MNIST
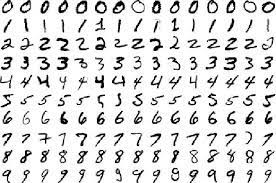
- 0 ~ 9 의 흑백으로 된 손글씨 숫자를 7만개 정도를 보유하고 있다.
- 이미지 한장의 크기는 28 * 28 크기를 지니고 있다.
실습
import
import tensorflow as tf
Data_load
mnist = tf.keras.datasets.mnist
(x_train , y_train) , (x_test , y_test) = mnist.load_data()
x_train.shape , y_train.shape , x_test.shape , y_test.shape

- image 분류 할때 가장 중요한점은 input_shape 을 반드시 확인하는것이다
모델을 학습 할때 이미지 데이터의 크기가 안맞으면 학습이 안되기 때문에 반드시 데이터 들의 크기를 확인하는것이 중요하다
Data PreProcessing
x_train , x_test = x_train / 255.0 , x_test / 255.0
- 데이터를 0 과 1 사이로 하는 min-max scaler 수행
- 안정성 + 모델 일반화에 도움을 준다
Model Composition
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28,28)),
tf.keras.layers.Dense(512 , activation = 'relu'),
tf.keras.layers.Dense(10 , activation='softmax')
])
Model Compile
model.compile(optimizer = 'adam' , loss='sparse_categorical_crossentropy',metrics = ['accuracy'])
Model fit
model.fit(x_train , y_train , epochs=5)
test Evaluate
test_loss , test_acc = model.evaluate(x_test , y_test)
print(f'test 정확도 :{test_acc}')
print(f'test loss : {test_loss}')