pandas :: Dataframe Operations
Series operation
import pandas as pd
from pandas import Series
from pandas import DataFrame
import numpy as np
s1 = Series(range(1,6), index=list("abcde"))
s1
a 1
b 2
c 3
d 4
e 5
dtype: int64
s2 = Series(range(5, 11), index=list("cdefgh"))
s2
c 5
d 6
e 7
f 8
g 9
h 10
dtype: int64
s1.add(s2)
a NaN
b NaN
c 8.0
d 10.0
e 12.0
f NaN
g NaN
h NaN
dtype: float64
s1 + s2
a NaN
b NaN
c 8.0
d 10.0
e 12.0
f NaN
g NaN
h NaN
dtype: float64
Dataframe operation
df1 = DataFrame(np.arange(9).reshape(3,3), columns=list("abc"))
df1
|
a |
b |
c |
0 |
0 |
1 |
2 |
1 |
3 |
4 |
5 |
2 |
6 |
7 |
8 |
df2 = DataFrame(np.arange(16).reshape(4,4), columns=list("abcd"))
df2
|
a |
b |
c |
d |
0 |
0 |
1 |
2 |
3 |
1 |
4 |
5 |
6 |
7 |
2 |
8 |
9 |
10 |
11 |
3 |
12 |
13 |
14 |
15 |
df1 + df2
|
a |
b |
c |
d |
0 |
0.0 |
2.0 |
4.0 |
NaN |
1 |
7.0 |
9.0 |
11.0 |
NaN |
2 |
14.0 |
16.0 |
18.0 |
NaN |
3 |
NaN |
NaN |
NaN |
NaN |
df1.add(df2, fill_value=0)
|
a |
b |
c |
d |
0 |
0.0 |
2.0 |
4.0 |
3.0 |
1 |
7.0 |
9.0 |
11.0 |
7.0 |
2 |
14.0 |
16.0 |
18.0 |
11.0 |
3 |
12.0 |
13.0 |
14.0 |
15.0 |
df1.add(df2, fill_value=2)
|
a |
b |
c |
d |
0 |
0.0 |
2.0 |
4.0 |
5.0 |
1 |
7.0 |
9.0 |
11.0 |
9.0 |
2 |
14.0 |
16.0 |
18.0 |
13.0 |
3 |
14.0 |
15.0 |
16.0 |
17.0 |
Series + Dataframe
df = DataFrame(np.arange(16).reshape(4,4), columns=list("abcd"))
df
|
a |
b |
c |
d |
0 |
0 |
1 |
2 |
3 |
1 |
4 |
5 |
6 |
7 |
2 |
8 |
9 |
10 |
11 |
3 |
12 |
13 |
14 |
15 |
s = Series(np.arange(10,14), index=list("abcd"))
s
a 10
b 11
c 12
d 13
dtype: int32
df + s
|
a |
b |
c |
d |
0 |
10 |
12 |
14 |
16 |
1 |
14 |
16 |
18 |
20 |
2 |
18 |
20 |
22 |
24 |
3 |
22 |
24 |
26 |
28 |
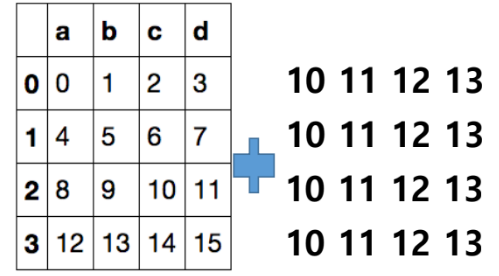
s2 = Series(np.arange(10,14))
s2
0 10
1 11
2 12
3 13
dtype: int32
df + s2
|
a |
b |
c |
d |
0 |
1 |
2 |
3 |
0 |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
1 |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
2 |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
3 |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
NaN |
df.add(s2, axis=0)
|
a |
b |
c |
d |
0 |
10 |
11 |
12 |
13 |
1 |
15 |
16 |
17 |
18 |
2 |
20 |
21 |
22 |
23 |
3 |
25 |
26 |
27 |
28 |
https://www.boostcourse.org/ai222/lecture/23822