◾데이터 나누기(Decision Tree)
1. 과적합
과적합(Overfitting)
: 기계 학습(machine learning)에서 학습 데이터를 과하게 학습(overfitting)하는 것을 뜻한다. 일반적으로 학습 데이타는 실제 데이타의 부분 집합이므로 학습데이타에 대해서는 오차가 감소하지만 실제 데이터에 대해서는 오차가 증가하게 된다.
지도 학습
: 학습 대상이 되는 데이터에 정답을 붙여서 학습시키고 모델을 얻어 완전히 새로운 데이터에 대한 '답'을 얻고자 하는 것
- 이전 포스트에서 만든 Decision Tree를 확인하면 복잡한 규칙으로 이루어진 것을 볼 수 있다.
from sklearn.tree import plot_tree
plt.figure(figsize=(12, 8))
plot_tree(iris_tree)
plt.show()
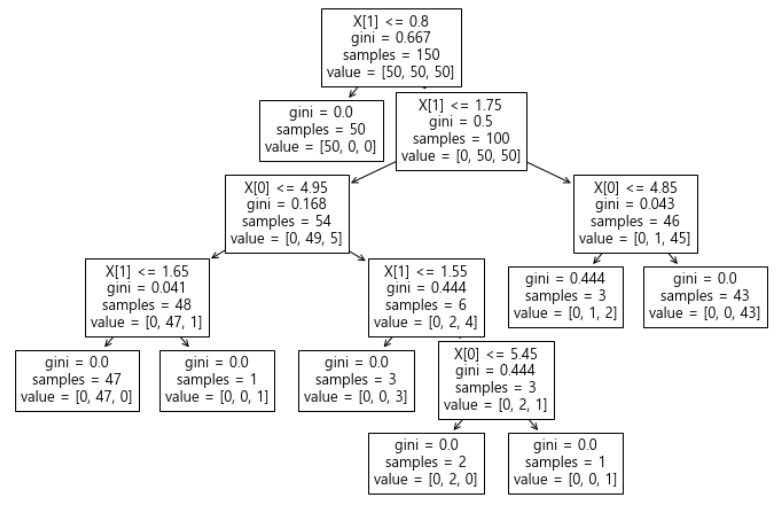
- mlxtend : sklearn에 없는 몇몇 기능을 가지고 있다.
from mlxtend.plotting import plot_decision_regions
plt.figure(figsize=(14, 8))
plot_decision_regions(X=iris.data[:, 2:], y=iris.target, clf=iris_tree, legend=2)
plt.show()
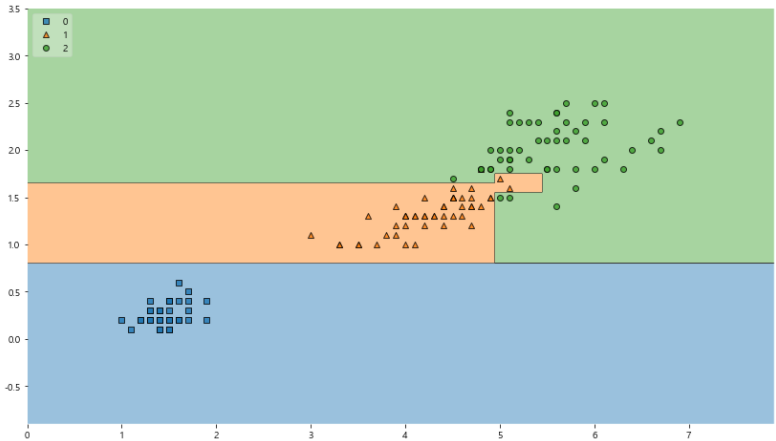
- 조건이 복잡하게 결정된 것을 알 수 있다.
- 모든 IRIS에 대해 맞는 경계선을 가질 수 있는가?
- 이 결과를 내가 가진 데이터를 벗어나서 일반화할 수 있는가
- 얻은 데이터는 유한하고 얻은 데이터를 이용해 일반화를 추구하게 된다.
- 복잡한 경계면은 모델의 성능을 결국 나쁘게 만든다.
