What is MONGO?
According to Mongo's homepage, it is "the most popular database for modern applications". It is commonly used in combination with Node.
Mongo is a document database, which we can use to store and retrieve complex data form.
정보를 저장해서 지속시켜야함. (데이터베이스의 기본)
Why use a Database? (Instead of just saving to a file?)
Databases can handle large amounts of data efficiently and store it compactly
They provide tools for easy insertion,querying,and updating of data.
They generally offer security features and control over access to data.
They (generally) scale well.
데이터베이스는 일반적으로 어마어마한 데이터를 효율적으로 저장하고 압축하여 관리하기 쉽고 접속하기 쉽게 만들어 줌. (데이터 사이즈를 더 작게 압축)
다양한 메소드와 언어로 된 수많은 도구와 레이어를 사용하면 데이터베이스 탑에서 데이터를 쉽게 삽입,문의,갱신,삭제 할 수 있음. (데이터 필터링 & 정렬 & 검색)
데이터베이스의 탑에는 레이어가 하나 있는데 데이터 관리 시스템이라고 불리운다.
이곳에서 보안기능이나 관리자로서의 접속을 누구에게 허용할지 제어하는 기능이 있음.
파일에 저장했을 때 보다 관리측면에서 조정과 관리가 훨씬 쉬움.
SQL (MySQL, Postgres, SQLite, Oracle, MS SQL Server)
NoSQL Database (MongoDB, CouchDB, Neo4j)
1) Homebrew 설치 - 모든 종류의 개발자 도구 설치를 도와주는 도구
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
2) brew tap mongodb/brew
3) brew install mongodb-community@6.0
4) brew services start mongodb-community@6.0
5) mongosh
show dbs
use (데이터베이스 이름)
Ctrl + c
Mongo가 데이터베이스 안에서 기대하는 타입의 데이터
출처: https://www.mongodb.com/json-and-bson
삽입 할 때 에는 '집합'의 형식으로
삽입 메소드 (insertOne, inserMany, insert)
- 'insert' 를 웬만하면 주로 사용
insertOne
insert
db.collection.find()
{ $set : { field1: value1, ... }}
db.deleteOne, deleteMany
db.cats.deleteMany( { } ) -> 전부 삭제
중첩되있을 때 데이터 찾기 (따옴표, 마침표)
$gt : (greater than) : '초과' 개념
$gte (greater than or equal) : '이상' 개념
$in (해당 특징이 들어간 데이터 찾기)
$nin (해당 특징이 없는 데이터 찾기)
$or ( 두개의 조건 중 어느 것에 해당하는 데이터 찾기)
O D M (Object Data Mapper or Object Document Mapper)
ODMs like Mongoose map documents coming from a database into usable Javascript objects.
Mongoose provides ways for us to model out our application data and define a schema. It offers easy ways to validate data and build complex queries from the comfort of JS.
Ex) Moongoose는 Mongo와 Node.js 를 연결해줌.
몽구스는 Node와 Mongo DB를 이어주는 기본 드라이버 그 이상.
JS 측면에서 아주 유용한 여러 기능 제공.
Mongo에서 회신,삽입하려는 데이터를 매핑시켜 메서드를 추가할 수 있는 사용가능한 JS 객체로 만듬.
또한 사전에 프리셋 스키마(Schema)를 정의 할 수 있으며 해당 데이터가 몽구스를 통해 레이아웃된 스키마를 따르도록 강제.
즉, Mongo를 개선시켜 JS측면에서 보다 친숙하고 강력하게 만든것. (데이터나 문서를 JS 객체로 매핑)
모든 툴은 기본적으로 데이터베이스를 번역해 사용하려는 프로그래밍 언어의 객체로 변환시켜 주는 것이다.
const mongoose = require('mongoose');
//몽구스 mongoDB에 연결하기 // movieApp은 데이터베이스 폴더명
mongoose.connect('mongodb://localhost:27017/movieApp')
.then(() => {
console.log("CONNECTION OPEN!")
}).catch(err => {
console("ERROR!" , err)
})
MODEL
Schema (Mongo의 각기 다른 키 집합을 JS의 다른 타입으로 구조를 짜는 것)
const movieSchema = new mongoose.Schema({
title: String,
year: Number,
score: Number,
rating: String
})
//Movie : 모델 이름 (단수형이면서 첫번째글자는 대문자로 작성해야함)
// mongoose는 Movie이름을 딴 'movies'라는 집합 생성 (자동으로 복수형이되고 첫글자 대문자로 만듦)
//집합이름: movies , 모델이름: Movie(타입은 클래스)
const Movie = mongoose.model('Movie', movieSchema)
데이터베이스에 실질적으로 저장하기
insertMany( )
MongDB에서 온 원본 결과를 다루거나 유효성 검사를 통과한 문서인 Promise를 반환합니다.
해당 메소드 사용할 땐 save 하지 않아도 됨.
모델의 '단일' 인스턴스를 생성할 경우에는 save 호출해서 데이터 베이스에 저장시켜야함.
하지만 insertMany를 호출하면 기본적으로 MongoDB에 바로 연결되어 한 번에 많이 입력할 수 있음.
Movie.find({})
findById( )
updateOne
updateMany( )
findOneAndUpdate( )
remove()
deleteMany()

findOneAndDelete ()
required : true;
const mongoose = require('mongoose');
//몽구스 mongoDB에 연결하기 // shopApp은 데이터베이스 폴더명
mongoose.connect('mongodb://localhost:27017/shopApp')
.then(() => {
console.log("CONNECTION OPEN!")
