
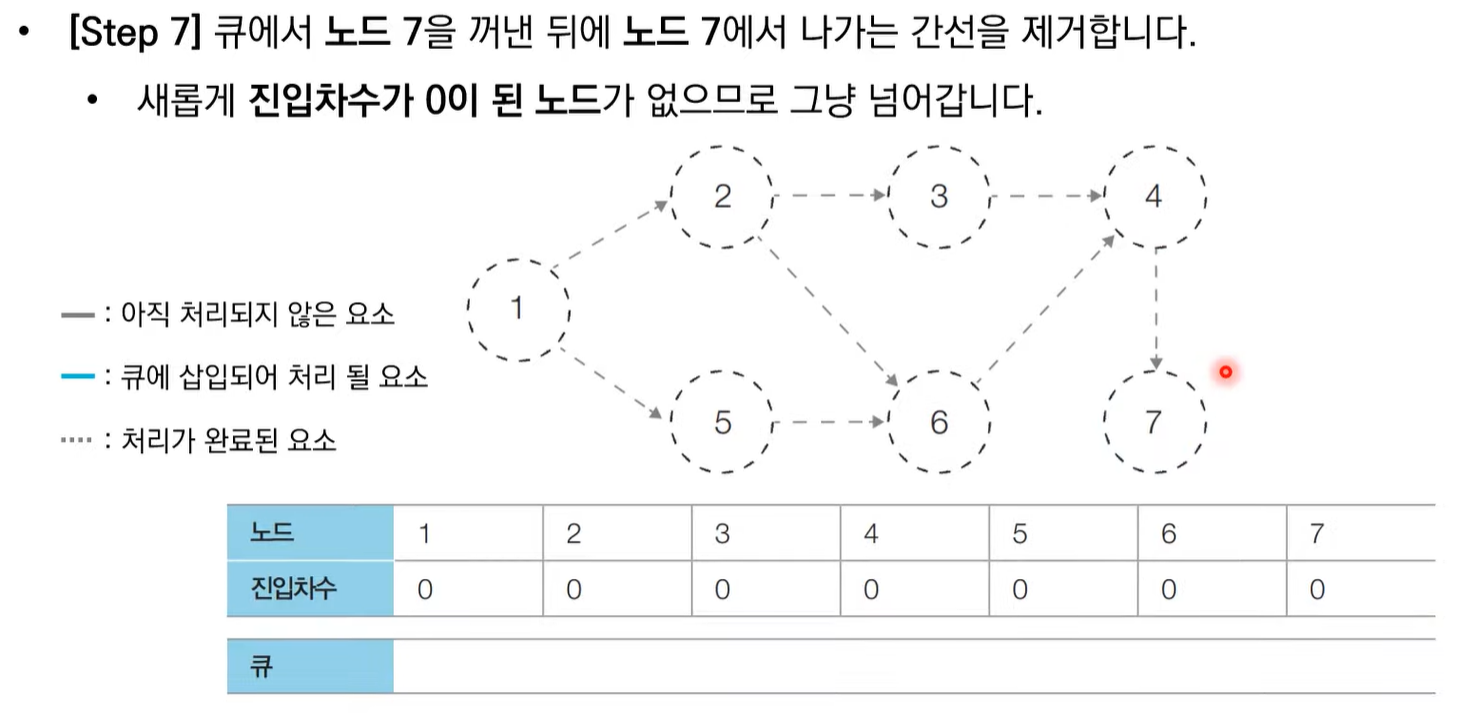
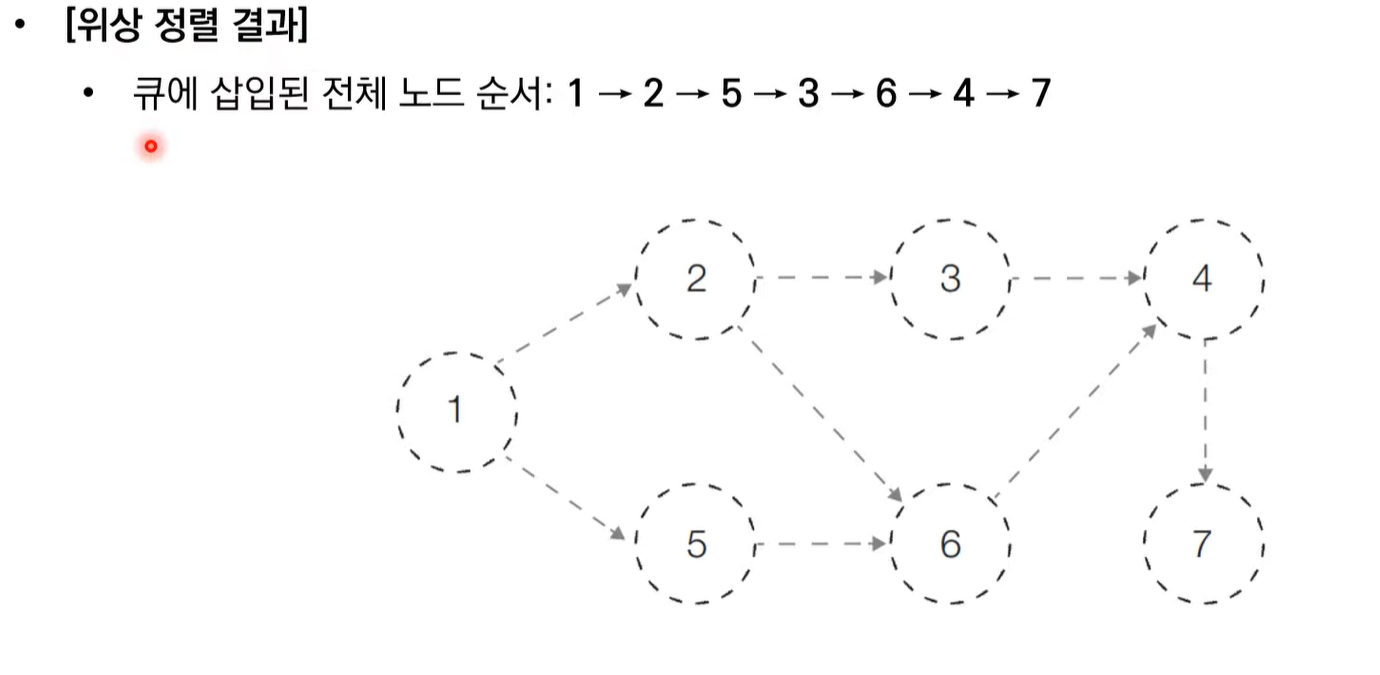
위상 정렬 특징
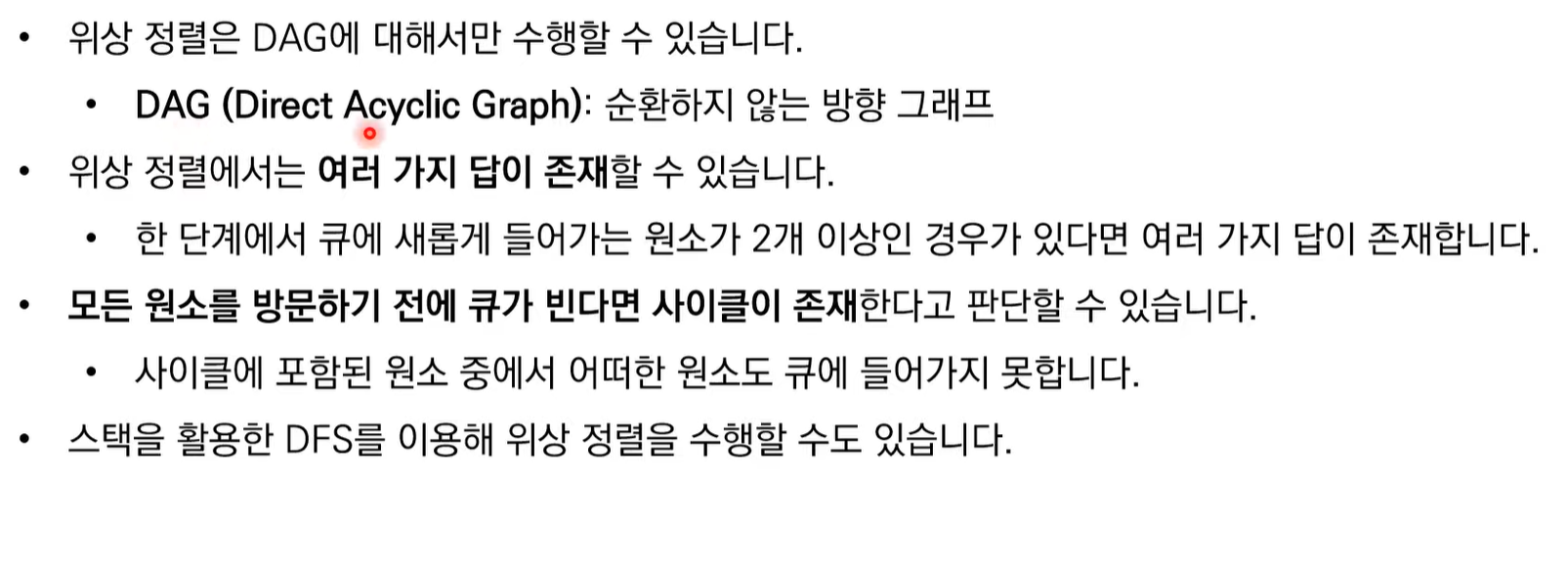
위상정렬 구현
from collections import deque
v,e = map(int,input().split())
indegree = [0] * (v+1)
graph = [[] for _ in range(v+1)]
for _ in range(e):
a,b = map(int,input().split())
graph[a].append(b)
indegree[b] += 1
def topology_sort():
result = []
q = deque()
for i in range(1,v+1):
if indegree[i] == 0:
q.append(i)
while q:
now = q.popleft()
result.append(now)
for i in graph[now]:
indegree[i] -= 1
if indegree[i] == 0:
q.append(i)
for i in result:
print(i,end = " ")
topology_sort()
위상 정렬 성능 분석
