Column
width 사용
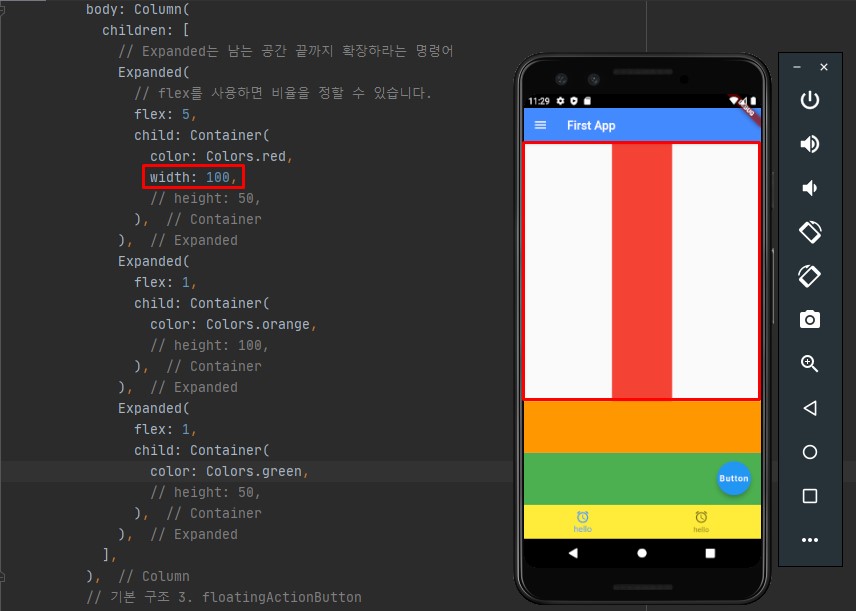
body: Column(
children: [
// Expanded는 남는 공간 끝까지 확장하라는 명령어
Expanded(
// flex를 사용하면 비율을 정할 수 있습니다.
flex: 5,
child: Container(
color: Colors.red,
width: 100,
// height: 50,
),
),
...
],
),
- width를 사용하면 너비를 지정할 수 있습니다.
- 나머지 영역이 부모의 최대 크기에 맞춰져 있으므로 red 영역은 가운데 정렬을 하게 됩니다.
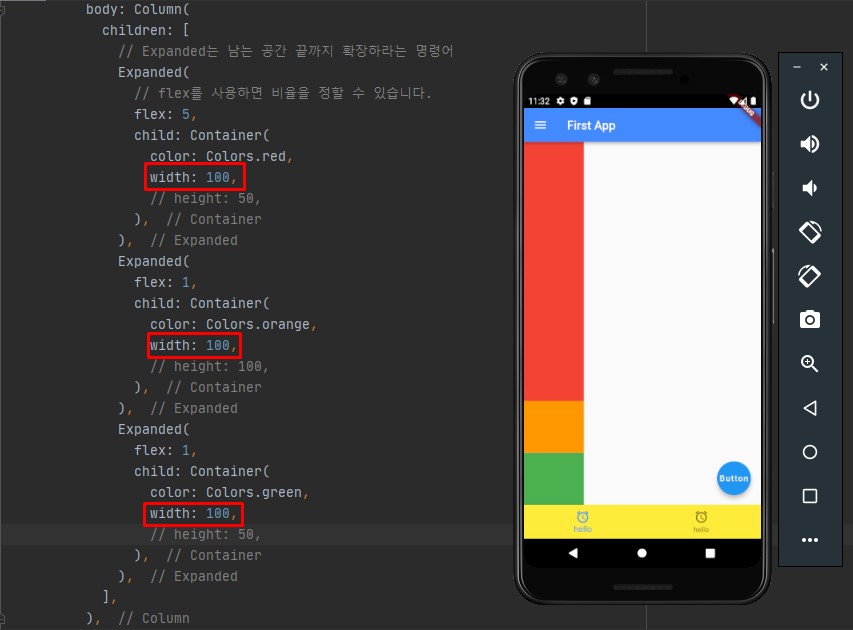
- 모두 width를 100으로 주면 정렬기준이 제일 왼쪽으로 줍니다.
- 하나라도 최대 크기까지 늘리면 다시 가운데 정렬이 됩니다.