}).catch(err => {
console("ERROR!" , err)
})
const prodcutSchema = new mongoose.Schema({
name : {
type: String,
required :true
},
price : {
type: Number,
required : true
}
});
Default
maxlength
const bike = new Product ({name:'Bike Helmet form Hemlet makers', price: 599})
array 적용
const prodcutSchema = new mongoose.Schema({
name : {
type: String,
required :true,
maxlength: 20
},
price : {
type: Number,
required : true,
min : 0
},
onSale : {
type:Boolean,
default: false
},
categories : [String]
});
const Product = mongoose.model('Product', prodcutSchema);
const bike = new Product ({name:'Bike Helmet', price: 599, categories:['Cycling', 'Safety']})
중첩된 속성 넣기
const prodcutSchema = new mongoose.Schema({
name : {
type: String,
required :true,
maxlength: 20
},
price : {
type: Number,
required : true,
min : 0
},
onSale : {
type:Boolean,
default: false
},
categories : [String],
qty: {
online: {
type: Number,
default:0
},
inStore: {
type:Number,
default : 0
}
}
});
~~~
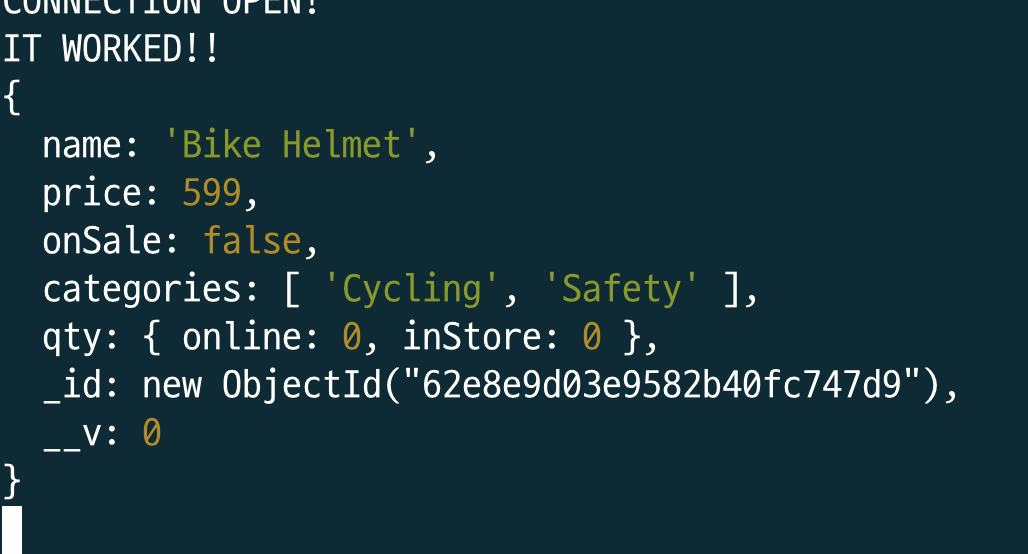
---
# 21) Mongoose 업데이트 유효성 검사하기
- 데이터베이스를 작업하는 많은 프로그램들이 뭔가를 만들면 유효성 검사가 자동으로 적용되는데 일시적으로 한 번 검사하고 말아버림.
- 계속해서 내가 적용한 스키마의 기준에 충족이 되는지에 대한 적합성 여부를 지속적으로 하고 싶다면
runValidator: truen 라고 적용해야함
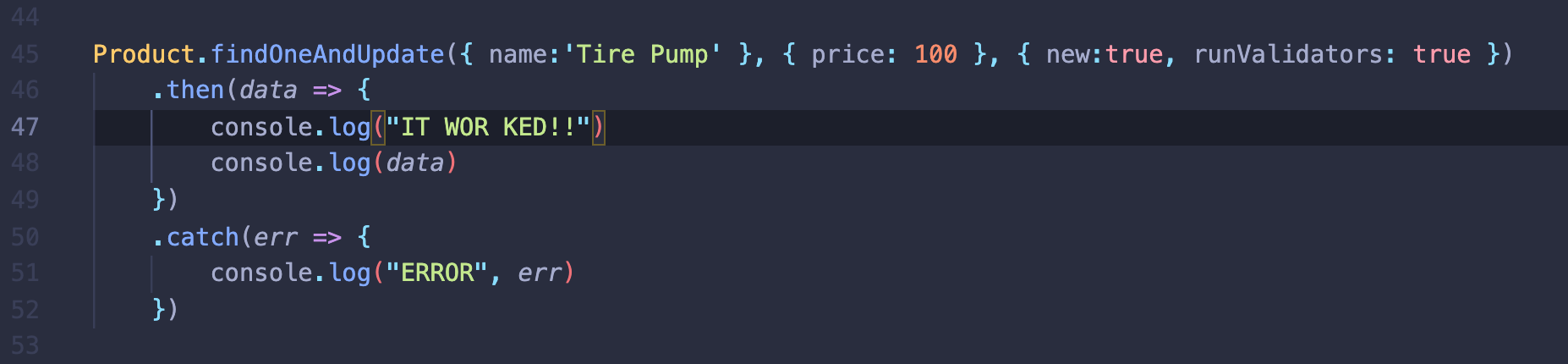
---
# 22) Mongoose 유효성 검사 오류
> enum
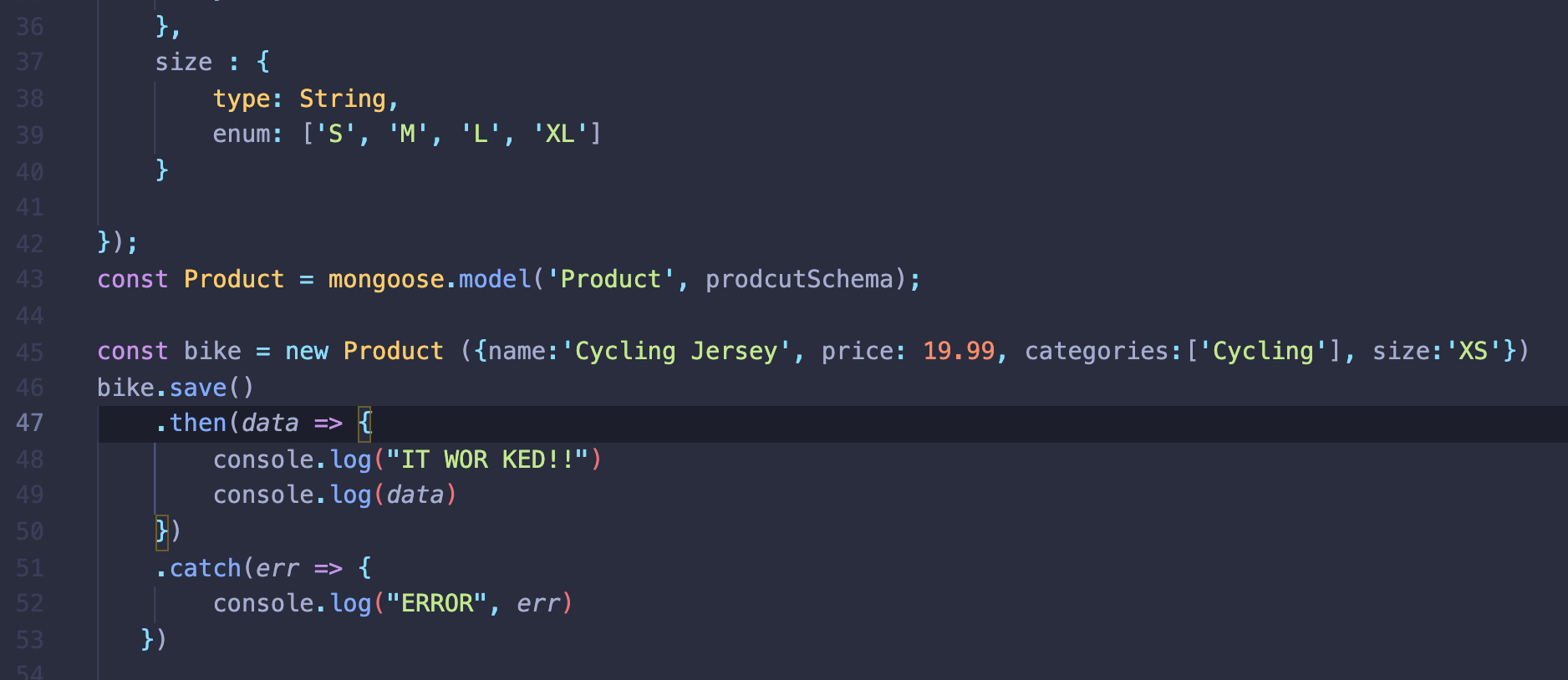

- enum: 선택지 안에서만 선택가능함 (S, M, L, XL) 의 선택지에서 XS를 선택해 오류발생.
---
# 23) 인스턴스 메소드
> 커스텀 메소드 스키마에 추가하기
- 정말 자주 쓰이며 Mongoose가 이미 제공하는 기능 외에 추가로 모델에 기능을 정의하거나 추가하는 방법.
- arrow(화살표)함수가 아닌 기존의 함수 표현식을 사용해야함 (중요) 화살표 함수는 함수에 대한 특정 값을 가질 수 없음.
