- Bare Minimum, Advanced, Nightmare 포함! 내가 만든 계산기에 적용시키기!
- 주석 참고
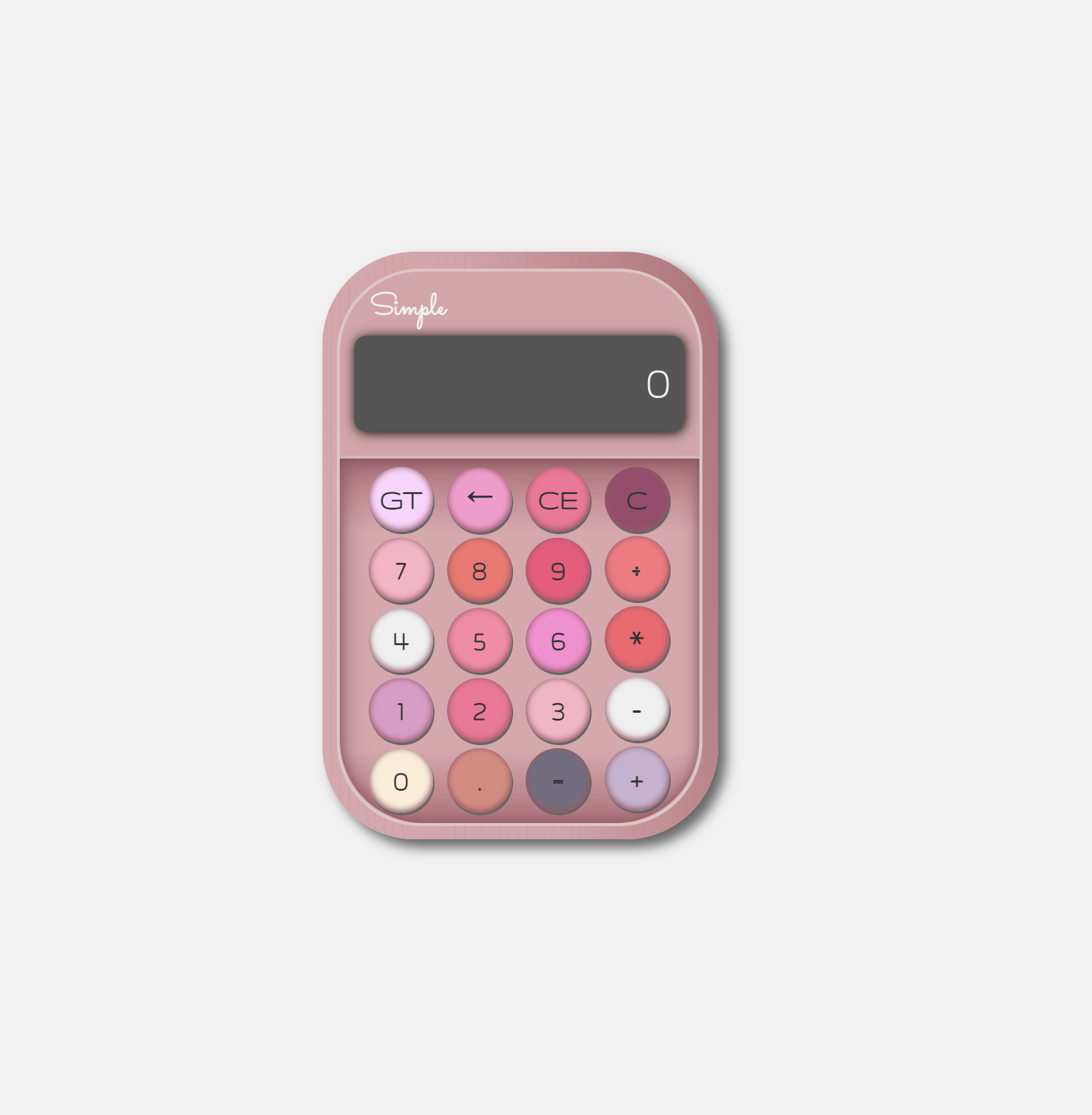
- 동영상은 어떻게 첨부하는걸까...
- 그 대신 노션에 구현 동영상 올려둔거 첨부합니다! 혹시라도 보시는 분 계시면 참고하시기 바랍니다!
구현 동영상
const calculator = document.querySelector(".calculator");
const buttons = calculator.querySelector(".calculator__buttons");
const firstOperend = document.querySelector(".calculator__operend--left");
const operator = document.querySelector(".calculator__operator");
const secondOperend = document.querySelector(".calculator__operend--right");
const calculatedResult = document.querySelector(".calculator__result");
function calculate(n1, operator, n2) {
let result = 0;
if (operator === "+") result = parseFloat(n1) + parseFloat(n2);
else if (operator === "-") result = parseFloat(n1) - parseFloat(n2);
else if (operator === "*") result = parseFloat(n1) * parseFloat(n2);
else if (operator === "/") result = parseFloat(n1) / parseFloat(n2);
return String(result);
}
buttons.addEventListener("click", function (event) {
const target = event.target;
const action = target.classList[0];
const buttonContent = target.textContent;
if (target.matches("button")) {
if (action === "number") {
if (firstOperend.textContent === "0") {
firstOperend.textContent = buttonContent;
} else {
secondOperend.textContent = buttonContent;
}
console.log("숫자 " + buttonContent + " 버튼");
}
if (action === "operator") {
console.log("연산자 " + buttonContent + " 버튼");
operator.textContent = buttonContent;
}
if (action === "decimal") {
console.log("소수점 버튼");
}
if (action === "clear") {
console.log("초기화 버튼");
firstOperend.textContent = "0";
secondOperend.textContent = "0";
operator.textContent = "+";
calculatedResult.textContent = "0";
}
if (action === "calculate") {
calculatedResult.textContent = calculate(
firstOperend.textContent,
operator.textContent,
secondOperend.textContent
);
console.log("계산 버튼");
}
}
});
const display = document.querySelector(".calculator__display--for-advanced");
let firstNum, operatorForAdvanced, previousKey, previousNum;
buttons.addEventListener("click", function (event) {
const target = event.target;
const action = target.classList[0];
const buttonContent = target.textContent;
if (target.matches("button")) {
if (action === "number") {
if (display.textContent === "0" || previousKey === "operator") {
display.textContent = buttonContent;
} else {
display.textContent += buttonContent;
}
previousKey = "number";
}
if (action === "operator") {
if (
firstNum &&
operatorForAdvanced &&
previousKey !== "calculate" &&
previousKey !== "operator"
) {
display.textContent = calculate(
firstNum,
operatorForAdvanced,
display.textContent
);
}
firstNum = display.textContent;
operatorForAdvanced = buttonContent;
previousKey = "operator";
}
if (action === "decimal") {
if (!display.textContent.includes(".") && previousKey !== "operator") {
display.textContent += ".";
} else if (previousKey === "operator") {
display.textContent = "0.";
}
previousKey = "decimal";
}
if (action === "clear") {
firstNum = undefined;
display.textContent = "0";
operatorForAdvanced = undefined;
previousKey = "clear";
}
if (action === "calculate") {
if (operatorForAdvanced) {
if (previousKey === "calculate") {
display.textContent = calculate(
display.textContent,
operatorForAdvanced,
previousNum
);
} else {
previousNum = display.textContent;
display.textContent = calculate(
firstNum,
operatorForAdvanced,
display.textContent
);
}
}
previousKey = "calculate";
}
}
});