- width가 모두 동일한 상태에서 가운데 정렬을 하고 싶을땐 Column에서 설정해야 합니다.
mainAxisAlignment를 이용한 세로 정렬
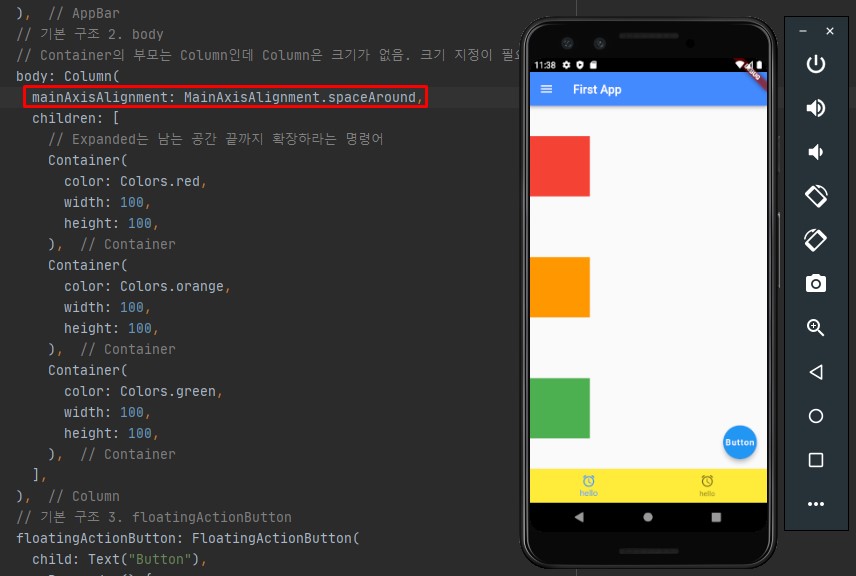
body: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
// Expanded는 남는 공간 끝까지 확장하라는 명령어
Container(
color: Colors.red,
width: 100,
height: 100,
),
Container(
color: Colors.orange,
width: 100,
height: 100,
),
Container(
color: Colors.green,
width: 100,
height: 100,
),
],
),
- Container의 Expanded에서 Alt + Enter를 입력해 Expanded(widget)을 제거합니다.
- Expanded를 제거했으므로 각 Container에 height 값을 지정해줍니다.
- Column에 mainAxisAlignment를 추가해줍니다.
- Column에서 mainAxisAlignment를 이용해서 세로 정렬 기준을 지정할 수 있습니다.
- spaceEvenly, spaceAround, spaceBetween, center 등을 이용해서 지정 가능합니다.
crossAxisAlignment를 이용한 가로 정렬
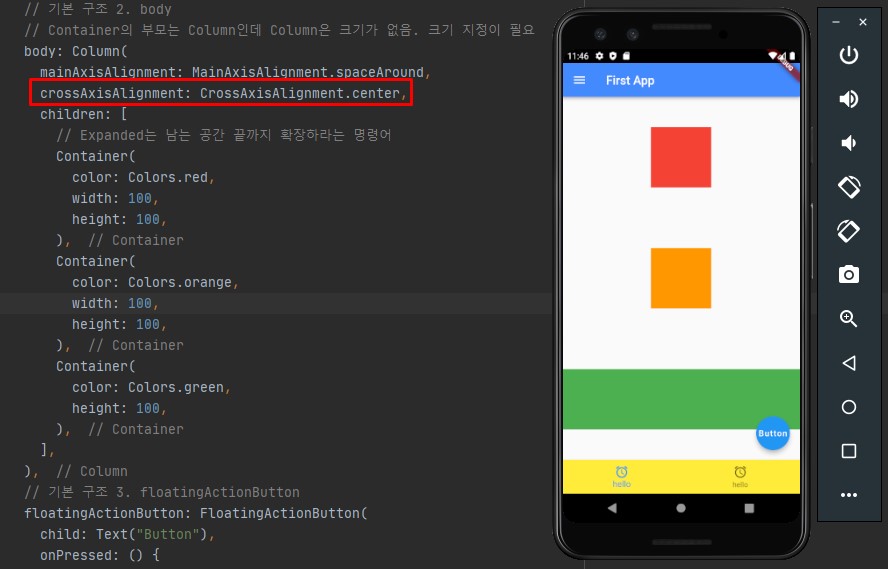
body: Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
// Expanded는 남는 공간 끝까지 확장하라는 명령어
Container(
color: Colors.red,
width: 100,
height: 100,
),
Container(
color: Colors.orange,
width: 100,
height: 100,
),
Container(
color: Colors.green,
height: 100,
),
],
),
- 우선 Container 하나의 width를 지워줍니다.