> Product의 인스턴스 생성 _**(p)**_ - 방법1
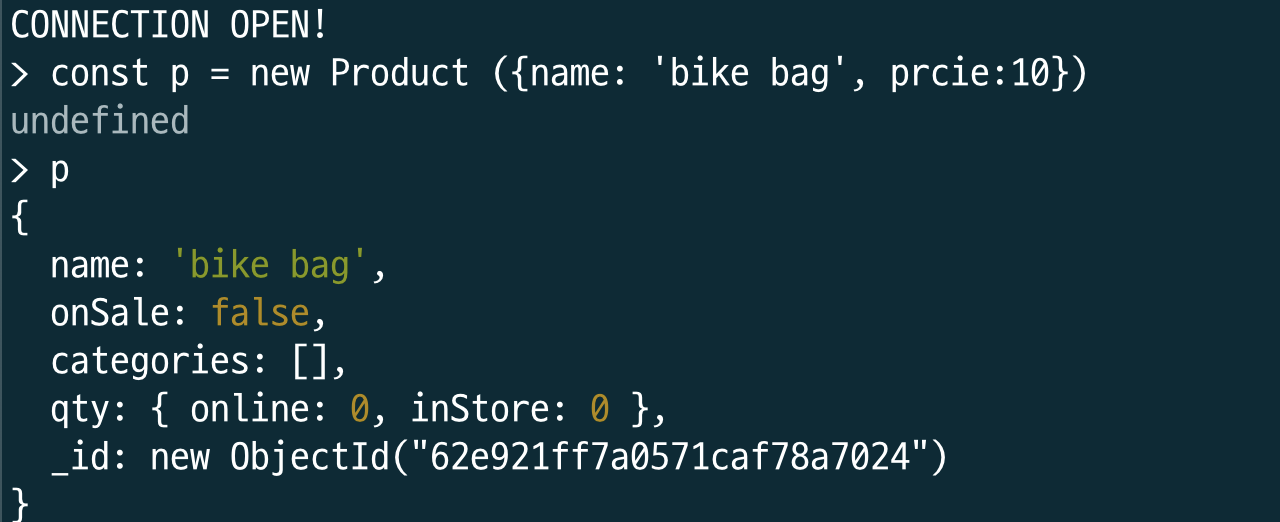
> 모든 각각의 Product 객체에서 엑세스 할 수 있는 함수 (greet)
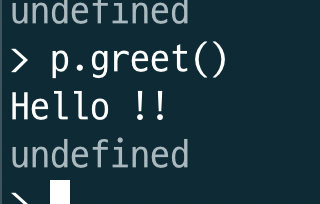
---
> 방법2
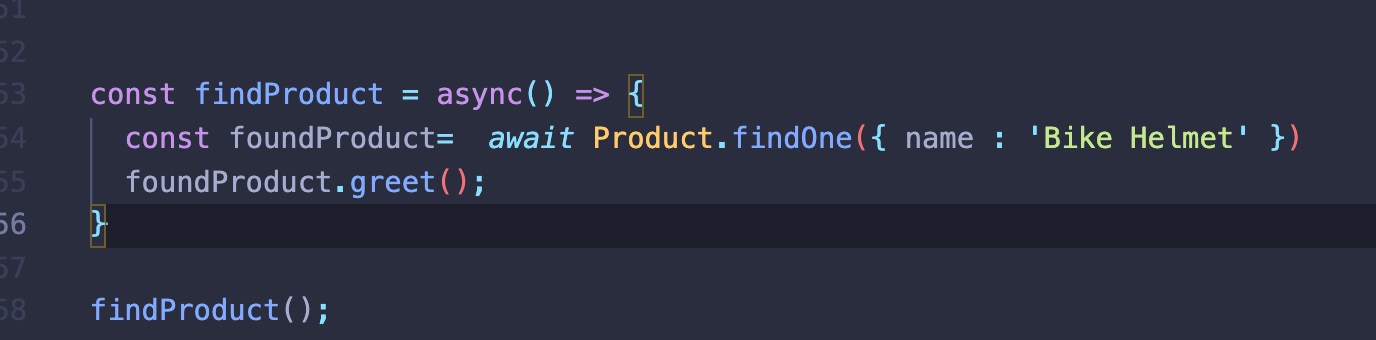
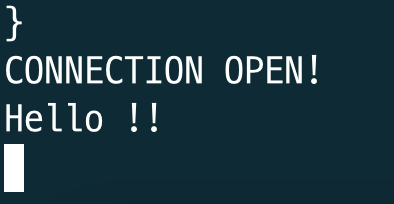
---
> this
const findProduct = async() => {
const foundProduct= await Product.findOne({ name : 'Bike Helmet' })
foundProduct.greet();
