1. (entity) Product
@Table
@Entity
@ToString
@Getter
@Setter
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column
private String name;
@Column
private Long price;
public Product() {
}
}
2. (dto) Product
public class Product {
private final String name;
private final Long price;
public Product(String name, Long price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public Long getPrice() {
return price;
}
}
3. ProductJpaRepository
public interface ProductJpaRepository extends JpaRepository<Product, Long> {
}
1. 상품 등록 API - POST
- ProductController
@PostMapping("api/products")
public String saveProduct(@RequestBody com.example.springboot.entity.Product product) {
productService.save(product);
return "정상 저장됐습니다.";
}
- ProductService
public void save(com.example.springboot.Products.Product product) {
productJpaRepository.save(product);
}
2. 상품 조회 API - GET
- ProductController
@GetMapping("api/products")
public List<com.example.springboot.entity.Product> findAll() { return productService.findAll(); }
- ProductService
public List<com.example.springboot.entity.Product> findAll() {
return productJpaRepository.findAll();
}
3 상품 삭제 API - DELETE
- ProductController
@DeleteMapping("api/products/{id}")
public String delete(@PathVariable Long id) {
productService.delete(id);
return "삭제되었습니다.";
}
- ProductService
public void delete(Long id) {
productJpaRepository.deleteById(id);
}
2. Postman으로 확인하기
http://localhost:8080/api/products
GET, POST, DELETE 모두 동일
1. POST
- POST > Body > JSON > 이름, 가격 입력 > SEND
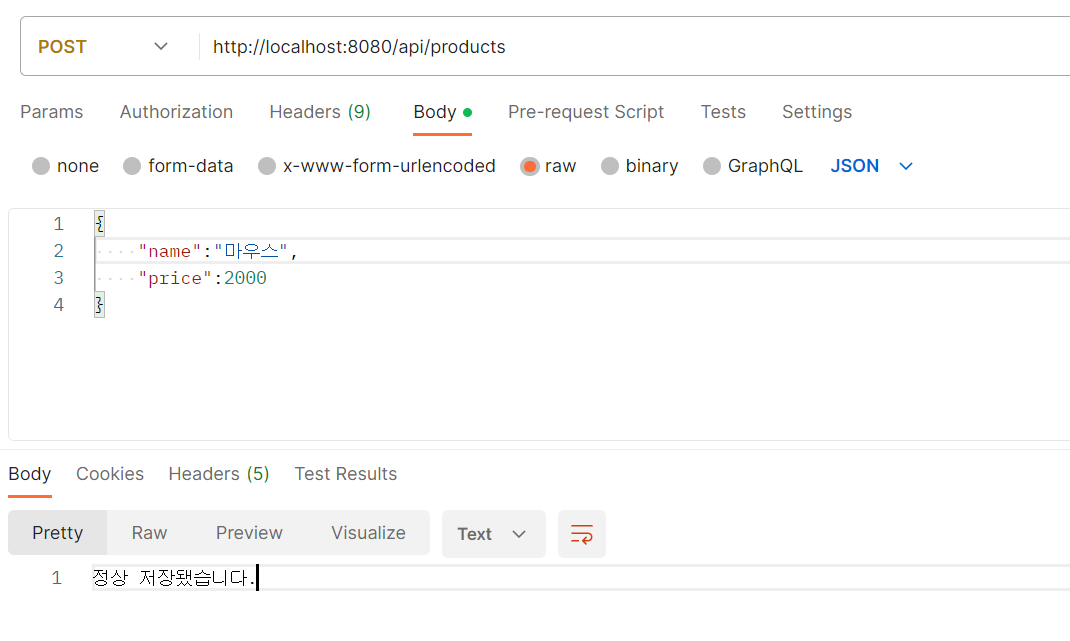
2. GET
- 저장한 상품을 조회할 수 있다.