- Column에 crossAxisAlignment를 추가해줍니다.
- Column에서 crossAxisAlignment를 이용해서 가로 정렬 기준을 지정할 수 있습니다.
stretch(제약조건)
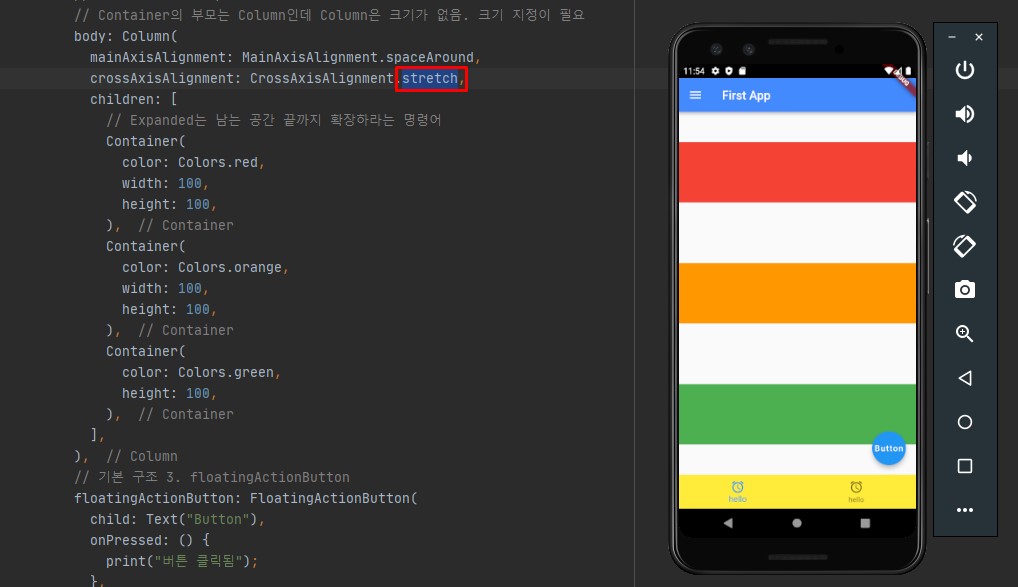
body: Column(
// 부모의 제약조건을 자식이 따라감
mainAxisAlignment: MainAxisAlignment.spaceAround,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
...
],
),
- stretch를 사용하면 Container의 width를 무시하고 전체 길이를 차지하게 됩니다.
- stretch는 컬럼에 있는 모든 요소를 끝가지 가게하라는 의미를 가집니다.
- 플러터는 부모의 크기에 맞게 자식이 따라갑니다. 즉 부모의 제약조건을 자식이 따라갑니다.
전체 코드
// ignore_for_file: prefer_const_literals_to_create_immutables, prefer_const_constructors
import 'package:flutter/material.dart';
// main 스레드는 runApp 을 실행시키고 종료됩니다.
void main() {
// 비동기로 실행됨(이벤트 루프에 등록된다)
runApp(FirstApp());
// sleep(Duration(seconds: 2));
// print("main 종료");
}
class FirstApp extends StatelessWidget {
const FirstApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
home: SafeArea(
child: Scaffold(
// 기본 구조 1. AppBar
appBar: AppBar(
backgroundColor: Colors.blueAccent,
title: Text("First App"),
leading: Icon(Icons.menu),
),
// 기본 구조 2. body
// Container의 부모는 Column인데 Column은 크기가 없음. 크기 지정이 필요
body: Column(
// 부모의 제약조건을 자식이 따라감
mainAxisAlignment: MainAxisAlignment.spaceAround,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
// Expanded는 남는 공간 끝까지 확장하라는 명령어
Container(
color: Colors.red,
width: 100,
// height: 100,
),
Container(
color: Colors.orange,
width: 100,
height: 100,
),
Container(
color: Colors.green,
height: 100,
),
],
),
// 기본 구조 3. floatingActionButton
floatingActionButton: FloatingActionButton(
child: Text("Button"),
onPressed: () {
print("버튼 클릭됨");
},
),
// 기본 구조 4. bottomNavigationBar
bottomNavigationBar: BottomNavigationBar(
items: [
BottomNavigationBarItem(
label: "hello", icon: Icon(Icons.access_alarm_rounded)),
BottomNavigationBarItem(
label: "hello", icon: Icon(Icons.access_alarm_rounded))
],
backgroundColor: Colors.yellow,
),
),
),
);
}
}