2. 데이터 분리(나누기)
- 데이터의 분리 : 훈련(Training), 검증(Validation), 평가(Testing)
- 확보한 데이터 중에서 모델 학습에 사용하지않고 빼둔 데이터를 이용해 모델 테스트
import pandas as pd
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
iris = load_iris()
features = iris.data[:, 2:]
labels = iris.target
X_train, X_test, Y_train, Y_test = train_test_split(features, labels,
test_size=0.2,
stratify=labels,
random_state=13)
iris_tree = DecisionTreeClassifier(max_depth=2, random_state=13)
iris_tree.fit(X_train, Y_train)
import matplotlib.pyplot as plt
from sklearn.tree import plot_tree
plt.figure(figsize=(12, 10))
plot_tree(iris_tree)
plt.show()
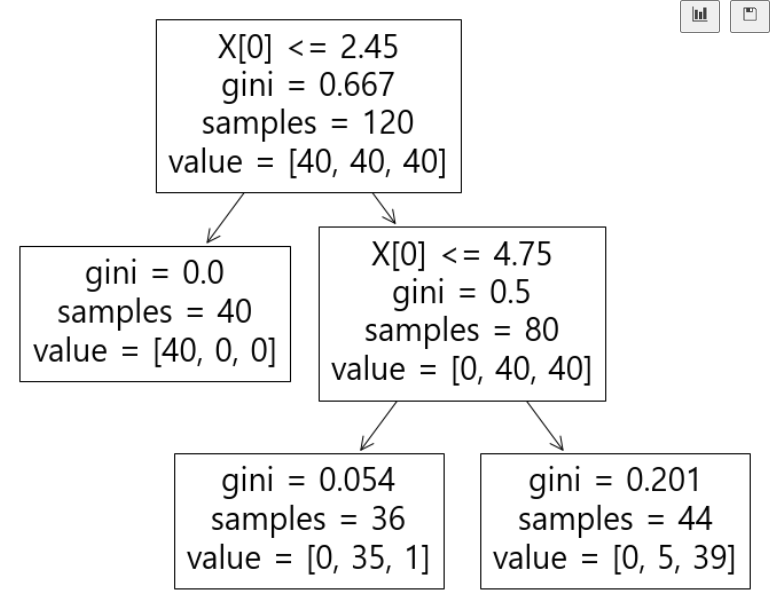
from sklearn.metrics import accuracy_score
y_pred_tr = iris_tree.predict(X_train)
accuracy_score(Y_train, y_pred_tr)

import matplotlib.pyplot as plt
from mlxtend.plotting import plot_decision_regions
plt.figure(figsize=(12, 8))
plot_decision_regions(X=X_train, y=Y_train, clf=iris_tree, legend=2)
plt.show()
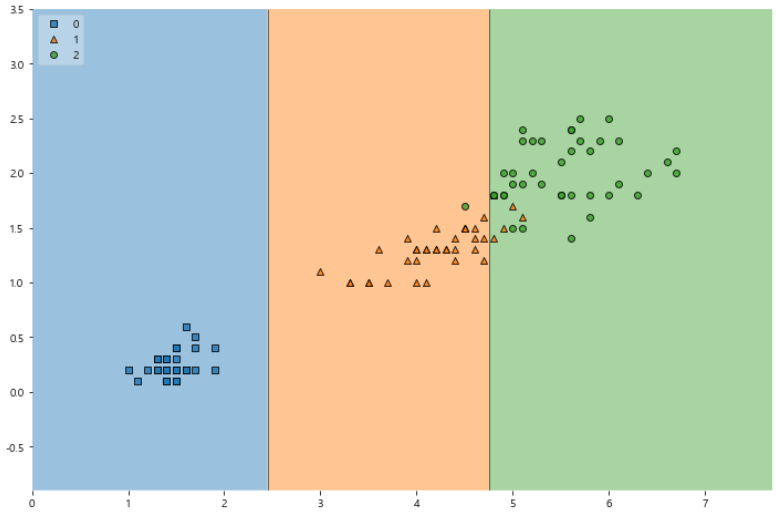
y_pred_test = iris_tree.predict(X_test)