}
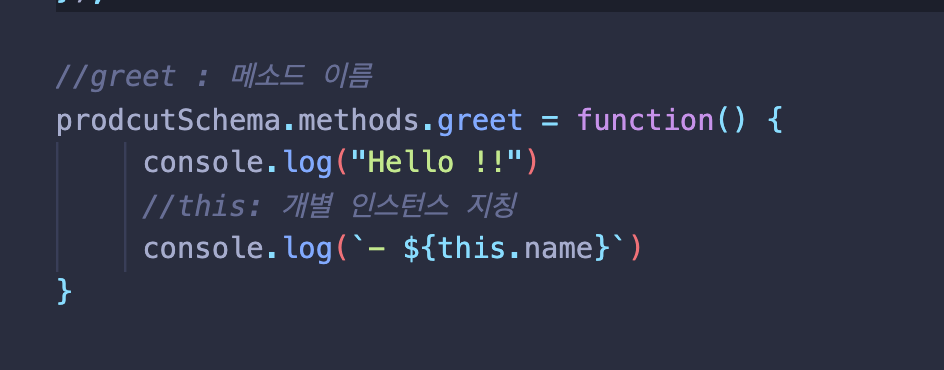
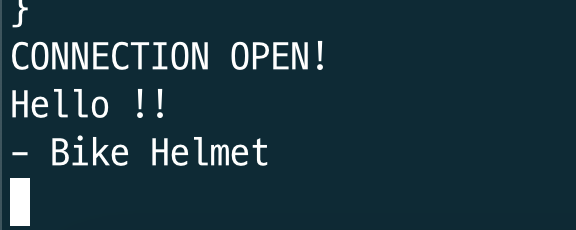
---
> 특정 상품에 toggle 메소드 적용
- 첫번째 'foundProduct'는 toggle 함수_** 전 **_(true)
- await founProduct.toggleOnSale(); 수행 후
- 두번째 'foundProduct'는 toggle 함수 _**후**_ (false)
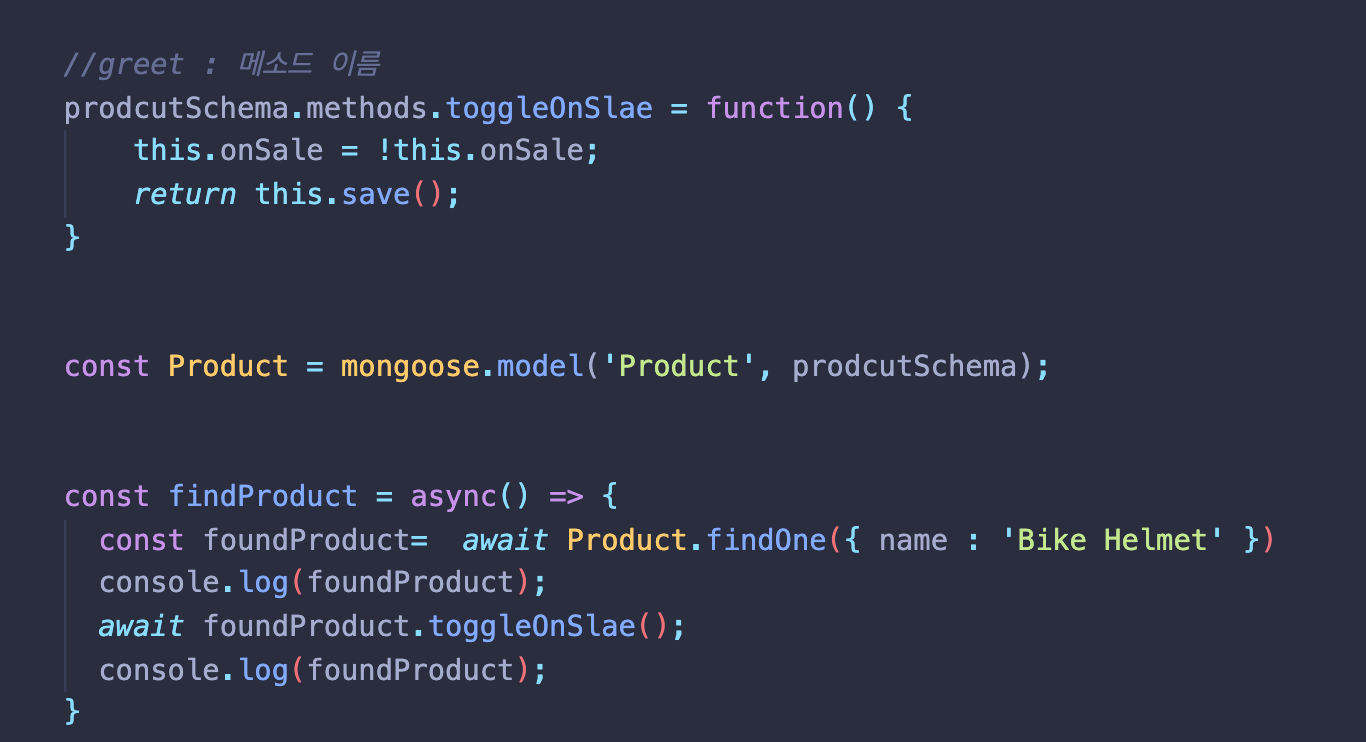
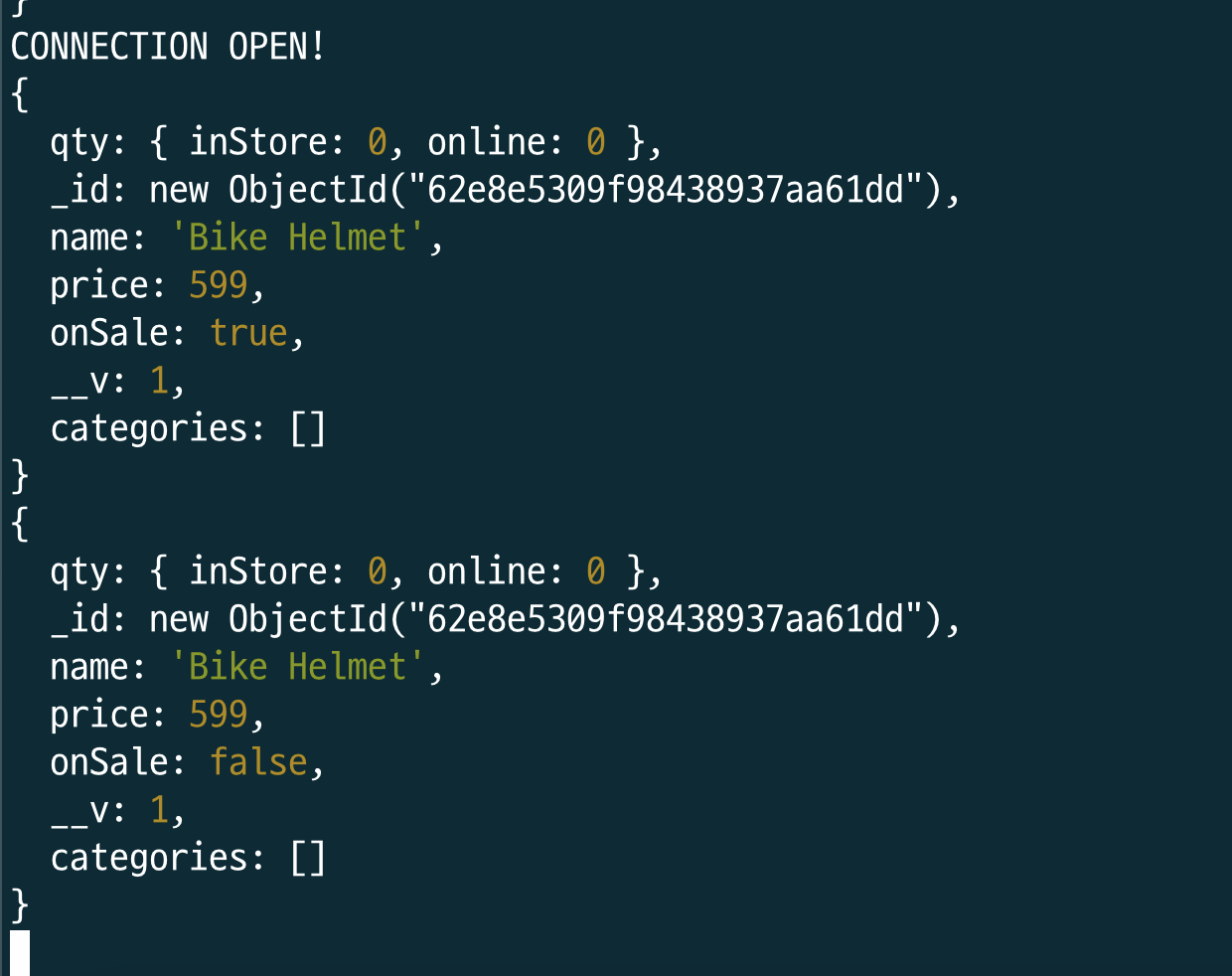
---
const Product = mongoose.model('Product', productSchema);
