hihello
Hihello는 무엇인지
- hihello를 예측하는 모델을 만들려고 함. 하나의 알파벳이 주어질 때 다음 문자를 예측할 수 있도록 하는 RNN 모델을 만들려고 함.
Data Setting(one-hot encoding)
- 벡터의 하나의 축에서만 1로 표현되고 나머지부분은 0으로 표현되는 방식으로 벡터의 차원의 수는 알파벳의 종류이다. 입력할때는 출력될 부분과 입력될부분을 나눠서 인덱스를 표현한 데이터를 넣어준다.
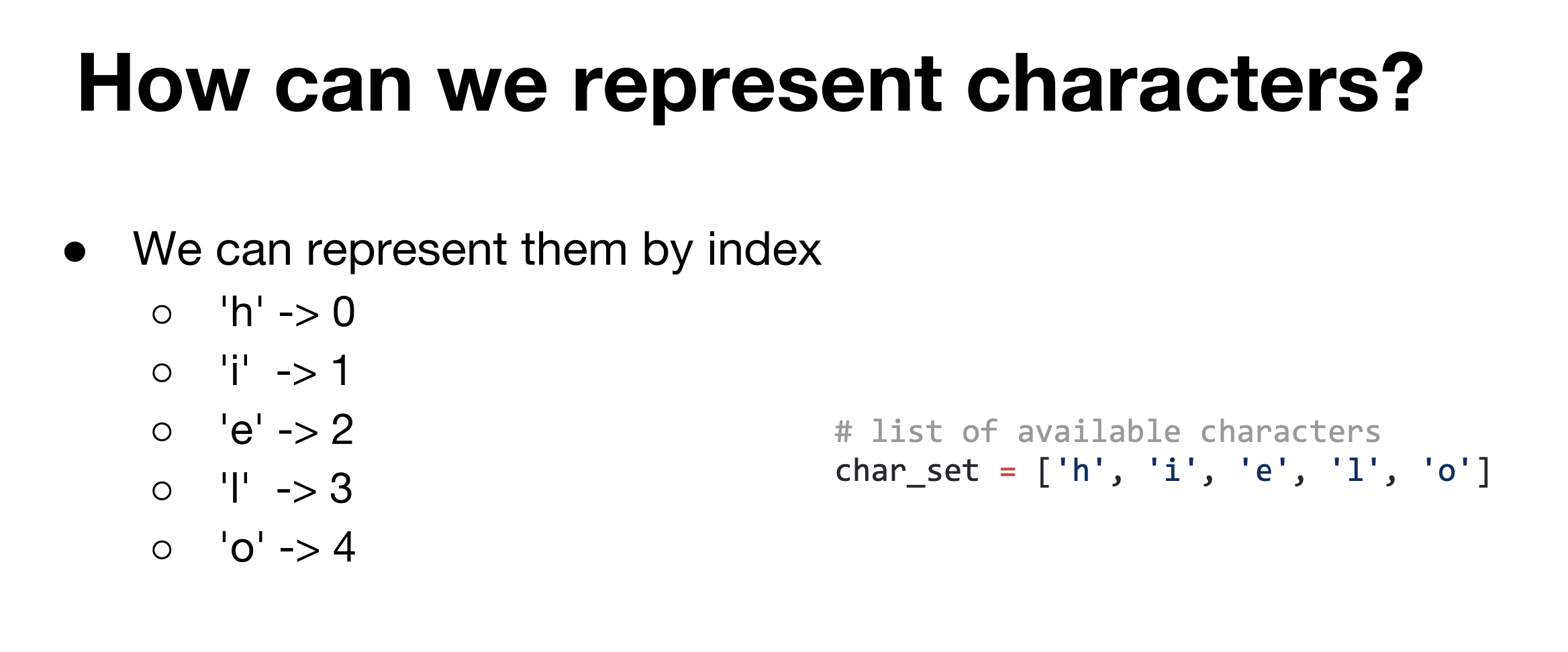
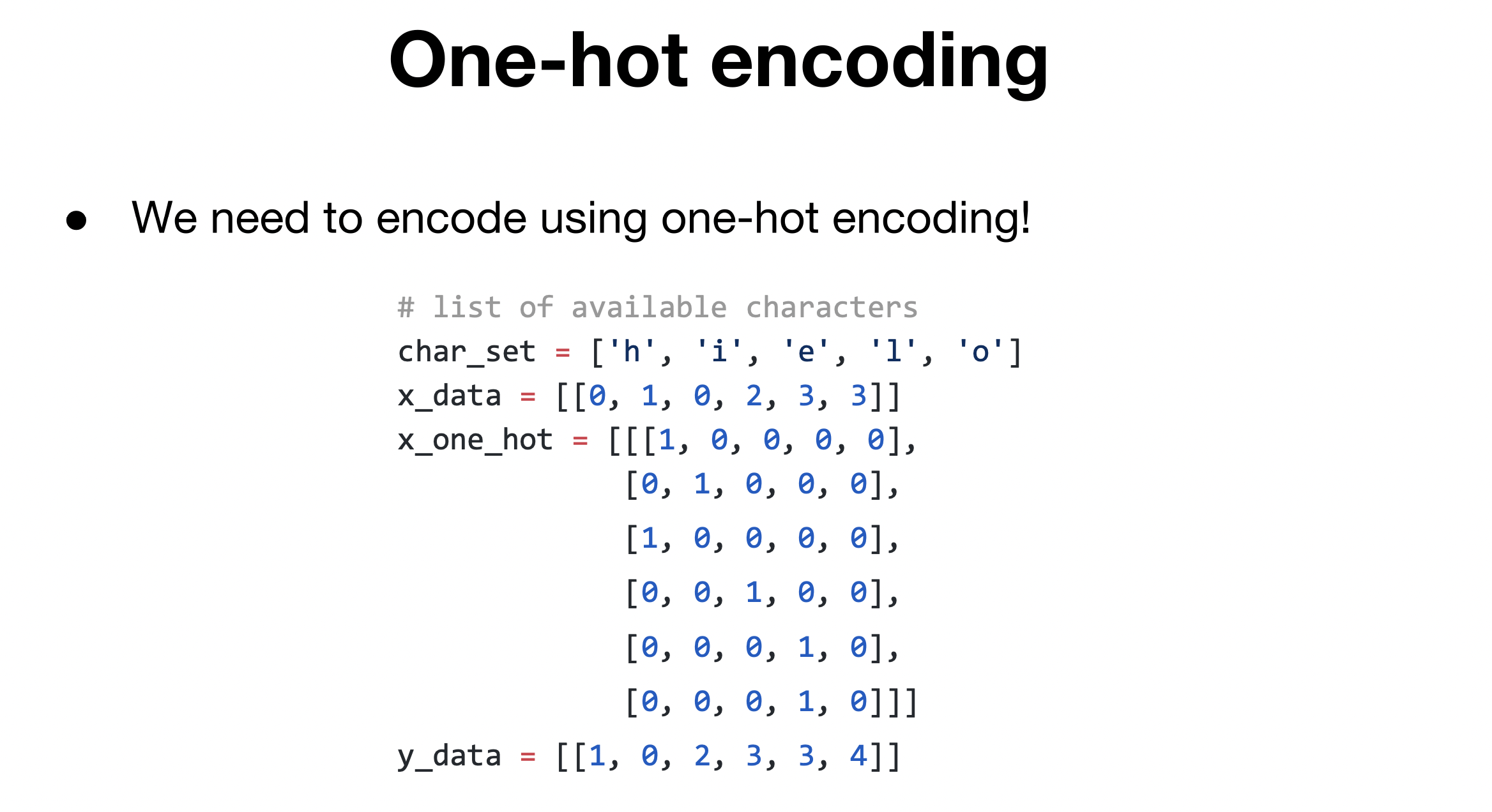
Cross Entropy Loss
- categorical 한 output을 출력할 때 사용하는 손실함수
- criterion(output,label) : 첫 번째 인자에서는 모델의 output, 두번째인자는 정답 label을 줌
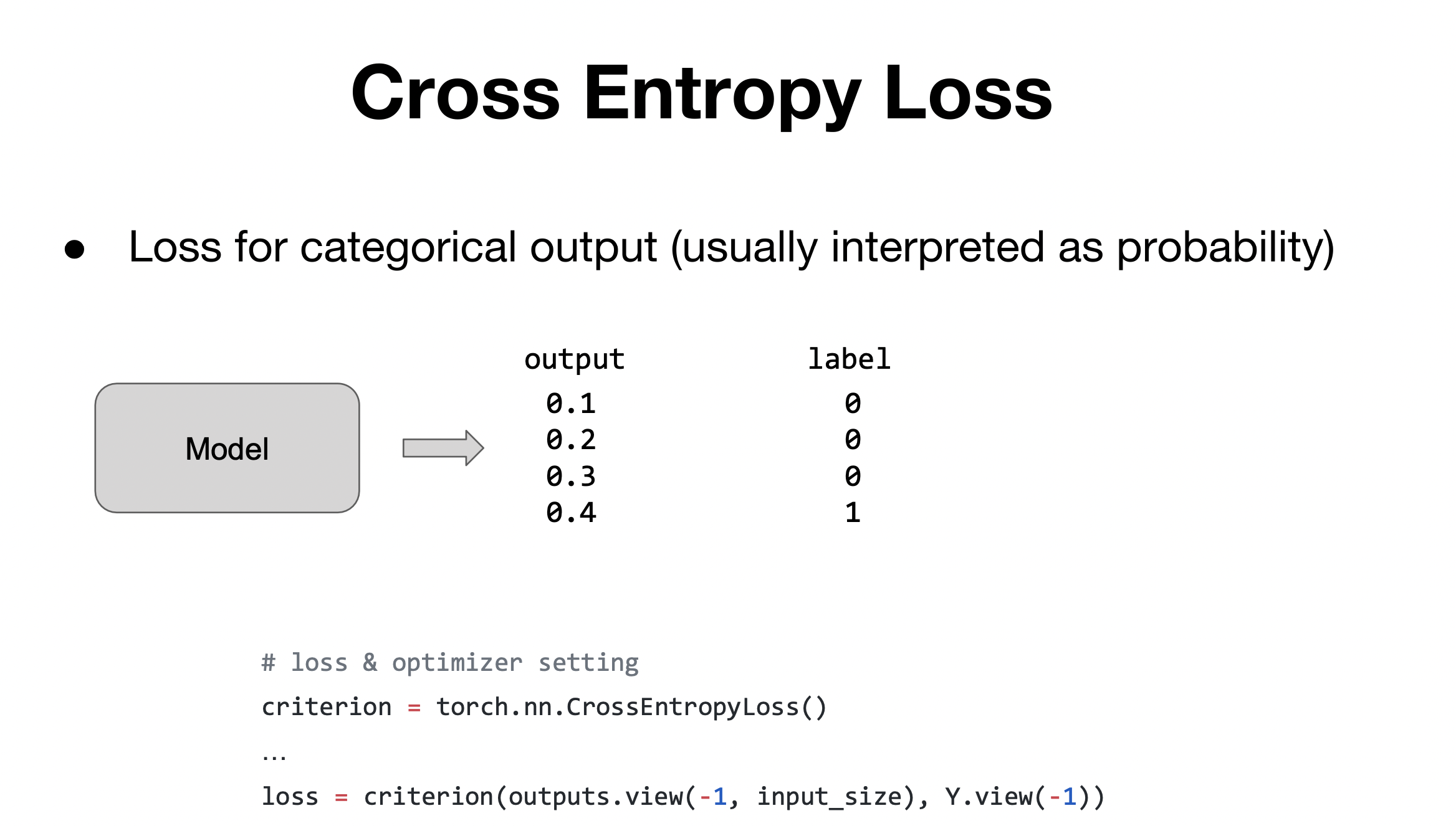
Code run through
import torch
import torch.optim as optim
import numpy as np
torch.manual_seed(0)
char_set = ['h', 'i', 'e', 'l', 'o']
input_size = len(char_set)
hidden_size = len(char_set)
learning_rate = 0.1
x_data = [[0, 1, 0, 2, 3, 3]]
x_one_hot = [[[1, 0, 0, 0, 0],
[0, 1, 0, 0, 0],
[1, 0, 0, 0, 0],
[0, 0, 1, 0, 0],
[0, 0, 0, 1, 0],
[0, 0, 0, 1, 0]]]