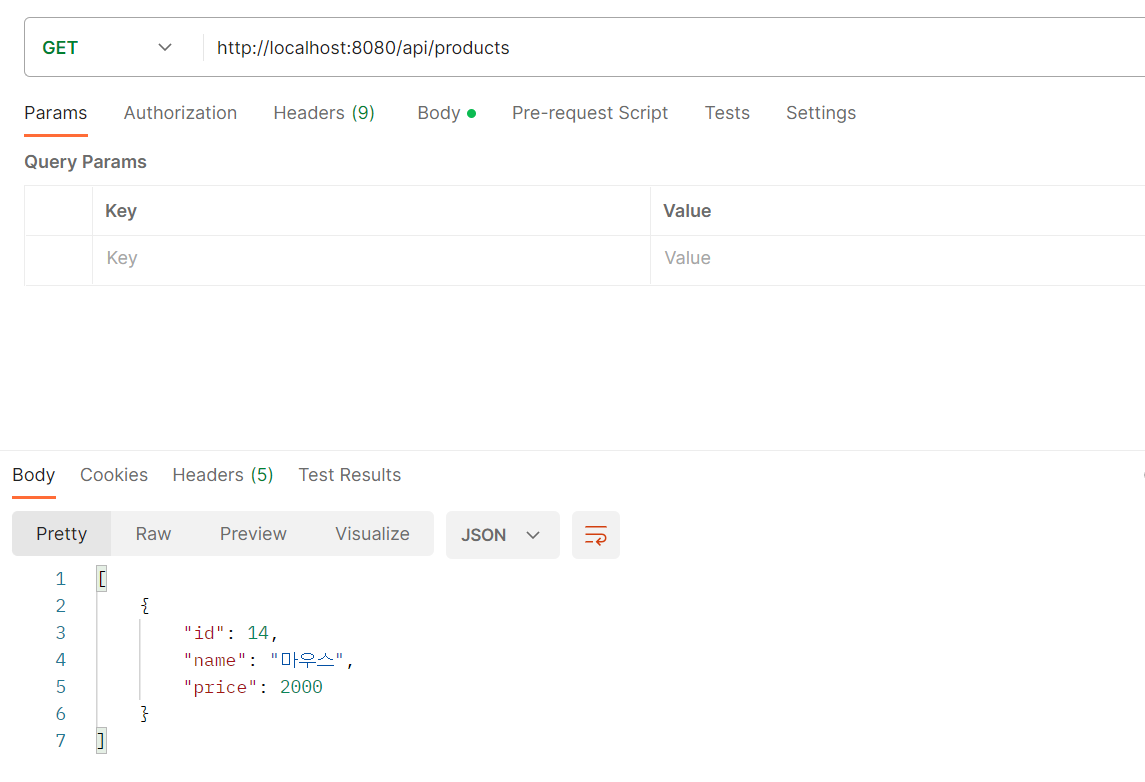
3. DELETE
http://localhost:8080/api/products
+ 상품 ID : 저장한 상품 삭제 가능
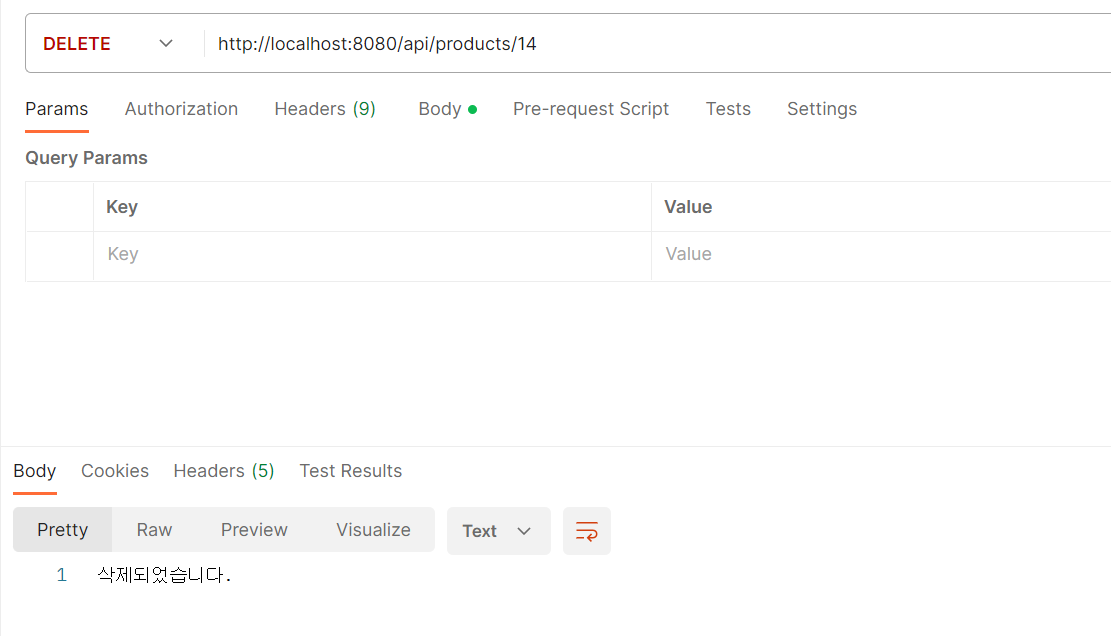
- GET을 이용해 삭제가 됐는지 확인해보았다.