> 일반적으로 데이터를 조작하거나 모델에 포함된 정보와 직접 관련된 작업이라면 모델에 넣는다.
---
# 24) 정적 메소드 추가하기
> 인스턴스가 아닌 '모델' 자체에 적용되는 정적 메소드
- 정적메소드는 개별 인스턴스에서 작동되는게 X
- 인스턴스 메소드는 this를 사용해 객체를 가르켰다면 정적 메소드는 this 자체적으로 해당 모델클래스를 가르킨다.


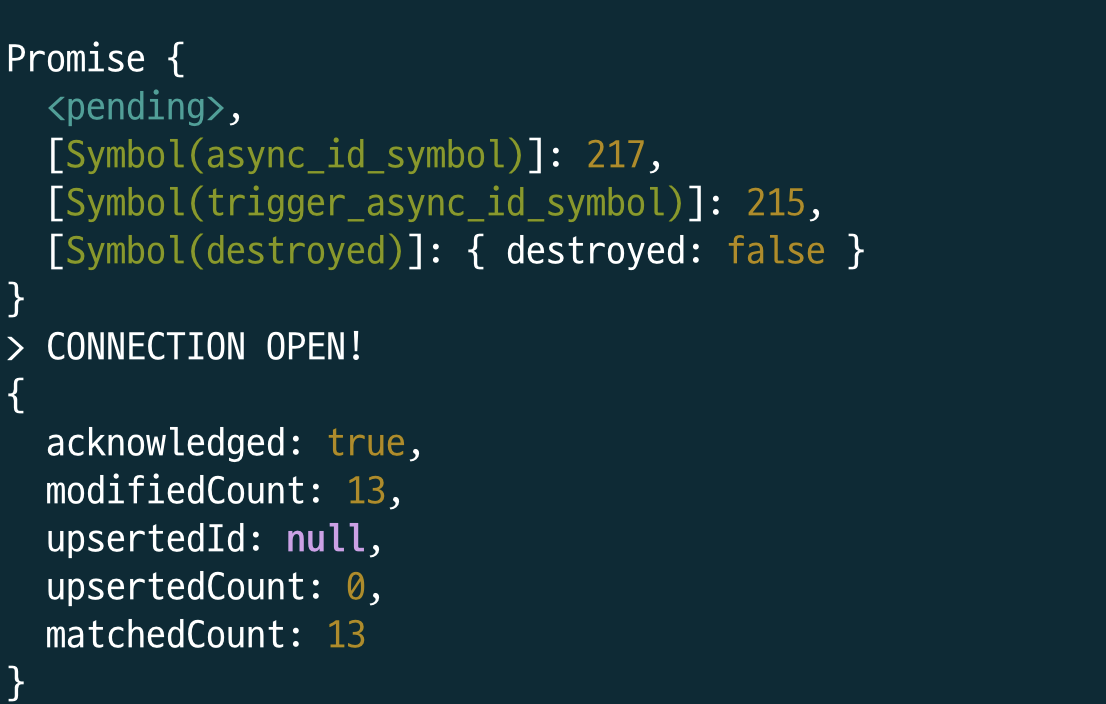
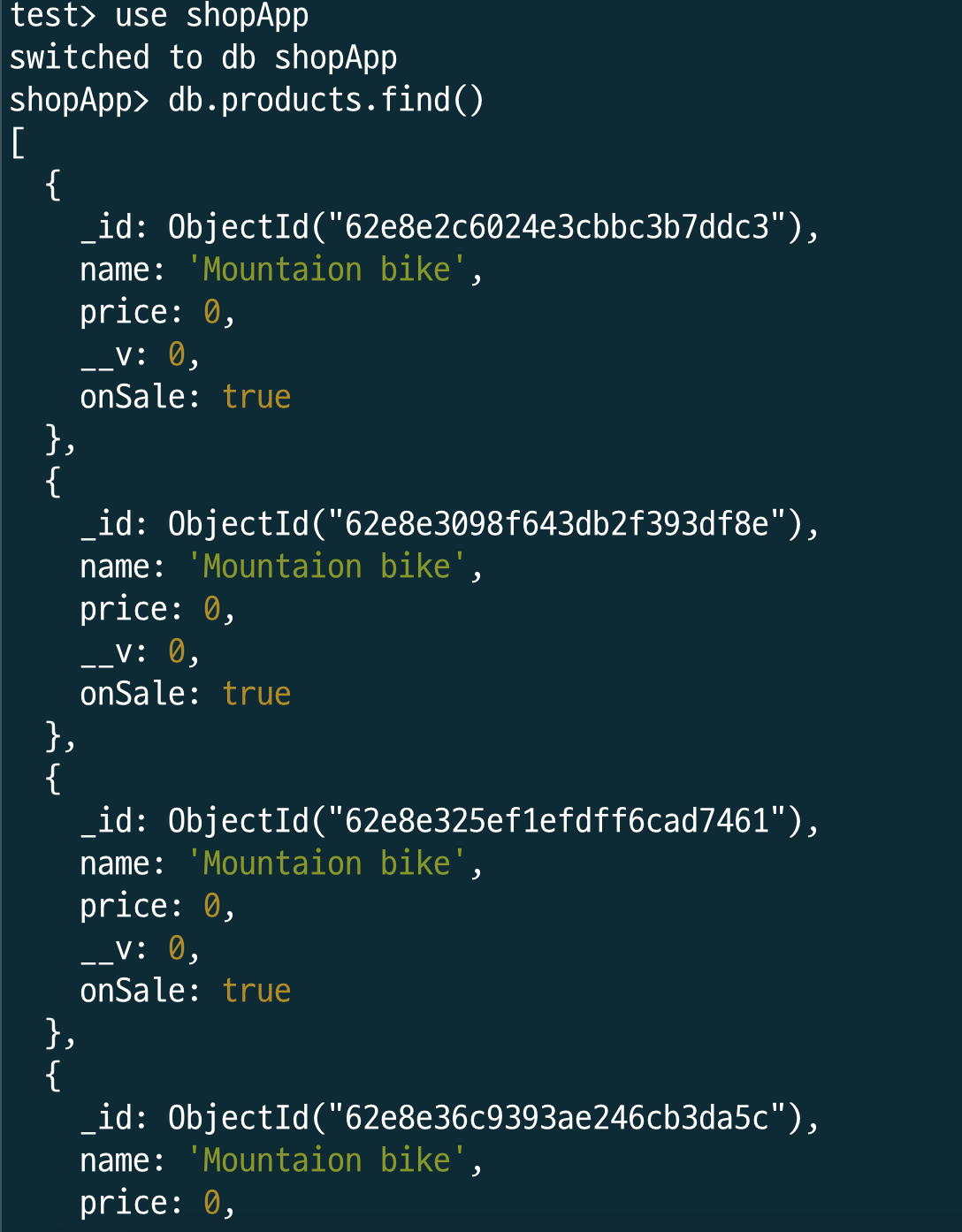
- 가격이 모두 제로로 변했음.
- 클래스나 모델 자체에 커스텀하고 메소드 적용
- 다시말해 fireSale의 static 메소드는 특정한 모델 'Product'와 직접적으로 관련이 있는것이 아닌 전체의 Product 모델과 관련있는 것이다.
- 보통 모델의 정적메소드는 _**항목찾기,업데이트,생성, 삭제**_등의 업무를 볼 때 유용한 방식.
- Ex) Products.find, Products.update
- 따라서 정적메소드 안에서의 this는 어느 특별한 인스턴스를 가르키는 것이 아닌 모델 _**'전체'**_를 가르킴.
---
# 25) 가상 Mongoose
> 실제 데이터베이스 자체에는 존재하지 않는 스키마에 특성을 추가할 수 있게함.
- 포인트는 데이터베이스 내부에 존재하는 것이 아닌 JS의 Mongoose 에서만 가능.
- 일반적으로 접근하는 정보를 가질 때 사용.
- 기존 데이터에서 가져와서 데이터베이스에 저장 가능.
