DataBindingClassView.vue
<template>
<div>
<div :class="{'text-red': hasError, active: isActive}">클래스 바인딩</div>
<div :class="class2">또 다른 클래스 바인딩</div>
</div>
</template>
<script>
export default {
data() {
return {
isActive: false,
hasError: true,
class2: ['active', 'text-red']
}
}
}
</script>
<style scoped>
.active {
background-color: greenyellow;
font-weight: bold;
}
.text-red {
color: red;
}
</style>
style scoped
- scoped 넣어줘야 현재 파일에서만 스타일 적용
- 안 넣으면 글로벌 적용
:class
객체 직접 작성하여 동적으로 클래스 생성
- :class="{ }" 중괄호 안에 '클래스 이름: bool' 작성
- 일반 단어면 ' '생략 가능 / text-red 처럼 - 있으면 ' '로 감싸주기
- bool 값은 직접 T/F 넣어줘도 되고, 데이터 바인딩도 가능
배열 데이터 바인딩
- data 배열에 넣고싶은 클래스 모두 작성
- :class="class1" 처럼 따옴표 안에 그대로 작성

DataBindingStyleView.vue
<template>
<div>
<div style="color: red; font-size: 30px">스타일 바인딩: red color, 30px font</div>
<div :style="style1">스타일 바인딩: green color, 30px font</div>
<button @click="style1.color='blue'">색상 바꾸기</button>
</div>
</template>
<script>
export default {
data() {
return {
style1: {
color: 'green',
fontSize: '30px'
}
}
}
}
</script>
<style>
</style>
style 직접 작성
- HTML와 같이 style=" "안에 css 작성
:style 객체 데이터 바인딩
- data에 style1을 객체 형식으로 작성
- css처럼 작성하되 camelCase로 작성하기!!!
- 값은 ' '로 묶어주기
- :style="style1" 처럼 그대로 적용
@click으로 색상 변경
- method를 이용해도 되지만 직접 변경도 가능
- @click="style1.color='blue'"
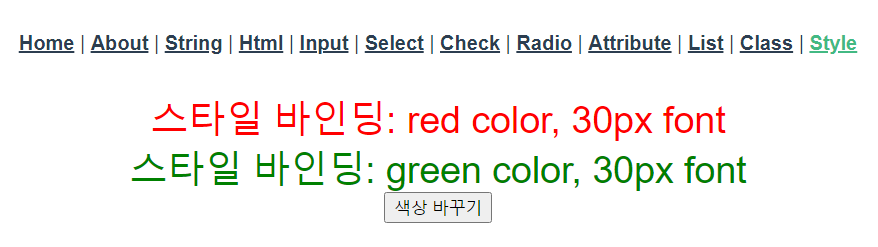
App.vue
<template>
<nav>
<router-link to="/">Home</router-link> |
<router-link to="/about">About</router-link> |
<router-link to="/databinding/string">String</router-link> |
<router-link to="/databinding/html">Html</router-link> |
<router-link to="/databinding/input">Input</router-link> |
<router-link to="/databinding/select">Select</router-link> |
<router-link to="/databinding/check">Check</router-link> |
<router-link to="/databinding/radio">Radio</router-link> |
<router-link to="/databinding/attribute">Attribute</router-link> |
<router-link to="/databinding/list">List</router-link> |
<router-link to="/databinding/class">Class</router-link> |
<router-link to="/databinding/style">Style</router-link>
</nav>
<router-view/>
</template>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
nav {
padding: 30px;
}
nav a {
font-weight: bold;
color: #2c3e50;
}
nav a.router-link-exact-active {
color: #42b983;
}
</style>
router/index.js
import { createRouter, createWebHistory } from 'vue-router'
import HomeView from '../views/HomeView.vue'
const routes = [
{
path: '/',
name: 'home',
component: HomeView
},
{
path: '/about',
name: 'about',
component: () => import( '../views/AboutView.vue')
},
{
path: '/databinding/string',
name: 'DataBindingStringView',
component: () => import( '../views/1_databinding/DataBindingStringView.vue')
},
{
path: '/databinding/html',
name: 'DataBindingHtmlView',
component: () => import( '../views/1_databinding/DataBindingHtmlView.vue')
},
{
path: '/databinding/input',
name: 'DataBindingInputView',
component: () => import( '../views/1_databinding/DataBindingInputView.vue')
},
{
path: '/databinding/select',
name: 'DataBindingSelectView',
component: () => import( '../views/1_databinding/DataBindingSelectView.vue')
},
{
path: '/databinding/check',
name: 'DataBindingCheckboxView',
component: () => import( '../views/1_databinding/DataBindingCheckboxView.vue')
},
{
path: '/databinding/radio',
name: 'DataBindingRadioView',
component: () => import( '../views/1_databinding/DataBindingRadioView.vue')
},
{
path: '/databinding/attribute',
name: 'DataBindingAttributeView',
component: () => import( '../views/1_databinding/DataBindingAttributeView.vue')
},
{
path: '/databinding/list',
name: 'DataBindingListView',
component: () => import( '../views/1_databinding/DataBindingListView.vue')
},
{
path: '/databinding/class',
name: 'DataBindingClassView',
component: () => import( '../views/1_databinding/DataBindingClassView.vue')
},
{
path: '/databinding/style',
name: 'DataBindingStyleView',
component: () => import( '../views/1_databinding/DataBindingStyleView.vue')
}
]
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes
})
export default router