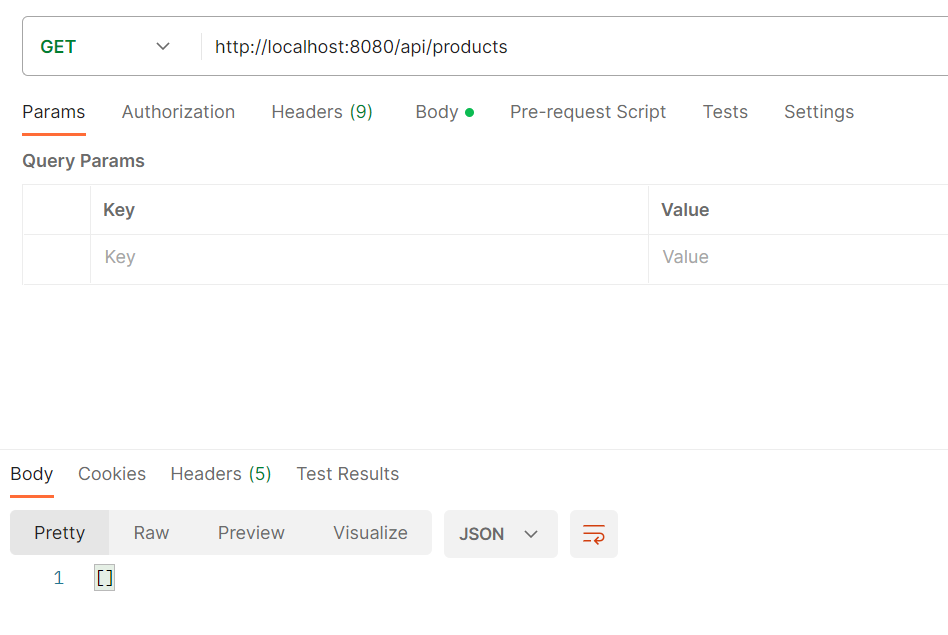
3. MySQL Workbench 확인하기
1. POST
- 저장한 마우스, 2000이 있는 걸 확인할 수 있다.
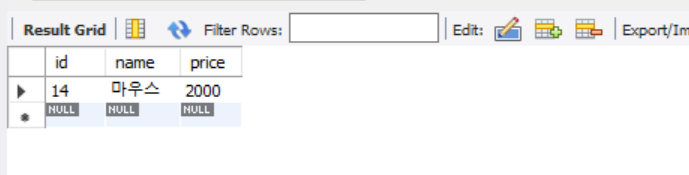
2. DELETE
- 저장한 상품을 삭제해서 아무것도 남아있지 않다.
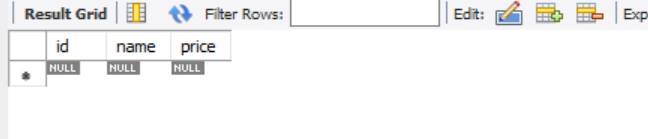