accuracy_score(Y_test, y_pred_test)

import matplotlib.pyplot as plt
from mlxtend.plotting import plot_decision_regions
scatter_highlight_kwargs = {'s' : 150, 'label' : 'Test data', 'alpha' : 0.9}
scatter_kwargs = {'s' : 120, 'edgecolor' : None, 'alpha' : 0.9}
plt.figure(figsize=(12, 8))
plot_decision_regions(X=features, y=labels,
X_highlight=X_test, clf=iris_tree, legend=2,
scatter_highlight_kwargs = scatter_highlight_kwargs,
scatter_kwargs = scatter_kwargs,
contourf_kwargs={'alpha' : 0.2}
)
plt.show()
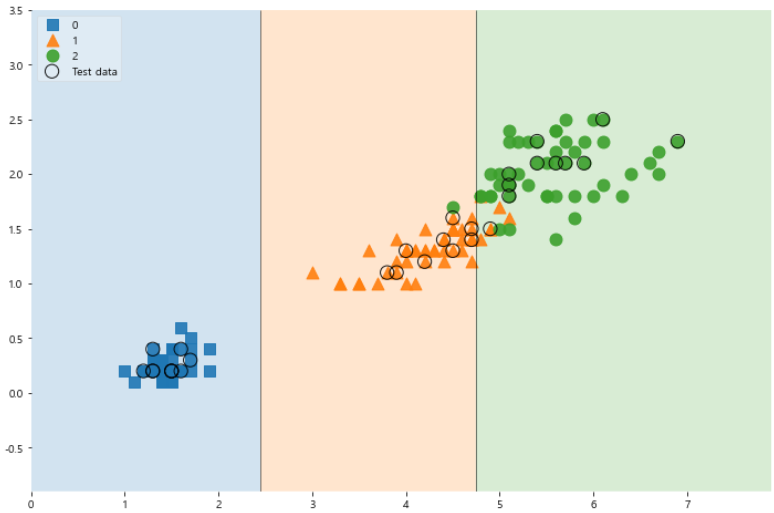
features = iris.data
labels = iris.target
x_train, x_test, y_train, y_test = train_test_split(features, labels,
test_size = 0.2,
stratify = labels,
random_state=13)
iris_tree = DecisionTreeClassifier(max_depth = 2, random_state = 13)
iris_tree.fit(x_train, y_train)
plt.figure(figsize=(12, 10))
plot_tree(iris_tree)
plt.show()
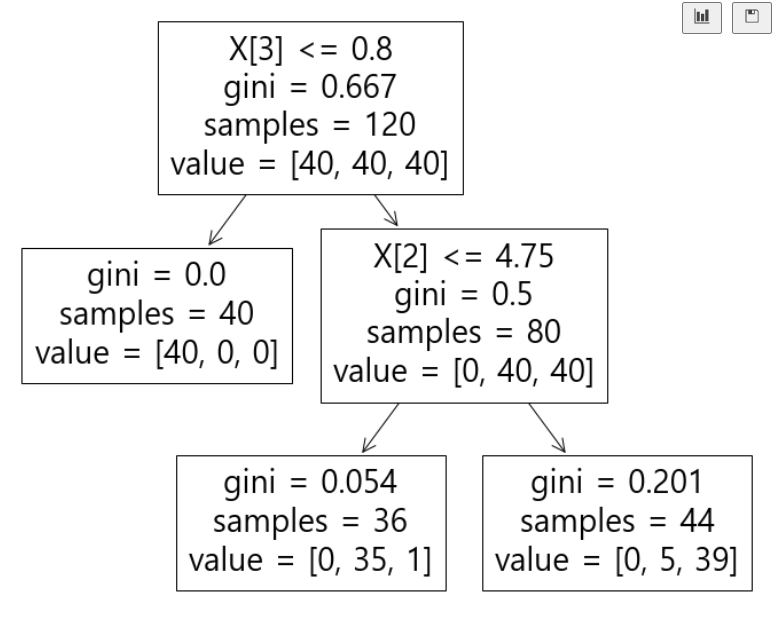
- 구현한 모델 테스트
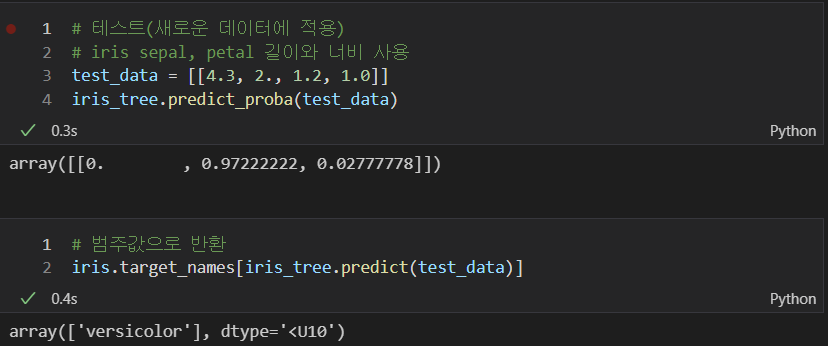
- 구현 모델의 특성
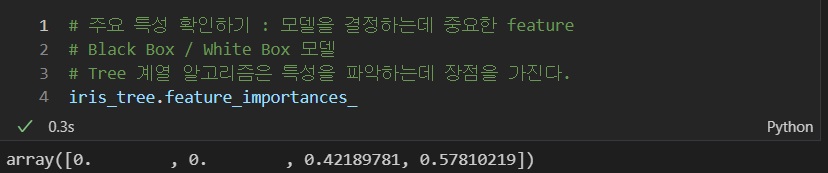