y_data = [[1, 0, 2, 3, 3, 4]]
X = torch.FloatTensor(x_one_hot)
Y = torch.LongTensor(y_data)
rnn = torch.nn.RNN(input_size, hidden_size, batch_first=True)
criterion = torch.nn.CrossEntropyLoss()
optimizer = optim.Adam(rnn.parameters(), learning_rate)
for i in range(100):
optimizer.zero_grad()
outputs, _status = rnn(X)
loss = criterion(outputs.view(-1, input_size), Y.view(-1))
loss.backward()
optimizer.step()
result = outputs.data.numpy().argmax(axis=2)
result_str = ''.join([char_set[c] for c in np.squeeze(result)])
print(i, "loss: ", loss.item(), "prediction: ", result, "true Y: ", y_data, "prediction str: ", result_str)
charseq
- hihello 보다 일반적인 단어의 예측 모델이며, hihello코드를 보다 일반화한 코드라고 생각하면 됨. 어떠한 sample이 들어와도 다음을 예측할 수 있음
- np.eye 간단하게 원하는 크기의 identity 메트릭스를 만들어줌.
import torch
import torch.optim as optim
import numpy as np
torch.manual_seed(0)
sample = " if you want you"
char_set = list(set(sample))
char_dic = {c: i for i, c in enumerate(char_set)}
print(char_dic)
dic_size = len(char_dic)
hidden_size = len(char_dic)
learning_rate = 0.1
sample_idx = [char_dic[c] for c in sample]
x_data = [sample_idx[:-1]]
x_one_hot = [np.eye(dic_size)[x] for x in x_data]
y_data = [sample_idx[1:]]
rnn = torch.nn.RNN(dic_size, hidden_size, batch_first=True)
criterion = torch.nn.CrossEntropyLoss()
optimizer = optim.Adam(rnn.parameters(), learning_rate)
for i in range(50):
optimizer.zero_grad()
outputs, _status = rnn(X)
loss = criterion(outputs.view(-1, dic_size), Y.view(-1))
loss.backward()
optimizer.step()
result = outputs.data.numpy().argmax(axis=2)
result_str = ''.join([char_set[c] for c in np.squeeze(result)])
print(i, "loss: ", loss.item(), "prediction: ", result, "true Y: ", y_data, "prediction str: ", result_str)