Light
struct LightColor
{
Vec4 diffuse;
Vec4 ambient;
Vec4 specular;
};
- Diffuse : 난반사
- ambient : 자연광
- specular : 정반사광
Green Directional Light
{
shared_ptr<GameObject> light = make_shared<GameObject>();
light->AddComponent(make_shared<Transform>());
light->AddComponent(make_shared<Light>());
light->GetLight()->SetLightDirection(Vec3(0.f, -1.f, 0.f));
light->GetLight()->SetLightType(LIGHT_TYPE::DIRECTIONAL_LIGHT);
light->GetLight()->SetDiffuse(Vec3(0.1f, 1.f, 0.1f));
light->GetLight()->SetAmbient(Vec3(0.f, 0.1f, 0.f));
light->GetLight()->SetSpecular(Vec3(0.1f, 0.1f, 0.1f));
scene->AddGameObject(light);
}
#pragma endregion
#pragma region Red Point Light
{
shared_ptr<GameObject> light = make_shared<GameObject>();
light->AddComponent(make_shared<Transform>());
light->GetTransform()->SetLocalPosition(Vec3(150.f, 150.f, 150.f));
light->AddComponent(make_shared<Light>());
light->GetLight()->SetLightType(LIGHT_TYPE::POINT_LIGHT);
light->GetLight()->SetDiffuse(Vec3(1.f, 0.1f, 0.1f));
light->GetLight()->SetAmbient(Vec3(0.1f, 0.f, 0.f));
light->GetLight()->SetSpecular(Vec3(0.1f, 0.1f, 0.1f));
light->GetLight()->SetLightRange(10000.f);
scene->AddGameObject(light);
}
#pragma endregion
#pragma region Blue Spot Light
{
shared_ptr<GameObject> light = make_shared<GameObject>();
light->AddComponent(make_shared<Transform>());
light->GetTransform()->SetLocalPosition(Vec3(-150.f, 0.f, 150.f));
light->AddComponent(make_shared<Light>());
light->GetLight()->SetLightDirection(Vec3(1.f, 0.f, 0.f));
light->GetLight()->SetLightType(LIGHT_TYPE::SPOT_LIGHT);
light->GetLight()->SetDiffuse(Vec3(0.f, 0.1f, 1.f));
light->GetLight()->SetSpecular(Vec3(0.1f, 0.1f, 0.1f));
light->GetLight()->SetLightRange(10000.f);
light->GetLight()->SetLightAngle(XM_PI / 4);
scene->AddGameObject(light);
}
- 초록색 Directional Light
- 빨간색 Point Light
- 파란색 Spot Light
- 각각의 빛의 Position과 방향, diffuse, ambient, specular 값을 지정해주고 Object에 추가해준다.
color.xyz = (totalColor.diffuse.xyz * color.xyz)
+ totalColor.ambient.xyz * color.xyz
+ totalColor.specular.xyz;
- Shader 코드를 분석해보면 diffuse와 ambient는 Color값과 관련이 있기 때문에 Color와 곱을 하고, specular의 경우 반사 각에 따른 반짝임(?)을 표현하는 값이기 때문에 Color를 곱하지 않고 적용한다.
실습
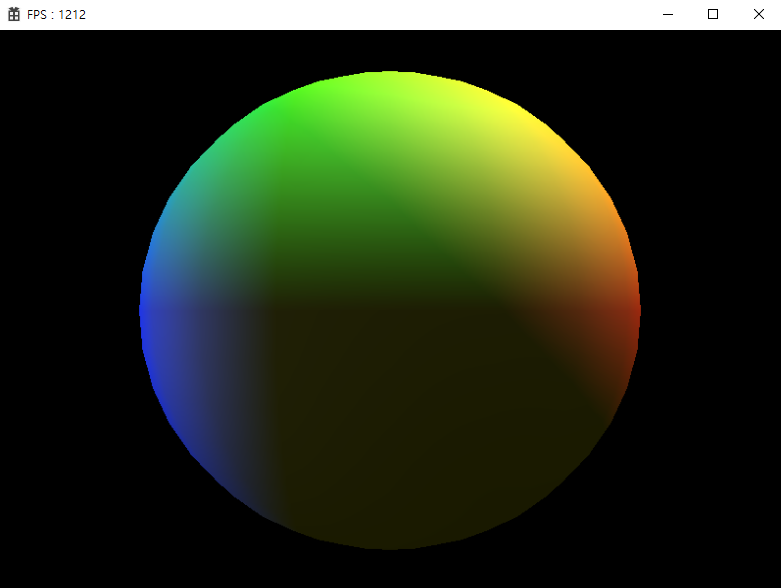
- 위 코드가 적용된 모습이다.