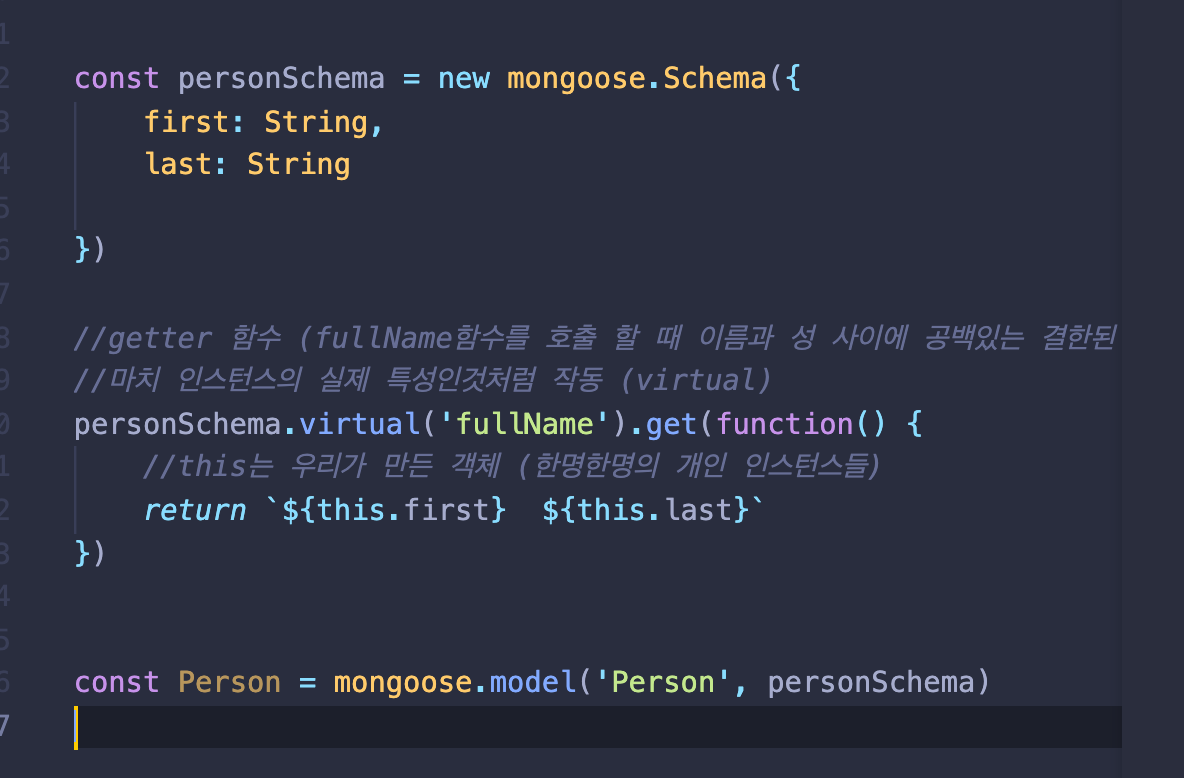
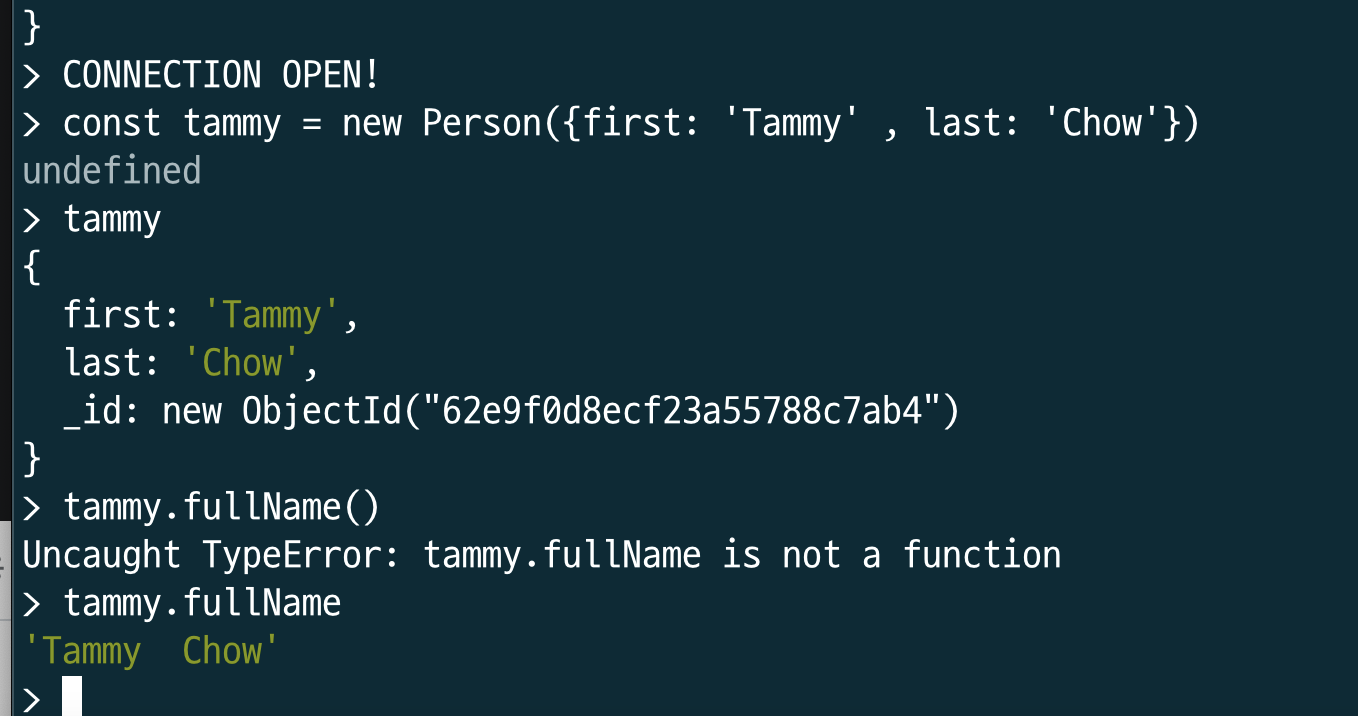
----