원하는 상황
- 시상 카드를 잡는다
- 시상 카드가 사라진다.
- bgm이 시작된다
- 후보자 카드가 5초에 한번씩 나오며 회전한다.
- 상장을 잡으면, BGM과 후보자 카드가 사라진다.
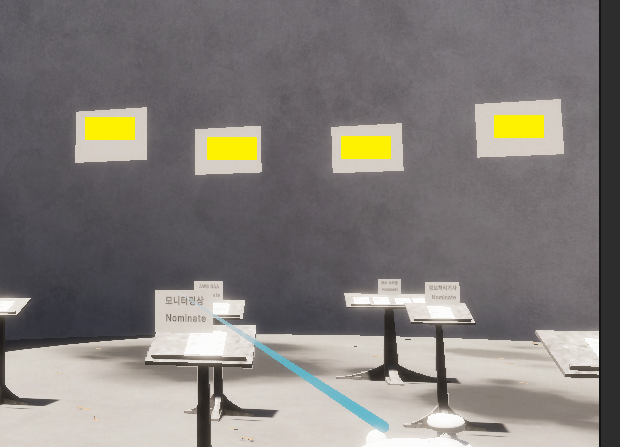
스크립트
- 시상 카드를 잡는다 => 시상카드 parentsObject
- 시상 카드가 사라진다. => OnSelectEntered 메소드
- bgm이 시작된다 => OnSelectEntered 메소드
- 후보자 카드가 5초에 한번씩 나오며 회전한다. => Update 메소드
- 상장을 잡으면, BGM과 후보자 카드가 사라진다. => OnGrabbed 메소드
using Oculus.Interaction;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.XR.Interaction.Toolkit;
using static Meta.WitAi.Data.AudioEncoding;
public class TouchNominate : MonoBehaviour
{
public GameObject parentsObject;
public GameObject awardObject;
public GameObject[] objects;
public GameObject newBGM;
private AudioSource previousAudio;
public float radius = 1f;
public float speed = 4f;
private float angle = 0f;
private XRSimpleInteractable interactable;
private XRGrabInteractable grabInteractable;
public float spawnInterval = 5f;
private int currentObjectIndex = 0;
private bool isSpawning = false;
private void Start()
{
interactable = parentsObject.GetComponent<XRSimpleInteractable>();
grabInteractable = awardObject.GetComponent<XRGrabInteractable>();
if (interactable != null)
{
interactable.selectEntered.AddListener(OnSelectEntered);
}
else
{
Debug.LogError("XRSimpleInteractable 컴포넌트를 찾을 수 없습니다.");
}
if (grabInteractable != null)
{
grabInteractable.selectEntered.AddListener(OnGrabbed);
}
else
{
Debug.LogError("XRGrabInteractable 컴포넌트를 찾을 수 없습니다.");
}
foreach (GameObject obj in objects)
{
obj.SetActive(false);
}
for (int i = 0; i < objects.Length; i++)
{
objects[i].transform.position = new Vector3(parentsObject.transform.position.x, parentsObject.transform.position.y - 0.5f, parentsObject.transform.position.z );
}
}
private void OnSelectEntered(SelectEnterEventArgs args)
{
if (previousAudio != null)
{
previousAudio.Stop();
}
newBGM.SetActive(true);
AudioSource newAudio = newBGM.GetComponent<AudioSource>();
if (newAudio != null)
{
newAudio.Play();
previousAudio = newAudio;
}
else
{
Debug.LogError("오디오 소스를 찾을 수 없습니다.");
}
parentsObject.GetComponent<MeshRenderer>().enabled = false;
Transform titleTransform = parentsObject.transform.Find("Title");
Transform contentTransform = parentsObject.transform.Find("Contents");
if (titleTransform != null)
{
titleTransform.gameObject.SetActive(false);
}
else
{
Debug.LogError("Title GameObject를 찾을 수 없습니다.");
}
if (contentTransform != null)
{
contentTransform.gameObject.SetActive(false);
}
else
{
Debug.LogError("Contents GameObject를 찾을 수 없습니다.");
}
if (!isSpawning)
{
StartCoroutine(SpawnObjects());
}
}
private IEnumerator SpawnObjects()
{
isSpawning = true;
while (currentObjectIndex < objects.Length)
{
objects[currentObjectIndex].SetActive(true);
yield return new WaitForSeconds(spawnInterval);
currentObjectIndex++;
}
isSpawning = false;
}
private void OnGrabbed(SelectEnterEventArgs args)
{
if (newBGM != null)
{
newBGM.GetComponent<AudioSource>().Stop();
}
parentsObject.SetActive(false);
}
void Update()
{
angle += speed * Time.deltaTime;
float radian = angle * Mathf.Deg2Rad;
for (int i = 0; i < currentObjectIndex; i++)
{
float offset = i * 1.5f * Mathf.PI / objects.Length;
float x = Mathf.Cos(radian + offset) * radius + parentsObject.transform.position.x;
float z = Mathf.Sin(radian + offset) * radius + parentsObject.transform.position.z;
objects[i].transform.position = new Vector3(x - 1.8f, parentsObject.transform.position.y + 0.8f, z);
}
}
}