- 위에서 초록빛이 직선으로 쬐어지고 있고, 왼쪽에선 파란 SpotLight 그리고 오른쪽에선 빨간 Point Light가 쬐어지고 있다.
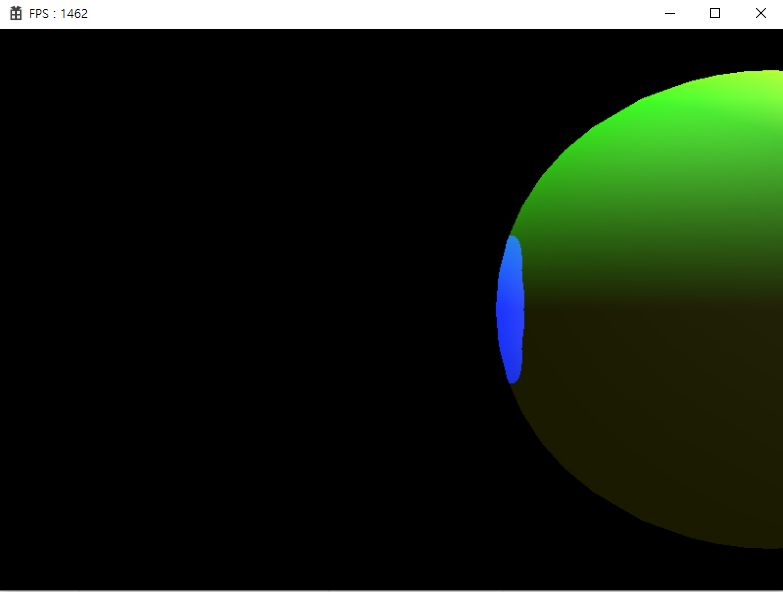
- 위 코드에서 파란색 SpotLight의 Angle를 줄여준 모습이다.
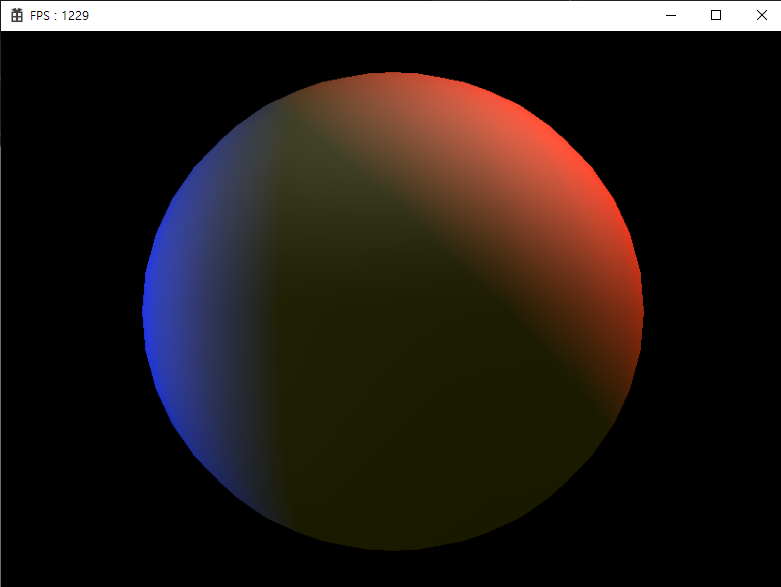
- 위 코드에서 Directional Light RGB 값을 0.1f,0.1f,0.1f 로 바꿔준 모습이다.